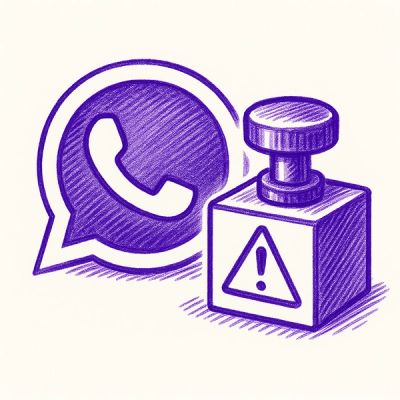
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
blake3-wasm
Advanced tools
The blake3-wasm package provides WebAssembly (WASM) bindings for the BLAKE3 cryptographic hash function, which is known for its high speed and security. This package allows you to leverage the performance benefits of WASM to compute BLAKE3 hashes efficiently in JavaScript environments.
Hashing a string
This feature allows you to compute the BLAKE3 hash of a given string. The code sample demonstrates how to hash the string 'hello world' and output the result in hexadecimal format.
const blake3 = require('blake3-wasm');
const hash = blake3.hash('hello world');
console.log(hash.toString('hex'));
Hashing a file
This feature allows you to compute the BLAKE3 hash of a file. The code sample demonstrates how to read a file into a buffer and then compute its BLAKE3 hash, outputting the result in hexadecimal format.
const fs = require('fs');
const blake3 = require('blake3-wasm');
const fileBuffer = fs.readFileSync('path/to/file');
const hash = blake3.hash(fileBuffer);
console.log(hash.toString('hex'));
Incremental hashing
This feature allows you to perform incremental hashing, which is useful for hashing data that is received in chunks. The code sample demonstrates how to create a hasher, update it with multiple chunks of data, and then compute the final hash.
const blake3 = require('blake3-wasm');
const hasher = blake3.createHasher();
hasher.update('hello');
hasher.update(' ');
hasher.update('world');
const hash = hasher.digest();
console.log(hash.toString('hex'));
The blake3 package provides a pure JavaScript implementation of the BLAKE3 hash function. While it does not leverage WebAssembly for performance, it is still a reliable option for environments where WASM is not available or desired.
The blake2 package provides implementations of the BLAKE2 hash function, which is a predecessor to BLAKE3. It offers good performance and security, but BLAKE3 is generally faster and more efficient.
The crypto-js package provides a wide range of cryptographic algorithms, including SHA-256, SHA-3, and HMAC. While it does not include BLAKE3, it is a versatile library for various cryptographic needs.
3.0.0 - 2022-10-08
blake3.load()
before using hash functionsdispose()
on hash instances is now optionalFAQs
BLAKE3 hashing for JavaScript: WebAssembly bindings only
The npm package blake3-wasm receives a total of 1,085,427 weekly downloads. As such, blake3-wasm popularity was classified as popular.
We found that blake3-wasm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.