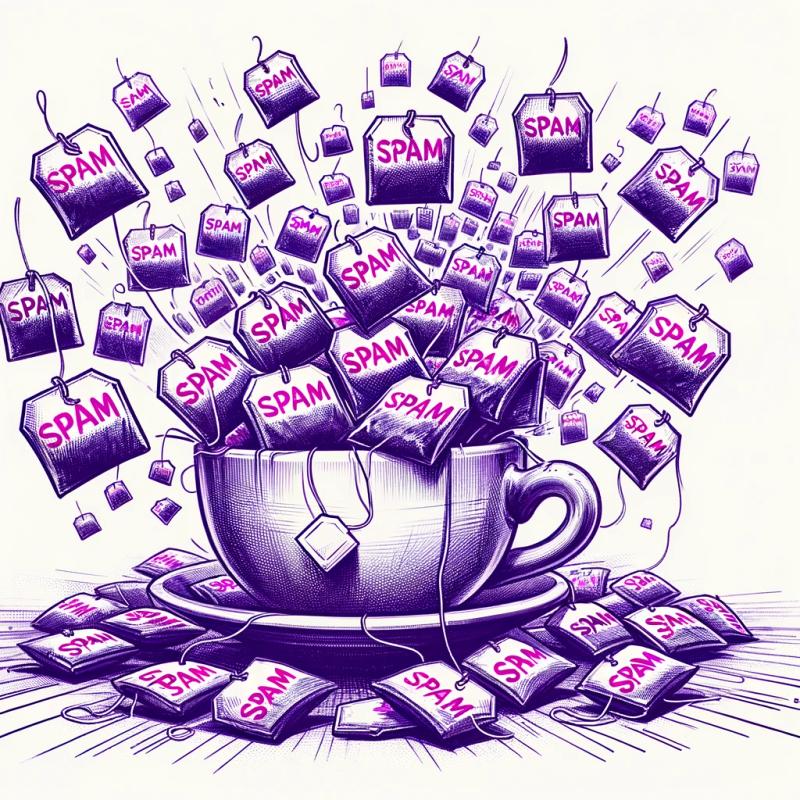
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
captchaai-npm
Advanced tools
Readme
Want you to get verified captcha tokens calling one function within your NodeJS application?
Run with this repo and find a fast way to perform web/api automations.
now binded: 🔥 AntiKasada & AntiAkamaiBMP. 🔥 HCaptcha & FunCaptcha Images Classification.
npm i captchaai-npm
Import module.
const Captchaai = require('captchaai-npm');
Declare singleton/handler.
const handler = new Captchaai(apikey); // task handler / solver
❗ There are 2 different versions in order to handle task results:
1️⃣ task-bind methods
example: check captchaai.io balance + run for one .hcaptchaproxyless()
const Captchaai = require('captchaai-npm');
const handler = new Captchaai('apikey', 1); // verbose level 1
let b = await handler.balance();
if(b > 0){ // usd balance
await handler.hcaptchaproxyless('https://websiteurl.com/', '000000-000000000-0000000')
.then(async response => {
if(response.error === 0){ console.log(response.solution) }
else{ console.log('error ' + JSON.stringify(response.apiResponse)) }
});
}
example: run for one HCaptchaTask with .hcaptcha()
with custom proxy.
const Captchaai = require('captchaai-npm');
const handler = new Captchaai('apikey', 1); // verbose level 1
let b = await handler.balance();
if(b > 0){ // usd balance
await handler.hcaptcha(
'https://websiteurl.com/',
'000000-000000000-0000000',
{ proxy: "proxyType:proxyAddress:proxyPort:proxyLogin:proxyPassword" } // 2nd proxyInfo format
)
.then(async response => {
if(response.error === 0){ console.log(response.solution) }
else{ console.log('error ' + JSON.stringify(response.apiResponse)) }
});
}
2️⃣ Run any task. Build taskData
schema for a task type.
example: build & run taskData
schema with custom proxy for HCaptchaTask.
const Captchaai = require('captchaai-npm');
const handler = new Captchaai('apikey');
const taskData = // build a task
{
type : 'HCaptchaTask',
websiteURL : 'https://website.com/',
websiteKey : '000000-00000-000000-000000000',
// also string format is supported with `proxy`
// proxyInfo: { proxy: "proxyType:proxyAddress:proxyPort:proxyLogin:proxyPassword" },
proxyInfo: { 'proxyType': 'http', 'proxyAddress': 'ip_address', 'proxyPort': 3221, 'proxyLogin': 'username', 'proxyPassword': 'password' },
}
handler.runAnyTask(taskData)
.then(async response => {
if(response.error === 0){ console.log(response.solution) }
else{ console.log('error ' + JSON.stringify(response.apiResponse)) }
});
All methods return the following schema.
Parameter | Type | Description |
---|---|---|
error | number | [-1] Request/Solving error. [0] Success solve. |
statusText | string | HTTP status string. |
apiResponse | object | Results/solution (captchaai.io API response). |
solution | object | Solution got from success solve. |
// ✅ success response
{
error: 0,
statusText: '200 OK',
apiResponse: {
errorId: 0,
taskId: '4e6c33f5-bc14-44d0-979e-d5f37b072c59',
status: 'ready',
solution: {
gRecaptchaResponse: '03AIIukzgCys9brSNnrVbwXE9mTesvkxQ-ocK ...'
}
}
}
// ❌ ERROR_INVALID_TASK_DATA response
{
error: -1,
statusText: '400 Bad Request',
apiResponse: {
errorCode: "ERROR_INVALID_TASK_DATA",
errorDescription: "clientKey error",
errorId: 1
}
}
Method | Returns |
---|---|
await handler.balance() | directly the float value or an error object |
await handler.getBalance() | succes or error object |
await handler.runAnyTask(taskData) | handle task results for a taskData schema passed. In order to build this object, use !reffered docs and check parameters by catpcha task type. |
taskData
schema it's shown in examples.proxyInfo
schema has 2 versions:{ 'proxy' : 'proxyType:proxyAddress:proxyPort:proxyLogin:proxyPassword' }
or
{ 'proxyType': 'http', 'proxyAddress': 'ip_address', 'proxyPort': 3221, 'proxyLogin': 'username', 'proxyPassword': 'password' }
(proxyLogin & proxyPassword are optionals)
retrieve solutions (tokens/coordenates) with the followings:
// * check required parameters for a website with API docs.
await handler.hcaptcha(websiteURL, websiteKey, proxyInfo, userAgent, isInvisible, enterprisePayload)
await handler.hcaptchaproxyless(websiteURL, websiteKey, userAgent, isInvisible, enterprisePayload)
await handler.hcaptchaclassification(question, queries, coordinate)
await handler.recaptchav2(websiteURL, websiteKey, proxyInfo, userAgent, isInvisible, recaptchaDataSValue, cookies)
await handler.recaptchav2proxyless(websiteURL, websiteKey, userAgent, isInvisible, recaptchaDataSValue, cookies)
await handler.recaptchav2enterprise(websiteURL, websiteKey, proxyInfo, userAgent, enterprisePayload, apiDomain, cookies)
await handler.recaptchav2enterpriseproxyless(websiteURL, websiteKey, userAgent, enterprisePayload, apiDomain, cookies)
await handler.recaptchav3(websiteURL, websiteKey, proxyInfo, pageAction, minScore)
await handler.recaptchav3proxyless(websiteURL, websiteKey, pageAction, minScore)
await handler.datadome(websiteURL, userAgent, captchaUrl, proxyInfo)
await handler.funcaptcha(websiteURL, websitePublicKey, proxyInfo, funcaptchaApiJSSubdomain, userAgent, data)
await handler.funcaptchaproxyless(websiteURL, websitePublicKey, funcaptchaApiJSSubdomain, userAgent, data)
await handler.funcaptchaclassification(image, question)
await handler.geetest(websiteURL, gt, challenge, geetestApiServerSubdomain, proxyInfo, version, userAgent, geetestGetLib, initParameters)
await handler.geetestproxyless(websiteURL, gt, challenge, geetestApiServerSubdomain, version, userAgent, geetestGetLib, initParameters)
await handler.image2text(body)
await handler.antikasada(pageURL, proxyInfo, onlyCD, userAgent) // *: pageUrl & proxyInfo are always required
await handler.antiakamaibmp(packageName, version, deviceId, deviceName, count) // *: packageName it's always required
pass null instead of empty for optional arguments
Currently unsupported API methods: ❌ ReCaptchaV2Classification
const handler = new Captchaai(apikey, verbose); // on handler initialization
Verbose level undefined || 0
: Dont print logs, just get response.
Verbose level 1
: Print logs about performed requests during execution.
Verbose level 2
: Appends full captchaai api response in verbose level 1 outputs.
HCaptchaClassification: Recognize the images that you need to click.
.hcaptchaclassification(question, queries, coordinate)
.puppeteer-extra
.FunCaptchaClassification (beta): Recognize the images that you need to click.
.funcaptchaclassification(image, question)
.AntiKasadaTask: Solving Kasada.
AntiAkamaiBMPTask: Solving Akamai Mobile.
FAQs
Want you to get verified captcha **tokens** calling one function within your NodeJS application?
The npm package captchaai-npm receives a total of 0 weekly downloads. As such, captchaai-npm popularity was classified as not popular.
We found that captchaai-npm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.