countries-db

This is a minimalistic library to work with countries data.
Usage
NodeJS
Install with npm or yarn:
npm install --save countries-db
Browser
Add the following script to your project (only ~9kb):
<script src="https://cdn.jsdelivr.net/gh/manuelmhtr/countries-db@latest/dist/index.js" type="text/javascript"></script>
<script src="https://cdn.jsdelivr.net/gh/manuelmhtr/countries-db@v1.2.0/dist/index.js" type="text/javascript"></script>
<script type="text/javascript">
var data = countriesDb.getCountry('US');
console.log(data);
</script>
API
.getCountry(id, [property])
Returns a country referenced by its id
.
If the property
argument is specified, it will only return the value of that property.
Example
const countriesDB = require('countries-db');
const population = countriesDB.getCountry('MX', 'population');
console.log(population);
const country = countriesDB.getCountry('MX');
console.log(country);
.getAllCountries()
Returns an object with the data of all countries.
Example
const countriesDB = require('countries-db');
const countries = countriesDB.getAllCountries();
console.log(countries);
Data models
Country
A country is defined by the following parameters:
id | String | The country ISO 3166-1 code. |
name | String | Preferred name of the country. |
officialName | String | The offcial name of the country. |
emoji | String | The Emoji flag of the country . |
emojiUnicode | String | The Emoji flag Unicode of the country . |
iso2 | String | ISO 3166-1 Alpha-2 code of the country. |
iso3 | String | ISO 3166-1 Alpha-3 code of the country. |
isoNumeric | String | ISO 3166-1 Numeric code of the country. |
geonameId | Integer | Unique identifier given by GeoNames. |
continentId | String | Id of the continent where the country is located. Valids are AF (Africa), AS (Asia), EU (Europe), NA (North America), OC (Oceania), SA (South America) and AN (Antarctica). |
population | Integer | The approximate population living in the place. |
elevation | Float | The approximate elevation from sea level. Value is expressed in meters. |
areaSqKm | Integer | Total area of the country. Expressed in squared kilometers. |
coordinates | Object | The geographic coordinates where the place is located. |
coordinates.latitude | Float | Latitude component from the geographic coordinates of the place. |
coordinates.longitude | Float | Longitude component from the geographic coordinates of the place. |
timezones | Array[String] | The list of timezones used in the country. |
domain | String | Top-level domain of the country. |
currencyCode | String | Code of the official currency of the country. |
currencyName | String | Name of the official currency of the country. |
postalCodeFormat | String | Format of the postal codes used in the country. |
postalCodeRegex | String | Regular expression to validate the postal codes used in the country. |
phoneCode | String | The international phone code to call a number in the country. |
neighborCountryIds | Array[String] | A list of ids of the countries that share border with it (neighbors). |
languages | Array[String] | A list of languages spoken in the country. |
locales | Array[String] | A list of locales (language + region) used in the country. |
{
id: 'DE',
name: 'Germany',
officialName: 'The Federal Republic of Germany',
emoji: 'π©πͺ',
emojiUnicode: 'U+1F1E9 U+1F1EA',
iso2: 'DE',
iso3: 'DEU',
isoNumeric: '276',
geonameId: 2921044,
continentId: 'EU',
population: 81802257,
elevation: 303,
areaSqKm: 357021,
coordinates: {
latitude: 51.5,
longitude: 10.5
},
timezones: [ 'Europe/Berlin', 'Europe/Busingen' ],
domain: '.de',
currencyCode: 'EUR',
currencyName: 'Euro',
postalCodeFormat: '#####',
postalCodeRegex: '^(\\d{5})$',
phoneCode: '+49',
neighborCountryIds: [ 'CH', 'PL', 'NL', 'DK', 'BE', 'CZ', 'LU', 'FR', 'AT' ],
languages: [ 'de' ],
locales: [ 'de' ]
}
Related projects
Working on something more complex?
Meet Spott:
- Search any city, country or administrative division in the world. By full strings or autocompletion.
- Find a place by an IP address.
- Access to more than 240,000 geographical places. In more than 20 languages.
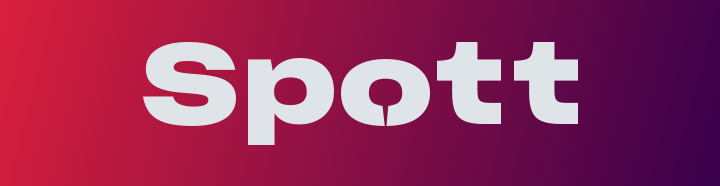
License
MIT