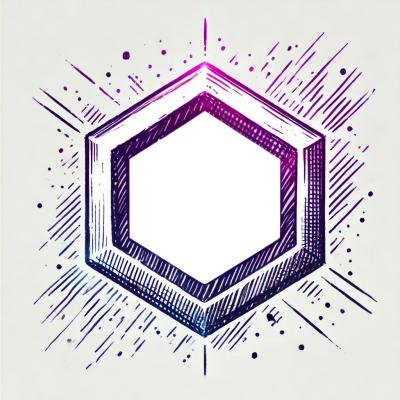
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
bb-media-selector
Advanced tools
A perfect Media Selector for React which works very much like a file manager. ## How to use ### Step 1: Install the media selector Run <code>npm install --save bb-media-selector</code> then you're good to go. ### Step 2: Setup the model #### 1st case, Joo
A perfect Media Selector for React which works very much like a file manager.
Run npm install --save bb-media-selector
then you're good to go.
If you're building a media selector for Joomla and you've already installed Pagebuilder V2, you actually do not need to do anything further. Just copy our example model below, replace the token
(if needed) and the baseURL
with yours. You can go to the step 3 now.
Our project follows MVP for the easiest understanding and usage since you can always build your own model. The matter of fact is that our Media Selector can't handle the model task because it very much depends on your server and database. You need to build your own model.
The main task of the model is interacting with the database then return a response, and that's it.
NOTE: Read how to build a model for more details
In somewhere of your code, set an action to call our Media Selector.
For example:
import React from 'react';
import * as model from 'somewhere/in/your/project/your-model';
import MediaSelector from './App';
export default class Caller extends React.Component {
handleClick = () => {
this.refs.mediaSelector.selectFile((src)=> {
console.log('output', src);
}, 'TYPE_IMAGE')
}
render() {
return <div>
<div onClick={this.handleClick}>Click me</div>
<MediaSelector ref="mediaSelector"/>
</div>
}
}
The Media Selector will open as a modal when it gets called by selectFile
function. The selectFile
needs 2 parameters:
1st callback
: returns the url
of the selected file.
2nd fileType
: 'TYPE_IMAGE' || 'TYPE_FILE'
The difference between the two is the non-image file will only be displayed when the TYPE_FILE
is passed through.
import React from 'react';
import $ from 'jquery';
const token = 'REPLACE_THIS_TOKEN';
export const baseURL = 'REPLACE_THIS_BASE_URL';
/**
* get all files and directories in images/...
* @param path
* @return {$.ajax}: Use .done(res => {}) to handle this ajax request
* expected output: type JSON String, array of {"name":"joomlashine","key":2,"file_size":0.2,"image_width":null,"image_height":null,"type":"dir"}
*/
export const getAllFiles = (path = '/') => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=getListFiles&dir=${path}&token=${token}`,
});
/**
* expected output: JSON string, array of {"type":"file","path":"joomlashine\/sample\/dona_thumb5.png","name":"dona_thumb5.png","image_width":"68","image_height":"68","file_size":"11.16"}
*/
export const getFullDirectory = (path = '/') => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=getFullDirectory&dir=${path}&token=${token}`,
})
/**
* handleUploadFile
* @param {event|*} e event when file is selected via: onChange={this.handleUpload.bind(this)}
* @param {string} path directory you want to upload to, default: '(images)/'
* @param {array} allowType: array of allowed file type name, ex: image
* @return {Promise}: use .then(res => {}) to handle this.
* expected output: JSON Object, {"message":"done","uri":"2016-02-10-1455067088-5968649-MayurGalaviaUnsplash.jpg","list":[{"name":"joomlashine","key":3,"file_size":0.2,"image_width":null,"image_height":null,"type":"dir"}]}
*/
export const handleUploadFile = (file, path = '/', allowType = []) => {
const reader = new FileReader();
reader.readAsDataURL(file);
return new Promise((resolve, reject) => {
reader.onload = (upload) => {
if (!file.type || !upload.target.result) {
return reject(
'No file selected');
}
if ((file.type && $.inArray(allowType, file.type.split('/')[0]) !==
-1) || allowType === []) {
return reject('File type not allowed!');
}
let data = {
dir: path,
data_uri: upload.target.result,
filename: file.name,
filetype: file.type,
}
uploadFile(data, resolve, reject, 0)
};
}).catch((err) => {
console.log('there is an error',err)
});
};
export const uploadFile = (data, resolve, reject, times) => {
$.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=uploadFile&token=${token}`,
type: 'POST',
data: data,
dataType: 'json',
error: (res) => {
console.log(res)
reject(res);
},
success: (res) => {
console.log(res)
if(res.message == 'Filename already exists!') {
times ++
if(times > 1) {
data.filename = data.filename.replace('('+ (times-1) + ')', '')
}
let fileExt = data.filename.substring(data.filename.lastIndexOf('.'), data.filename.length)
data.filename = data.filename.replace(fileExt, '') + '('+times+')' + fileExt
uploadFile(data, resolve, reject, times)
} else {
resolve(res);
}
},
});
}
/**
* create a folder in (images)/...
* @param {string} inPath: where the new folder will be put
* @param {string} name: name of the new folder
* @return {$.ajax} : Use .done(res => {}) to handle this ajax request
* expected output: JSON string, {"success":true,"message":"New folder successfully created!","path":"\/joomlashine\/NewFolder"}
*/
export const createFolder = (inPath, name) => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=createFolder&token=${token}&dir=${inPath}&name=${name}`,
});
/**
* delete a folder in (images)/...
* @param {string} path: directory folder to be deleted
* @return {$.ajax} : Use .done(res => {}) to handle this ajax request
* expected output: JSON string, {"success":true,"message":"The folder \/joomlashine\/sample has been deleted!","path":"\/joomlashine\/sample"}
*/
export const deleteFolder = path => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=deleteFolder&token=${token}&dir=${path}`,
});
/**
* rename a folder in (images)/...
* @param {string} path: directory name of the folder
* @param {string} newPath: new path of the new folder
* @return {$.ajax} : Use .done(res => {}) to handle this ajax request
* expected output: JSON string, {"success":true,"message":"Successfully moved\/renamed folder!","path":"\/joomlashine\/NewFolder","newPath":"\/joomlashine\/NewFolderchan"}
*/
export const renameFolder = (path, newPath) => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=renameFolder&token=${token}&dir=${path}&newPath=${newPath}`,
});
/**
* delete a file in (images)/...
* @param {string} filePath: path of file to be deleted
* @return {$.ajax} : Use .done(res => {}) to handle this ajax request
* expected output: JSON string, {"success":true,"message":"The folder \/joomlashine\/placeholder\/business-img-1.jpg has been deleted!","path":"\/joomlashine\/placeholder\/business-img-1.jpg"}
*/
export const deleteFile = filePath => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=deleteFile&token=${token}&dir=${filePath}`,
});
/**
* rename a file in (images)/...
* @param {string} path: directory name of the folder
* @param {string} newPath: new path of the new folder
* @return {$.ajax} : Use .done(res => {}) to handle this ajax request
* expected output: JSON string, {"success":true,"message":"Successfully moved\/renamed file!","path":"\/joomlashine\/placeholder\/background.jpg","newPath":"\/joomlashine\/placeholder\/backgroundsilver.jpg"}
*/
export const renameFile = (path, newPath) => $.ajax({
url: `${baseURL}index.php?pb2ajax=1&task=renameFile&token=${token}&dir=${path}&newPath=${newPath}`,
});
You need to implement all the methods in the example model above because they are the core functions of the media selector.
The hardest part actually lays on the PHP code you write. Please be very strict on the output of your API coding. Let's take a careful look at the expected output
at the comment section of each functions. Please make sure you respond the right output.
If you are having issues, please let us know.
We have a mailing list located at: mrsilver256@gmail.com
FAQs
A perfect Media Selector for React which works very much like a file manager. ## How to use ### Step 1: Install the media selector Run <code>npm install --save bb-media-selector</code> then you're good to go. ### Step 2: Setup the model #### 1st case, Joo
The npm package bb-media-selector receives a total of 2 weekly downloads. As such, bb-media-selector popularity was classified as not popular.
We found that bb-media-selector demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.