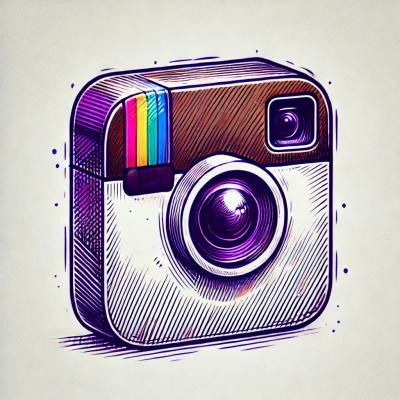
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
create-hmac
Advanced tools
The create-hmac package is a Node.js module that allows you to create HMAC (Hash-based Message Authentication Code) digests using a variety of hashing algorithms. HMAC is a mechanism for message authentication using cryptographic hash functions. This package can be used to generate secure, tamper-proof codes for message verification and authentication purposes.
Creating HMAC Digests
This code demonstrates how to create an HMAC digest using the SHA-256 hashing algorithm and a secret key. The 'update' method is used to input the message, and the 'digest' method outputs the HMAC digest in hexadecimal format.
const createHmac = require('create-hmac');
const hmac = createHmac('sha256', 'secret-key').update('message').digest('hex');
console.log(hmac);
The 'crypto' module is a built-in Node.js module that provides cryptographic functionality, including a set of wrappers for OpenSSL's hash, HMAC, cipher, decipher, sign, and verify functions. It is similar to create-hmac but offers a broader range of cryptographic operations.
jsSHA is a JavaScript implementation of the entire family of SHA hashes as well as HMAC. It is similar to create-hmac but supports a wider range of SHA algorithms and can be used in both browser and Node.js environments.
hash.js is a JavaScript hash library that supports HMAC and various hash algorithms like SHA and MD5. It is similar to create-hmac but is more lightweight and has fewer dependencies, making it suitable for environments where package size is a concern.
Node style HMACs for use in the browser, with native HMAC functions in node. API is the same as HMACs in node:
var createHmac = require('create-hmac')
var hmac = createHmac('sha224', Buffer.from('secret key'))
hmac.update('synchronous write') //optional encoding parameter
hmac.digest() // synchronously get result with optional encoding parameter
hmac.write('write to it as a stream')
hmac.end() //remember it's a stream
hmac.read() //only if you ended it as a stream though
FAQs
node style hmacs in the browser
The npm package create-hmac receives a total of 8,957,219 weekly downloads. As such, create-hmac popularity was classified as popular.
We found that create-hmac demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.