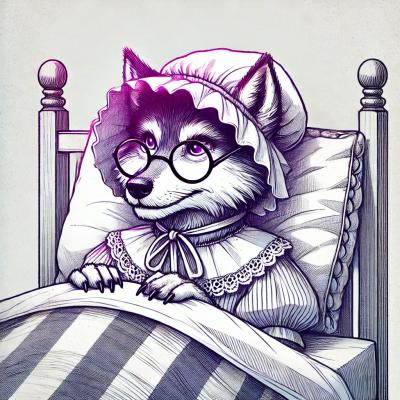
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The auto-bind npm package is used to automatically bind methods of a class to their instance. This is particularly useful in React components to avoid having to manually bind 'this' in the constructor for every method that needs it.
Automatic binding of class methods
This feature automatically binds all methods of a class instance to the instance itself, ensuring that 'this' within the methods refers to the class instance even when the method is extracted and called separately.
const autoBind = require('auto-bind');
class MyClass {
constructor() {
autoBind(this);
}
myMethod() {
// method body that uses 'this'
}
}
const instance = new MyClass();
const { myMethod } = instance;
myMethod(); // 'this' is correctly bound to the instance
bind-methods is a package that binds an object's methods to itself. It is similar to auto-bind but does not automatically exclude constructor, React lifecycle methods, or methods already bound. It requires you to specify which methods to bind.
class-autobind is another package that offers automatic binding of class methods to the instance. It is similar to auto-bind but is implemented as a decorator, which can be used in projects that support JavaScript decorators.
react-autobind is designed specifically for React components to automatically bind methods to the component instance. It is similar to auto-bind but is tailored for React and can be more convenient for React developers.
Automatically bind methods to their class instance
$ npm install auto-bind
const autoBind = require('auto-bind');
class Unicorn {
constructor(name) {
this.name = name;
autoBind(this);
}
message() {
return `${this.name} is awesome!`;
}
}
const unicorn = new Unicorn('Rainbow');
// Grab the method off the class instance
const message = unicorn.message;
// Still bound to the class instance
message();
//=> 'Rainbow is awesome!'
// Without `autoBind(this)`, the above would have resulted in
message();
//=> Error: Cannot read property 'name' of undefined
Bind methods in self
to their class instance. Returns the self
object.
Type: Object
Object with methods to bind.
Type: Object
Type: Array<string|RegExp>
Bind only the given methods.
Type: Array<string|RegExp>
Bind methods except for the given methods.
Same as autoBind
, but excludes the default React component methods.
class Foo extends React.Component {
constructor(props) {
super(props);
autoBind.react(this);
}
// …
}
MIT © Sindre Sorhus
FAQs
Automatically bind methods to their class instance
The npm package auto-bind receives a total of 1,648,285 weekly downloads. As such, auto-bind popularity was classified as popular.
We found that auto-bind demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.