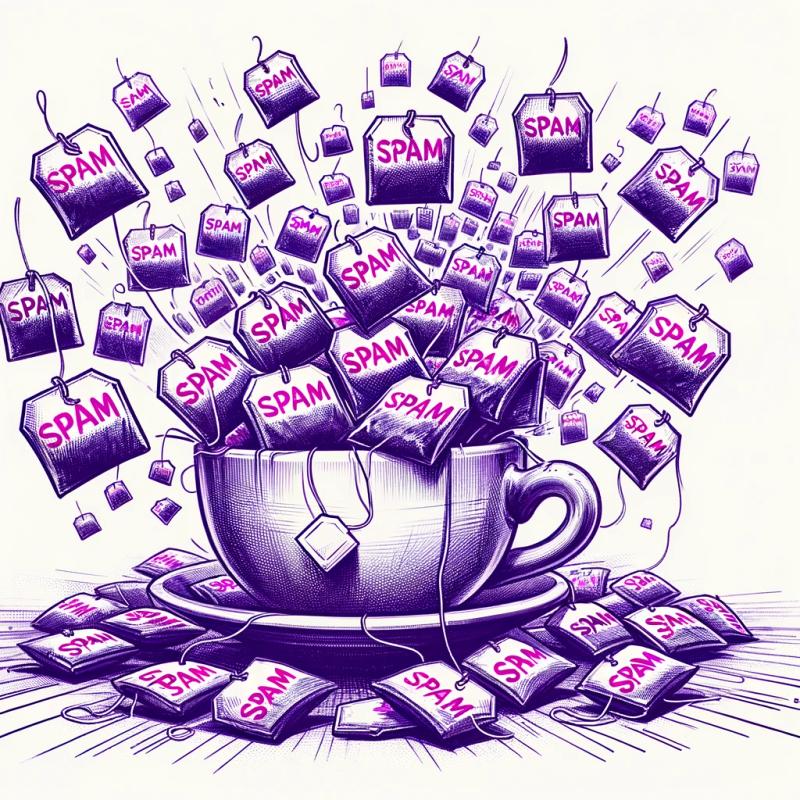
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
atomic-router
Advanced tools
Readme
Simple routing implementation that provides abstraction layer instead of inline URL's and does not break your architecture
history
instance and it works everywhere)❗️ Attention: At the moment atomic-router team collecting issues and feature requests to improve current design. Upgrade atomic-router version with caution. We are going to write migration guide when/if the release will contain breaking changes. Thank you for reporting issues 🧡
$ npm install effector atomic-router
Create your routes wherever you want:
// pages/home
import { createRoute } from 'atomic-router';
export const homeRoute = createRoute();
// pages/posts
import { createRoute } from 'atomic-router';
export const postsRoute = createRoute<{ postId: string }>();
And then create a router
// app/routing
import { createHistoryRouter } from 'atomic-router';
import { createBrowserHistory, createMemoryHistory } from 'history';
import { homeRoute } from '@/pages/home';
import { postsRoute } from '@/pages/posts';
const routes = [
{ path: '/', route: homeRoute },
{ path: '/posts', route: postsRoute },
];
const router = createHistoryRouter({
routes: routes,
});
// Attach history
const history = isSsr ? createMemoryHistory() : createBrowserHistory();
router.setHistory(history);
There are 3 purposes for using atomic routes:
export const getPostFx = createEffect<{ postId: string }, Post>(
({ postId }) => {
return api.get(`/posts/${postId}`);
}
);
export const $post = restore(getPostFx.doneData, null);
postPage.$params
change://route.ts
import { createRoute } from 'atomic-router';
import { getPostFx } from './model';
const postPage = createRoute<{ postId: string }>();
sample({
source: postPage.$params,
filter: postPage.$isOpened,
target: getPostFx,
});
Imagine that we have a good architecture, where our code can be presented as a dependency tree.
So, we don't make neither circular imports, nor they go backwards.
For example, we have Card -> PostCard -> PostsList -> PostsPage
flow, where PostsList
doesn't know about PostsPage
, PostCard
doesn't know about PostsList
etc.
But now we need our PostCard
to open PostsPage
route.
And usually, we add extra responisbility by letting it know what the route is
const PostCard = ({ id }) => {
const post = usePost(id);
return (
<Card>
<Card.Title>{post.title}</Card.Title>
<Card.Description>{post.title}</Card.Description>
{/* NOOOO! */}
<Link to={postsPageRoute} params={{ postId: id }}>
Read More
</Link>
</Card>
);
};
With atomic-router
, you can create a "personal" route for this card:
const readMoreRoute = createRoute<{ postId: id }>();
And then you can just give it the same path as your PostsPage
has:
const routes = [
{ path: '/posts/:postId', route: readMoreRoute },
{ path: '/posts/:postId', route: postsPageRoute },
];
Both will work perfectly fine as they are completely independent
// Params is an object-type describing query params for your route
const route = createRoute<Params>();
// Stores
route.$isOpened; // Store<boolean>
route.$params; // Store<{ [key]: string }>
route.$query; // Store<{ [key]: string }>
// Events (only watch 'em)
route.opened; // Event<{ params: RouteParams, query: RouteQuery }>
route.updated; // Event<{ params: RouteParams, query: RouteQuery }>
route.closed; // Event<{ params: RouteParams, query: RouteQuery }>
// Effects
route.open; // Effect<RouteParams>
route.navigate; // Effect<{ params: RouteParams, query: RouteQuery }>
// Note: Store, Event and Effect is imported from 'effector' package
FAQs
Simple routing implementation that provides abstraction layer instead of inline URL's and does not break your architecture
The npm package atomic-router receives a total of 385 weekly downloads. As such, atomic-router popularity was classified as not popular.
We found that atomic-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.