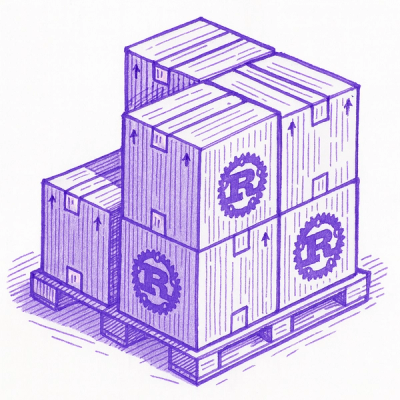
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
b-validate
Advanced tools
Javascript type validate.
npm i b-validate
import bv from 'b-validate';
bv(123)
.number.min(2)
.max(10)
.collect((error) => {
console.log(error); // { value: 123, type: 'number', message: '123 is not less than 10' }
// if no error, error equal to null
});
// or get error message like this:
const error = bv('b-validate').string.isRequired.match(/Validator/).end;
// { value: 'b-validate', type: 'string', message: '`b-validate` is not match pattern /Validator/' }
import { Schema } from 'b-validate';
const schema = new Schema({
name: [
{
type: 'string',
required: true,
message: '必填字段',
},
{
type: 'string',
maxLength: 10,
message: '最大长度是10',
},
],
age: [
{
type: 'number',
min: 2,
max: 5,
message: '在2和5之间',
},
],
email: [
{
type: 'email',
message: '邮箱格式不对',
},
],
ip: [
{
type: 'ip',
message: 'ip格式不对',
},
],
url: [
{
type: 'url',
message: 'url格式不对',
},
],
custom: [
{
validator: (value, callback) => {
if (value > 10) {
callback('不能大于10!');
}
},
},
],
// Async validate
async: [
{
validator: async (value, callback) => {
if (value > 10) {
callback('不能大于10!');
}
},
},
],
});
schema.validate(
{
name: 'pengjiyuan is a nice boy',
age: 24,
email: 'pengjiyuan@bytedance.com',
ip: '127.0.0.1',
url: 'https://bytedancecom',
custom: 20,
async: 20,
},
(errors) => {
console.log(errors);
/*
* {
* value: 'pengjiyuan is a nice boy', type: 'string', message: '最大长度是10' },
* age: { value: 24, type: 'number', message: '在2和5之间' },
* url: { value: 'https://bytedancecom', type: 'url', message: 'url格式不对' },
* custom: { value: 20, type: 'custom', message: '不能大于10!' }
* }
*/
}
);
Customize the validate messages by options.validateMessages
or (new schema({})).messages
/ bv.setGlobalConfig({ validateMessages: {} })
.
import bv from 'b-validate';
// set validateMessages for specific validator
const error = bv('', {validateMessages: {required: '必须有值'}}).string.isRequired.end.message;
// output: 必须有值
import bv from 'b-validate';
// set global validatemessages
bv.setGlobalConfig({ validateMessages: {required: '必须有值'} });
const error = bv('').string.isRequired.end.message;
// output: 必须有值
import bv from 'b-validate';
const error = bv('', {validateMessages: {required: '必须有值'}}).string.isRequired.end.message;
// output: 必须有值
import { Schema } from 'b-validate';
import zhCN from 'b-validate/es/locale/zh-CN';
const schema = new Schema({
name: [{ type: 'string', length: 4 }],
});
schema.messages(zhCN);
schema.validate({name: 'aaa'}, errors => {
console.log(errors);
// {name: { message: '字符数必须是 4' }}
})
Validate string's max length.
Validate string's min length.
Validate string's length
Validate whether string is match regex pattern.
Validate whether string is uppercase.
Validate whether string is lowercase.
bv('vvvv').string.isRequired.minLength(2).maxLength(10).lowercase.end; // pass
Minimum value.
Maximum value.
Equal to number.
In range min ~ max.
Is it a positive number?
Is it a negative number?
bv(123).number.min(2).max(250).positive.end; // pass
Validate array's length
Whether array includes some array.
Deep equal to other array
bv([1, 2, 3]).array.length(3).includes([1, 2]).deepEqual([1, 2, 3]).end; // pass
Object has some keys?
Deep equal to other object.
Empty object.
bv({ a: 1, b: 2 }).object.hasKeys(['a', 'b']).deepEqual({ a: 1, b: 2 }).not.empty.end; // pass
bv('abc@qq.com').type.email.end; // pass
bv('https://baidu.com').type.url.end; // pass
bv('127.0.0.1').type.ip.end; // pass
bv('xxxxxx').custom.validate(
(value, callback) => {
if (value !== 'x') {
callback(`Expect x but got ${value}`);
}
},
(error) => {
console.log(error); // { value: 'xxxxxx', type: 'string', message: 'Expect x but got xxxxxx' }
}
);
bv('xxxxxx').custom.validate(
async (value, callback) => {
const apiValue = api.getValue();
if (value !== apiValue) {
callback(`Expect x but got ${apiValue}`);
}
},
(error) => {
console.log(error);
}
);
FAQs
Javascript type validate
The npm package b-validate receives a total of 14,559 weekly downloads. As such, b-validate popularity was classified as popular.
We found that b-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.