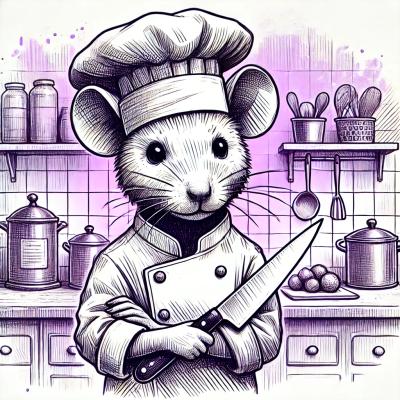
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
common-errors
Advanced tools
Common error classes and utility functions
npm install common-errors
Applicable when a resource is already in use, for example unique key constraints like a username.
new AlreadyInUseError(entityName, arg1, [arg2, arg3, arg4, ...])
Arguments
entityName
- the entity that owns the protected resourceargs
- the fields or attributes that are already in use// Example
throw new errors.AlreadyInUseError('user', 'username');
Applicable when there's a generic problem with an argument received by a function call.
new ArgumentError(argumentName[, inner_error])
Arguments
argumentName
- the name of the argument that has a probleminner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.ArgumentError('username', err);
Applicable when an argument received by a function call is null/undefined or empty.
new ArgumentNullError(argumentName[, inner_error])
Arguments
argumentName
- the name of the argument that is nullinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.ArgumentNullError('username', err);
Applicable when an operation requires authentication
new AuthenticationRequiredError(message, [inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.AuthenticationRequiredError("Please provide authentication.", err)
Applicable when an error occurs on a connection.
new ConnectionError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.ConnectionError('database connection no longer available', err);
Applicable when an error occurs on or with an external data source.
new DataError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.DataError('Too many rows returned from database', err);
Applicable when an error occurs while using memcached.
new MemcachedError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.MemcachedError('Expected value not found', err);
Applicable when an error occurs while using MongoDB.
new MongoDBError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.MongoDBError('Retrieved value not in expected format', err);
Applicable when an error occurs while using redis.
new RedisError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.RedisError('expected value not found in redis', err);
Applicable when a transaction was unexpectedly rolled back.
new RollbackError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.RollbackError('database transaction was unexpectedly rolled back', err);
Applicable when an error occurs while using a SQL database.
new SQLError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.SQLError('foreign key constraint violated', err);
Applicable when an error unexpectedly interrupts a transaction.
new TransactionError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.data.TransactionError('transaction already complete', err);
This is roughly the same as the native Error class. It additionally supports an inner_error attribute.
new Error(message, [inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.Error("Please provide authentication.", err)
Represents a message and a HTTP status code.
new HttpStatusError(status_code[, message])
Arguments
status_code
- any HTTP status code integermessage
- any message// Example
throw new errors.HttpStatusError(404, "Not Found");
new HttpStatusError(err[, req])
Figure out a proper status code and message from a given error. The current mapping of error codes to HTTP status codes is as follows:
{
"ValidationError": 400,
"ArgumentError": 400,
"AuthenticationRequiredError": 401,
"NotPermittedError": 403,
"ArgumentNullError": 404 // , or 400 depending on what's wrong with the request
"NotFoundError": 404,
"NotSupportedError": 405,
"AlreadyInUseError": 409,
}
To change the mappings, modify HttpStatusError.message_map
and HttpStatusError.code_map
Arguments
err
- any instanceof Errorreq
- the request object// Example
throw new errors.HttpStatusError(err, req);
Applicable when an invalid operation occurs.
new InvalidOperationError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.InvalidOperationError('divide by zero', err);
Base class for Errors while accessing information using streams, files and directories.
new IOError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.IOError("Could not open file", err)
Applicable when part of a file or directory cannot be found.
new DirectoryNotFoundError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.DirectoryNotFoundError("/var/log", err)
Applicable when trying to access a drive or share that is not available.
new DriveNotFoundError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.DriveNotFoundError("c", err)
Applicable when reading is attempted past the end of a stream.
new EndOfStreamError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.EndOfStreamError("EOS while reading header", err)
Applicable when a file is found and read but cannot be loaded.
new FileLoadError(message[, inner_error])
Arguments
file_name
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.FileLoadError("./package.json", err)
Applicable when an attempt to access a file that does not exist on disk fails.
new FileNotFoundError(message[, inner_error])
Arguments
file_name
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.io.FileNotFoundError("./package.json", err)
Applicable when an error occurs on a socket.
new SocketError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.SocketError('socket no longer available', err);
Applicable when an attempt to retrieve data yielded no result.
new NotFoundError(entity_name[, inner_error])
Arguments
entity_name
- a description for what was not foundinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.NotFoundError("User", err)
Applicable when a requested method or operation is not implemented.
new NotImplementedError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.NotImplementedError("Method is not yet implemented.", err)
Applicable when an operation is not permitted
new NotPermittedError(message[, inner_error])
Arguments
message
- any messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.NotPermittedError("username cannot be changed once set.", err)
Applicable when a certain condition is not supported by your application.
new NotSupportedError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.NotSupportedError('Zero values', err);
Applicable when there is not enough memory to continue the execution of a program.
new OutOfMemoryError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.OutOfMemoryError('Maximum mem size exceeded.', err);
Represents an error that occurs when a numeric variable or parameter is outside of its valid range. This is roughly the same as the native RangeError class. It additionally supports an inner_error attribute.
new RangeError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.RangeError("Value must be between " + MIN + " and " + MAX, err);
Represents an error when a non-existent variable is referenced. This is roughly the same as the native ReferenceError class. It additionally supports an inner_error attribute.
new ReferenceError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.ReferenceError("x is not defined", err);
Applicable when the execution stack overflows because it contains too many nested method calls.
new StackOverflowError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.StackOverflowError('Stack overflow detected.', err);
Represents an error when trying to interpret syntactically invalid code. This is roughly the same as the native SyntaxError class. It additionally supports an inner_error attribute.
new SyntaxError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.SyntaxError("Unexpected token a", err);
Applicable when an operation takes longer than the alloted amount.
new TimeoutError(time[, inner_error])
Arguments
time
- a time durationinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.TimeoutError('100ms', err);
Represents an error when a value is not of the expected type. This is roughly the same as the native TypeError class. It additionally supports an inner_error attribute.
new TypeError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.TypeError("number is not a function", err);
Represents an error when a value is not of the expected type. This is roughly the same as the native URIError class. It additionally supports an inner_error attribute.
new URIError(message[, inner_error])
Arguments
message
- a messageinner_error
- the Error instance that caused the current error. Stack trace will be appended.// Example
throw new errors.URIError("URI malformed", err);
Useful for denoting a problem with a user-defined value. Generally, you won't throw this error. It serializes to JSON, and it can also function as an envelope for multiple errors.
new ValidationError(message, [code], [field])
Arguments
message
- any messagecode
- an optional error codefield
- an optional description of the dataMethods
addError(error)
- add an error object to the errors
array, and return this
.addErrors(errors)
- append an array of error objects to the errors
array, and return this
.// Example
function validateUsername(username){
var errors = new errors.ValidationError();
if(username.length < 3) errors.addError(new errors.ValidationError("username must be at least two characters long", "VAL_MIN_USERNAME_LENGTH", "username"));
if(/-%$*&!/.test(username)) errors.addError(new errors.ValidationError("username may not contain special characters", "VAL_USERNAME_SPECIALCHARS", "username"));
return errors;
}
Modifies an error's stack to include the current stack and logs it to stderr. Useful for logging errors received by a callback.
log(err[, message])
Arguments
err
- any error or error message received from a callbackmessage
- any message you'd like to prepend// Example
mysql.query('SELECT * `FROM` users', function(err, results){
if(err) return errors.log(err, "Had trouble retrieving users.");
console.log(results);
});
Modifies an error's stack to include the current stack without logging it. Useful for logging errors received by a callback.
prependCurrentStack(err)
Arguments
err
- any error or error message received from a callback// Example
mysql.query('SELECT * `FROM` users', function(err, results){
if(err) {
return errors.prependCurrentStack(err); // caller has better idea of source of err
}
console.log(results);
});
Simple interface for generating a new Error class type.
helpers.generateClass(name[, options])
Arguments
name
- The full name of the new Error classoptions
extends
- The base class for the new Error class. Default is Error
.globalize
- Boolean (default true
) to store the Error in global space so that the Error is equivalent to others included from other versions of the module.args
- Array of names of values to accept and store from the class constructor. Default is ['message', 'inner_error']
.generateMessage
- A function for defining a custom error message.// Example
var ArgumentNullError = helpers.generateClass("ArgumentNullError", {
extends: ArgumentError,
args: ['argumentName'],
generateMessage: function(){
return "Missing argument: " + this.argumentName;
}
});
throw new ArgumentNullError("username");
Express middleware for preventing the web server from crashing when an error is thrown from an asynchronous context.
Any error that would have caused a crash is logged to stderr.
// Example
var app = express();
app.use(express.static(__dirname + '/../public'));
app.use(express.bodyParser());
app.use(errors.middleware.crashProtector());
//insert new middleware here
app.get('/healthcheck', function (req, res, next){res.send('YESOK')});
app.use(app.router);
app.use(errors.middleware.errorHandler);
module.exports = app;
Express middleware that translates common errors into HTTP status codes and messages.
// Example
var app = express();
app.use(express.static(__dirname + '/../public'));
app.use(express.bodyParser());
app.use(errors.middleware.crashProtector());
//insert new middleware here
app.get('/healthcheck', function (req, res, next){res.send('YESOK')});
app.use(app.router);
app.use(errors.middleware.errorHandler);
module.exports = app;
This library was developed by David Fenster at Shutterstock
Please do! Check out our Contributing guidelines.
MIT © 2013-2017 Shutterstock Images, LLC
FAQs
Common error classes and utility functions
The npm package common-errors receives a total of 4,101 weekly downloads. As such, common-errors popularity was classified as popular.
We found that common-errors demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.