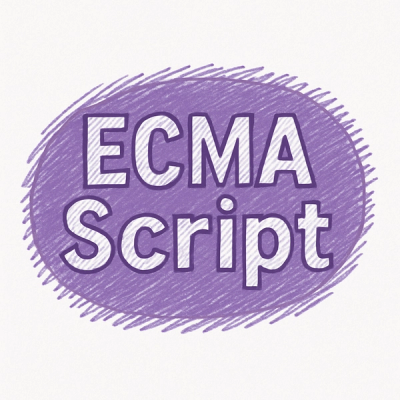
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Optimized bcrypt in plain JavaScript with zero dependencies, with TypeScript support. Compatible to 'bcrypt'.
The bcryptjs npm package is a library that allows developers to hash and compare passwords securely in Node.js applications. It is a pure JavaScript implementation of the bcrypt password hashing algorithm and is compatible with the C++ bcrypt binding on npm. It's designed to be a reliable and secure way to handle password storage and verification.
Hashing Passwords
This feature allows you to securely hash passwords using bcrypt. The `genSalt` function generates a salt, and the `hash` function applies the bcrypt hashing algorithm to the password along with the salt.
const bcrypt = require('bcryptjs');
const password = 'myPassword123';
bcrypt.genSalt(10, function(err, salt) {
bcrypt.hash(password, salt, function(err, hash) {
// Store hash in your password DB.
});
});
Comparing Passwords
This feature is used to compare a plaintext password with a previously hashed one to check if they match. It is commonly used during the login process to verify user credentials.
const bcrypt = require('bcryptjs');
const password = 'myPassword123';
const hash = '$2a$10$N9qo8uLOickgx2ZMRZoMye';
bcrypt.compare(password, hash, function(err, isMatch) {
if (err) throw err;
console.log('Password match:', isMatch);
});
Argon2 is another password hashing library that won the Password Hashing Competition and is considered one of the most secure options. It is different from bcryptjs in that it provides resistance to GPU cracking attacks and can be configured for additional memory hardness.
Scrypt is a password-based key derivation function that is designed to be costly computationally and memory-wise, making it difficult to perform large-scale custom hardware attacks. It is similar to bcryptjs in its goals but uses a different approach to achieve them.
PBKDF2 (Password-Based Key Derivation Function 2) is part of RSA Laboratories' Public-Key Cryptography Standards series, and it's widely used for password hashing. Unlike bcryptjs, PBKDF2 can be used not only for password hashing but also for deriving cryptographic keys from passwords.
Optimized bcrypt in JavaScript with zero dependencies, with TypeScript support. Compatible to the C++ bcrypt binding on Node.js and also working in the browser.
Besides incorporating a salt to protect against rainbow table attacks, bcrypt is an adaptive function: over time, the iteration count can be increased to make it slower, so it remains resistant to brute-force search attacks even with increasing computation power. (see)
While bcrypt.js is compatible to the C++ bcrypt binding, it is written in pure JavaScript and thus slower (about 30%), effectively reducing the number of iterations that can be processed in an equal time span.
The maximum input length is 72 bytes (note that UTF-8 encoded characters use up to 4 bytes) and the length of generated
hashes is 60 characters. Note that maximum input length is not implicitly checked by the library for compatibility with
the C++ binding on Node.js, but should be checked with bcrypt.truncates(password)
where necessary.
The package exports an ECMAScript module with an UMD fallback.
$> npm install bcryptjs
import bcrypt from "bcryptjs";
https://cdn.jsdelivr.net/gh/dcodeIO/bcrypt.js@TAG/index.js
(ESM)https://cdn.jsdelivr.net/npm/bcryptjs@VERSION/index.js
(ESM)https://cdn.jsdelivr.net/npm/bcryptjs@VERSION/umd/index.js
(UMD)https://unpkg.com/bcryptjs@VERSION/index.js
(ESM)https://unpkg.com/bcryptjs@VERSION/umd/index.js
(UMD)Replace TAG
respectively VERSION
with a specific version or omit it (not recommended in production) to use latest.
When using the ESM variant in a browser, the crypto
import needs to be stubbed out, for example using an import map. Bundlers should omit it automatically.
To hash a password:
const salt = bcrypt.genSaltSync(10);
const hash = bcrypt.hashSync("B4c0/\/", salt);
// Store hash in your password DB
To check a password:
// Load hash from your password DB
bcrypt.compareSync("B4c0/\/", hash); // true
bcrypt.compareSync("not_bacon", hash); // false
Auto-gen a salt and hash:
const hash = bcrypt.hashSync("bacon", 10);
To hash a password:
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash("B4c0/\/", salt);
// Store hash in your password DB
bcrypt.genSalt(10, (err, salt) => {
bcrypt.hash("B4c0/\/", salt, function (err, hash) {
// Store hash in your password DB
});
});
To check a password:
// Load hash from your password DB
await bcrypt.compare("B4c0/\/", hash); // true
await bcrypt.compare("not_bacon", hash); // false
// Load hash from your password DB
bcrypt.compare("B4c0/\/", hash, (err, res) => {
// res === true
});
bcrypt.compare("not_bacon", hash, (err, res) => {
// res === false
});
Auto-gen a salt and hash:
await bcrypt.hash("B4c0/\/", 10);
// Store hash in your password DB
bcrypt.hash("B4c0/\/", 10, (err, hash) => {
// Store hash in your password DB
});
Note: Under the hood, asynchronous APIs split an operation into small chunks. After the completion of a chunk, the execution of the next chunk is placed on the back of the JS event queue, efficiently yielding for other computation to execute.
Usage: bcrypt <input> [rounds|salt]
Callback<T
>: (err: Error | null, result?: T) => void
Called with an error on failure or a value of type T
upon success.
ProgressCallback: (percentage: number) => void
Called with the percentage of rounds completed (0.0 - 1.0), maximally once per MAX_EXECUTION_TIME = 100
ms.
RandomFallback: (length: number) => number[]
Called to obtain random bytes when both Web Crypto API and Node.js
crypto are not available.
bcrypt.genSaltSync(rounds?: number
): string
Synchronously generates a salt. Number of rounds defaults to 10 when omitted.
bcrypt.genSalt(rounds?: number
): Promise<string>
Asynchronously generates a salt. Number of rounds defaults to 10 when omitted.
bcrypt.genSalt([rounds: number
, ]callback: Callback<string>
): void
Asynchronously generates a salt. Number of rounds defaults to 10 when omitted.
bcrypt.truncates(password: string
): boolean
Tests if a password will be truncated when hashed, that is its length is greater than 72 bytes when converted to UTF-8.
bcrypt.hashSync(password: string
, salt?: number | string
): string
Synchronously generates a hash for the given password. Number of rounds defaults to 10 when omitted.
bcrypt.hash(password: string
, salt: number | string
): Promise<string>
Asynchronously generates a hash for the given password.
bcrypt.hash(password: string
, salt: number | string
, callback: Callback<string>
, progressCallback?: ProgressCallback
): void
Asynchronously generates a hash for the given password.
bcrypt.compareSync(password: string
, hash: string
): boolean
Synchronously tests a password against a hash.
bcrypt.compare(password: string
, hash: string
): Promise<boolean>
Asynchronously compares a password against a hash.
bcrypt.compare(password: string
, hash: string
, callback: Callback<boolean>
, progressCallback?: ProgressCallback
)
Asynchronously compares a password against a hash.
bcrypt.getRounds(hash: string
): number
Gets the number of rounds used to encrypt the specified hash.
bcrypt.getSalt(hash: string
): string
Gets the salt portion from a hash. Does not validate the hash.
bcrypt.setRandomFallback(random: RandomFallback
): void
Sets the pseudo random number generator to use as a fallback if neither Web Crypto API nor Node.js crypto are available. Please note: It is highly important that the PRNG used is cryptographically secure and that it is seeded properly!
Building the UMD fallback:
$> npm run build
Running the tests:
$> npm test
Based on work started by Shane Girish at bcrypt-nodejs, which is itself based on javascript-bcrypt (New BSD-licensed).
FAQs
Optimized bcrypt in plain JavaScript with zero dependencies, with TypeScript support. Compatible to 'bcrypt'.
The npm package bcryptjs receives a total of 3,383,664 weekly downloads. As such, bcryptjs popularity was classified as popular.
We found that bcryptjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.