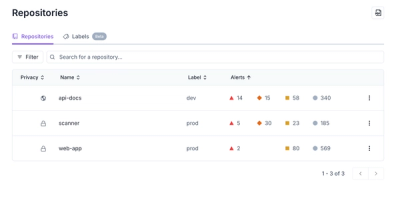
Product
Redesigned Repositories Page: A Faster Way to Prioritize Security Risk
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
blue-button-util
Advanced tools
Common utility methods for Amida-Tech repositories
This library provides common Javascript utilities that are used in other Amida-Tech libraries.
##Utilities
You can install using npm and require
to use in node.js
var bbu = require('blue-button-util');
var ob = bbu.object; // object library
var obs = bbu.object; // objectset library
var arrs = bbu.arrayset; // arrayset library
var dtt = bbu.datetime; // datetime library
var pred = bbu.pred; // predicate library
var jsonpath = bbu.jsonpath; // jsonpath library
The following methods are provided
object.exists
object.deepValue
objectset.compact
objectset.deepValue
arrayset.append
datetime.dateToModel
datetime.dateTimeToModel
datetime.modelToDate
datetime.modelToDateTime
predicate.hasProperty
predicate.hasNoProperty
predicate.hasNoProperties
predicate.inValueSet
predicate.propertyValue
predicate.falsyPropertyValue
predicate.and
predicate.or
jsonpath.instance
object
LibraryProvides utility methods for objects.
####exists(obj)Checks if obj
is not undefined
or null
var r0 = ob.exists(null);
var r1 = ob.exists(undefined);
var r2 = ob.exists('anything else');
console.log(r0); // false
console.log(r1); // false
console.log(r2); // true
####deepValue(obj, deepProperty)
Returns obj
deep values where deepProperty
can be '.' delimited keys
var input = {
a: {
b: {
c: 1
},
d: 2
},
e: 3
};
var r0 = ob.deepValue(null, 'any');
var r1 = ob.deepValue(input, 'a.b');
var r2 = ob.deepValue(input, 'a.b.c');
var r3 = ob.deepValue(input, 'a.e.f');
var r4 = ob.deepValue(input, 'a.f');
var r5 = ob.deepValue('primary data types', 'any');
console.log(r0); // null
console.log(r1); // {c: 1}
console.log(r2); // 1
console.log(r3); // null
console.log(r4); // null
console.log(r5); // null
Nothing special is done for arrays so you can specify indices in deepProperty
var input = [{
a: 1
}, {
b: ['value']
}];
var r0 = ob.deepValue(input, '0.a');
var r1 = ob.deepValue(input, '1.b');
var r2 = ob.deepValue(input, '1.b.0');
console.log(r0); // 1
console.log(r1); // ['value']
console.log(r2); // 'value'
objectset
LibraryProvides utility methods that modify an object.
####objectset.compact(obj)Recursively removes all null
and undefined
values from obj
. No special handling is done for resulting empty objects or arrays
var obj = {
a: 1,
b: null,
c: {
d: undefined,
e: 4
},
f: {
g: null
}
};
obs.compact(obj);
console.log(obj); // {a: 1, c:{e:4}, f:{}}
####objectset.deepValue(obj, deepProperty, value)
Assigns value
to deepProperty
of obj
possibly creating multiple objects
var obj = {};
obs.deepValue(obj, 'a.b', 'value');
console.log(obj); // {a: {b: 'value'}}
Existing keys are kept for destination obj
but their values might ve overridden
var obj = {
a: 1,
b: {
c: 2,
d: 3
}
};
obs.deepValue(obj, 'b.c', {
e: 4
});
console.log(obj); // {a: 1, b: {c: {e: 4}, d: 3}}
arrayset
LibraryProvides utility methods that modify an array.
#### append(arr, arrToAppend)Appends arrToAppend
elements to arr
var arr = ['a', 'b'];
arrs.append(arr, ['c', 'd']);
console.log(arr); // ['a', 'b', 'c', 'd'];
datetime
LibraryProvides conversion methods to/from blue-button-model datetimes from/to ISO datetimes.
#### datetime.dateToModel(d)Converts ISO date d
to blue-button-model datetime
var r0 = dtt.dateToModel('2014');
var r1 = dtt.dateToModel('2014-02');
var r2 = dtt.dateToModel('2014-02-07');
var r3 = dtt.dateToModel('2014-02-07T12:45:04.000Z');
console.log(r0); // {date: '2014-01-01T00:00:00.000Z', precision: 'year'}
console.log(r1); // {date: '2014-02-01T00:00:00.000Z', precision: 'month'}
console.log(r2); // {date: '2014-02-07T00:00:00.000Z', precision: 'day'}
console.log(r3); // {date: '2014-02-07T00:00:00.000Z', precision: 'day'}
#### datetime.dateTimeToModel(dt)
Converts ISO datetime d
to blue-button-model datetime
var r0 = dtt.dateTimeToModel('2014');
var r1 = dtt.dateTimeToModel('2014-02');
var r2 = dtt.dateTimeToModel('2014-02-07');
var r3 = dtt.dateTimeToModel('2014-02-07T12:45:04.000Z');
console.log(r0); // {date: '2014-01-01T00:00:00.000Z', precision: 'year'}
console.log(r1); // {date: '2014-02-01T00:00:00.000Z', precision: 'month'}
console.log(r2); // {date: '2014-02-07T00:00:00.000Z', precision: 'day'}
console.log(r3); // {date: '2014-02-07T12:45:04.000Z', precision: 'second'}
Millisecond piece is ignored even when it is not zero.
#### datetime.modelToDate(dt)Converts blue-button-model datetime dt
to ISO date
var r0 = dtt.modelToDate({
date: '2014-01-01T00:00:00.000Z',
precision: 'year'
});
var r1 = dtt.modelToDate({
date: '2014-02-01T00:00:00.000Z',
precision: 'month'
});
var r2 = dtt.modelToDate({
date: '2014-02-07T00:00:00.000Z',
precision: 'day'
});
var r3 = dtt.modelToDate({
date: '2014-02-07T12:45:04.000Z',
precision: 'second'
});
console.log(r0); // '2014'
console.log(r1); // '2014-02'
console.log(r2); // '2014-02-07'
console.log(r3); // '2014-02-07'
#### datetime.modelToDateTime(dt)
Converts blue-button-model datetime dt
to ISO datetime
var r0 = dtt.modelToDateTime({
date: '2014-01-01T00:00:00.000Z',
precision: 'year'
});
var r1 = dtt.modelToDateTime({
date: '2014-02-01T00:00:00.000Z',
precision: 'month'
});
var r2 = dtt.modelToDateTime({
date: '2014-02-07T00:00:00.000Z',
precision: 'day'
});
var r3 = dtt.modelToDateTime({
date: '2014-02-07T12:45:04.000Z',
precision: 'second'
});
var r4 = dtt.modelToDateTime({
date: '2014-02-07T12:45:04.010Z',
precision: 'second'
});
console.log(r0); // '2014'
console.log(r1); // '2014-02'
console.log(r2); // '2014-02-07'
console.log(r3); // '2014-02-07T12:45:04.000Z'
console.log(r4); // '2014-02-07T12:45:04.010Z'
### `predicate` Library
Provides methods that that return predicate functions on one argument.
#### hasProperty(deepProperty)Returns a predicate that checks if input
has the listed deep property
var f = pred.hasProperty('a.b');
var r0 = f(null);
var r1 = f({
a: {
c: 1
}
});
var r2 = f({
a: {
b: 1
}
});
console.log(r0); // false
console.log(r1); // false
console.log(r2); // true
#### hasNoProperty(deepProperty)
Returns a predicate that checks if input
has not the listed deep property
var f = pred.hasNoProperty('a.b');
var r0 = f(null);
var r1 = f({
a: {
c: 1
}
});
var r2 = f({
a: {
b: 1
}
});
console.log(r0); // true
console.log(r1); // true
console.log(r2); // false
#### hasNoProperties(deepProperties)
Returns a predicate that checks if input
has none of the listed deep properties
var f = pred.hasNoProperties(['a.b', 'c']);
var r0 = f(null);
var r1 = f({
a: {
c: 1
}
});
var r2 = f({
a: {
b: 1
}
});
var r3 = f({
a: {
c: 1
},
c: 5
});
console.log(r0); // true
console.log(r1); // true
console.log(r2); // false
console.log(r3); // false
#### inValueSet(valueSet)
Returns a predicate that checks if input
is one of the values
var f = pred.inValueSet(['Resolved', 'Active']);
var r0 = f('Any');
var r1 = f('Resolved');
var r2 = f('Active');
console.log(r0); // false
console.log(r1); // true
console.log(r2); // true
#### propertyValue(deepProperty)
Returns a predicate that checks input
's deep property
var f = pred.propertyValue('a.b');
var r0 = f(null);
var r1 = f({
a: 1
});
var r2 = f({
a: {
b: 1
}
});
var r3 = f({
a: {
b: {
c: 2
}
}
});
var r4 = f({
a: {
b: null
}
});
var r5 = f({
a: {
b: false
}
});
console.log(r0); // falsy
console.log(r1); // falsy
console.log(r2); // truthy
console.log(r3); // truthy
console.log(r4); // falsy
console.log(r5); // falsy
#### falsyPropertyValue(deepProperty)
Returns a predicate that checks if input
's deep property is falsy
var f = pred.falsyPropertyValue('a.b');
var r0 = f(null);
var r1 = f({
a: 1
});
var r2 = f({
a: {
b: 1
}
});
var r3 = f({
a: {
b: {
c: 2
}
}
});
var r4 = f({
a: {
b: null
}
});
var r5 = f({
a: {
b: false
}
});
console.log(r0); // truthy
console.log(r1); // truthy
console.log(r2); // falsy
console.log(r3); // falsy
console.log(r4); // truthy
console.log(r5); // truthy
#### and(fns)
Returns a predicate that and's other predicates
var f0 = pred.hasProperty('a.b');
var f1 = pred.hasProperty('a.c');
var f = pred.and([f0, f1]);
var r0 = f(null);
var r1 = f({
a: 1
});
var r2 = f({
a: {
b: 1
}
});
var r3 = f({
a: {
c: 1
}
});
var r4 = f({
a: {
b: 1,
c: 2
}
});
console.log(r0); // false
console.log(r1); // false
console.log(r2); // false
console.log(r3); // false
console.log(r4); // true
#### or(fns)
Returns a predicate that or's other predicates
var f0 = pred.hasProperty('a.b');
var f1 = pred.hasProperty('a.c');
var f = pred.or([f0, f1]);
var r0 = f(null);
var r1 = f({
a: 1
});
var r2 = f({
a: {
b: 1
}
});
var r3 = f({
a: {
c: 1
}
});
var r4 = f({
a: {
b: 1,
c: 2
}
});
console.log(r0); // false
console.log(r1); // false
console.log(r2); // true
console.log(r3); // true
console.log(r4); // true
jsonpath
LibraryThis library provides an implementation of JSONPath with extended syntax for functions. The code is originally based on and keeps all functionality of this implementation. API and code is structured so that no performance penalties are paid when parent (^
) and path functionalities are not used.
In addition to functionality described here, this library implements ability to add functions as part of JSONPath. The example below illustrates the additional functionality.
#### instance(inputExpr, opts)Returns a JSONPath evaluator.
var example = {
"store": {
"book": [{
"category": "reference",
"author": "Nigel Rees",
"title": "Sayings of the Century",
"price": 8.95
}, {
"category": "fiction",
"author": "Evelyn Waugh",
"title": "Sword of Honour",
"price": 12.99
}, {
"category": "fiction",
"author": "Herman Melville",
"title": "Moby Dick",
"isbn": "0-553-21311-3",
"price": 8.99
}, {
"category": "fiction",
"author": "J. R. R. Tolkien",
"title": "The Lord of the Rings",
"isbn": "0-395-19395-8",
"price": 22.99
}],
"bicycle": {
"color": "red",
"price": 19.95
}
}
};
var options = {
functions: {
round: function (obj) {
return Math.round(obj);
}
}
};
var jp = jsonpath.instance('$.store..price.round()', options);
var result = jp(example);
console.log(result); // [ 9, 13, 9, 23, 20 ]
FAQs
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Slopsquatting is a new supply chain threat where AI-assisted code generators recommend hallucinated packages that attackers register and weaponize.
Security News
Multiple deserialization flaws in PyTorch Lightning could allow remote code execution when loading untrusted model files, affecting versions up to 2.4.0.