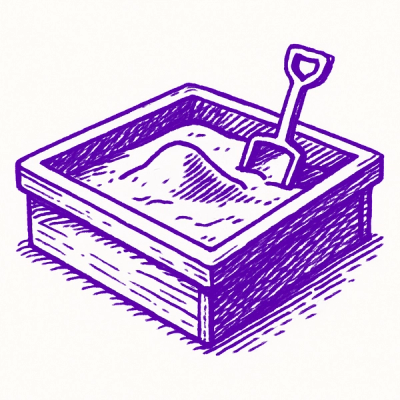
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
chai-openapi-response-validator
Advanced tools
Use Chai to assert that HTTP responses satisfy an OpenAPI spec
Use Chai to assert that HTTP responses satisfy an OpenAPI spec.
If your server's behaviour doesn't match your API documentation, then you need to correct your server, your documentation, or both. The sooner you know the better.
This plugin lets you automatically test whether your server's behaviour and documentation match. It extends the Chai Assertion Library to support the OpenAPI standard for documenting REST APIs. In your JavaScript tests, you can simply assert expect(responseObject).to.satisfyApiSpec
Features:
$ref
in response definitions (i.e. $ref: '#/definitions/ComponentType/ComponentName'
)axios
, request-promise
, supertest
, superagent
, and chai-http
If you've come here to help contribute - thanks! Take a look at the contributing docs to get started.
npm install --save-dev chai-openapi-response-validator
yarn add --dev chai-openapi-response-validator
ES6 / TypeScript
import chaiResponseValidator from 'chai-openapi-response-validator';
CommonJS / JavaScript
const chaiResponseValidator = require('chai-openapi-response-validator').default;
// Set up Chai
import chai from 'chai';
const expect = chai.expect;
// Import this plugin
import chaiResponseValidator from 'chai-openapi-response-validator';
// Load an OpenAPI file (YAML or JSON) into this plugin
chai.use(chaiResponseValidator('path/to/openapi.yml'));
// Write your test (e.g. using Mocha)
describe('GET /example/endpoint', () => {
it('should satisfy OpenAPI spec', async () => {
// Get an HTTP response from your server (e.g. using axios)
const res = await axios.get('http://localhost:3000/example/endpoint');
expect(res.status).to.equal(200);
// Assert that the HTTP response satisfies the OpenAPI spec
expect(res).to.satisfyApiSpec;
});
});
path/to/openapi.yml
):openapi: 3.0.0
info:
title: Example API
version: 1.0.0
paths:
/example:
get:
responses:
200:
description: Response body should be an object with fields 'stringProperty' and 'integerProperty'
content:
application/json:
schema:
type: object
required:
- stringProperty
- integerProperty
properties:
stringProperty:
type: string
integerProperty:
type: integer
openapi.yml
:// Response includes:
{
status: 200,
body: {
stringProperty: 'string',
integerProperty: 123,
},
};
// Response includes:
{
status: 200,
body: {
stringProperty: 'string',
integerProperty: 'invalid (should be an integer)',
},
};
AssertionError: expected res to satisfy API spec
expected res to satisfy the '200' response defined for endpoint 'GET /example/endpoint' in your API spec
res did not satisfy it because: integerProperty should be integer
res contained: {
body: {
stringProperty: 'string',
integerProperty: 'invalid (should be an integer)'
}
}
}
The '200' response defined for endpoint 'GET /example/endpoint' in API spec: {
'200': {
description: 'Response body should be a string',
content: {
'application/json': {
schema: {
type: 'string'
}
}
}
},
}
// Set up Chai
import chai from 'chai';
const expect = chai.expect;
// Import this plugin and the function you want to test
import chaiResponseValidator from 'chai-openapi-response-validator';
import { functionToTest } from 'path/to/your/code';
// Load an OpenAPI file (YAML or JSON) into this plugin
chai.use(chaiResponseValidator('path/to/openapi.yml'));
// Write your test (e.g. using Mocha)
describe('functionToTest()', () => {
it('should satisfy OpenAPI spec', async () => {
// Assert that the function returns a value satisfying a schema defined in your OpenAPI spec
expect(functionToTest()).to.satisfySchemaInApiSpec('ExampleSchemaObject');
});
});
path/to/openapi.yml
):openapi: 3.0.0
info:
title: Example API
version: 1.0.0
paths:
/example:
get:
responses:
200:
description: Response body should be an ExampleSchemaObject
content:
application/json:
schema: '#/components/schemas/ExampleSchemaObject'
components:
schemas:
ExampleSchemaObject:
type: object
required:
- stringProperty
- integerProperty
properties:
stringProperty:
type: string
integerProperty:
type: integer
ExampleSchemaObject
:// object includes:
{
stringProperty: 'string',
integerProperty: 123,
};
ExampleSchemaObject
:// object includes:
{
stringProperty: 123,
integerProperty: 123,
};
AssertionError: expected object to satisfy schema 'ExampleSchemaObject' defined in API spec:
object did not satisfy it because: stringProperty should be string
object was: {
{
stringProperty: 123,
integerProperty: 123
}
}
}
The 'ExampleSchemaObject' schema in API spec: {
type: 'object',
required: [
'stringProperty'
'integerProperty'
],
properties: {
stringProperty: {
type: 'string'
},
integerProperty: {
type: 'integer'
}
}
}
// Set up Chai
import chai from 'chai';
const expect = chai.expect;
// Import this plugin
import chaiResponseValidator from 'chai-openapi-response-validator';
// Get an object representing your OpenAPI spec
const openApiSpec = {
openapi: '3.0.0',
info: {
title: 'Example API',
version: '0.1.0',
},
paths: {
'/example/endpoint': {
get: {
responses: {
200: {
description: 'Response body should be a string',
content: {
'application/json': {
schema: {
type: 'string',
},
},
},
},
},
},
},
},
};
// Load that OpenAPI object into this plugin
chai.use(chaiResponseValidator(openApiSpec));
// Write your test (e.g. using Mocha)
describe('GET /example/endpoint', () => {
it('should satisfy OpenAPI spec', async () => {
// Get an HTTP response from your server (e.g. using axios)
const res = await axios.get('http://localhost:3000/example/endpoint');
expect(res.status).to.equal(200);
// Assert that the HTTP response satisfies the OpenAPI spec
expect(res).to.satisfyApiSpec;
});
});
// Set up Chai
import chai from 'chai';
const expect = chai.expect;
// Import this plugin and an HTTP client (e.g. axios)
import chaiResponseValidator from 'chai-openapi-response-validator';
import axios from 'axios';
// Write your test (e.g. using Mocha)
describe('GET /example/endpoint', () => {
// Load your OpenAPI spec from a web endpoint
before(async () => {
const response = await axios.get('url/to/openapi/spec');
const openApiSpec = response.data; // e.g. { openapi: '3.0.0', <etc> };
chai.use(chaiResponseValidator(openApiSpec));
});
it('should satisfy OpenAPI spec', async () => {
// Get an HTTP response from your server (e.g. using axios)
const res = await axios.get('http://localhost:3000/example/endpoint');
expect(res.status).to.equal(200);
// Assert that the HTTP response satisfies the OpenAPI spec
expect(res).to.satisfyApiSpec;
});
});
FAQs
Use Chai to assert that HTTP responses satisfy an OpenAPI spec
The npm package chai-openapi-response-validator receives a total of 5,997 weekly downloads. As such, chai-openapi-response-validator popularity was classified as popular.
We found that chai-openapi-response-validator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.