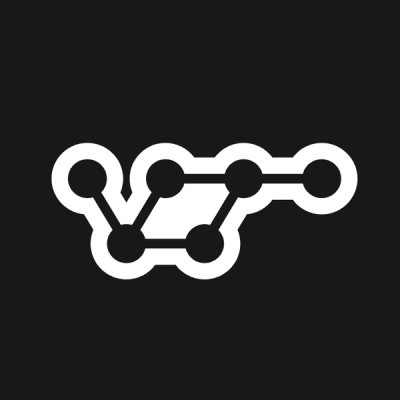
Security News
vlt Launches Real-Time Dependency Analysis Powered by Socket
vlt adds real-time security selectors powered by Socket, enabling developers to query and analyze package risks directly in their dependency graph.
cron-parser
Advanced tools
The cron-parser npm package is a utility for parsing crontab instructions. It allows users to interpret cron schedule expressions and calculate the next run times for jobs. It is useful for scheduling tasks in Node.js applications.
Parsing Cron Expressions
This feature allows users to parse a cron expression and get the next execution times. The code sample demonstrates how to parse a cron expression that runs every 2 minutes and log the next execution time.
const cronParser = require('cron-parser');
try {
const interval = cronParser.parseExpression('*/2 * * * *');
console.log('Date: ', interval.next().toString()); // Get the next date
} catch (err) {
console.error('Error: ' + err.message);
}
Iterating Over Execution Times
This feature allows users to iterate over the execution times of a cron job. The code sample demonstrates how to get the next 5 execution times for a cron expression that runs at the start of every hour.
const cronParser = require('cron-parser');
try {
const interval = cronParser.parseExpression('0 * * * *');
for (let i = 0; i < 5; i++) {
console.log('Date: ', interval.next().toString()); // Get the next 5 dates
}
} catch (err) {
console.error('Error: ' + err.message);
}
Handling Cron Expression with Timezone
This feature allows users to parse a cron expression with a specific timezone. The code sample demonstrates how to parse a cron expression that runs at the start of every hour, considering the timezone of Europe/Amsterdam.
const cronParser = require('cron-parser');
try {
const options = {
currentDate: new Date('Wed, 26 Dec 2012 14:38:53 GMT'),
tz: 'Europe/Amsterdam'
};
const interval = cronParser.parseExpression('0 * * * *', options);
console.log('Date: ', interval.next().toString()); // Get the next date considering timezone
} catch (err) {
console.error('Error: ' + err.message);
}
node-cron is a task scheduler in pure JavaScript for Node.js based on cron syntax. It allows you to schedule tasks to be executed at specific times or intervals. It is similar to cron-parser but also includes the ability to execute the jobs, not just parse cron expressions.
node-schedule is a flexible cron-like and not-cron-like job scheduler for Node.js. It allows for more complex scheduling than standard cron expressions, offering a higher level of customization. It is similar to cron-parser in parsing cron expressions but also provides an extensive job scheduling system.
bree is a job scheduler for Node.js with cron and human-friendly syntax. It supports cron expressions and allows for scheduling jobs with additional features like job timeouts and concurrency control. Bree is more feature-rich compared to cron-parser, which focuses solely on parsing cron expressions.
agenda is a light and flexible job scheduling library for Node.js. It uses MongoDB for job storage and offers features like job prioritization, concurrency, and repeating jobs. While cron-parser is focused on parsing cron expressions, agenda provides a full job scheduling system with persistence.
A JavaScript library for parsing and manipulating cron expressions. Features timezone support, DST handling, and iterator capabilities.
npm install cron-parser
* * * * * *
┬ ┬ ┬ ┬ ┬ ┬
│ │ │ │ │ │
│ │ │ │ │ └─ day of week (0-7, 1L-7L) (0 or 7 is Sun)
│ │ │ │ └────── month (1-12, JAN-DEC)
│ │ │ └─────────── day of month (1-31, L)
│ │ └──────────────── hour (0-23)
│ └───────────────────── minute (0-59)
└────────────────────────── second (0-59, optional)
Character | Description | Example |
---|---|---|
* | Any value | * * * * * (every minute) |
? | Any value (alias for * ) | ? * * * * (every minute) |
, | Value list separator | 1,2,3 * * * * (1st, 2nd, and 3rd minute) |
- | Range of values | 1-5 * * * * (every minute from 1 through 5) |
/ | Step values | */5 * * * * (every 5th minute) |
L | Last day of month/week | 0 0 L * * (midnight on last day of month) |
# | Nth day of month | 0 0 * * 1#1 (first Monday of month) |
Expression | Description | Equivalent |
---|---|---|
@yearly | Once a year at midnight of January 1 | 0 0 0 1 1 * |
@monthly | Once a month at midnight of first day | 0 0 0 1 * * |
@weekly | Once a week at midnight on Sunday | 0 0 0 * * 0 |
@daily | Once a day at midnight | 0 0 0 * * * |
@hourly | Once an hour at the beginning of the hour | 0 0 * * * * |
@minutely | Once a minute | 0 * * * * * |
@secondly | Once a second | * * * * * * |
@weekdays | Every weekday at midnight | 0 0 0 * * 1-5 |
@weekends | Every weekend at midnight | 0 0 0 * * 0,6 |
Field | Values | Special Characters | Aliases |
---|---|---|---|
second | 0-59 | * ? , - / | |
minute | 0-59 | * ? , - / | |
hour | 0-23 | * ? , - / | |
day of month | 1-31 | * ? , - / L | |
month | 1-12 | * ? , - / | JAN -DEC |
day of week | 0-7 | * ? , - / L # | SUN -SAT (0 or 7 is Sunday) |
Option | Type | Description |
---|---|---|
currentDate | Date | string | number | Current date. Defaults to current local time in UTC |
endDate | Date | string | number | End date of iteration range. Sets iteration range end point |
startDate | Date | string | number | Start date of iteration range. Set iteration range start point |
tz | string | Timezone (e.g., 'Europe/London') |
strict | boolean | Enable strict mode validation |
When using string dates, the following formats are supported:
import { CronExpressionParser } from 'cron-parser';
try {
const interval = CronExpressionParser.parse('*/2 * * * *');
// Get next date
console.log('Next:', interval.next().toString());
// Get next 3 dates
console.log(
'Next 3:',
interval.take(3).map((date) => date.toString()),
);
// Get previous date
console.log('Previous:', interval.prev().toString());
} catch (err) {
console.log('Error:', err.message);
}
import { CronExpressionParser } from 'cron-parser';
const options = {
currentDate: '2023-01-01T00:00:00Z',
endDate: '2024-01-01T00:00:00Z',
tz: 'Europe/London',
};
try {
const interval = CronExpressionParser.parse('0 0 * * *', options);
console.log('Next:', interval.next().toString());
} catch (err) {
console.log('Error:', err.message);
}
For working with crontab files, use the CronFileParser:
import { CronFileParser } from 'cron-parser';
// Async file parsing
try {
const result = await CronFileParser.parseFile('/path/to/crontab');
console.log('Variables:', result.variables);
console.log('Expressions:', result.expressions);
console.log('Errors:', result.errors);
} catch (err) {
console.log('Error:', err.message);
}
// Sync file parsing
try {
const result = CronFileParser.parseFileSync('/path/to/crontab');
console.log('Variables:', result.variables);
console.log('Expressions:', result.expressions);
console.log('Errors:', result.errors);
} catch (err) {
console.log('Error:', err.message);
}
In several implementations of CRON, it's ambiguous to specify both the Day Of Month and Day Of Week parameters simultaneously, as it's unclear which one should take precedence. Despite this ambiguity, this library allows both parameters to be set by default, although the resultant behavior might not align with your expectations.
To resolve this ambiguity, you can activate the strict mode of the library. When strict mode is enabled, the library enforces several validation rules:
These validations help ensure that your cron expressions are unambiguous and correctly formatted.
import { CronExpressionParser } from 'cron-parser';
// This will throw an error in strict mode because it uses both dayOfMonth and dayOfWeek
const options = {
currentDate: new Date('Mon, 12 Sep 2022 14:00:00'),
strict: true,
};
try {
// This will throw an error in strict mode
CronExpressionParser.parse('0 0 12 1-31 * 1', options);
} catch (err) {
console.log('Error:', err.message);
// Error: Cannot use both dayOfMonth and dayOfWeek together in strict mode!
}
// This will also throw an error in strict mode because it has fewer than 6 fields
try {
CronExpressionParser.parse('0 20 15 * *', { strict: true });
} catch (err) {
console.log('Error:', err.message);
// Error: Invalid cron expression, expected 6 fields
}
The library supports parsing the range 0L - 7L
in the weekday
position of the cron expression, where the L
means "last occurrence of this weekday for the month in progress".
For example, the following expression will run on the last Monday of the month at midnight:
import { CronExpressionParser } from 'cron-parser';
// Last Monday of every month at midnight
const lastMonday = CronExpressionParser.parse('0 0 0 * * 1L');
// You can also combine L expressions with other weekday expressions
// This will run every Monday and the last Wednesday of the month
const mixedWeekdays = CronExpressionParser.parse('0 0 0 * * 1,3L');
// Last day of every month
const lastDay = CronExpressionParser.parse('0 0 L * *');
import { CronExpressionParser } from 'cron-parser';
const interval = CronExpressionParser.parse('0 */2 * * *');
// Using for...of
for (const date of interval) {
console.log('Iterator value:', date.toString());
if (someCondition) break;
}
// Using take() for a specific number of iterations
const nextFiveDates = interval.take(5);
console.log(
'Next 5 dates:',
nextFiveDates.map((date) => date.toString()),
);
The library provides robust timezone support using Luxon, handling DST transitions correctly:
import { CronExpressionParser } from 'cron-parser';
const options = {
currentDate: '2023-03-26T01:00:00',
tz: 'Europe/London',
};
const interval = CronExpressionParser.parse('0 * * * *', options);
// Will correctly handle DST transition
console.log('Next dates during DST transition:');
console.log(interval.next().toString());
console.log(interval.next().toString());
console.log(interval.next().toString());
You can modify cron fields programmatically using CronFieldCollection.from
and construct a new expression:
import { CronExpressionParser, CronFieldCollection, CronHour, CronMinute } from 'cron-parser';
// Parse original expression
const interval = CronExpressionParser.parse('0 7 * * 1-5');
// Create new collection with modified fields using raw values
const modified = CronFieldCollection.from(interval.fields, {
hour: [8],
minute: [30],
dayOfWeek: [1, 3, 5],
});
console.log(modified.stringify()); // "30 8 * * 1,3,5"
// You can also use CronField instances
const modified2 = CronFieldCollection.from(interval.fields, {
hour: new CronHour([15]),
minute: new CronMinute([30]),
});
console.log(modified2.stringify()); // "30 15 * * 1-5"
The CronFieldCollection.from
method accepts either CronField instances or raw values that would be valid for creating new CronField instances. This is particularly useful when you need to modify only specific fields while keeping others unchanged.
MIT
FAQs
Node.js library for parsing crontab instructions
The npm package cron-parser receives a total of 3,824,629 weekly downloads. As such, cron-parser popularity was classified as popular.
We found that cron-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt adds real-time security selectors powered by Socket, enabling developers to query and analyze package risks directly in their dependency graph.
Security News
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.
Product
Socket's Rubygems ecosystem support is moving from beta to GA, featuring enhanced security scanning to detect supply chain threats beyond traditional CVEs in your Ruby dependencies.