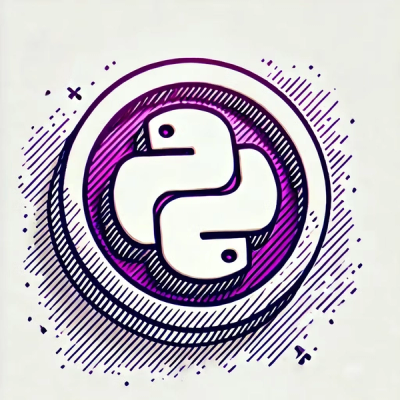
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
broccoli-debug
Advanced tools
The broccoli-debug npm package is a tool for debugging Broccoli.js build pipelines. It allows developers to inspect and visualize the intermediate states of their build trees, making it easier to understand and troubleshoot the build process.
Debugging Build Trees
This feature allows you to insert debug checkpoints in your Broccoli build pipeline. By labeling different stages of the build process, you can inspect the state of the build tree at various points.
const debugTree = require('broccoli-debug');
let tree = someBroccoliTree;
tree = debugTree(tree, 'initial');
// Perform some transformations
// Debug the transformed tree
tree = debugTree(tree, 'transformed');
Visualizing Build Trees
This feature allows you to visualize the build tree at different stages. By using the debugTree function with appropriate labels, you can generate visual representations of the build tree, which can be helpful for understanding the build process and identifying issues.
const debugTree = require('broccoli-debug');
const broccoli = require('broccoli');
let tree = someBroccoliTree;
tree = debugTree(tree, 'visualize');
const builder = new broccoli.Builder(tree);
builder.build().then(() => {
console.log('Build complete. Check the debug output for visualization.');
});
broccoli-inspector is another tool for inspecting Broccoli.js build pipelines. It provides similar functionality to broccoli-debug, allowing developers to visualize and debug their build trees. However, broccoli-inspector focuses more on providing a graphical interface for inspecting the build process, whereas broccoli-debug is more code-centric.
broccoli-viz is a visualization tool for Broccoli.js build pipelines. It generates visual representations of the build tree, similar to broccoli-debug's visualization feature. The main difference is that broccoli-viz is specifically designed for creating visual outputs, while broccoli-debug also includes debugging capabilities.
Utility for build pipeline authors to allow trivial debugging of the Broccoli pipelines they author.
Heavily inspired by @stefanpenner's
broccoli-stew's debug
's helper,
but improved in a few ways:
.png
's as utf8
text).To allow consumers to debug the internals of various stages in your build pipeline,
you create a new instance of BroccoliDebug
and return it instead.
Something like this:
var BroccoliDebug = require('broccoli-debug');
let tree = new BroccoliDebug(input, `ember-engines:${this.name}:addon-input`);
Obviously, this would get quite verbose to do many times, so we have created a shortcut to easily create a number of debug trees with a shared prefix:
let debugTree = BroccoliDebug.buildDebugCallback(`ember-engines:${this.name}`);
let tree1 = debugTree(input1, 'addon-input');
// tree1.debugLabel => 'ember-engines:<some-name>:addon-input'
let tree2 = debugTree(input2, 'addon-output');
// tree2.debugLabel => 'ember-engines:<some-name>:addon-output
Folks wanting to inspect the state of the build pipeline at that stage, would do the following:
BROCCOLI_DEBUG=ember-engines:* ember b
Now you can take a look at the state of that input tree by:
ls DEBUG/ember-engines/*
interface BroccoliDebugOptions {
/**
The label to use for the debug folder. By default, will be placed in `DEBUG/*`.
*/
label: string
/**
The base directory to place the input node contents when debugging is enabled.
Chooses the default in this order:
* `process.env.BROCCOLI_DEBUG_PATH`
* `path.join(process.cwd(), 'DEBUG')`
*/
baseDir: string
/**
Should the tree be "always on" for debugging? This is akin to `debugger`, its very
useful while actively working on a build pipeline, but is likely something you would
remove before publishing.
*/
force?: boolean
}
class BroccoliDebug {
/**
Builds a callback function for easily generating `BroccoliDebug` instances
with a shared prefix.
*/
static buildDebugCallback(prefix: string): (node: any, labelOrOptions: string | BroccoliDebugOptions) => BroccoliNode
constructor(node: BroccoliNode, labelOrOptions: string | BroccoliDebugOptions);
debugLabel: string;
}
git clone git@github.com:broccolijs/broccoli-debug.git
cd broccoli-debug
yarn
yarn test
FAQs
Enable easy debugging of broccoli pipelines with broccoli-debug!
The npm package broccoli-debug receives a total of 162,592 weekly downloads. As such, broccoli-debug popularity was classified as popular.
We found that broccoli-debug demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.