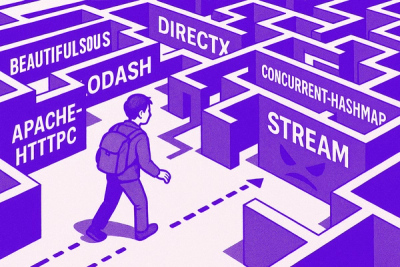
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
basicscroll
Advanced tools
Standalone parallax scrolling for mobile and desktop with CSS variables
Standalone parallax scrolling for mobile and desktop with CSS variables.
basicScroll allows you to change CSS variables depending on the scroll position. Use the variables directly in your CSS to animate whatever you want. Highly inspired by skrollr and Reactive Animations with CSS Variables.
Name | Description | Link | Author |
---|---|---|---|
Default | Includes most features | Try it on CodePen | |
Callback | Animate properties in JS via callbacks | Try it on CodePen | |
Parallax scene | A composition of multiple, moving layers | Try it on CodePen | @electerious |
Rolling eyes | Custom element to track scrolling | Try it on CodePen | @electerious |
Headline explosion | Animated letters | Try it on CodePen | @electerious |
Scroll and morph | Morph text using CSS clip-path | Try it on CodePen | @mikaelainalem |
Parallax with JS | Several examples and a debug mode | Try it on CodePen | AnimatiCSS |
Name | Link |
---|---|
š Parallax scrolling with JS controlled CSS variables | Read it on Medium |
š¬ Apple-like scroll animations | Watch it on YouTube |
š¬ Parallax effect tutorial (šŖšø) | Watch it on YouTube |
basicScroll depends on the following browser features and APIs:
Some of these APIs are capable of being polyfilled in older browsers. Check the linked resources above to determine if you must polyfill to achieve your desired level of browser support.
We recommend installing basicScroll using npm or yarn.
npm install basicscroll
yarn add basicscroll
Include the JS file at the end of your body
tagā¦
<script src="dist/basicScroll.min.js"></script>
ā¦or skip the JS file and use basicScroll as a module:
const basicScroll = require('basicscroll')
import * as basicScroll from 'basicscroll'
This demo shows how to to change the opacity of an element when the user scrolls. The element starts to fade as soon as the top of the element reaches the bottom of the viewport. A opacity of .99
is reached when the middle of the element is in the middle of the viewport.
Tip: Animating from .01
to .99
avoids the repaints that normally occur when the element changes from fully transparent to translucent and from translucent to fully visible.
const instance = basicScroll.create({
elem: document.querySelector('.element'),
from: 'top-bottom',
to: 'middle-middle',
props: {
'--opacity': {
from: .01,
to: .99
}
}
})
instance.start()
.element {
/*
* Use the same CSS variable as specified in our instance.
*/
opacity: var(--opacity);
/*
* The will-change CSS property provides a way for authors to hint browsers about the kind of changes
* to be expected on an element, so that the browser can setup appropriate optimizations ahead of time
* before the element is actually changed.
*/
will-change: opacity;
}
Creates a new basicScroll instance.
Be sure to assign your instance to a variable. Using your instance, you canā¦
Examples:
const instance = basicScroll.create({
from: '0',
to: '100px',
props: {
'--opacity': {
from: 0,
to: 1
}
}
})
const instance = basicScroll.create({
elem: document.querySelector('.element'),
from: 'top-bottom',
to: 'bottom-top',
props: {
'--translateY': {
from: '0',
to: '100%',
timing: 'elasticOut'
}
}
})
const instance = basicScroll.create({
elem: document.querySelector('.element'),
from: 'top-middle',
to: 'bottom-middle',
inside: (instance, percentage, props) => {
console.log('viewport is inside from and to')
},
outside: (instance, percentage, props) => {
console.log('viewport is outside from and to')
}
})
Parameters:
data
{Object}
An object of data.Returns:
{Object}
The created instance.Each basicScroll instance has a handful of handy functions. Below are all of them along with a short description.
Starts to animate the instance. basicScroll will track the scroll position and adjust the props of the instance accordingly. An update will be performed only when the scroll position has changed.
Example:
instance.start()
Stops to animate the instance. All props of the instance will keep their last value.
Example:
instance.stop()
Destroys the instance. Should be called when the instance is no longer needed. All props of the instance will keep their last value.
Example:
instance.destroy()
Triggers an update of an instance, even when the instance is currently stopped.
Example:
const props = instance.update()
Returns:
{Object}
Applied props.Converts the start and stop position of the instance to absolute values. basicScroll relies on those values to start and stop the animation at the right position. It runs the calculation once during the instance creation. .calculate()
should be called when elements have altered their position or when the size of the site/viewport has changed.
Example:
instance.calculate()
Returns true
when the instance is started and false
when the instance is stopped.
Example:
instance.isActive()
Returns:
{Boolean}
Returns calculated data. More or less a parsed version of the data used for the instance creation. The data might change when calling the calculate function.
Example:
instance.getData()
Returns:
{Object}
Parsed data.The data object can include the following properties:
{
/*
* DOM element/node.
*/
elem: null,
/*
* Start and stop position.
*/
from: null,
to: null,
/*
* Direct mode.
*/
direct: false,
/*
* Track window size changes.
*/
track: true,
/*
* Callback functions.
*/
inside: (instance, percentage, props) => {},
outside: (instance, percentage, props) => {},
/*
* Props.
*/
props: {
/*
* Property name / CSS Custom Properties.
*/
'--name': {
/*
* Start and end values.
*/
from: null,
to: null,
/*
* Animation timing.
*/
timing: 'ease'
}
}
}
Type: Node
Default: null
Optional: true
A DOM element/node.
The position and size of the element will be used to convert the start and stop position to absolute values. How else is basicScroll supposed to know when to start and stop an animation with relative values?
You can skip the property when using absolute values.
Example:
{
elem: document.querySelector('.element')
/* ... */
}
Type: Integer|String
Default: null
Optional: false
basicScroll starts to animate the props when the scroll position is above from
and below to
. Absolute and relative values are allowed.
Relative values require a DOM element/node. The first part of the value describes the element position, the last part describes the viewport position: <element>-<viewport>
. middle-bottom
in from
specifies that the animation starts when the middle of the element reaches the bottom of the viewport.
Known relative values: top-top
, top-middle
, top-bottom
, middle-top
, middle-middle
, middle-bottom
, bottom-top
, bottom-middle
, bottom-bottom
It's possible to track a custom anchor when you want to animate for a specific viewport height or when you need to start and end with an offset.
Examples:
{
/* ... */
from: '0px',
to: '100px',
/* ... */
}
{
/* ... */
from: 0,
to: 360,
/* ... */
}
{
/* ... */
from: 'top-middle',
to: 'bottom-middle',
/* ... */
}
Type: Boolean|Node
Default: false
Optional: true
basicScroll applies all props globally by default. This way you can use variables everywhere in your CSS, even when the instance tracks just one element. Set direct
to true
or to a DOM element/node to apply all props directly to the DOM element/node or to the DOM element/node you have specified. This also allows you to animate CSS properties, not just CSS variables.
false
: Apply props globally (default)true
: Apply props to the DOM element/nodeNode
: Apply props to a DOM element/node of your choiceExamples:
<!-- direct: false -->
<html style="--name: 0;">
<div class="trackedElem"></div>
<div class="anotherElem"></div>
</html>
<!-- direct: true -->
<html>
<div class="trackedElem" style="--name: 0;"></div>
<div class="anotherElem"></div>
</html>
<!-- direct: document.querySelector('.anotherElem') -->
<html>
<div class="trackedElem"></div>
<div class="anotherElem" style="--name: 0;"></div>
</html>
Type: Boolean
Default: true
Optional: true
basicScroll automatically recalculates and updates instances when the size of the window changes. You can disable the tracking for each instance individually when you want to take care of it by yourself.
Note: basicScroll only tracks the window size. You still must recalculate and update your instances manually when you modify your site. Each modification that changes the layout of the page should trigger such an update in your code.
Example:
const instance = basicScroll.create({
elem: document.querySelector('.element'),
from: 'top-bottom',
to: 'bottom-top',
track: false,
props: {
'--opacity': {
from: 0,
to: 1
}
}
})
// Recalculate and update your instance manually when the tracking is disabled.
// Debounce this function in production to avoid unnecessary calculations.
window.onresize = function() {
instance.calculate()
instance.update()
}
Type: Function
Default: () => {}
Optional: true
inside
callback executes when the user scrolls and the viewport is within the given start and stop position.outside
callback executes when the user scrolls and the viewport is outside the given start and stop position.Both callbacks receive the current instance, a percentage and the calculated properties:
< 0%
percent = Scroll position is below from
= 0%
percent = Scroll position is from
= 100%
percent = Scroll position is to
> 100%
percent = Scroll position is above from
Example:
{
/* ... */
inside: (instance, percentage, props) => {},
outside: (instance, percentage, props) => {},
/* ... */
}
Type: Object
Default: {}
Optional: true
Values to animate when the scroll position changes.
Each prop of the object represents a CSS property or CSS Custom Property (CSS variables). Custom CSS properties always start with two dashes. A prop with the name --name
is accessible with var(--name)
in CSS.
More about CSS custom properties.
Example:
{
/* ... */
props: {
'--one-variable': { /* ... */ },
'--another-variable': { /* ... */ }
}
}
Type: Integer|String
Default: null
Optional: false
Works with all kinds of units. basicScroll uses the unit of to
when from
has no unit.
Examples:
'--name': {
/* ... */
from: '0',
to: '100px',
/* ... */
}
'--name': {
/* ... */
from: '50%',
to: '100%',
/* ... */
}
'--name': {
/* ... */
from: '0',
to: '1turn',
/* ... */
}
Type: String|Function
Default: linear
Optional: true
A known timing or a custom function. Easing functions get just one argument, which is a value between 0 and 1 (the percentage of how much of the animation is done). The function should return a value between 0 and 1 as well, but for some timings a value less than 0 or greater than 1 is just fine.
Known timings: backInOut
, backIn
, backOut
, bounceInOut
, bounceIn
, bounceOut
, circInOut
, circIn
, circOut
, cubicInOut
, cubicIn
, cubicOut
, elasticInOut
, elasticIn
, elasticOut
, expoInOut
, expoIn
, expoOut
, linear
, quadInOut
, quadIn
, quadOut
, quartInOut
, quartIn
, quartOut
, quintInOut
, quintIn
, quintOut
, sineInOut
, sineIn
, sineOut
Examples:
'--name': {
/* ... */
timing: 'circInOut'
}
'--name': {
/* ... */
timing: (t) => t * t
}
transform
and opacity
and use will-change
to hint browsers about the kind of changes. This way the browser can setup appropriate optimizations ahead of time before the element is actually changed.transform: translateY(var(--ty)); transition: transform .1s
.[3.0.4] - 2021-01-17
FAQs
Standalone parallax scrolling for mobile and desktop with CSS variables
The npm package basicscroll receives a total of 4,456 weekly downloads. As such, basicscroll popularity was classified as popular.
We found that basicscroll demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.