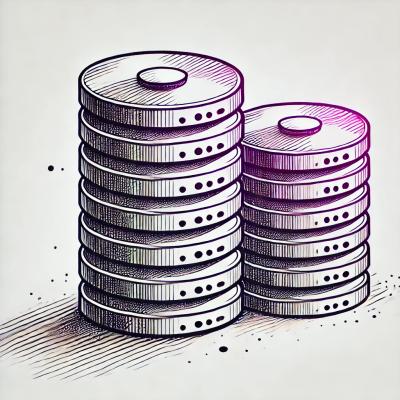
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
container-ioc
Advanced tools
is a Dependency Injection / Inversion of Control (IoC) container package for Javascript and Node.js applications powered by Typescript . It manages the dependencies between classes, so that applications stay easy to change and maintain as they grow.
npm install --save container-ioc
Code examples below are written in Typescript. Check examples/javascript for examples written in Javascript.
Possible values for types: Symbol, string, Object.
interface IApplication {
run(): void;
}
interface IService {
serve(): void;
}
const TApplication = Symbol('IApplication');
const TService = Symbol('IService');
import { Injectable, Inject } from 'container-ioc';
@Injectable()
export class Application implements IApplication {
constructor(@Inject(TService) private service: IService) {}
run(): void {
this.service.serve();
}
}
@Injectable()
export class Service implements IService {
serve(): void {
// serves
}
}
import { Container } from 'container-ioc';
let container = new Container();
container.register([
{ token: TApplication, useClass: Application },
{ token: TService, useClass: Service }
]);
let app = container.resolve(TApplication);
app.run();
Since Javascript does not support parameter decorators, use alternative API for declaring dependencies. In this case we don't use Inject decorator. See examples/javascript for more.
@Injectable([TService])
class Service {
constructor(service) {
this.service = service;
}
}
By default, containers resolve singletons when using useClass and useFactory. Default life time for all items in a container can be set by passing an option object to it's contructor with defailtLifeTime attribute. Possible values: LifeTime.PerRequest (resolves instances) and LifeTime.Persistent (resolves singletons);
import { LifeTime } from 'container-ioc';
const container = new Container({
defaultLifeTime: LifeTime.PerRequest
});
You can also specify life time individually for each item in a container by specifying lifeTime attribute.
container.register([
{
token: TService,
useClass: Service,
lifeTime: LifeTime.PerRequest
}
]);
container.register([
{
token: TService,
useFactory: () => {
return {
serve(): void {}
}
},
lifeTime: LifeTime.Persistent
}
]);
If a container can't find a value within itself, it will look it up in ascendant containers. There a 3 ways to set a parent for a container.
const parentContainer = new Container();
const childContainer = parentContainer.createChild();
const parent = new Container();
const child = new Container();
child.setParent(parent);
const parent = new Container();
const child = new Container({
parent: parent
});
/* Without injections */
container.register([
{
token: 'TokenForFactory',
useFactory: () => {
return 'any-value';
}
}
]);
/* With injections */
container.register([
{ token: 'EnvProvider', useClass: EnvProvider },
{
token: 'TokenForFactory',
useFactory: (envProvider) => {
// do something
return 'something';
},
inject: ['EnvProvider']
}
]);
container.register([
{ token: 'IConfig', useValue: {}}
]);
container.register([
App
]);
Is the same as:
container.register([
{ token: App, useClass: App }
]);
Become a contributor to this project. Feel free to submit an issue or a pull request.
see CONTRIBUTION.md for more information.
Please see also our Code of Conduct.
FAQs
Dependency Injection and Inversion of Control (IoC) container
The npm package container-ioc receives a total of 184 weekly downloads. As such, container-ioc popularity was classified as not popular.
We found that container-ioc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.