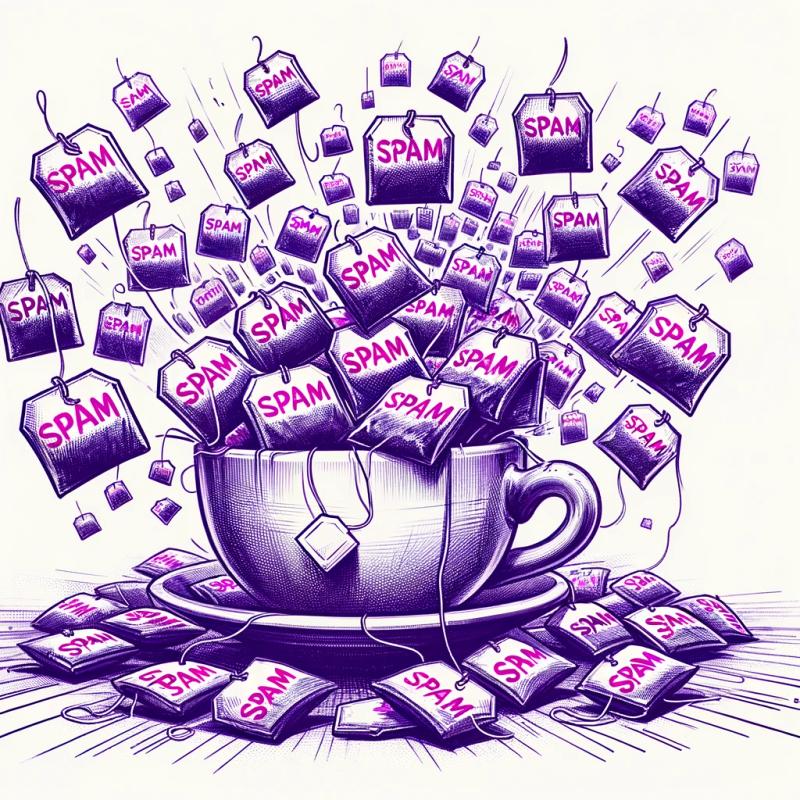
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
backbone-articulation
Advanced tools
Readme
+-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-+
|B||a||c||k||b||o||n||e||-||A||r||t||i||c||u||l||a||t||i||o||n||.||j||s|
+-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-++-+
Backbone-Articulation.js enhances Backbone.js model attributes with object serialization and deserialization.
#Download Latest (0.3.4):
Please see the release notes for upgrade pointers.
###Module Loading
Backbone-Articulation.js is compatible with RequireJS, CommonJS, Brunch and AMD module loading. Module names:
Due to monkey patching, you must include the dependencies in this order:
class SomeClass
constructor: (int_value, string_value, date_value) ->
this.int_value = int_value;
this.string_value = string_value;
this.date_value = date_value;
toJSON: ->
return {
_type:'SomeClass',
int_value:this.int_value,
string_value:this.string_value,
date_value:JSONS.serialize(this.date_value)
}
@fromJSON: (json) -> # note: this is a class method
if (json._type!='SomeClass') return null;
return new SomeClass(json.int_value, json.string_value, JSONS.deserialize(json.date_value));
Then if you put an instance in your model's attributes, it automatically gets serialized and deserialized for you:
instance = new Backbone.Model({id: 'spiffy'});
instance.save({embedded_some_class: new SomeClass(1, 'two', new Date())})
instance2 = new Backbone.Model({id: 'spiffy'});
instance2.fetch({
success: -> eq(_.isEqual(instance.get('embedded_some_class').toJSON(), instance2.get('embedded_some_class').toJSON()), "automatically serialized and deserialized a class!")
})
Note: You can use heterogenous lifecycles paradigms in the same model's attributes and even embed Backbone models in your attributes (by adding type attribute)!
If you choose 2a) - take a look at the examples below and:
Backbone.Articulation.TYPE_UNDERSCORE_SINGULARIZE = true;
That's it! Go crazy!
A big thank you to Jeremy Ashkenas and DocumentCloud for making all of this Backbone awesomeness possible.
###Installing:
###Commands:
FAQs
Backbone-Articulation.js enhances Backbone.js model attributes with object serialization and deserialization.
The npm package backbone-articulation receives a total of 3 weekly downloads. As such, backbone-articulation popularity was classified as not popular.
We found that backbone-articulation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.