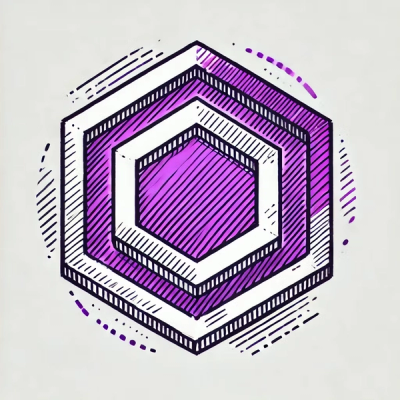
Security News
ESLint Adds Official Support for Linting HTML
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
chartjs-color
Advanced tools
The chartjs-color npm package is a utility for color manipulation and conversion, designed to work seamlessly with Chart.js. It allows users to create, manipulate, and convert colors in various formats.
Color Creation
This feature allows you to create color objects from color names, hex codes, or RGB values. The example demonstrates creating a color object from the color name 'red' and converting it to a hex string.
const Color = require('chartjs-color');
const red = Color('red');
console.log(red.hexString()); // Outputs: #ff0000
Color Manipulation
This feature allows you to manipulate colors by lightening, darkening, or adjusting their saturation. The example shows how to lighten the color red by 50%.
const Color = require('chartjs-color');
const red = Color('red');
const lightRed = red.lighten(0.5);
console.log(lightRed.hexString()); // Outputs a lighter shade of red
Color Conversion
This feature allows you to convert colors between different formats such as RGB, HSL, and hex. The example demonstrates converting the color red to RGB and HSL string formats.
const Color = require('chartjs-color');
const red = Color('red');
console.log(red.rgbString()); // Outputs: rgb(255, 0, 0)
console.log(red.hslString()); // Outputs: hsl(0, 100%, 50%)
The 'color' package is a comprehensive library for color conversion and manipulation. It supports a wide range of color models and provides extensive methods for color transformations. Compared to chartjs-color, it offers more advanced features and flexibility for complex color operations.
The 'tinycolor2' package is a lightweight color manipulation library that supports color conversion and manipulation. It is known for its simplicity and ease of use, making it a good alternative for basic color operations. While it may not be as feature-rich as 'color', it is more lightweight and easier to integrate for simple tasks.
The 'chroma-js' package is a powerful library for color manipulation and conversion, with a focus on color scales and interpolation. It is particularly useful for creating color gradients and palettes. Compared to chartjs-color, chroma-js offers more advanced features for color scale generation and interpolation.
JavaScript library for color conversion and manipulation with support for CSS color strings.
var color = Color("#7743CE");
color.alpha(0.5).lighten(0.5);
console.log(color.hslString()); // "hsla(262, 59%, 81%, 0.5)"
$ npm install color
var Color = require("color")
var color = Color("rgb(255, 255, 255)")
var color = Color({r: 255, g: 255, b: 255})
var color = Color().rgb(255, 255, 255)
var color = Color().rgb([255, 255, 255])
Pass any valid CSS color string into Color()
or a hash of values. Also load in color values with rgb()
, hsl()
, hsv()
, hwb()
, and cmyk()
.
color.red(120)
Set the values for individual channels with alpha
, red
, green
, blue
, hue
, saturation
(hsl), saturationv
(hsv), lightness
, whiteness
, blackness
, cyan
, magenta
, yellow
, black
color.rgb() // {r: 255, g: 255, b: 255}
Get a hash of the rgb values with rgb()
, similarly for hsl()
, hsv()
, and cmyk()
color.rgbArray() // [255, 255, 255]
Get an array of the values with rgbArray()
, hslArray()
, hsvArray()
, and cmykArray()
.
color.red() // 255
Get the value for an individual channel.
color.hslString() // "hsl(320, 50%, 100%)"
Different CSS String formats for the color are on hexString
, rgbString
, percentString
, hslString
, hwbString
, and keyword
(undefined if it's not a keyword color). "rgba"
and "hsla"
are used if the current alpha value of the color isn't 1
.
color.luminosity(); // 0.412
The WCAG luminosity of the color. 0 is black, 1 is white.
color.contrast(Color("blue")) // 12
The WCAG contrast ratio to another color, from 1 (same color) to 21 (contrast b/w white and black).
color.light(); // true
color.dark(); // false
Get whether the color is "light" or "dark", useful for deciding text color.
color.negate() // rgb(0, 100, 255) -> rgb(255, 155, 0)
color.lighten(0.5) // hsl(100, 50%, 50%) -> hsl(100, 50%, 75%)
color.darken(0.5) // hsl(100, 50%, 50%) -> hsl(100, 50%, 25%)
color.saturate(0.5) // hsl(100, 50%, 50%) -> hsl(100, 75%, 50%)
color.desaturate(0.5) // hsl(100, 50%, 50%) -> hsl(100, 25%, 50%)
color.greyscale() // #5CBF54 -> #969696
color.whiten(0.5) // hwb(100, 50%, 50%) -> hwb(100, 75%, 50%)
color.blacken(0.5) // hwb(100, 50%, 50%) -> hwb(100, 50%, 75%)
color.clearer(0.5) // rgba(10, 10, 10, 0.8) -> rgba(10, 10, 10, 0.4)
color.opaquer(0.5) // rgba(10, 10, 10, 0.8) -> rgba(10, 10, 10, 1.0)
color.rotate(180) // hsl(60, 20%, 20%) -> hsl(240, 20%, 20%)
color.rotate(-90) // hsl(60, 20%, 20%) -> hsl(330, 20%, 20%)
color.mix(Color("yellow")) // cyan -> rgb(128, 255, 128)
color.mix(Color("yellow"), 0.3) // cyan -> rgb(77, 255, 179)
// chaining
color.green(100).greyscale().lighten(0.6)
You can can create a copy of an existing color object using clone()
:
color.clone() // -> New color object
And more to come...
The API was inspired by color-js. Manipulation functions by CSS tools like Sass, LESS, and Stylus.
FAQs
Color conversion and manipulation with CSS string support
The npm package chartjs-color receives a total of 718,915 weekly downloads. As such, chartjs-color popularity was classified as popular.
We found that chartjs-color demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.