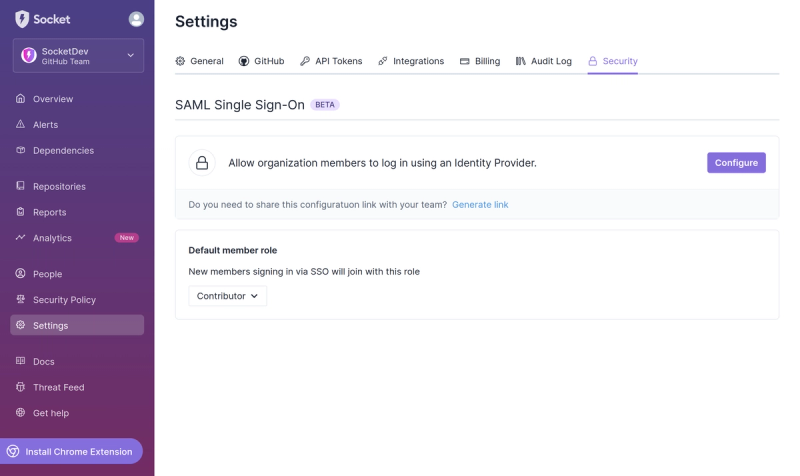
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
class-mocker
Advanced tools
Readme
Class-mocker is library for mocking dependencies.
$ npm i --save-dev class-mocker
For example you has controller and repository. The controller is curled from the repository.
Repository example
export class UserRepository {
private readonly data: Map<number, User> = new Map<number, User>();
constructor() {
// Initialize data for emulation requests to database
for (let id = 0; id < 5; id++) {
this.data.set(id, new User(id, `User${id}`, `P@$$W0RD`,`user${id}@test-mail.it`));
}
}
async findById(id: number): Promise<User> {
// Emulate request to database
await awaitTimeout(1000);
return this.data.get(id);
}
async findAll(): Promise<User[]> {
// Emulate request to database
await awaitTimeout(1000);
return Array.of(...this.data.values())
}
}
function awaitTimeout(timeout: number): Promise<void> {
return new Promise<void>(resolve => setTimeout(resolve, timeout));
}
Controller example
export class UserController {
constructor(private readonly userRepository: UserRepository) {
}
async find(id: number): Promise<Response<User | string>> {
try {
const user = await this.userRepository.findById(id);
if (!user) {
return new Response(404, 'Not found.');
}
return new Response(200, user);
} catch (e) {
return new Response(500, 'Internal server error.');
}
}
async findAll(): Promise<Response<User[] | string>> {
try {
return new Response(200, await this.userRepository.findAll());
} catch (e) {
return new Response(500, 'Internal server error.');
}
}
}
Mock creation
Mock by return
const userRepositoryMock = new Mock(UserRepository)
.expect('findAll', {return: []});
Mock on call
const userRepositoryMock = new Mock(UserRepository)
.expect('findById', {call: (id: number) => {
expect(id).toBe(2); // Check argument
return new User(2, 'ReplacedUser2', 'P@$$W0RD', 'replaced2@test-mail.it');
}});
Mock on thrown error
const userRepositoryMock = new Mock(UserRepository)
.expect('findAll', {throw: new Error('Database is not available')});
Mock by order
const userRepositoryMock = new Mock(UserRepository)
.expect('findById', {
at: 1,
return: new User(4, 'ReplacedUser4', 'P@$$W0RD', 'replaced2@test-mail.it'),
})
.expect('findById', {at: 2, return: null});
Enjoy!
FAQs
Class-mocker is library for mocking dependencies.
The npm package class-mocker receives a total of 4 weekly downloads. As such, class-mocker popularity was classified as not popular.
We found that class-mocker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.