command-line-usage
Advanced tools
Comparing version
'use strict'; | ||
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol ? "symbol" : typeof obj; }; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
var tableLayout = require('table-layout'); | ||
var ansi = require('ansi-escape-sequences'); | ||
var os = require('os'); | ||
var t = require('typical'); | ||
var UsageOptions = require('./usage-options'); | ||
var OptionList = require('./option-list'); | ||
var Content = require('./content'); | ||
var arrayify = require('array-back'); | ||
module.exports = getUsage; | ||
module.exports = commandLineUsage; | ||
var Lines = function () { | ||
function Lines() { | ||
_classCallCheck(this, Lines); | ||
this.list = []; | ||
} | ||
_createClass(Lines, [{ | ||
key: 'add', | ||
value: function add(content) { | ||
var _this = this; | ||
arrayify(content).forEach(function (line) { | ||
return _this.list.push(ansi.format(line)); | ||
}); | ||
} | ||
}, { | ||
key: 'emptyLine', | ||
value: function emptyLine() { | ||
this.list.push(''); | ||
} | ||
}, { | ||
key: 'header', | ||
value: function header(text) { | ||
if (text) { | ||
this.add(ansi.format(text, ['underline', 'bold'])); | ||
this.emptyLine(); | ||
function commandLineUsage(sections) { | ||
sections = arrayify(sections); | ||
if (sections.length) { | ||
var output = sections.map(function (section) { | ||
if (section.optionList) { | ||
return new OptionList(section); | ||
} else if (section.content) { | ||
return new Content(section); | ||
} | ||
} | ||
}, { | ||
key: 'toString', | ||
value: function toString() { | ||
return this.list.join(os.EOL); | ||
} | ||
}]); | ||
return Lines; | ||
}(); | ||
function getUsage(sections) { | ||
if (sections && sections.length) { | ||
var _ret = function () { | ||
var output = new Lines(); | ||
sections.forEach(function (section) { | ||
if (section.optionList) { | ||
if (section.hide && section.hide.length) { | ||
section.optionList = section.optionList.filter(function (definition) { | ||
return section.hide.indexOf(definition.name) === -1; | ||
}); | ||
} | ||
output.header(section.header); | ||
output.add(optionList(section.optionList, section.group)); | ||
output.emptyLine(); | ||
} else if (section.content) { | ||
output.add(renderSection(section.header, section.content)); | ||
} else if (section.banner) { | ||
output.header(section.header); | ||
output.add(section.banner); | ||
} | ||
}); | ||
return { | ||
v: '\n' + output | ||
}; | ||
}(); | ||
if ((typeof _ret === 'undefined' ? 'undefined' : _typeof(_ret)) === "object") return _ret.v; | ||
} | ||
} | ||
function optionList(definitions, group) { | ||
if (!definitions || definitions && !definitions.length) { | ||
throw new Error('you must pass option definitions to getUsage.optionList()'); | ||
} | ||
var columns = []; | ||
if (group === '_none') { | ||
definitions = definitions.filter(function (def) { | ||
return !t.isDefined(def.group); | ||
}); | ||
} else if (group) { | ||
definitions = definitions.filter(function (def) { | ||
return arrayify(def.group).indexOf(group) > -1; | ||
}); | ||
return '\n' + output.join('\n'); | ||
} | ||
definitions.forEach(function (def) { | ||
columns.push({ | ||
option: getOptionNames(def, 'bold'), | ||
description: ansi.format(def.description) | ||
}); | ||
}); | ||
return tableLayout.lines(columns, { | ||
padding: { left: ' ', right: ' ' }, | ||
columns: [{ name: 'option', nowrap: true }, { name: 'description', maxWidth: 80 }] | ||
}); | ||
} | ||
function getOptionNames(definition, optionNameStyles) { | ||
var names = []; | ||
var type = definition.type ? definition.type.name.toLowerCase() : ''; | ||
var multiple = definition.multiple ? '[]' : ''; | ||
if (type) type = type === 'boolean' ? '' : '[underline]{' + type + multiple + '}'; | ||
type = ansi.format(definition.typeLabel || type); | ||
if (definition.alias) { | ||
names.push(ansi.format('-' + definition.alias, optionNameStyles)); | ||
} | ||
names.push(ansi.format('--' + definition.name, optionNameStyles) + ' ' + type); | ||
return names.join(', '); | ||
} | ||
function renderSection(header, content) { | ||
var lines = new Lines(); | ||
if (header) { | ||
lines.header(header); | ||
} | ||
if (!content) { | ||
return lines.list; | ||
} else { | ||
var _ret2 = function () { | ||
var defaultPadding = { left: ' ', right: ' ' }; | ||
if (t.isString(content)) { | ||
lines.add(tableLayout.lines({ column: content }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})); | ||
} else if (Array.isArray(content) && content.every(t.isString)) { | ||
content.forEach(function (row) { | ||
lines.add(tableLayout.lines({ column: row }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})); | ||
}); | ||
lines.add(); | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
lines.add(tableLayout.lines(content, { | ||
padding: defaultPadding | ||
})); | ||
} else if (t.isPlainObject(content)) { | ||
if (!content.options || !content.data) { | ||
throw new Error('must have an "options" or "data" property\n' + JSON.stringify(content)); | ||
} | ||
Object.assign({ padding: defaultPadding }, content.options); | ||
lines.add(tableLayout.lines(content.data.map(function (row) { | ||
return ansiFormatRow(row); | ||
}), content.options)); | ||
} else { | ||
var message = 'invalid input - \'content\' must be a string, array of strings, or array of plain objects:\n\n' + JSON.stringify(content); | ||
throw new Error(message); | ||
} | ||
lines.emptyLine(); | ||
return { | ||
v: lines.list | ||
}; | ||
}(); | ||
if ((typeof _ret2 === 'undefined' ? 'undefined' : _typeof(_ret2)) === "object") return _ret2.v; | ||
} | ||
} | ||
function ansiFormatRow(row) { | ||
for (var key in row) { | ||
row[key] = ansi.format(row[key]); | ||
} | ||
return row; | ||
} |
@@ -11,5 +11,5 @@ module.exports = [ | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms. This description is needlessly long unless you count testing of the description column maxWidth useful.', | ||
name: 'timeout', description: 'Timeout value in ms.', | ||
alias: 't', type: Number, typeLabel: '[underline]{ms}' | ||
} | ||
] |
@@ -7,3 +7,3 @@ const getUsage = require('../') | ||
{ | ||
header: 'Brezhnev', | ||
header: 'Soviet Union', | ||
content: { | ||
@@ -18,3 +18,3 @@ options: { | ||
{ | ||
one: 'On his 70th birthday he was awarded the rank of Marshal of the Soviet Union – the highest military honour in the Soviet Union. After being awarded the medal, he attended an 18th Army Veterans meeting, dressed in a long coat and saying; "Attention, Marshal\'s coming!" He also conferred upon himself the rare [bold]{Order of Victory} in 1978 — the only time the decoration was ever awarded outside of World War II. (This medal was posthumously revoked in 1989 for not meeting the criteria for citation.) \n\nBrezhnev\'s weakness for undeserved medals was proven by his poorly written memoirs recalling his military service during World War II, which treated the little-known and minor Battle of Novorossiysk as the decisive military theatre.', | ||
one: "At the beginning of World War II, Stalin signed a non-aggression pact with Hitler's Germany; the treaty delayed confrontation between the two countries.\n\nIn June 1941 the Germans invaded, opening the largest and bloodiest theater of war in history. Soviet war casualties accounted for the highest proportion of the conflict in the cost of acquiring the upper hand over Axis forces at intense battles such as Stalingrad. Soviet forces eventually captured Berlin in 1945.\n\nThe territory overtaken by the Red Army became satellite states of the Eastern Bloc. The Cold War emerged in 1947 as the Soviet bloc confronted the Western states that united in the North Atlantic Treaty Organization in 1949.", | ||
two: ussr | ||
@@ -21,0 +21,0 @@ } |
@@ -22,7 +22,8 @@ const getUsage = require('../') | ||
{ | ||
banner: [ | ||
content: [ | ||
'[italic]{This app was tested by dragons in Wales.}', | ||
'', | ||
wales | ||
] | ||
], | ||
raw: true | ||
} | ||
@@ -29,0 +30,0 @@ ] |
@@ -11,13 +11,12 @@ const getUsage = require('../') | ||
name: 'src', description: 'The input files to process', | ||
type: String, multiple: true, defaultOption: true, typeLabel: '[underline]{file} ...', | ||
group: 'main' | ||
multiple: true, defaultOption: true, typeLabel: '[underline]{file} ...', | ||
group: 'input' | ||
}, | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms', | ||
alias: 't', type: Number, typeLabel: '[underline]{ms}', | ||
alias: 't', typeLabel: '[underline]{ms}', | ||
group: 'main' | ||
}, | ||
{ | ||
name: 'plugin', description: 'A plugin path', | ||
type: String | ||
name: 'plugin', description: 'A plugin path', type: String | ||
} | ||
@@ -34,3 +33,3 @@ ] | ||
optionList: optionDefinitions, | ||
group: 'main' | ||
group: [ 'main', 'input' ] | ||
}, | ||
@@ -37,0 +36,0 @@ { |
const getUsage = require('../') | ||
const header = require('./assets/ansi-header') | ||
const ansi = require('ansi-escape-sequences') | ||
const optionDefinitions = require('./assets/example-options') | ||
const sections = [ | ||
{ | ||
banner: ansi.format(header, 'red') | ||
content: ansi.format(header, 'red'), | ||
raw: true | ||
}, | ||
@@ -10,0 +10,0 @@ { |
'use strict' | ||
const tableLayout = require('table-layout') | ||
const ansi = require('ansi-escape-sequences') | ||
const os = require('os') | ||
const t = require('typical') | ||
const UsageOptions = require('./usage-options') | ||
const OptionList = require('./option-list') | ||
const Content = require('./content') | ||
const arrayify = require('array-back') | ||
@@ -12,55 +9,21 @@ | ||
*/ | ||
module.exports = getUsage | ||
module.exports = commandLineUsage | ||
class Lines { | ||
constructor () { | ||
this.list = [] | ||
} | ||
add (content) { | ||
arrayify(content).forEach(line => this.list.push(ansi.format(line))) | ||
} | ||
emptyLine () { | ||
this.list.push('') | ||
} | ||
header (text) { | ||
if (text) { | ||
this.add(ansi.format(text, [ 'underline', 'bold' ])) | ||
this.emptyLine() | ||
} | ||
} | ||
toString () { | ||
return this.list.join(os.EOL) | ||
} | ||
} | ||
/** | ||
* @param {optionDefinition[]} - an array of [option definition](https://github.com/75lb/command-line-args#exp_module_definition--OptionDefinition) objects. In addition to the regular definition properties, command-line-usage will look for: | ||
* | ||
* - `description` - a string describing the option. | ||
* - `typeLabel` - a string to replace the default type string (e.g. `<string>`). It's often more useful to set a more descriptive type label, like `<ms>`, `<files>`, `<command>` etc. | ||
* | ||
* @param options {module:usage-options} - see [UsageOptions](#exp_module_usage-options--UsageOptions). | ||
* Generates a usage guide suitable for a command-line app. | ||
* @param {Section|Section[]} - One of more section objects ({@link module:command-line-usage~content} or {@link module:command-line-usage~optionList}). | ||
* @returns {string} | ||
* @alias module:command-line-usage | ||
*/ | ||
function getUsage (sections) { | ||
if (sections && sections.length) { | ||
const output = new Lines() | ||
sections.forEach(section => { | ||
function commandLineUsage (sections) { | ||
sections = arrayify(sections) | ||
if (sections.length) { | ||
const output = sections.map(section => { | ||
if (section.optionList) { | ||
/* filter out hidden definitions */ | ||
if (section.hide && section.hide.length) { | ||
section.optionList = section.optionList.filter(definition => section.hide.indexOf(definition.name) === -1) | ||
} | ||
output.header(section.header) | ||
output.add(optionList(section.optionList, section.group)) | ||
output.emptyLine() | ||
return new OptionList(section) | ||
} else if (section.content) { | ||
output.add(renderSection(section.header, section.content)) | ||
} else if (section.banner) { | ||
output.header(section.header) | ||
output.add(section.banner) | ||
return new Content(section) | ||
} | ||
}) | ||
return `\n${output}` | ||
return '\n' + output.join('\n') | ||
} | ||
@@ -70,114 +33,67 @@ } | ||
/** | ||
* A helper for getting a column-format list of options and descriptions. Useful for inserting into a custom usage template. | ||
* A Content section comprises a header and one or more lines of content. | ||
* @typedef content | ||
* @property header {string} - The section header, always bold and underlined. | ||
* @property content {string|string[]|object[]} - One or more lines of text. For table layout, supply the content as an array of objects. The property names of each object are not important, so long as they are consistent throughout the array. | ||
* @property raw {boolean} - Set to true to avoid indentation and wrapping. Useful for banners. | ||
* @example | ||
* Simple string of content. The syntax for ansi formatting is documented [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
* ```js | ||
* { | ||
* header: 'A typical app', | ||
* content: 'Generates something [italic]{very} important.' | ||
* } | ||
* ``` | ||
* | ||
* @param {optionDefinition[]} - the definitions to Display | ||
* @param [group] {string} - if specified, will output the options in this group. The special group `'_none'` will return options without a group specified. | ||
* @returns {string[]} | ||
* An array of strings is interpreted as lines, to be joined by the system newline character. | ||
* ```js | ||
* { | ||
* header: 'A typical app', | ||
* content: [ | ||
* 'First line.', | ||
* 'Second line.' | ||
* ] | ||
* } | ||
* ``` | ||
* | ||
* An array of recordset-style objects are rendered in table layout. | ||
* ```js | ||
* { | ||
* header: 'A typical app', | ||
* content: [ | ||
* { colA: 'First row, first column.', colB: 'First row, second column.'}, | ||
* { colA: 'Second row, first column.', colB: 'Second row, second column.'} | ||
* ] | ||
* } | ||
* ``` | ||
*/ | ||
function optionList (definitions, group) { | ||
if (!definitions || (definitions && !definitions.length)) { | ||
throw new Error('you must pass option definitions to getUsage.optionList()') | ||
} | ||
const columns = [] | ||
if (group === '_none') { | ||
definitions = definitions.filter(def => !t.isDefined(def.group)) | ||
} else if (group) { | ||
definitions = definitions.filter(def => arrayify(def.group).indexOf(group) > -1) | ||
} | ||
definitions.forEach(def => { | ||
columns.push({ | ||
option: getOptionNames(def, 'bold'), | ||
description: ansi.format(def.description) | ||
}) | ||
}) | ||
return tableLayout.lines(columns, { | ||
padding: { left: ' ', right: ' ' }, | ||
columns: [ | ||
{ name: 'option', nowrap: true }, | ||
{ name: 'description', maxWidth: 80 } | ||
] | ||
}) | ||
} | ||
function getOptionNames (definition, optionNameStyles) { | ||
const names = [] | ||
let type = definition.type ? definition.type.name.toLowerCase() : '' | ||
const multiple = definition.multiple ? '[]' : '' | ||
if (type) type = type === 'boolean' ? '' : `[underline]{${type}${multiple}}` | ||
type = ansi.format(definition.typeLabel || type) | ||
if (definition.alias) { | ||
names.push(ansi.format('-' + definition.alias, optionNameStyles)) | ||
} | ||
names.push(ansi.format(`--${definition.name}`, optionNameStyles) + ' ' + type) | ||
return names.join(', ') | ||
} | ||
function renderSection (header, content) { | ||
const lines = new Lines() | ||
if (header) { | ||
lines.header(header) | ||
} | ||
if (!content) { | ||
return lines.list | ||
} else { | ||
const defaultPadding = { left: ' ', right: ' ' } | ||
/* string content */ | ||
if (t.isString(content)) { | ||
lines.add(tableLayout.lines({ column: content }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})) | ||
/* array of strings */ | ||
} else if (Array.isArray(content) && content.every(t.isString)) { | ||
content.forEach(row => { | ||
lines.add(tableLayout.lines({ column: row }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})) | ||
}) | ||
lines.add() | ||
/* array of objects (use column layout) */ | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
lines.add(tableLayout.lines(content, { | ||
padding: defaultPadding | ||
})) | ||
/* { options: object, content: object[] } */ | ||
} else if (t.isPlainObject(content)) { | ||
if (!content.options || !content.data) { | ||
throw new Error('must have an "options" or "data" property\n' + JSON.stringify(content)) | ||
} | ||
Object.assign( | ||
{ padding: defaultPadding }, | ||
content.options | ||
) | ||
lines.add(tableLayout.lines( | ||
content.data.map(row => ansiFormatRow(row)), | ||
content.options | ||
)) | ||
} else { | ||
const message = `invalid input - 'content' must be a string, array of strings, or array of plain objects:\n\n${JSON.stringify(content)}` | ||
throw new Error(message) | ||
} | ||
lines.emptyLine() | ||
return lines.list | ||
} | ||
} | ||
function ansiFormatRow (row) { | ||
for (const key in row) { | ||
row[key] = ansi.format(row[key]) | ||
} | ||
return row | ||
} | ||
/** | ||
* A OptionList section adds a table displaying details of the available options. | ||
* @typedef optionList | ||
* @property [header] {string} - The section header, always bold and underlined. | ||
* @property optionList {OptionDefinition[]} - an array of [option definition](https://github.com/75lb/command-line-args#optiondefinition-) objects. In addition to the regular definition properties, command-line-usage will look for: | ||
* | ||
* - `description` - a string describing the option. | ||
* - `typeLabel` - a string to replace the default type string (e.g. `<string>`). It's often more useful to set a more descriptive type label, like `<ms>`, `<files>`, `<command>` etc. | ||
* @property [group] {string|string[]} - If specified, only options from this particular group will be printed. [Example](https://github.com/75lb/command-line-usage/blob/master/example/groups.js). | ||
* @property [hide] {string|string[]} - The names of one of more option definitions to hide from the option list. [Example](https://github.com/75lb/command-line-usage/blob/master/example/hide.js). | ||
* | ||
* @example | ||
* { | ||
* header: 'Options', | ||
* optionList: [ | ||
* { | ||
* name: 'help', alias: 'h', description: 'Display this usage guide.' | ||
* }, | ||
* { | ||
* name: 'src', description: 'The input files to process', | ||
* multiple: true, defaultOption: true, typeLabel: '[underline]{file} ...' | ||
* }, | ||
* { | ||
* name: 'timeout', description: 'Timeout value in ms. This description is needlessly long unless you count testing of the description column maxWidth useful.', | ||
* alias: 't', typeLabel: '[underline]{ms}' | ||
* } | ||
* ] | ||
* } | ||
*/ |
{ | ||
"name": "command-line-usage", | ||
"author": "Lloyd Brookes <75pound@gmail.com>", | ||
"version": "3.0.0", | ||
"version": "3.0.1", | ||
"description": "Generates command-line usage information", | ||
@@ -26,3 +26,3 @@ "repository": "https://github.com/75lb/command-line-usage.git", | ||
"array-back": "^1.0.3", | ||
"table-layout": "~0.2.0", | ||
"table-layout": "~0.2.1", | ||
"feature-detect-es6": "^1.3.0", | ||
@@ -32,3 +32,3 @@ "typical": "^2.4.2" | ||
"devDependencies": { | ||
"babel-preset-es2015": "^6.6.0", | ||
"babel-preset-es2015": "^6.9.0", | ||
"jsdoc-to-markdown": "^1.3.6", | ||
@@ -35,0 +35,0 @@ "tape": "^4.5.1" |
291
README.md
@@ -8,57 +8,94 @@ [](https://www.npmjs.org/package/command-line-usage) | ||
# command-line-usage | ||
A simple template to create a usage guide. It was extracted from [command-line-args](https://github.com/75lb/command-line-args) to facilitate arbitrary use. | ||
A simple module for creating a usage guide. | ||
## Synopis | ||
A usage guide is built from an arbitrary number of sections, e.g. a description section, synopsis, option list, examples, footer etc. Each section has a bold, underlined header and some content (a paragraph, table, option list, banner etc.) | ||
The <code><a href="#commandlineusagesections--string-">commandLineUsage()</a></code> function takes one or more `section` objects (<code><a href="#commandlineusagecontent">content</a></code> or <code><a href="#commandlineusageoptionlist">optionList</a></code>) as input. | ||
Inline ansi formatting can be used anywhere within section content using the formatting syntax described [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
This script: | ||
```js | ||
var getUsage = require('command-line-usage'); | ||
var usage = getUsage(definitions, options) | ||
const getUsage = require('command-line-usage') | ||
const sections = [ | ||
{ | ||
header: 'A typical app', | ||
content: 'Generates something [italic]{very} important.' | ||
}, | ||
{ | ||
header: 'Options', | ||
optionList: [ | ||
{ | ||
name: 'input', typeLabel: '[underline]{file}', | ||
description: 'The input to process.' | ||
}, | ||
{ | ||
name: 'help', description: 'Print this usage guide.' | ||
} | ||
] | ||
} | ||
] | ||
const usage = getUsage(sections) | ||
console.log(usage) | ||
``` | ||
Inline ansi formatting can be used anywhere within the usage template using the formatting syntax described [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
Outputs this guide: | ||
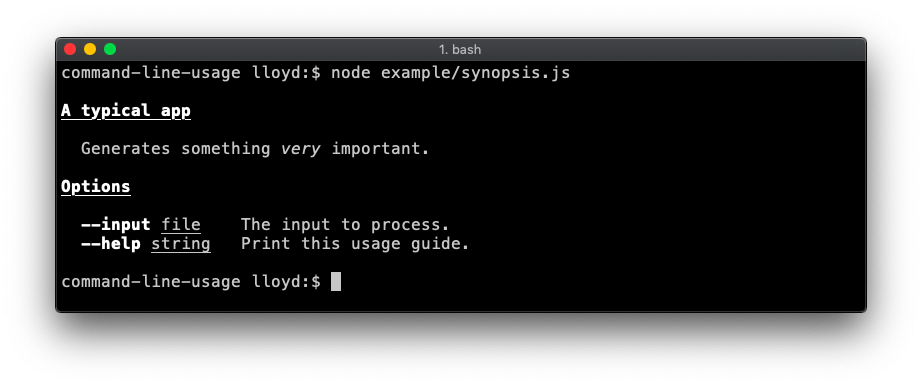 | ||
## Examples | ||
### Simple | ||
A `description` field is added to each option definition. A `title`, `description` and simple `footer` are set in the getUsage options. [Code](https://github.com/75lb/command-line-usage/blob/master/example/simple.js). | ||
A fairly typical usage guide with three sections - description, option list and footer. [Code](https://github.com/75lb/command-line-usage/blob/master/example/simple.js). | ||
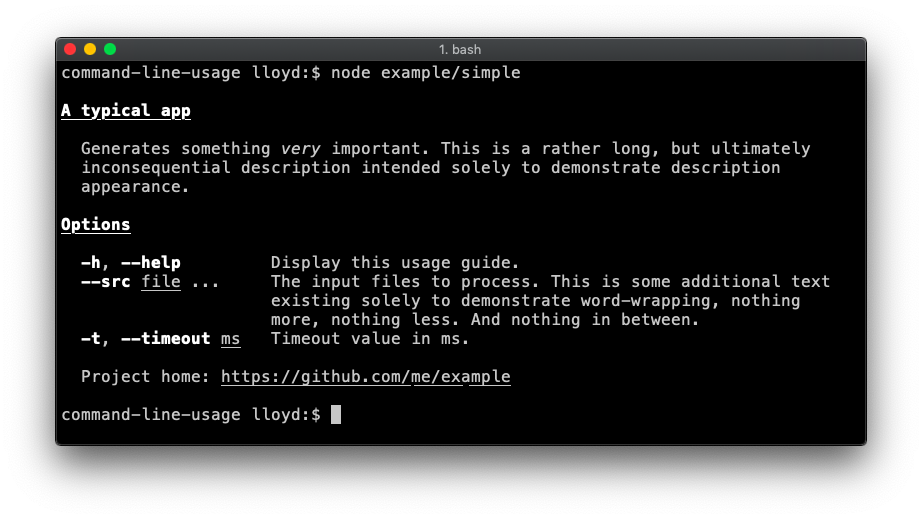 | ||
### Groups | ||
Demonstrates breaking the options up into groups. This example also sets a `typeLabel` on each option definition (e.g. a `typeLabel` value of `files` is more meaningful than the default `string[]`). [Code](https://github.com/75lb/command-line-usage/blob/master/example/groups.js). | ||
### Option List groups | ||
Demonstrates breaking the option list up into groups. [Code](https://github.com/75lb/command-line-usage/blob/master/example/groups.js). | ||
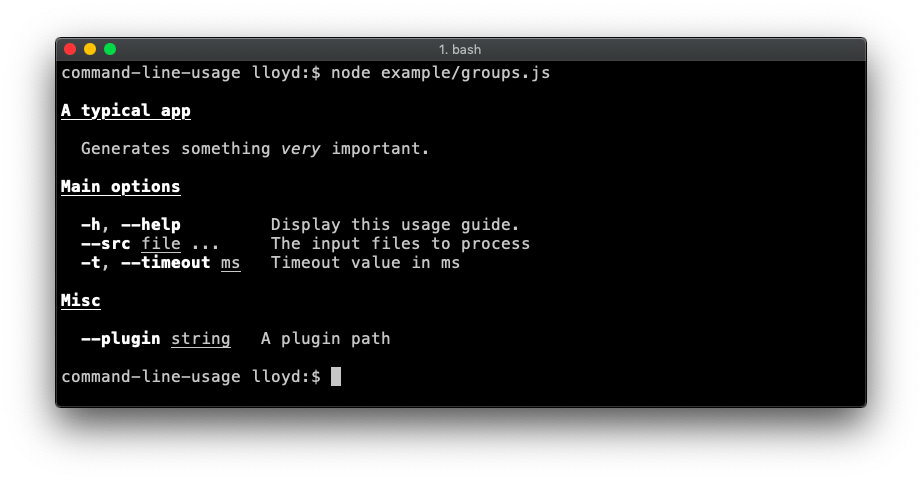 | ||
### Header | ||
Here, the `title` is replaced with a `header` banner. This example also adds a `synopsis` list. [Code](https://github.com/75lb/command-line-usage/blob/master/example/header.js). | ||
### Banners | ||
A banner is created by adding the `raw: true` property to your `content`. This flag disables any formatting on the content, displaying it raw as supplied. | ||
#### Header | ||
Demonstrates a banner at the top. This example also adds a `synopsis` section. [Code](https://github.com/75lb/command-line-usage/blob/master/example/header.js). | ||
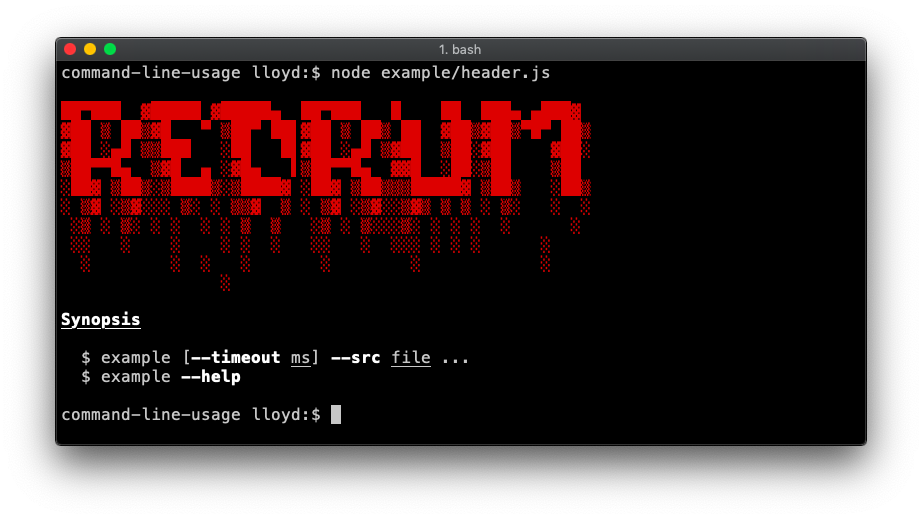 | ||
### Footer | ||
The footer is displayed at the end of the template. [Code](https://github.com/75lb/command-line-usage/blob/master/example/footer.js). | ||
#### Footer | ||
Demonstrates a footer banner. [Code](https://github.com/75lb/command-line-usage/blob/master/example/footer.js). | ||
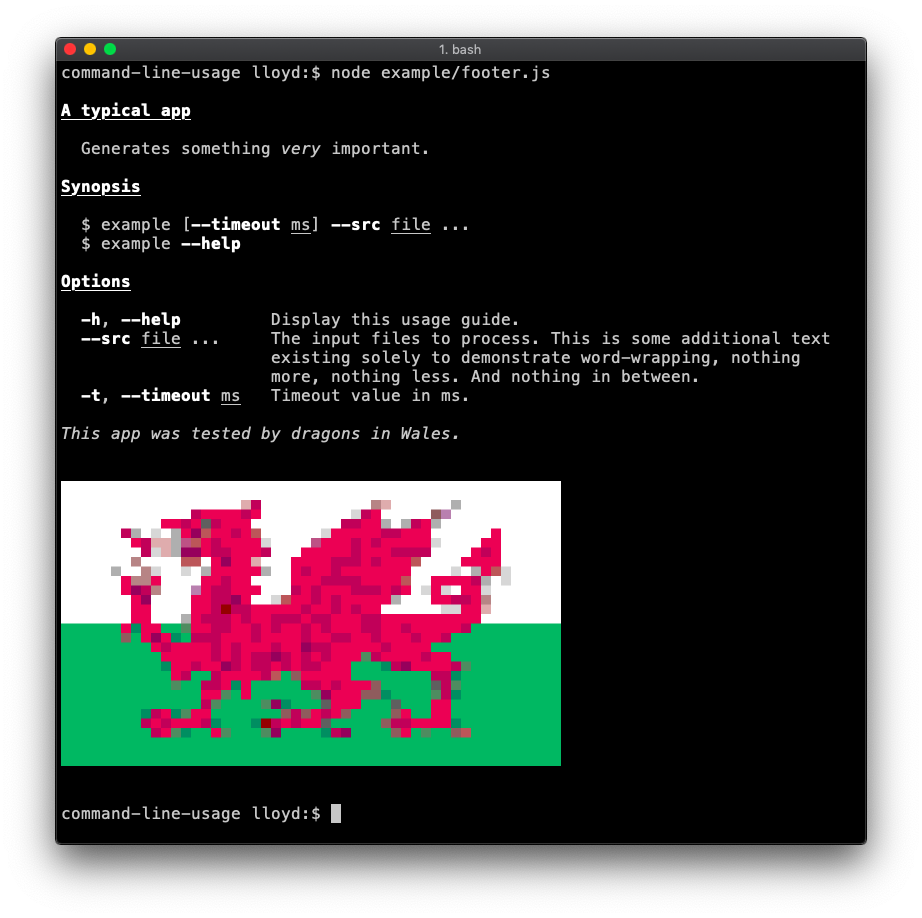 | ||
### Examples (column layout) | ||
A list of `examples` is added. In this case the example list is defined as an array of objects (each with consistently named properties) so will be formatted by [table-layout](https://github.com/75lb/table-layout). [Code](https://github.com/75lb/command-line-usage/blob/master/example/examples.js). | ||
### Examples section (table layout) | ||
An examples section is added. To achieve this table layout, supply the `content` as an array of objects. The property names of each object are not important, so long as they are consistent throughout the array. [Code](https://github.com/75lb/command-line-usage/blob/master/example/examples.js). | ||
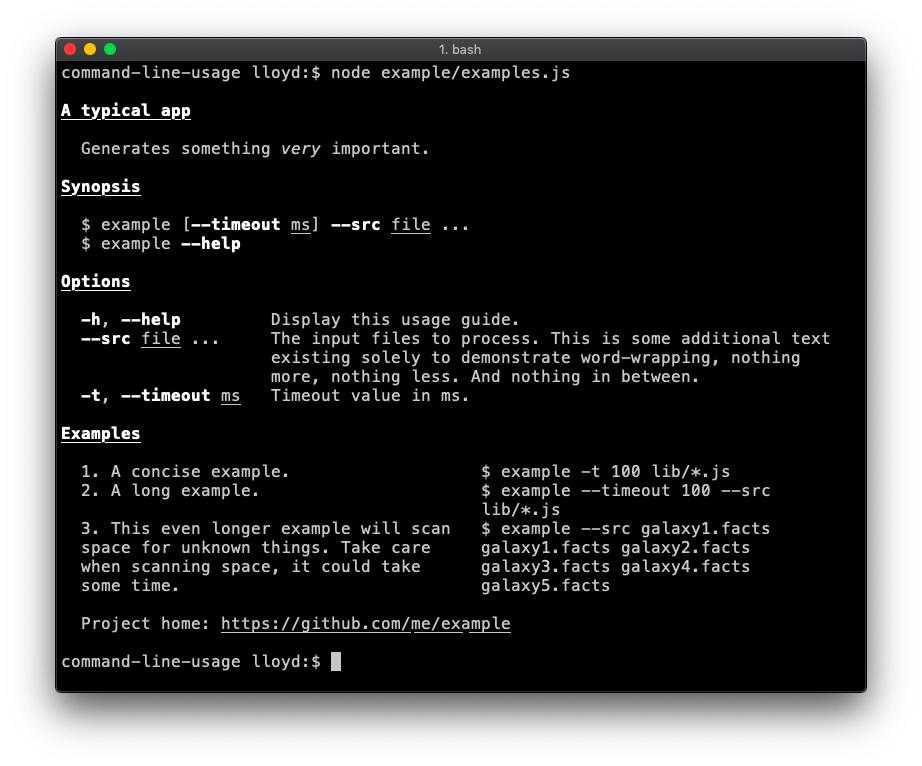 | ||
### Description (column layout) | ||
Demonstrates usage of custom column layout in the description. In this case the second column (containing the hammer and sickle) has `nowrap` enabled, as the input is already formatted as desired. [Code](https://github.com/75lb/command-line-usage/blob/master/example/description-columns.js). | ||
### Command list | ||
Useful if your app is [command-driven](https://github.com/75lb/command-line-commands), like git or npm. [Code](https://github.com/75lb/command-line-usage/blob/master/example/command-list.js). | ||
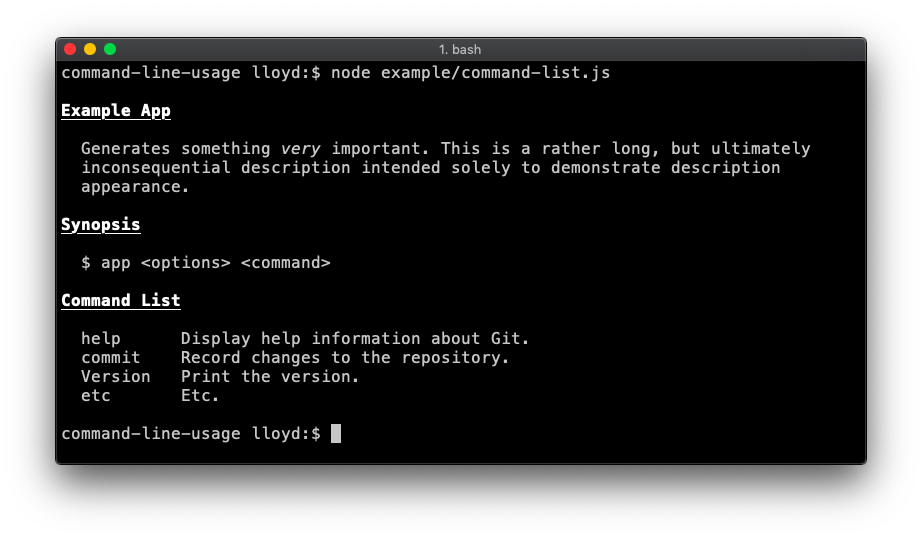 | ||
### Description section (table layout) | ||
Demonstrates use of table layout in the description. In this case the second column (containing the hammer and sickle) has `nowrap` enabled, as the input is already formatted as desired. [Code](https://github.com/75lb/command-line-usage/blob/master/example/description-columns.js). | ||
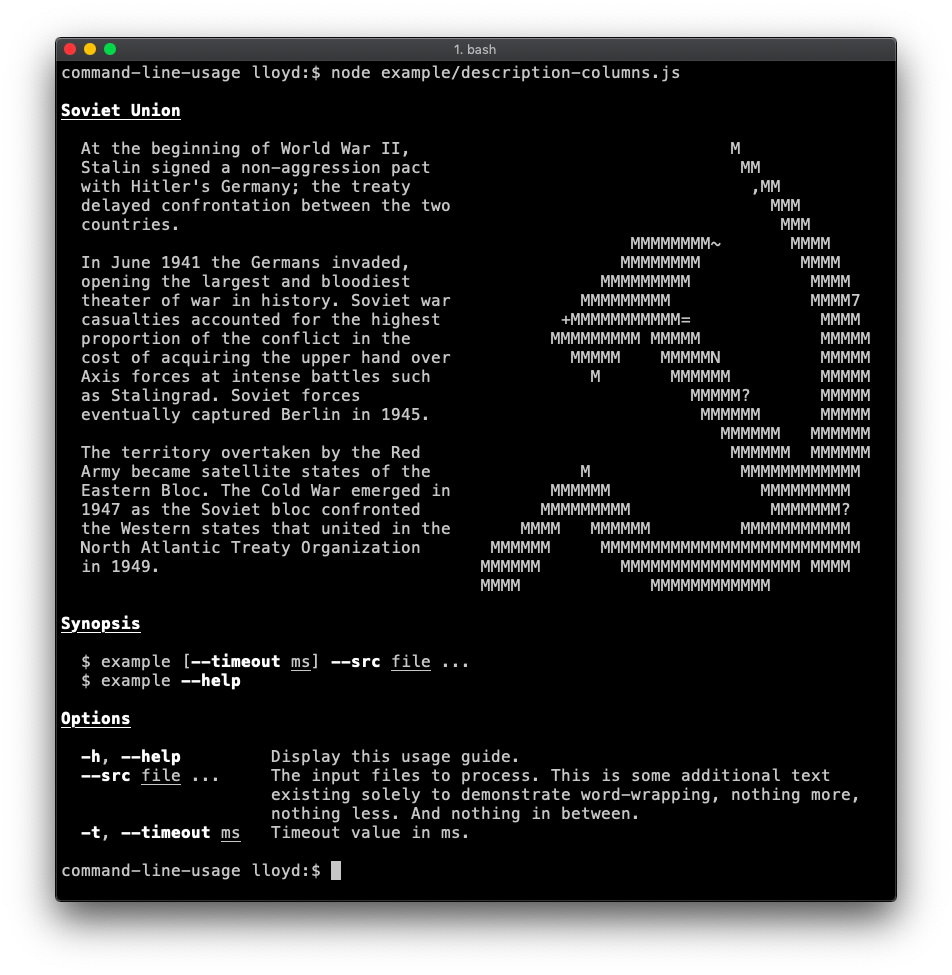 | ||
### Custom | ||
Demonstrates a custom template. The `getUsage.optionList()` method exists for users that want the option list and nothing else. [Code](https://github.com/75lb/command-line-usage/blob/master/example/custom.js). | ||
## API Reference | ||
 | ||
# API Reference | ||
* [command-line-usage](#module_command-line-usage) | ||
* [getUsage(sections, options)](#exp_module_command-line-usage--getUsage) ⇒ <code>string</code> ⏏ | ||
* [~optionList(definitions, [group])](#module_command-line-usage--getUsage..optionList) ⇒ <code>Array.<string></code> | ||
* [commandLineUsage(sections)](#exp_module_command-line-usage--commandLineUsage) ⇒ <code>string</code> ⏏ | ||
* [~content](#module_command-line-usage--commandLineUsage..content) | ||
* [~optionList](#module_command-line-usage--commandLineUsage..optionList) | ||
<a name="exp_module_command-line-usage--getUsage"></a> | ||
<a name="exp_module_command-line-usage--commandLineUsage"></a> | ||
### getUsage(sections, options) ⇒ <code>string</code> ⏏ | ||
### commandLineUsage(sections) ⇒ <code>string</code> ⏏ | ||
Generates a usage guide suitable for a command-line app. | ||
**Kind**: Exported function | ||
@@ -73,24 +110,19 @@ <table> | ||
<tr> | ||
<td>sections</td><td><code>Array.<optionDefinition></code></td><td><p>an array of <a href="https://github.com/75lb/command-line-args#exp_module_definition--OptionDefinition">option definition</a> objects. In addition to the regular definition properties, command-line-usage will look for:</p> | ||
<ul> | ||
<li><code>description</code> - a string describing the option.</li> | ||
<li><code>typeLabel</code> - a string to replace the default type string (e.g. <code><string></code>). It's often more useful to set a more descriptive type label, like <code><ms></code>, <code><files></code>, <code><command></code> etc.</li> | ||
</ul> | ||
<td>sections</td><td><code>Section</code> | <code>Array.<Section></code></td><td><p>One of more section objects (<a href="#module_command-line-usage--commandLineUsage..content">content</a> or <a href="#module_command-line-usage--commandLineUsage..optionList">optionList</a>).</p> | ||
</td> | ||
</tr><tr> | ||
<td>options</td><td><code><a href="#module_usage-options">usage-options</a></code></td><td><p>see <a href="#exp_module_usage-options--UsageOptions">UsageOptions</a>.</p> | ||
</td> | ||
</tr> </tbody> | ||
</table> | ||
<a name="module_command-line-usage--getUsage..optionList"></a> | ||
<a name="module_command-line-usage--commandLineUsage..content"></a> | ||
#### getUsage~optionList(definitions, [group]) ⇒ <code>Array.<string></code> | ||
A helper for getting a column-format list of options and descriptions. Useful for inserting into a custom usage template. | ||
#### commandLineUsage~content | ||
A Content section comprises a header and one or more lines of content. | ||
**Kind**: inner method of <code>[getUsage](#exp_module_command-line-usage--getUsage)</code> | ||
**Kind**: inner typedef of <code>[commandLineUsage](#exp_module_command-line-usage--commandLineUsage)</code> | ||
**Properties** | ||
<table> | ||
<thead> | ||
<tr> | ||
<th>Param</th><th>Type</th><th>Description</th> | ||
<th>Name</th><th>Type</th><th>Description</th> | ||
</tr> | ||
@@ -100,154 +132,99 @@ </thead> | ||
<tr> | ||
<td>definitions</td><td><code>Array.<optionDefinition></code></td><td><p>the definitions to Display</p> | ||
<td>header</td><td><code>string</code></td><td><p>The section header, always bold and underlined.</p> | ||
</td> | ||
</tr><tr> | ||
<td>[group]</td><td><code>string</code></td><td><p>if specified, will output the options in this group. The special group <code>'_none'</code> will return options without a group specified.</p> | ||
<td>content</td><td><code>string</code> | <code>Array.<string></code> | <code>Array.<object></code></td><td><p>One or more lines of text. For table layout, supply the content as an array of objects. The property names of each object are not important, so long as they are consistent throughout the array.</p> | ||
</td> | ||
</tr><tr> | ||
<td>raw</td><td><code>boolean</code></td><td><p>Set to true to avoid indentation and wrapping. Useful for banners.</p> | ||
</td> | ||
</tr> </tbody> | ||
</table> | ||
<a name="exp_module_usage-options--UsageOptions"></a> | ||
## UsageOptions ⏏ | ||
The class describes all valid options for the `getUsage` function. Inline formatting can be used within any text string supplied using valid [ansi-escape-sequences formatting syntax](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
**Kind**: Exported class | ||
* [UsageOptions](#exp_module_usage-options--UsageOptions) ⏏ | ||
* _instance_ | ||
* [.header](#module_usage-options--UsageOptions+header) : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
* [.title](#module_usage-options--UsageOptions+title) : <code>string</code> | ||
* [.description](#module_usage-options--UsageOptions+description) : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
* [.synopsis](#module_usage-options--UsageOptions+synopsis) : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
* [.groups](#module_usage-options--UsageOptions+groups) : <code>object</code> | ||
* [.examples](#module_usage-options--UsageOptions+examples) : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
* [.footer](#module_usage-options--UsageOptions+footer) : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
* [.hide](#module_usage-options--UsageOptions+hide) : <code>string</code> | <code>Array.<string></code> | ||
* _inner_ | ||
* [~textBlock](#module_usage-options--UsageOptions..textBlock) : <code>string</code> | <code>Array.<string></code> | <code>Array.<object></code> | <code>Object</code> | ||
<a name="module_usage-options--UsageOptions+header"></a> | ||
### options.header : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
Use this field to display a banner or header above the main body. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
<a name="module_usage-options--UsageOptions+title"></a> | ||
### options.title : <code>string</code> | ||
The title line at the top of the usage, typically the name of the app. By default it is underlined but this formatting can be overridden by passing a [module:usage-options~textObject](module:usage-options~textObject). | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
**Example** | ||
Simple string of content. The syntax for ansi formatting is documented [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
```js | ||
{ title: "my-app" } | ||
``` | ||
<a name="module_usage-options--UsageOptions+description"></a> | ||
### options.description : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
A description to go underneath the title. For example, some words about what the app is for. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
<a name="module_usage-options--UsageOptions+synopsis"></a> | ||
### options.synopsis : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
An array of strings highlighting the main usage forms of the app. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
<a name="module_usage-options--UsageOptions+groups"></a> | ||
### options.groups : <code>object</code> | ||
Specify which groups to display in the output by supplying an object of key/value pairs, where the key is the name of the group to include and the value is a string or textObject. If the value is a string it is used as the group title. Alternatively supply an object containing a `title` and `description` string. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
**Example** | ||
```js | ||
{ | ||
main: { | ||
title: "Main options", | ||
description: "This group contains the most important options." | ||
}, | ||
misc: "Miscellaneous" | ||
header: 'A typical app', | ||
content: 'Generates something [italic]{very} important.' | ||
} | ||
``` | ||
<a name="module_usage-options--UsageOptions+examples"></a> | ||
### options.examples : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
Examples | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
<a name="module_usage-options--UsageOptions+footer"></a> | ||
### options.footer : <code>[textBlock](#module_usage-options--UsageOptions..textBlock)</code> | ||
Displayed at the foot of the usage output. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
**Example** | ||
An array of strings is interpreted as lines, to be joined by the system newline character. | ||
```js | ||
{ | ||
footer: "Project home: [underline]{https://github.com/me/my-app}" | ||
header: 'A typical app', | ||
content: [ | ||
'First line.', | ||
'Second line.' | ||
] | ||
} | ||
``` | ||
<a name="module_usage-options--UsageOptions+hide"></a> | ||
### options.hide : <code>string</code> | <code>Array.<string></code> | ||
If you want to hide certain options from the output, specify their names here. This is sometimes used to hide deprecated options or the `defaultOption`. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
**Example** | ||
An array of recordset-style objects are rendered in table layout. | ||
```js | ||
{ | ||
hide: "files" | ||
header: 'A typical app', | ||
content: [ | ||
{ colA: 'First row, first column.', colB: 'First row, second column.'}, | ||
{ colA: 'Second row, first column.', colB: 'Second row, second column.'} | ||
] | ||
} | ||
``` | ||
<a name="module_usage-options--UsageOptions..textBlock"></a> | ||
<a name="module_command-line-usage--commandLineUsage..optionList"></a> | ||
### options~textBlock : <code>string</code> | <code>Array.<string></code> | <code>Array.<object></code> | <code>Object</code> | ||
A text block can be a string: | ||
#### commandLineUsage~optionList | ||
A OptionList section adds a table displaying details of the available options. | ||
**Kind**: inner typedef of <code>[commandLineUsage](#exp_module_command-line-usage--commandLineUsage)</code> | ||
**Properties** | ||
<table> | ||
<thead> | ||
<tr> | ||
<th>Name</th><th>Type</th><th>Description</th> | ||
</tr> | ||
</thead> | ||
<tbody> | ||
<tr> | ||
<td>header</td><td><code>string</code></td><td><p>The section header, always bold and underlined.</p> | ||
</td> | ||
</tr><tr> | ||
<td>optionList</td><td><code>Array.<OptionDefinition></code></td><td><p>an array of <a href="https://github.com/75lb/command-line-args#optiondefinition-">option definition</a> objects. In addition to the regular definition properties, command-line-usage will look for:</p> | ||
<ul> | ||
<li><code>description</code> - a string describing the option.</li> | ||
<li><code>typeLabel</code> - a string to replace the default type string (e.g. <code><string></code>). It's often more useful to set a more descriptive type label, like <code><ms></code>, <code><files></code>, <code><command></code> etc.</li> | ||
</ul> | ||
</td> | ||
</tr><tr> | ||
<td>group</td><td><code>string</code> | <code>Array.<string></code></td><td><p>If specified, only options from this particular group will be printed. <a href="https://github.com/75lb/command-line-usage/blob/master/example/groups.js">Example</a>.</p> | ||
</td> | ||
</tr><tr> | ||
<td>hide</td><td><code>string</code> | <code>Array.<string></code></td><td><p>The names of one of more option definitions to hide from the option list. <a href="https://github.com/75lb/command-line-usage/blob/master/example/hide.js">Example</a>.</p> | ||
</td> | ||
</tr> </tbody> | ||
</table> | ||
**Example** | ||
```js | ||
{ | ||
description: 'This is a single-line description.' | ||
} | ||
``` | ||
.. or multiple strings: | ||
```js | ||
{ | ||
description: [ | ||
'This is a multi-line description.', | ||
'A new string in the array represents a new line.' | ||
] | ||
} | ||
``` | ||
.. or an array of objects. In which case, it will be formatted by [table-layout](https://github.com/75lb/table-layout): | ||
```js | ||
{ | ||
description: { | ||
column1: 'This will go in column 1.', | ||
column2: 'Second column text.' | ||
} | ||
} | ||
``` | ||
If you want set specific table-layout options, pass an object with two properties: `options` and `data`. | ||
```js | ||
{ | ||
description: { | ||
options: { | ||
columns: [ | ||
{ name: 'two', width: 40, nowrap: true } | ||
] | ||
header: 'Options', | ||
optionList: [ | ||
{ | ||
name: 'help', alias: 'h', description: 'Display this usage guide.' | ||
}, | ||
data: { | ||
column1: 'This will go in column 1.', | ||
column2: 'Second column text.' | ||
{ | ||
name: 'src', description: 'The input files to process', | ||
multiple: true, defaultOption: true, typeLabel: '[underline]{file} ...' | ||
}, | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms. This description is needlessly long unless you count testing of the description column maxWidth useful.', | ||
alias: 't', typeLabel: '[underline]{ms}' | ||
} | ||
} | ||
] | ||
} | ||
``` | ||
**Kind**: inner typedef of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
* * * | ||
© 2015-16 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/75lb/jsdoc-to-markdown). |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
855037
95.15%39
25.81%820
5.53%228
-9.16%1
Infinity%Updated