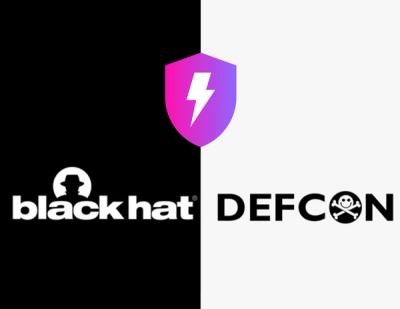
Security News
Meet Socket at BlackHat and DEF CON in Las Vegas
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
command-line-args
Advanced tools
Package description
The command-line-args npm package is a library for parsing command-line arguments in Node.js applications. It allows developers to define options and parse them from the command line, making it easier to handle user inputs and configurations.
Basic Argument Parsing
This feature allows you to define and parse basic command-line arguments. In this example, the 'file' option expects a string value, and the 'verbose' option is a boolean flag.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String },
{ name: 'verbose', type: Boolean }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Default Values
This feature allows you to set default values for options. If the user does not provide a value for 'file' or 'verbose', the defaults 'default.txt' and 'false' will be used, respectively.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String, defaultValue: 'default.txt' },
{ name: 'verbose', type: Boolean, defaultValue: false }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Multiple Values
This feature allows you to handle multiple values for a single option. In this example, the 'files' option can accept multiple string values.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'files', type: String, multiple: true, defaultOption: true }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Aliases
This feature allows you to define aliases for options. In this example, 'help' can be accessed with '-h' and 'version' with '-v'.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'help', alias: 'h', type: Boolean },
{ name: 'version', alias: 'v', type: Boolean }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Yargs is a popular library for parsing command-line arguments in Node.js. It provides a rich set of features, including command handling, argument validation, and automatic help generation. Compared to command-line-args, yargs offers more advanced features and a more user-friendly API.
Commander is another widely-used library for building command-line interfaces in Node.js. It supports option parsing, command definitions, and automatic help generation. Commander is known for its simplicity and ease of use, making it a good alternative to command-line-args for simpler use cases.
Minimist is a lightweight library for parsing command-line arguments. It provides basic functionality for handling options and arguments, but lacks some of the advanced features found in command-line-args, yargs, and commander. Minimist is a good choice for projects that require minimal overhead.
Readme
A library to parse command-line options.
If your app requires a git-like command interface, consider using command-line-commands.
You can set options using the main notation standards (getopt, getopt_long, etc.). These commands are all equivalent, setting the same values:
$ example --verbose --timeout=1000 --src one.js --src two.js
$ example --verbose --timeout 1000 --src one.js two.js
$ example -vt 1000 --src one.js two.js
$ example -vt 1000 one.js two.js
To access the values, first describe the options your app accepts (see option definitions).
const commandLineArgs = require('command-line-args')
const optionDefinitions = [
{ name: 'verbose', alias: 'v', type: Boolean },
{ name: 'src', type: String, multiple: true, defaultOption: true },
{ name: 'timeout', alias: 't', type: Number }
]
The type
property is a setter function (the value supplied is passed through this), giving you full control over the value received.
Next, parse the options using commandLineArgs():
const options = commandLineArgs(optionDefinitions)
options
now looks like this:
{
files: [
'one.js',
'two.js'
],
verbose: true,
timeout: 1000
}
When dealing with large amounts of options it often makes sense to group them.
A usage guide can be generated using command-line-usage, for example:
Notation rules for setting command-line options.
--example
at the beginning or end of the arg list makes no difference.Boolean
do not need to supply a value. Setting --flag
or -f
will set that option's value to true
. This is the only type with special behaviour.--option value
--option=value
-o value
--list one two three
--list one --list two --list three
-a -b -c
-abc
Imagine we are using "grep-tool" to search for the string '-f'
:
$ grep-tool --search -f
We have an issue here: command-line-args will assume we are setting two options (--search
and -f
). In actuality, we are passing one option (--search
) and one value (-f
). In cases like this, avoid ambiguity by using --option=value
notation:
$ grep-tool --search=-f
$ npm install command-line-args --save
$ npm install -g command-line-args
If you install globally you get the command-line-args
test-harness. You test by piping in a module which exports an option definitions array. You can then view the output for the args you pass.
For example:
$ cat example/typical.js | command-line-args lib/* --timeout=1000
{ src:
[ 'lib/command-line-args.js',
'lib/definition.js',
'lib/definitions.js',
'lib/option.js' ],
timeout: 1000 }
object
⏏Returns an object containing all options set on the command line. By default it parses the global process.argv
array.
Kind: Exported function
Throws:
UNKNOWN_OPTION
if the user sets an option without a definitionNAME_MISSING
if an option definition is missing the required name
propertyINVALID_TYPE
if an option definition has a type
value that's not a functionINVALID_ALIAS
if an alias is numeric, a hyphen or a length other than 1DUPLICATE_NAME
if an option definition name was used more than onceDUPLICATE_ALIAS
if an option definition alias was used more than onceDUPLICATE_DEFAULT_OPTION
if more than one option definition has defaultOption: true
Param | Type | Description |
---|---|---|
definitions | Array.<definition> | An array of OptionDefinition objects |
[argv] | Array.<string> | An array of strings, which if passed will be parsed instead of process.argv . |
Example
const commandLineArgs = require('command-line-args')
const options = commandLineArgs([
{ name: 'file' },
{ name: 'verbose' },
{ name: 'depth'}
])
Describes a command-line option. Additionally, you can add description
and typeLabel
propeties and make use of command-line-usage.
Kind: Exported class
string
function
string
boolean
boolean
*
string
| Array.<string>
string
The only required definition property is name
, so the simplest working example is
[
{ name: "file" },
{ name: "verbose" },
{ name: "depth"}
]
In this case, the value of each option will be either a Boolean or string.
# | Command line args | .parse() output |
---|---|---|
1 | --file | { file: true } |
2 | --file lib.js --verbose | { file: "lib.js", verbose: true } |
3 | --verbose very | { verbose: "very" } |
4 | --depth 2 | { depth: "2" } |
Unicode option names and aliases are valid, for example:
[
{ name: 'один' },
{ name: '两' },
{ name: 'три', alias: 'т' }
]
Kind: instance property of OptionDefinition
function
The type
value is a setter function (you receive the output from this), enabling you to be specific about the type and value received.
You can use a class, if you like:
const fs = require('fs')
function FileDetails(filename){
if (!(this instanceof FileDetails)) return new FileDetails(filename)
this.filename = filename
this.exists = fs.existsSync(filename)
}
const cli = commandLineArgs([
{ name: 'file', type: FileDetails },
{ name: 'depth', type: Number }
])
# | Command line args | .parse() output |
---|---|---|
1 | --file asdf.txt | { file: { filename: 'asdf.txt', exists: false } } |
The --depth
option expects a Number
. If no value was set, you will receive null
.
# | Command line args | .parse() output |
---|---|---|
2 | --depth | { depth: null } |
3 | --depth 2 | { depth: 2 } |
Kind: instance property of OptionDefinition
string
getopt-style short option names. Can be any single character (unicode included) except a digit or hypen.
[
{ name: "hot", alias: "h", type: Boolean },
{ name: "discount", alias: "d", type: Boolean },
{ name: "courses", alias: "c" , type: Number }
]
# | Command line | .parse() output |
---|---|---|
1 | -hcd | { hot: true, courses: null, discount: true } |
2 | -hdc 3 | { hot: true, discount: true, courses: 3 } |
Kind: instance property of OptionDefinition
boolean
Set this flag if the option takes a list of values. You will receive an array of values, each passed through the type
function (if specified).
[
{ name: "files", type: String, multiple: true }
]
# | Command line | .parse() output |
---|---|---|
1 | --files one.js two.js | { files: [ 'one.js', 'two.js' ] } |
2 | --files one.js --files two.js | { files: [ 'one.js', 'two.js' ] } |
3 | --files * | { files: [ 'one.js', 'two.js' ] } |
Kind: instance property of OptionDefinition
boolean
Any unclaimed command-line args will be set on this option. This flag is typically set on the most commonly-used option to make for more concise usage (i.e. $ myapp *.js
instead of $ myapp --files *.js
).
[
{ name: "files", type: String, multiple: true, defaultOption: true }
]
# | Command line | .parse() output |
---|---|---|
1 | --files one.js two.js | { files: [ 'one.js', 'two.js' ] } |
2 | one.js two.js | { files: [ 'one.js', 'two.js' ] } |
3 | * | { files: [ 'one.js', 'two.js' ] } |
Kind: instance property of OptionDefinition
*
An initial value for the option.
[
{ name: "files", type: String, multiple: true, defaultValue: [ "one.js" ] },
{ name: "max", type: Number, defaultValue: 3 }
]
# | Command line | .parse() output |
---|---|---|
1 | { files: [ 'one.js' ], max: 3 } | |
2 | --files two.js | { files: [ 'two.js' ], max: 3 } |
3 | --max 4 | { files: [ 'one.js' ], max: 4 } |
Kind: instance property of OptionDefinition
string
| Array.<string>
When your app has a large amount of options it makes sense to organise them in groups.
There are two automatic groups: _all
(contains all options) and _none
(contains options without a group
specified in their definition).
[
{ name: "verbose", group: "standard" },
{ name: "help", group: [ "standard", "main" ] },
{ name: "compress", group: [ "server", "main" ] },
{ name: "static", group: "server" },
{ name: "debug" }
]
# | Command Line | .parse() output |
---|---|---|
1 | --verbose |
|
2 | --debug |
|
3 | --verbose --debug --compress |
|
4 | --compress |
|
Kind: instance property of OptionDefinition
© 2014-16 Lloyd Brookes <75pound@gmail.com>. Documented by jsdoc-to-markdown.
FAQs
A mature, feature-complete library to parse command-line options.
We found that command-line-args demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
Security News
Learn how Socket's 'Non-Existent Author' alert helps safeguard your dependencies by identifying npm packages published by deleted accounts. This is one of the fastest ways to determine if a package may be abandoned.
Security News
In July, the Python Software Foundation mounted a quick response to address a leaked GitHub token, elected new board members, and added more members to the team supporting PSF and PyPI infrastructure.