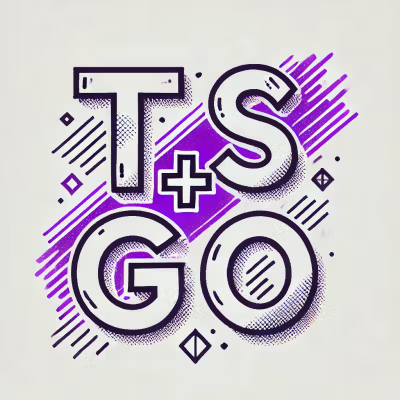
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
corvid-storeon
Advanced tools
A tiny event-based state manager Storeon for Corvid by Wix.
You can use demo template or install from Package Manager.
public/store.js
import { createStoreon } from "corvid-storeon";
const counter = (store) => {
store.on("@init", () => ({ count: 0 }));
store.on("increment", ({ count }) => ({ count: count + 1 }));
};
export const { getState, dispatch, connect, connectPage } = createStoreon([counter]);
Page Code
import { dispatch, connect, connectPage } from "public/store.js";
// Subscribe for state property "count".
// The callback function will be run when the page loads ($w.onReady())
// and each time when property "count" would change.
connect("count", ({ count }) => {
$w("#text1").text = String(count);
});
// Wrapper around $w.onReady()
// The callback function will be run once.
connectPage((state) => {
$w("#button1").onClick(() => {
// Emit event
dispatch("increment");
});
});
You use the Package Manager to manage the npm packages in your site.
Latest available version: v2.3.1
Check status
Creates a store that holds the complete state tree of your app and returns 4 methods for work with the app state.
const { getState, dispatch, connect, connectPage } = createStoreon(modules);
Syntax
function createStoreon(Array<Module | false>): Store
type Store = {
getState: function
dispatch: function
connect: function
connectPage: function
}
Returns an object that holds the complete state of your app.
const state = getState();
Syntax
function getState(): object
Emits an event with optional data.
dispatch("event/type", { value: 123 });
Syntax
function dispatch(event: string, [data: any]): void
Connects to state by property key. It will return the function disconnect from the store.
const disconnect = connect("key", (state) => { });
disconnect();
You can connect for multiple keys, the last argument must be a function.
connect("key1", "key2", (state) => { });
Syntax
function connect(key: string, [key: string, ...], handler: ConnectHandler): Disconnect
callback ConnectHandler(state: object): void | Promise<void>
function Disconnect(): void
Sets the function that runs when all the page elements have finished loading.
(wrapper around $w.onReady()
)
connectPage((state) => { });
Syntax
function connectPage(initFunction: ReadyHandler): void
callback ReadyHandler(state: object): void | Promise<void>
The store should be created with createStoreon()
function.
It accepts a list of the modules.
Each module is just a function, which will accept a store and bind their event listeners.
import wixWindow from "wix-window";
import { createStoreon } from "corvid-storeon";
// Business logic
function appModule(store) {
store.on("@init", () => {
return {
items: [],
};
});
store.on("items/add", ({ items }, item) => {
return {
items: [...items, item],
};
});
}
// Devtools
function logger(store) {
store.on("@dispatch", (state, [event, data]) => {
if (event === "@changed") {
const keys = Object.keys(data).join(', ');
console.log(`changed: ${keys}`, state);
} else if (typeof data !== "undefined") {
console.log(`action: ${event}`, data);
} else {
console.log(`action: ${event}`);
}
});
}
export default createStoreon([
appModule,
(wixWindow.viewMode === "Preview" && logger),
]);
Syntax
function createStoreon(Array<Module | false>): Store
function Module(store: StoreonStore): void
type StoreonStore = {
get: function
on: function
dispatch: function
}
Returns an object that holds the complete state of your app. The app state is always an object.
const state = store.get();
Syntax
function get(): object
Adds an event listener. store.on()
returns cleanup function.
This function will remove the event listener.
const unbind = store.on("event/type", (state, data) => { });
unbind();
Syntax
function on(event: string, listener: EventListener): Unbind
callback EventListener(state: object, [data: any]): Result
function Unbind(): void
type Result = object | void | Promise<void> | false
Emits an event with optional data.
store.dispatch("event/type", { value: "abc" });
Syntax
function dispatch(event: string, [data: any]): void
There are 4 built-in events:
@init
It will be fired in createStoreon()
. The best moment to set an initial state.
store.on("@init", () => { });
@ready
Added in: v2.0.0
It will be fired in $w.onReady()
when all the page elements have finished loading.
store.on("@ready", (state) => { });
@dispatch
It will be fired on every new action (on dispatch()
calls and @changed
event).
It receives an array with the event name and the event’s data.
Can be useful for debugging.
store.on("@dispatch", (state, [event, data]) => { });
@changed
It will be fired when any event changes the state. It receives object with state changes.
store.on("@changed", (state, changes) => { });
You can dispatch any other events. Just do not start event names with @
.
If the event listener returns an object, this object will update the state. You do not need to return the whole state, return an object with changed keys.
// "products": {} will be added to state on initialization
store.on("@init", () => {
return { products: { } };
});
Event listener accepts the current state as a first argument and optional event object as a second.
So event listeners can be a reducer as well. As in Redux’s reducers, you should change immutable.
store.on("products/save", ({ products }, product) => {
return {
products: { ...products, [product._id]: product },
};
});
$w("#buttonAdd").onClick(() => {
dispatch("products/save", {
_id: uuid(),
name: $w("#inputName").value,
});
});
You can dispatch other events in event listeners. It can be useful for async operations.
store.on("products/add", async (_, product) => {
try {
await wixData.save("Products", product);
store.dispatch("products/save", product);
} catch (error) {
store.dispatch("errors/database", error);
}
});
FAQs
This package has been deprecated
The npm package corvid-storeon receives a total of 2 weekly downloads. As such, corvid-storeon popularity was classified as not popular.
We found that corvid-storeon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.