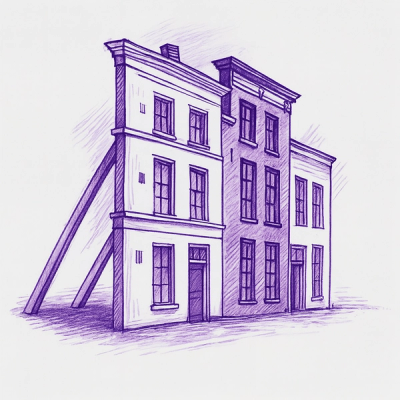
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
1. Install: ~~~ npm install codebeat ~~~ 2. Import: ~~~ const Codebeat = require('codebeat') ~~~ 3. Instantiate with voice config: ~~~ const melody = new Codebeat({ tempo: 128, //default is 60 instrumen
npm install codebeat
const Codebeat = require('codebeat')
const melody = new Codebeat({
tempo: 128, //default is 60
instrument: 'square', //default is 'sine'
timeSig: '3/4', //default is '4/4'
loop: true //default is false
})
melody.update({notes: `
t d4, t d_4,
t d4, q a#5,
q b5, q rest
`})
melody.play()
Note: Up to six audio contexts (voices) can be active on the window object. Each instance of Codebeat creates a new context.
Continue reading below to learn more about writting music in Codebeat.
Codebeat is a javascript library that provides a simple language for music composition.
A note's duration is the relative amount of time it is played, known as a subdivision. The names of common subdivisions are abbreviated as follows:
Note: 3 triplets = 2 eighths
A note's pitch is the name for its frequency in Hertz. D in the 5th octave is written as d5
. Similarly, G-flat in the 3rd octave is g_3
, and C-sharp in the 6th octave is c#6
. A pitch can be between a0
and g#7
, inclusive.
General format: [note_duration][space][note_pitch][comma]
In practice: h d5, q g_3, q c#6
etc.
Note: any amount of tabs, newlines, or whitespace can appear after the comma, but not between the duration and pitch
To simplify the writing process, groups of notes may be assigned to variables (separated with a semicolon) and repeated notes may be multiplied by an integer value.
motif = h d5, q g_3, q c#6;
t d4, motif,
t a4, motif
h c4 * 8, t b_3 * 3
To play multiple notes simultaneously, Codebeat provides the +
operator. Doing so produces a chord constituted of the pitches provided for the specified duration. The notes within a chord must be played for the same duration.
chord = h d3 + a3 + g3;
h d3, chord,
h a3, chord
Like physical instruments, Codebeat can alter the pitch of a note over time until it reaches a second pitch. This is done with the -
operator. Both notes are independent of each other, which means they may be any of the allowed durations and pitches.
slide = h e2 - z c3;
e f3, slide,
h b3, slide
However, slides cannot be chained as in the below example.
h e2 - z c3 - e f4 - h d3
FAQs
A browser-based audio programming language for music composition and audio synthesis.
The npm package codebeat receives a total of 3 weekly downloads. As such, codebeat popularity was classified as not popular.
We found that codebeat demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.