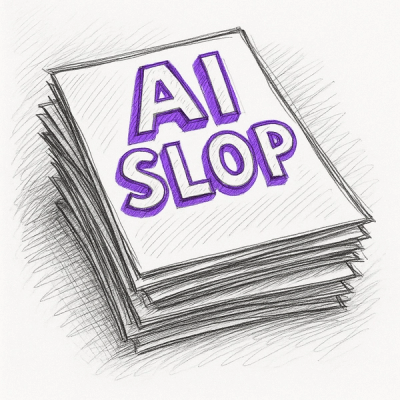
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
checked-exceptions
Advanced tools
A utility library to create and manage checked exceptions in typescript
A utility library to create and manage checked exceptions in typescript.
npm:
npm i checked-exceptions --save
yarn:
yarn add checked-exceptions
A checked exception can be created by just importing check
function for the library and passing it the type
of the exception.
import {check} from 'checked-exceptions'
const NotImplemented = check('NotImplemented')
throw new NotImplemented()
Once a checked exception class has been created, an instance of the checked exception can be created using the new
operator or using the static of()
function, for eg:
Using the new operator
const err = new NotImplemented()
Using the of function
const err = NotImplemented.of()
A custom exception with additional meta data can be created by passing a second argument for eg:
type User = {id: number; name: string}
const UserIdNotFound = check(
'UserIdNotFound',
(user: User) => `Could not find user with id: ${user.id}`
)
throw new UserIdNotFound({id: 1900, name: 'Foo'})
The second argument is of type function
and is used to generate the message string when the exception is thrown.
The default properties of an exception such as stack
and message
work like they do in a typical Error
object. Additional properties such as data
, type
is also added, for eg:
type User = {id: number; name: string}
const UserIdNotFound = check(
'UserIdNotFound',
(user: User) => `Could not find user with id: ${user.id}`
)
const err = new UserIdNotFound({id: 1900, name: 'Foo'})
console.log(err.data.id) // prints 1900
console.log(err.type) // prints 'UserIdNotFound'
FAQs
A utility library to create and manage checked exceptions in typescript
The npm package checked-exceptions receives a total of 1,430 weekly downloads. As such, checked-exceptions popularity was classified as popular.
We found that checked-exceptions demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.