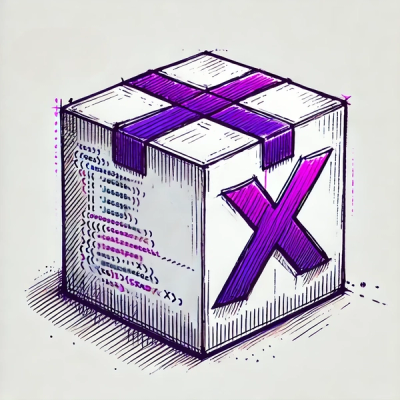
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
cordova-plugin-android-fingerprint-auth-fix
Advanced tools
This plugin fixes no fingerprint hardware available error
Please consult the changelog.
This plugin was created referencing the Fingerprint Dialog sample and the Confirm Credential sample referenced by the Android 6.0 APIs webpage.
This plugin will open a native dialog fragment prompting the user to authenticate using their fingerprint. If the device has a secure lockscreen (pattern, PIN, or password), the user may opt to authenticate using that method as a backup.
This plugin will only work on devices whose manufacturers have implemented the Android 6.0 Fingerprint Authentication API. This plugin does not support the Samsung Pass SDK and not all Samsung devices implement the Android 6.0 Fingerprint Authentication API. If you are testing this plugin on a Samsung device and it is not working, please check the device compatibility before reporting an issue.
cordova plugin add cordova-plugin-android-fingerprint-auth
ionic cordova plugin add cordova-plugin-android-fingerprint-auth
meteor add cordova:cordova-plugin-android-fingerprint-auth@1.2.7 (or present version)
isAvailable()
to check the fingerprint status.encrypt()
or decrypt()
show the Authentication Dialog.delete()
when you want to delete the cipher for the user.If you are not concerned with encrypting credentials and just want device authentication (fingerprint or backup), just call encrypt()
with a clientId
and look for a callback to the successCallback
.
encrypt()
.decrypt()
to return password.Opens a native dialog fragment to use the device hardware fingerprint scanner to authenticate against fingerprints registered for the device.
Param | Type | Description |
---|---|---|
isAvailable | boolean | Fingerprint Authentication Dialog is available for use. |
isHardwareDetected | boolean | Device has hardware fingerprint sensor. |
hasEnrolledFingerprints | boolean | Device has any fingerprints enrolled. |
Example
FingerprintAuth.isAvailable(isAvailableSuccess, isAvailableError);
/**
* @return {
* isAvailable:boolean,
* isHardwareDetected:boolean,
* hasEnrolledFingerprints:boolean
* }
*/
function isAvailableSuccess(result) {
console.log("FingerprintAuth available: " + JSON.stringify(result));
if (result.isAvailable) {
var encryptConfig = {}; // See config object for required parameters
FingerprintAuth.encrypt(encryptConfig, encryptSuccessCallback, encryptErrorCallback);
}
}
function isAvailableError(message) {
console.log("isAvailableError(): " + message);
}
Param | Type | Default | Description |
---|---|---|---|
clientId | String | undefined | (REQUIRED) Used as the alias for your app's secret key in the Android Key Store. Also used as part of the Shared Preferences key for the cipher userd to encrypt the user credentials. |
username | String | undefined | Used to create credential string for encrypted token and as alias to retrieve the cipher. |
password | String | undefined | Used to create credential string for encrypted token |
token | String | undefined | Data to be decrypted. Required for decrypt() . |
disableBackup | boolean | false | Set to true to remove the "USE BACKUP" button |
maxAttempts | number | 5 | The device max is 5 attempts. Set this parameter if you want to allow fewer than 5 attempts. |
locale | String | "en_US" | Change the language displayed on the authentication dialog.
|
userAuthRequired | boolean | false | Require the user to authenticate with a fingerprint to authorize every use of the key. New fingerprint enrollment will invalidate key and require backup authenticate to re-enable the fingerprint authentication dialog. |
encryptNoAuth | boolean | undefined | Bypass authentication and just encrypt input. If true this option will not display the authentication dialog for fingerprint or backup credentials. It will just encrypt the input and return a token. |
dialogTitle | String | undefined | Set the title of the fingerprint authentication dialog. |
dialogMessage | String | undefined | Set the message of the fingerprint authentication dialog. |
dialogHint | String | undefined | Set the hint displayed by the fingerprint icon on the fingerprint authentication dialog. |
Param | Type | Description |
---|---|---|
withFingerprint | boolean | User authenticated using a fingerprint |
withBackup | boolean | User authenticated using backup credentials. |
token | String | Will contain the base64 encoded credentials upon successful fingerprint authentication. |
Example
var encryptConfig = {
clientId: "myAppName",
username: "currentUser",
password: "currentUserPassword"
};
FingerprintAuth.encrypt(encryptConfig, successCallback, errorCallback);
function successCallback(result) {
console.log("successCallback(): " + JSON.stringify(result));
if (result.withFingerprint) {
console.log("Successfully encrypted credentials.");
console.log("Encrypted credentials: " + result.token);
} else if (result.withBackup) {
console.log("Authenticated with backup password");
}
}
function errorCallback(error) {
if (error === FingerprintAuth.ERRORS.FINGERPRINT_CANCELLED) {
console.log("FingerprintAuth Dialog Cancelled!");
} else {
console.log("FingerprintAuth Error: " + error);
}
}
Param | Type | Description |
---|---|---|
withFingerprint | boolean | User authenticated using a fingerprint |
withBackup | boolean | User authenticated using backup credentials. |
password | String | Will contain the decrypted password upon successful fingerprint authentication. |
Example
var decryptConfig = {
clientId: "myAppName",
username: "currentUser",
token: "base64encodedUserCredentials"
};
FingerprintAuth.decrypt(decryptConfig, successCallback, errorCallback);
function successCallback(result) {
console.log("successCallback(): " + JSON.stringify(result));
if (result.withFingerprint) {
console.log("Successful biometric authentication.");
if (result.password) {
console.log("Successfully decrypted credential token.");
console.log("password: " + result.password);
}
} else if (result.withBackup) {
console.log("Authenticated with backup password");
}
}
function errorCallback(error) {
if (error === FingerprintAuth.ERRORS.FINGERPRINT_CANCELLED) {
console.log("FingerprintAuth Dialog Cancelled!");
} else {
console.log("FingerprintAuth Error: " + error);
}
}
Used to delete a cipher.
Param | Type | Default | Description |
---|---|---|---|
clientId | String | undefined | (REQUIRED) Used as the alias for your key in the Android Key Store. |
username | String | undefined | Identify which cipher to delete. |
Example
FingerprintAuth.delete({
clientId: "myAppName",
username: "usernameToDelete"
}, successCallback, errorCallback);
function successCallback(result) {
console.log("Successfully deleted cipher: " + JSON.stringify(result));
}
function errorCallback(error) {
console.log(error);
}
Used to dismiss a Fingerprint Authentication Dialog if one is being displayed
Example
FingerprintAuth.dismiss(successCallback, errorCallback);
function successCallback(result) {
console.log("Successfully dismissed FingerprintAuth dialog: " + JSON.stringify(result));
}
function errorCallback(error) {
console.log(error);
}
JSON Object
Property | Type | Value |
---|---|---|
BAD_PADDING_EXCEPTION | String | "BAD_PADDING_EXCEPTION" |
CERTIFICATE_EXCEPTION | String | "BAD_PADDING_EXCEPTION" |
FINGERPRINT_CANCELLED | String | "FINGERPRINT_CANCELLED" |
FINGERPRINT_DATA_NOT_DELETED | String | "FINGERPRINT_DATA_NOT_DELETED" |
FINGERPRINT_ERROR | String | "FINGERPRINT_ERROR" |
FINGERPRINT_NOT_AVAILABLE | String | "FINGERPRINT_NOT_AVAILABLE" |
FINGERPRINT_PERMISSION_DENIED | String | "FINGERPRINT_PERMISSION_DENIED" |
FINGERPRINT_PERMISSION_DENIED_SHOW_REQUEST | String | "FINGERPRINT_PERMISSION_DENIED_SHOW_REQUEST" |
ILLEGAL_BLOCK_SIZE_EXCEPTION | String | "ILLEGAL_BLOCK_SIZE_EXCEPTION" |
INIT_CIPHER_FAILED | String | "INIT_CIPHER_FAILED" |
INVALID_ALGORITHM_PARAMETER_EXCEPTION | String | "INVALID_ALGORITHM_PARAMETER_EXCEPTION" |
IO_EXCEPTION | String | "IO_EXCEPTION" |
JSON_EXCEPTION | String | "JSON_EXCEPTION" |
MINIMUM_SDK | String | "MINIMUM_SDK" |
MISSING_ACTION_PARAMETERS | String | "MISSING_ACTION_PARAMETERS" |
MISSING_PARAMETERS | String | "MISSING_PARAMETERS" |
NO_SUCH_ALGORITHM_EXCEPTION | String | "NO_SUCH_ALGORITHM_EXCEPTION" |
SECURITY_EXCEPTION | String | "SECURITY_EXCEPTION" |
FRAGMENT_NOT_EXIST | String | "FRAGMENT_NOT_EXIST |
FAQs
This plugin fixes no fingerprint hardware available error
We found that cordova-plugin-android-fingerprint-auth-fix demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.