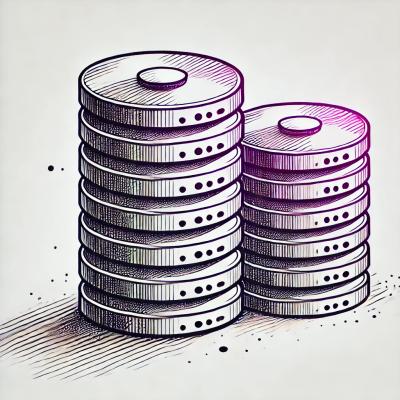
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
The callsite npm package allows developers to access the call stack of V8 engines in Node.js applications. It provides a way to inspect and manipulate the call stack, which can be useful for debugging, logging, and tracking the flow of an application. The package offers a straightforward API to retrieve an array of call sites (stack frames), enabling developers to programmatically explore and utilize call stack information.
Accessing the call stack
This feature allows developers to access the current call stack. The code sample demonstrates how to use the callsite package to retrieve the call stack within a function and then print the file name of the caller function.
const callsite = require('callsite');
function sampleFunction() {
const stack = callsite();
const requester = stack[1].getFileName();
console.log(`Called by ${requester}`);
}
sampleFunction();
The 'stack-trace' package provides similar functionality to 'callsite' by allowing developers to get stack traces of V8 JavaScript engines. It offers more detailed control over the stack trace, such as filtering and mapping of stack frames. Compared to 'callsite', 'stack-trace' might offer a more comprehensive API for working with stack traces.
This package is designed to parse and extract stack traces from Error objects. While 'callsite' focuses on accessing the current call stack directly, 'error-stack-parser' is more about analyzing stack traces from existing Error objects. It can be particularly useful for error handling and logging.
Access to v8's "raw" CallSite
s.
$ npm install callsite
var stack = require('callsite');
foo();
function foo() {
bar();
}
function bar() {
baz();
}
function baz() {
console.log();
stack().forEach(function(site){
console.log(' \033[36m%s\033[90m in %s:%d\033[0m'
, site.getFunctionName() || 'anonymous'
, site.getFileName()
, site.getLineNumber());
});
console.log();
}
Because you can do weird, stupid, clever, wacky things such as:
MIT
FAQs
access to v8's CallSites
The npm package callsite receives a total of 3,244,976 weekly downloads. As such, callsite popularity was classified as popular.
We found that callsite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.