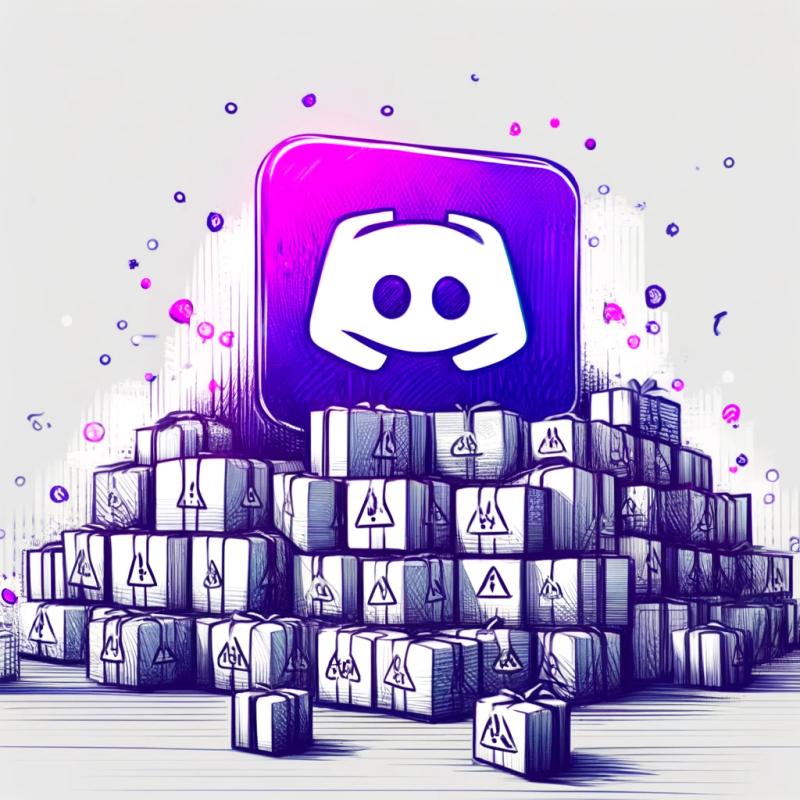
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
clamp-it
Advanced tools
Readme
Clamps numbers to given bounds.
npm install clamp-it --save
You may find yourself needing a number to be at least a certain
value. So you used Math.max()
:
value = Math.max(0, value); // make sure value is at least 0
You may find yourself needing a number to be at most a certain
value. So you used Math.min()
:
value = Math.min(100, value); // value cannot exceed 100
You may find yourself needing a number to be within a closed range.
So you used both Math.min()
and Math.max()
:
value = Math.min(100, Math.min(0, value)); // value must be in [0, 100]
And you may ask yourself: How did we get here? Why am I using max
to
specify a minimum. Why am I using min
to specify a maximum. This
isn't my beautiful house! This isn't my beautiful wife!
Introducing clamp-it
— clamps values using easy-to-understand
language!
Need a number to be at least a certain value?
const { atLeast } = require("clamp-it");
// later:
value = atLeast(0).clamp(value);
Need a number to be at most a certain value?
const { atMost } = require("clamp-it");
// later:
value = atMost(100).clamp(value);
Need a number to be within a closed range?
const { within } = require("clamp-it");
// later:
value = within(0, 100).clamp(value);
Or even spicier: 🌶
const { atLeast } = require("clamp-it");
// later:
value = atLeast(0).atMost(100).clamp(value);
No. You can clamp values the old-fashioned way with min()
or max()
.
Or you could write helper functions that delegate to min()
and max()
like
so (go ahead and copy-paste this):
function atLeast(bound, value) {
return Math.max(bound, value);
}
function atMost(bound, value) {
return Math.min(bound, value);
}
function within(lower, upper, value) {
return Math.min(upper, Math.max(lower, value));
}
I created this repo mostly as an exercise in API design and because I wanted to try property-based testing in TypeScript.
I wanted an API in which mistakes in its usage would be obvious. Hence
the names atLeast()
, atMost()
, and within()
. I wanted to be able
to chain the atLeast()
with atMost()
to create a self-documenting
way to specify a closed range.
I also tested a whole bunch of properties and had to decided whether
using NaNs
as a bound made any sense (spoilers: no).
I don't intend clamp-it
to be used in production, but you absolutely
can.
FAQs
Clamps values within ranges
We found that clamp-it demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.