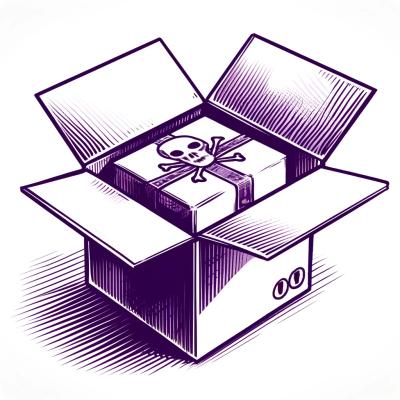
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
codemirror-json-schema
Advanced tools
Codemirror 6 extensions that provide full JSONSchema support for `@codemirror/lang-json` and `codemirror-json5`
Codemirror 6 extensions that provide full JSON Schema support for @codemirror/lang-json
& codemirror-json5
language modes
This is now a full-featured library for json schema for json
, json5
and yaml
as cm6 extensions!
StateField
and linting refreshschema.description
and custom formatHover
and formatError
configurationTo give you as much flexibility as possible, everything codemirror related is a peer or optional dependency
Based on whether you want to support json4, json5 or both, you will need to install the relevant language mode for our library to use.
formatHover
and/or formatError
configuration will be passed to markdown-it
which doesn't handle html by default.jsonSchema()
or json5Schema()
modes. See the custom usages below to learn how to use the new stateExtensions
and handleRefresh
exports.with auto-install-peers true
or similar:
npm install --save @codemirror/lang-json codemirror-json-schema
without auto-install-peers true
:
npm install --save @codemirror/lang-json codemirror-json-schema @codemirror/language @codemirror/lint @codemirror/view @codemirror/state @lezer/common
This sets up @codemirror/lang-json
and our extension for you.
If you'd like to have more control over the related configurations, see custom usage below
import { EditorState } from "@codemirror/state";
import { jsonSchema } from "codemirror-json-schema";
const schema = {
type: "object",
properties: {
example: {
type: "boolean",
},
},
};
const json5State = EditorState.create({
doc: "{ example: true }",
extensions: [jsonSchema(schema)],
});
This approach allows you to configure the json mode and parse linter, as well as our linter, hovers, etc more specifically.
import { EditorState } from "@codemirror/state";
import { linter } from "@codemirror/lint";
import { hoverTooltip } from "@codemirror/view";
import { json, jsonParseLinter, jsonLanguage } from "@codemirror/lang-json";
import {
jsonSchemaLinter,
jsonSchemaHover,
jsonCompletion,
stateExtensions,
handleRefresh
} from "codemirror-json-schema";
const schema = {
type: "object",
properties: {
example: {
type: "boolean",
},
},
};
const state = EditorState.create({
doc: `{ "example": true }`,
extensions: [
json(),
linter(jsonParseLinter(), {
// default is 750ms
delay: 300
}),
linter(jsonSchemaLinter(), {
needsRefresh: handleRefresh,
}),
jsonLanguage.data.of({
autocomplete: jsonCompletion(),
}),
hoverTooltip(jsonSchemaHover()),
stateExtensions(schema)
];
})
with auto-install-peers true
or similar:
npm install --save codemirror-json5 codemirror-json-schema
without auto-install-peers true
:
npm install --save codemirror-json5 codemirror-json-schema @codemirror/language @codemirror/lint @codemirror/view @codemirror/state @lezer/common
This sets up codemirror-json5
mode for you.
If you'd like to have more control over the related configurations, see custom usage below
import { EditorState } from "@codemirror/state";
import { json5Schema } from "codemirror-json-schema/json5";
const schema = {
type: "object",
properties: {
example: {
type: "boolean",
},
},
};
const json5State = EditorState.create({
doc: `{
example: true,
// json5 is awesome!
}`,
extensions: [json5Schema(schema)],
});
This approach allows you to configure the json5 mode and parse linter, as well as our linter, hovers, etc more specifically.
import { EditorState } from "@codemirror/state";
import { linter } from "@codemirror/lint";
import { json5, json5ParseLinter, json5Language } from "codemirror-json5";
import {
json5SchemaLinter,
json5SchemaHover,
json5Completion,
} from "codemirror-json-schema/json5";
import { stateExtensions, handleRefresh } from "codemirror-json-schema";
const schema = {
type: "object",
properties: {
example: {
type: "boolean",
},
},
};
const json5State = EditorState.create({
doc: `{
example: true,
// json5 is awesome!
}`,
extensions: [
json5(),
linter(json5ParseLinter(), {
// the default linting delay is 750ms
delay: 300,
}),
linter(
json5SchemaLinter({
needsRefresh: handleRefresh,
})
),
hoverTooltip(json5SchemaHover()),
json5Language.data.of({
autocomplete: json5Completion(),
}),
stateExtensions(schema),
],
});
If you want to, you can provide schema dynamically, in several ways.
This works the same for either json or json5, using the underlying codemirror 6 StateFields, via the updateSchema
method export.
In this example
jsonSchema()
and json5Schema()
modesimport { EditorState } from "@codemirror/state";
import { EditorView } from "@codemirror/view";
import { json5Schema } from "codemirror-json-schema/json5";
import { updateSchema } from "codemirror-json-schema";
const json5State = EditorState.create({
doc: `{
example: true,
// json5 is awesome!
}`,
// note: you can still provide initial
// schema when creating state
extensions: [json5Schema()],
});
const editor = new EditorView({ state: json5State });
const schemaSelect = document.getElementById("schema-selection");
schemaSelect!.onchange = async (e) => {
const val = e.target!.value!;
if (!val) {
return;
}
// parse the remote schema spec to json
const data = await (
await fetch(`https://json.schemastore.org/${val}`)
).json();
// this will update the schema state field, in an editor specific way
updateSchema(editor, data);
};
if you are using the "custom path" with this approach, you will need to configure linting refresh as well:
import { linter } from "@codemirror/lint";
import { json5SchemaLinter } from "codemirror-json-schema/json5";
import { handleRefresh } from "codemirror-json-schema";
const state = EditorState.create({
// ...
extensions: [
linter(json5SchemaLinter(), {
needsRefresh: handleRefresh,
})
];
}
monaco-json
and monaco-yaml
both provide json schema features for json, cson and yaml, and we want the nascent codemirror 6 to have them as well!
Also, json5 is slowly growing in usage, and it needs full language support for the browser!
0.7.9
#133 4fd7cc6
Thanks @imolorhe! - Get sub schema using parsed data for additional context
#137 29e2da5
Thanks @xdavidwu! - Fix description markdown rendering in completion
#144 ef7f336
Thanks @imolorhe! - updated to use fine grained shiki bundle
#139 bfbe613
Thanks @NickTomlin! - Move non essential packages to devDependencies
#140 bceace2
Thanks @NickTomlin! - Add CONTRIBUTING.md file
FAQs
Codemirror 6 extensions that provide full JSONSchema support for `@codemirror/lang-json` and `codemirror-json5`
The npm package codemirror-json-schema receives a total of 15,705 weekly downloads. As such, codemirror-json-schema popularity was classified as popular.
We found that codemirror-json-schema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.