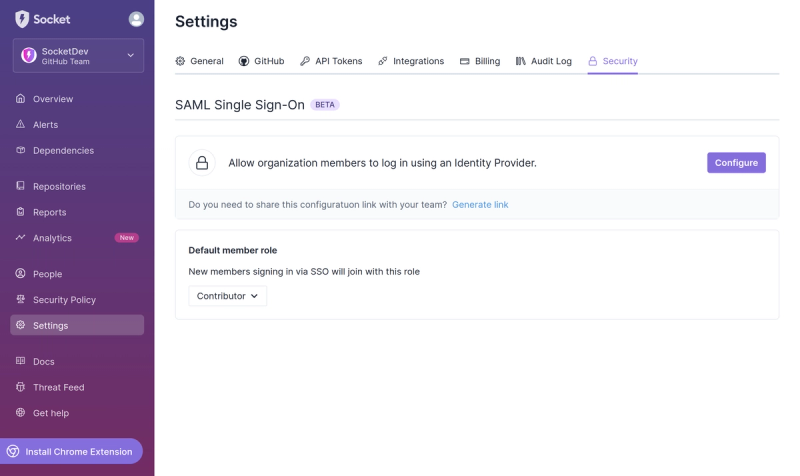
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
bigval
Advanced tools
Easier calculation and formatting of crypto values with decimals. Useful for smart contract interaction.
Readme
Javascript library for representing arbitrarily large or small crypto values with decimals.
bigval
is useful for handling extremely large crypto numbers with precision, e.g. when performing arithmetic with balances.
It is inspired by ethval.
Features:
npm install --save bigval
Initializing a value:
const { BigVal } = require('bigval')
const v1 = new BigVal(1000000000000000000)
const v2 = new BigVal('1000000000000000000')
const v3 = new BigVal('0.00000001')
const v4 = new BigVal('0xde0b6b3a7640000')
const v5 = new BigVal('b1000000000000000000')
const v6 = new BigVal(new BigVal(1000000000000000000))
Scaling:
const v6 = new BigVal(6, 'coins')
const sameAsV6 = new BigVal('6000000000000000000', 'min')
const sameAsV6Again = new BigVal('6000000000000000000')
Scaling is based on no. of decimals:
const v = new BigVal(100, 'min', { decimals: 2 }) // same as new BigVal(1, 'coins', { decimals: 2 })
console.log( v.scale ) // 'min'
const v2 = v.toCoinScale()
console.log( v2.scale ) // 'coins'
console.log( v2.toString() ) // "1"
const v3 = v2.toMinScale()
console.log( v3.scale ) // 'min'
console.log( v3.toString() ) // "100"
Simple arithmetic leaves original unchanged:
const v = new BigVal(1000000000000000000)
const v2 = v.add(32) // v2 is a new instance of BigVal
console.log(v2 !== v) // true
console.log(v2.toString()) // "1000000000000000032"
console.log(v.toString()) // "1000000000000000000" - original unchanged
Initializing using from()
static convenience method:
const v6 = BigVal::from('1', 'coins', { decimals: 2 })
const v6_1 = v6.toMinScale()
console.log( v6_1.scale ) // 'min'
console.log( v6_1.toString() ) // "100"
Initializing using fromStr()
static method:
const v7 = BigVal::fromStr('2 coins') // same as BigVal::from(2, 'coins')
const v8 = BigVal::fromStr('0.5 min') // same as BigVal::from(0.5, 'min')
const v9 = BigVal::fromStr('0xff', { decimals: 2 }) // same as BigVal::from(0xff, undefined, { decimals: 2 })
Output in different types:
const v = new BigVal(255)
console.log( v.toString() ) // "255"
console.log( v.toString(10) ) // "255"
console.log( v.toString(16) ) // "FF"
console.log( v.toString(2) ) // "1111111"
console.log( v.toNumber() ) // 255
Decimals and rounding:
const v = new BigVal(100)
const v2 = v.div(3)
console.log( v2.toString() ) // "33.333333333333333"
console.log( v2.toFixed(1) ) // "33.3"
const v3 = v2.round()
console.log( v3.toString() ) // "33"
const v4 = new BigVal(0.00000001)
console.log(v4.decimalCount) // 8
Flexible input types:
const v = new BigVal(255)
// these are all the same
v.add(32)
v.add('32')
v.add(new BigVal(32))
Logical operators:
const v = new BigVal(255)
const isGreater = v.gte(254)
console.log( isGreater ) // true
At any given time a BigVal
instance operates at a particular number scale. The scale is based on the the no. of decimals
specified in the configuration (BigValConfig
). By default the no. of decimals
is 18.
The min
scale (this is the default) is for numbers which do not have decimal places since they are already denominated in the smallest possible unit. The coins
scale is for numbers which implicitly have decimal places.
For example, if a given BigVal
has decimals = 2
then the following two numbers are equivalent in value:
min
, value = 100
coins
, value = 1
If decimals = 18
(this is the default) then the following two numbers are equivalent in value:
min
, value = 1000000000000000000
coins
, value = 1
The use of scales like this makes it easy to convert between chain-friendly and user-friendly values and perform arithmetic at the desired precision.
To build both ESM and CommonJS output:
yarn build
To re-build the CommonJS output on chnage:
yarn dev
To test:
yarn test
To build the docs:
yarn build-docs
To publish a new release (this will create a tag, publish to NPM and publish the latest docs):
yarn release
MIT
FAQs
Easier calculation and formatting of crypto values with decimals. Useful for smart contract interaction.
The npm package bigval receives a total of 12 weekly downloads. As such, bigval popularity was classified as not popular.
We found that bigval demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.