command-line-usage
Advanced tools
Comparing version
'use strict'; | ||
var OptionList = require('./option-list'); | ||
var Content = require('./content'); | ||
var ContentSection = require('./content-section'); | ||
var arrayify = require('array-back'); | ||
@@ -16,3 +16,3 @@ | ||
} else { | ||
return new Content(section); | ||
return new ContentSection(section); | ||
} | ||
@@ -19,0 +19,0 @@ }); |
'use strict'; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
var tableLayout = require('table-layout'); | ||
var Table = require('table-layout'); | ||
var ansi = require('ansi-escape-sequences'); | ||
var t = require('typical'); | ||
var Section = require('./section'); | ||
var Content = function (_Section) { | ||
_inherits(Content, _Section); | ||
function Content(section) { | ||
var Content = function () { | ||
function Content(content) { | ||
_classCallCheck(this, Content); | ||
var _this = _possibleConstructorReturn(this, (Content.__proto__ || Object.getPrototypeOf(Content)).call(this)); | ||
this._content = content; | ||
} | ||
var defaultPadding = { left: ' ', right: ' ' }; | ||
var content = section.content; | ||
var raw = section.raw; | ||
_this.header(section.header); | ||
_createClass(Content, [{ | ||
key: 'lines', | ||
value: function lines() { | ||
var content = this._content; | ||
var defaultPadding = { left: ' ', right: ' ' }; | ||
if (content) { | ||
if (raw) { | ||
_this.add(content); | ||
} else if (t.isString(content)) { | ||
_this.add(tableLayout.lines({ column: ansi.format(content) }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})); | ||
} else if (Array.isArray(content) && content.every(t.isString)) { | ||
var rows = content.map(function (string) { | ||
return { column: ansi.format(string) }; | ||
}); | ||
_this.add(tableLayout.lines(rows, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})); | ||
_this.add(); | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
_this.add(tableLayout.lines(content, { | ||
padding: defaultPadding | ||
})); | ||
} else if (t.isPlainObject(content)) { | ||
if (!content.options || !content.data) { | ||
throw new Error('must have an "options" or "data" property\n' + JSON.stringify(content)); | ||
if (content) { | ||
if (t.isString(content)) { | ||
var table = new Table({ column: ansi.format(content) }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
}); | ||
return table.renderLines(); | ||
} else if (Array.isArray(content) && content.every(t.isString)) { | ||
var rows = content.map(function (string) { | ||
return { column: ansi.format(string) }; | ||
}); | ||
var _table = new Table(rows, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
}); | ||
return _table.renderLines(); | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
var _table2 = new Table(content.map(function (row) { | ||
return ansiFormatRow(row); | ||
}), { | ||
padding: defaultPadding | ||
}); | ||
return _table2.renderLines(); | ||
} else if (t.isPlainObject(content)) { | ||
if (!content.options || !content.data) { | ||
throw new Error('must have an "options" or "data" property\n' + JSON.stringify(content)); | ||
} | ||
var options = Object.assign({ padding: defaultPadding }, content.options); | ||
if (options.columns) { | ||
options.columns = options.columns.map(function (column) { | ||
if (column.nowrap) { | ||
column.noWrap = column.nowrap; | ||
delete column.nowrap; | ||
} | ||
return column; | ||
}); | ||
} | ||
var _table3 = new Table(content.data.map(function (row) { | ||
return ansiFormatRow(row); | ||
}), options); | ||
return _table3.renderLines(); | ||
} else { | ||
var message = 'invalid input - \'content\' must be a string, array of strings, or array of plain objects:\n\n' + JSON.stringify(content); | ||
throw new Error(message); | ||
} | ||
var options = Object.assign({ padding: defaultPadding }, content.options); | ||
_this.add(tableLayout.lines(content.data.map(function (row) { | ||
return ansiFormatRow(row); | ||
}), options)); | ||
} else { | ||
var message = 'invalid input - \'content\' must be a string, array of strings, or array of plain objects:\n\n' + JSON.stringify(content); | ||
throw new Error(message); | ||
} | ||
_this.emptyLine(); | ||
} | ||
return _this; | ||
} | ||
}]); | ||
return Content; | ||
}(Section); | ||
}(); | ||
@@ -69,0 +78,0 @@ function ansiFormatRow(row) { |
@@ -10,3 +10,3 @@ 'use strict'; | ||
var Section = require('./section'); | ||
var tableLayout = require('table-layout'); | ||
var Table = require('table-layout'); | ||
var ansi = require('ansi-escape-sequences'); | ||
@@ -51,6 +51,7 @@ var t = require('typical'); | ||
_this.add(tableLayout.lines(columns, { | ||
var table = new Table(columns, { | ||
padding: { left: ' ', right: ' ' }, | ||
columns: [{ name: 'option', nowrap: true }, { name: 'description', maxWidth: 80 }] | ||
})); | ||
columns: [{ name: 'option', noWrap: true }, { name: 'description', maxWidth: 80 }] | ||
}); | ||
_this.add(table.renderLines()); | ||
@@ -57,0 +58,0 @@ _this.emptyLine(); |
'use strict' | ||
const OptionList = require('./option-list') | ||
const Content = require('./content') | ||
const ContentSection = require('./content-section') | ||
const arrayify = require('array-back') | ||
@@ -24,3 +24,3 @@ | ||
} else { | ||
return new Content(section) | ||
return new ContentSection(section) | ||
} | ||
@@ -36,3 +36,9 @@ }) | ||
* @property header {string} - The section header, always bold and underlined. | ||
* @property content {string|string[]|object[]} - One or more lines of text. For table layout, supply the content as an array of objects. The property names of each object are not important, so long as they are consistent throughout the array. | ||
* @property content {string|string[]|object[]} - Overloaded property, accepting data in one of four formats: | ||
* | ||
* 1. A single string (one line of text) | ||
* 2. An array of strings (multiple lines of text) | ||
* 3. An array of objects (recordset-style data). In this case, the data will be rendered in table format. The property names of each object are not important, so long as they are consistent throughout the array. | ||
* 4. An object with two properties - `data` and `options`. In this case, the data and options will be passed directly to the underlying [table layout](https://github.com/75lb/table-layout) module for rendering. | ||
* | ||
* @property raw {boolean} - Set to true to avoid indentation and wrapping. Useful for banners. | ||
@@ -69,2 +75,18 @@ * @example | ||
* ``` | ||
* | ||
* An object with `data` and `options` properties will be passed directly to the underlying [table layout](https://github.com/75lb/table-layout) module for rendering. | ||
* ```js | ||
* { | ||
* header: 'A typical app', | ||
* content: { | ||
* data: [ | ||
* { colA: 'First row, first column.', colB: 'First row, second column.'}, | ||
* { colA: 'Second row, first column.', colB: 'Second row, second column.'} | ||
* ], | ||
* options: { | ||
* maxWidth: 60 | ||
* } | ||
* } | ||
* } | ||
* ``` | ||
*/ | ||
@@ -71,0 +93,0 @@ |
'use strict' | ||
const tableLayout = require('table-layout') | ||
const Table = require('table-layout') | ||
const ansi = require('ansi-escape-sequences') | ||
const t = require('typical') | ||
const Section = require('./section') | ||
class Content extends Section { | ||
constructor (section) { | ||
super() | ||
class Content { | ||
constructor (content) { | ||
this._content = content | ||
} | ||
lines () { | ||
const content = this._content | ||
const defaultPadding = { left: ' ', right: ' ' } | ||
const content = section.content | ||
const raw = section.raw | ||
this.header(section.header) | ||
if (content) { | ||
/* add content without indentation or wrapping */ | ||
if (raw) { | ||
this.add(content) | ||
/* string content */ | ||
} else if (t.isString(content)) { | ||
this.add(tableLayout.lines({ column: ansi.format(content) }, { | ||
if (t.isString(content)) { | ||
const table = new Table({ column: ansi.format(content) }, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})) | ||
}) | ||
return table.renderLines() | ||
@@ -30,13 +27,14 @@ /* array of strings */ | ||
const rows = content.map(string => ({ column: ansi.format(string) })) | ||
this.add(tableLayout.lines(rows, { | ||
const table = new Table(rows, { | ||
padding: defaultPadding, | ||
maxWidth: 80 | ||
})) | ||
this.add() | ||
}) | ||
return table.renderLines() | ||
/* array of objects (use table-layout) */ | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
this.add(tableLayout.lines(content, { | ||
const table = new Table(content.map(row => ansiFormatRow(row)), { | ||
padding: defaultPadding | ||
})) | ||
}) | ||
return table.renderLines() | ||
@@ -52,6 +50,19 @@ /* { options: object, data: object[] } */ | ||
) | ||
this.add(tableLayout.lines( | ||
/* convert nowrap to noWrap to avoid breaking compatibility */ | ||
if (options.columns) { | ||
options.columns = options.columns.map(column => { | ||
if (column.nowrap) { | ||
column.noWrap = column.nowrap | ||
delete column.nowrap | ||
} | ||
return column | ||
}) | ||
} | ||
const table = new Table( | ||
content.data.map(row => ansiFormatRow(row)), | ||
options | ||
)) | ||
) | ||
return table.renderLines() | ||
} else { | ||
@@ -61,4 +72,2 @@ const message = `invalid input - 'content' must be a string, array of strings, or array of plain objects:\n\n${JSON.stringify(content)}` | ||
} | ||
this.emptyLine() | ||
} | ||
@@ -65,0 +74,0 @@ } |
'use strict' | ||
const Section = require('./section') | ||
const tableLayout = require('table-layout') | ||
const Table = require('table-layout') | ||
const ansi = require('ansi-escape-sequences') | ||
@@ -39,9 +39,7 @@ const t = require('typical') | ||
this.add(tableLayout.lines(columns, { | ||
const table = new Table(columns, { | ||
padding: { left: ' ', right: ' ' }, | ||
columns: [ | ||
{ name: 'option', nowrap: true }, | ||
{ name: 'description', maxWidth: 80 } | ||
] | ||
})) | ||
columns: [{ name: 'option', noWrap: true }, { name: 'description', maxWidth: 80 }] | ||
}) | ||
this.add(table.renderLines()) | ||
@@ -48,0 +46,0 @@ this.emptyLine() |
{ | ||
"name": "command-line-usage", | ||
"author": "Lloyd Brookes <75pound@gmail.com>", | ||
"version": "3.0.7", | ||
"version": "3.0.8", | ||
"description": "Generates command-line usage information", | ||
@@ -21,3 +21,3 @@ "repository": "https://github.com/75lb/command-line-usage.git", | ||
"engines": { | ||
"node": ">=0.10.0" | ||
"node": ">=0.12.0" | ||
}, | ||
@@ -32,3 +32,3 @@ "scripts": { | ||
"array-back": "^1.0.3", | ||
"table-layout": "~0.2.3", | ||
"table-layout": "^0.3.0", | ||
"feature-detect-es6": "^1.3.1", | ||
@@ -41,3 +41,3 @@ "typical": "^2.6.0" | ||
"jsdoc-to-markdown": "^2.0.1", | ||
"test-runner": "^0.2.5" | ||
"test-runner": "^0.3.0" | ||
}, | ||
@@ -44,0 +44,0 @@ "standard": { |
@@ -8,12 +8,12 @@ [](https://www.npmjs.org/package/command-line-usage) | ||
# command-line-usage | ||
A simple module for creating a usage guide. | ||
A simple, data-driven module for creating a usage guide. | ||
## Synopis | ||
A usage guide is built from an arbitrary number of sections, e.g. a description section, synopsis, option list, examples, footer etc. Each section has a bold, underlined header and some content (a paragraph, table, option list, banner etc.) | ||
A usage guide is created by first defining an arbitrary number of sections, e.g. a description section, synopsis, option list, examples, footer etc. Each section has an optional header and some content. Each section must be of type <code><a href="#commandlineusagecontent">content</a></code> or <code><a href="#commandlineusageoptionlist">optionList</a></code>. | ||
The <code><a href="#commandlineusagesections--string-">commandLineUsage()</a></code> function takes one or more `section` objects (<code><a href="#commandlineusagecontent">content</a></code> or <code><a href="#commandlineusageoptionlist">optionList</a></code>) as input. | ||
This section data is passed to <code><a href="#commandlineusagesections--string-">commandLineUsage()</a></code> which renders the usage guide. | ||
Inline ansi formatting can be used anywhere within section content using the formatting syntax described [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
This script: | ||
For example, this script: | ||
```js | ||
@@ -50,3 +50,3 @@ const getUsage = require('command-line-usage') | ||
## Examples | ||
## More examples | ||
@@ -87,6 +87,11 @@ ### Simple | ||
### Description section (table layout) | ||
Demonstrates use of table layout in the description. In this case the second column (containing the hammer and sickle) has `nowrap` enabled, as the input is already formatted as desired. [Code](https://github.com/75lb/command-line-usage/blob/master/example/description-columns.js). | ||
Demonstrates supplying specific [table layout](https://github.com/75lb/table-layout) options to achieve more advanced layout. In this case the second column (containing the hammer and sickle) has a fixed `width` of 40 and `noWrap` enabled (as the input is already formatted as desired). [Code](https://github.com/75lb/command-line-usage/blob/master/example/description-columns.js). | ||
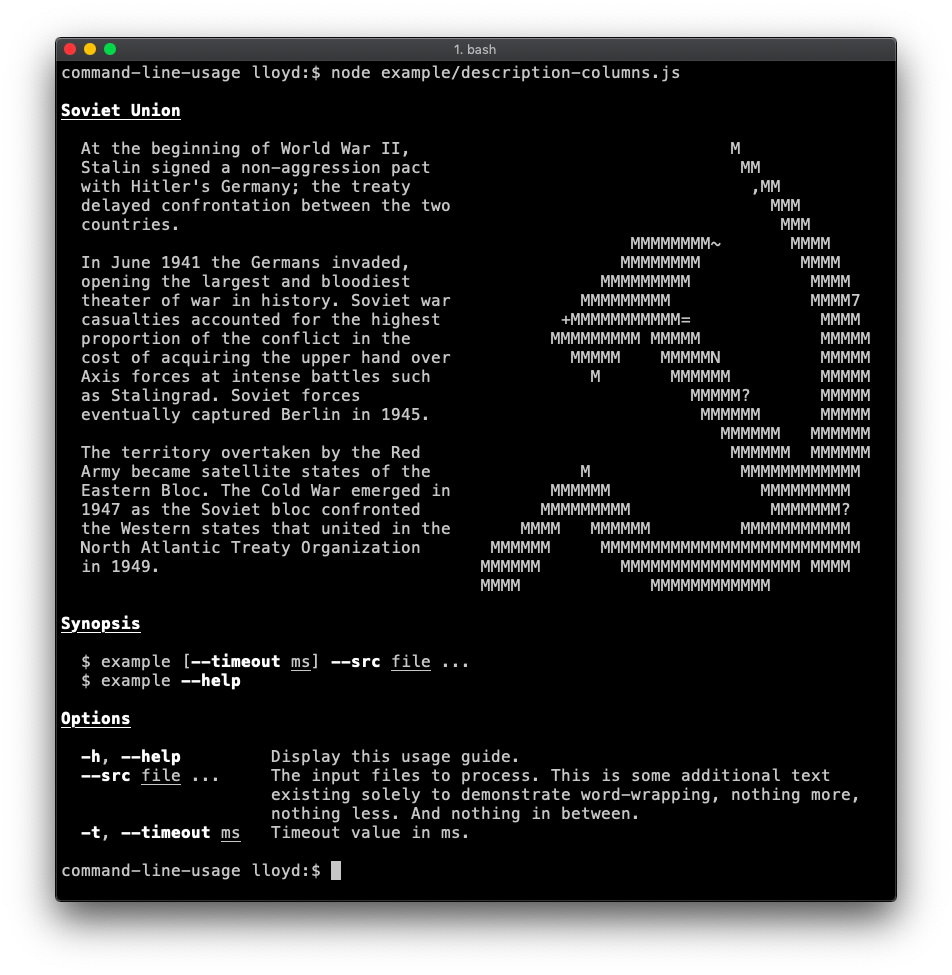 | ||
### Whitespace | ||
By default, whitespace from the beginning of each line is trimmed to ensure wrapped text always aligns neatly to the left edge of the column. This can be undesirable when whitespace is intentional like the indented bullet points shown in this example. The two ways to disable whitespace trimming are shown in [this example code](https://github.com/75lb/command-line-usage/blob/master/example/whitespace.js). | ||
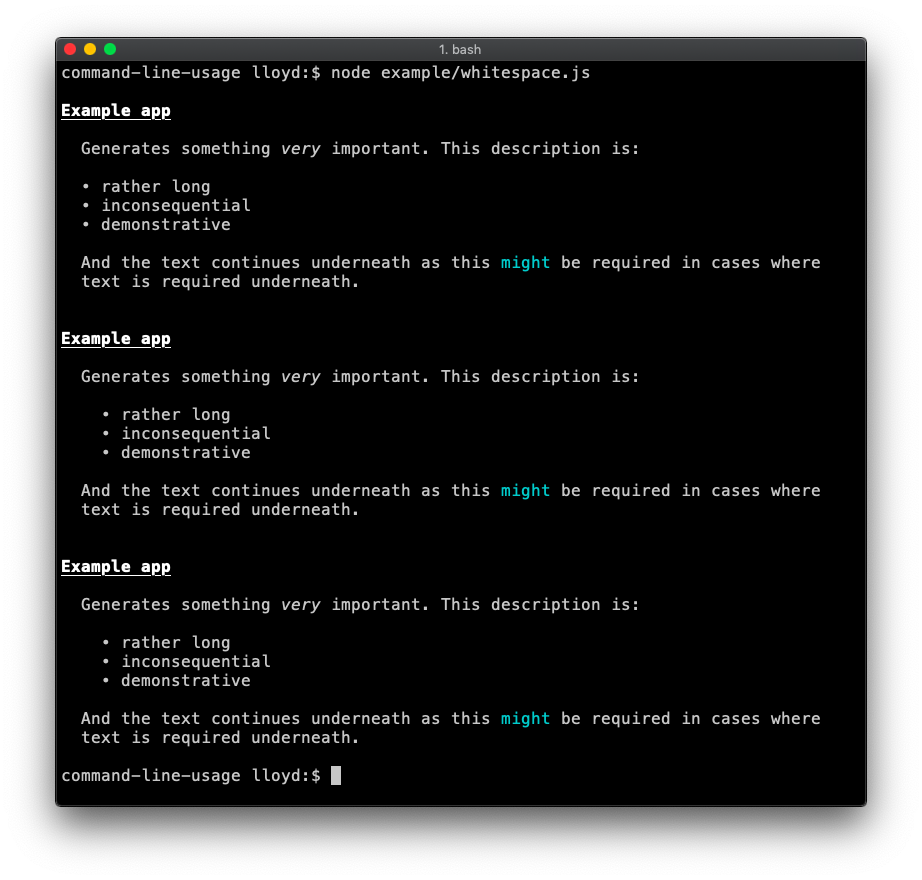 | ||
## API Reference | ||
@@ -138,3 +143,9 @@ | ||
</tr><tr> | ||
<td>content</td><td><code>string</code> | <code>Array.<string></code> | <code>Array.<object></code></td><td><p>One or more lines of text. For table layout, supply the content as an array of objects. The property names of each object are not important, so long as they are consistent throughout the array.</p> | ||
<td>content</td><td><code>string</code> | <code>Array.<string></code> | <code>Array.<object></code></td><td><p>Overloaded property, accepting data in one of four formats:</p> | ||
<ol> | ||
<li>A single string (one line of text)</li> | ||
<li>An array of strings (multiple lines of text)</li> | ||
<li>An array of objects (recordset-style data). In this case, the data will be rendered in table format. The property names of each object are not important, so long as they are consistent throughout the array.</li> | ||
<li>An object with two properties - <code>data</code> and <code>options</code>. In this case, the data and options will be passed directly to the underlying <a href="https://github.com/75lb/table-layout">table layout</a> module for rendering.</li> | ||
</ol> | ||
</td> | ||
@@ -177,2 +188,18 @@ </tr><tr> | ||
``` | ||
An object with `data` and `options` properties will be passed directly to the underlying [table layout](https://github.com/75lb/table-layout) module for rendering. | ||
```js | ||
{ | ||
header: 'A typical app', | ||
content: { | ||
data: [ | ||
{ colA: 'First row, first column.', colB: 'First row, second column.'}, | ||
{ colA: 'Second row, first column.', colB: 'Second row, second column.'} | ||
], | ||
options: { | ||
maxWidth: 60 | ||
} | ||
} | ||
} | ||
``` | ||
<a name="module_command-line-usage--commandLineUsage..optionList"></a> | ||
@@ -179,0 +206,0 @@ |
34020
17.28%14
16.67%526
19.27%257
11.74%+ Added
+ Added
+ Added
- Removed
- Removed
- Removed
- Removed
- Removed
- Removed
- Removed
- Removed
- Removed
- Removed
Updated