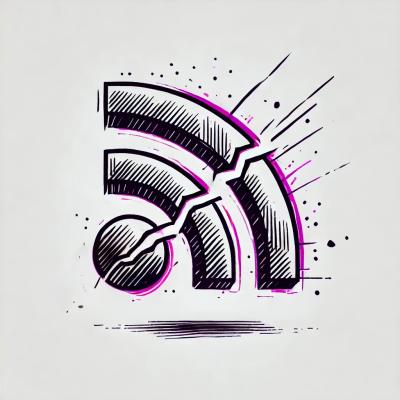
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
cyl-hooks-api
Advanced tools
这是一套基于react上下文的api管理方法,内置许多功能性的hooks
npm install --save cyl-hooks-api
Or:
yarn add cyl-hooks-api
Or:
pnpm add cyl-hooks-api
ApiProvider
ApiProvider
组件必须在所有组件的入口出使用,这样才能保证页面种的各种hooks使用正常,如果还是不懂,那就请学习一下
react Context 的使用
import React from 'react'
import ReactDOM from 'react-dom'
import App from './App'
import {ApiProvider} from "cyl-hooks-api"
/**
* @tip 可以不是axios,随便一个对象就可以,只要是实现了 get post delete put 这几个方法就可以
*/
import axios from "axios"
ReactDOM.render(
<React.StrictMode>
<ApiProvider httpClient={axios}>
<App />
</ApiProvider>
</React.StrictMode>,
document.getElementById('root')
)
useApi
这个hooks用户获取上下文
T 入口出 ApiProvider 这个组件传入的 `httpClient` 类型
import {useApi} from "cyl-hooks-api"
export default function Component(){
const api = useApi()
return <div>我是组件</div>
}
useApiState
这个hooks用于请求接口并获取接口返回结果以及请求过程的状态,以及请求的错误
`test.ts`
import {ContextInstance,Context} from "cyl-hooks-api"
export interface Test {
id:number
}
export function getTest(ctx:Context<ContextInstance,any>,id:number):Promise<Test>{
return ctx.get("/test",{
param:{
id
}
})
}
import {useApi,useApiState} from "cyl-hooks-api"
import {getTest} from "./test.ts"
export default function Component(){
const api = useApi()
const [id,setId] = useState(0)
const [test,loading,error] = useApiState(api,getTest,id)
return <div>
<div>状态:{loading ? "请求中" : "请求完成"}</div>
<div>错误:{error ? error?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(test)}</div>
</div>
}
useApiAnyError
切记是一个组件里面的 这个hooks用户一个组件的所有错误,意思就是一个组件所有的请求只要出错误这个hooks都会捕获到。
import {useApi,useApiState,useApiAnyError} from "cyl-hooks-api"
import {getTest} from "./test.ts"
import {getapp} from "./app.ts"
export default function Component(){
const api = useApi()
const [id,setId] = useState(0)
const [test] = useApiState(api,getTest,id)
const [app] = useApiState(api,getapp,id)
const anyError = useApiAnyError(api)
return <div>
<div>错误:{anyError ? anyError?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(test)}</div>
</div>
}
useApiAnyLoading
切记是一个组件里面的 这个hooks用户一个组件的所有请求状态,意思就是一个组件所有的请求只要有一个没有完成它就还是请求中。
import {useApi,useApiState,useApiAnyLoading} from "cyl-hooks-api"
import {getTest} from "./test.ts"
import {getapp} from "./app.ts"
export default function Component(){
const api = useApi()
const [id,setId] = useState(0)
const [test] = useApiState(api,getTest,id)
const [app] = useApiState(api,getapp,id)
const AnyLoading = useApiAnyLoading(api)
return <div>
<div>状态:{AnyLoading ? "请求中" : "请求完成"}</div>
<div>结果:{JSON.stringify(test)}</div>
</div>
}
useCoEffect
与useEffect类似,可以接受一个Generator函数callback, callback函数中可以 yield Promise,与async函数中的await Promise功能相同 当deps发生变化或Component被卸载的时候, 这个Generator函数的执行会中断在当前的yield位置。 中断时既不执行下一个语句,也不抛出异常, 但finally块在中断后仍会被执行。 callback函数的finally块中可以使用isCancelled检查 当前执行过程是否被取消
import {useApi} from "cyl-hooks-api"
import {useCoEffect} from "cyl-hooks-api/useCo"
import {getTest} from "./test.ts"
import {getapp} from "./app.ts"
export default function Component(){
const api = useApi()
const [appId,setAppId] = useState(0)
const [testId,setTestId] = useState(0)
useCoEffect(function *(){
yield getapp(api,appId)
yield getTest(api,testId)
console.log("请求完毕")
},[])
return <div>
useCoEffect
</div>
}
useCoCallback
与useCallback类似,可以接受一个Generator函数callback, callback函数中可以 yield Promise,与async函数中的await Promise功能相同 当deps发生变化或Component被卸载的时候, 这个Generator函数的执行会中断在当前的yield位置。 中断时既不执行下一个语句,也不抛出异常, 但finally块在中断后仍会被执行。 callback函数的finally块中可以使用isCancelled检查 当前执行过程是否被取消
import {useApi,useApiState} from "cyl-hooks-api"
import {useCoCallback} from "cyl-hooks-api/useCo"
import {getTest} from "./test.ts"
import {getApp} from "./app.ts"
export default function Component(){
const api = useApi()
const [testId,setTestId] = useState(0)
const [test,loading,error] = useApiState(api,getTest,testId)
const onChangeTest = useCoCallback(function *(){
const i = yield getApp()
setTestId(i)
},[testId])
return <div>
<div>状态:{loading ? "请求中" : "请求完成"}</div>
<div>错误:{error ? error?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(test)}</div>
<button onclick={onChangeTest}>更新</button>
</div>
}
useApiCollectionFetcher
通过上一个useApiState 返回的数据【必须是数组】里面的某个唯一字段来请求另一个接口
import {useApi,useApiState} from "cyl-hooks-api"
import {useApiCollectionFetcher} from "cyl-hooks-api/useCollector"
import {getTests} from "./test.ts"
import {getAppByTestId} from "./app.ts"
export default function Component(){
const api = useApi()
const [testId,setTestId] = useState(0)
const [tests] = useApiState(api,getTests)
const [apps,loading,error] =useApiCollectionFetcher(api,tests,t=>t?.id,getAppByTestId)
return <div>
<div>状态:{loading ? "请求中" : "请求完成"}</div>
<div>错误:{error ? error?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(apps)}</div>
<button onclick={onChangeTest}>更新</button>
</div>
}
useCollector
取出数据【必须是数组对象】中的一个字段然后以数组返回
import {useApi,useApiState} from "cyl-hooks-api"
import {useCollector} from "cyl-hooks-api/useCollector"
import {getTests} from "./test.ts"
import {getAppByTestId} from "./app.ts"
export default function Component(){
const api = useApi()
const [testId,setTestId] = useState(0)
const [tests,loading,error] = useApiState(api,getTests)
const testIds = useCollector(tests,t=>t?.id)
return <div>
<div>状态:{loading ? "请求中" : "请求完成"}</div>
<div>错误:{error ? error?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(testIds)}</div>
<button onclick={onChangeTest}>更新</button>
</div>
}
useWebsocket
连接websocket
import {useApi,useApiState} from "cyl-hooks-api"
import {logger} from "cyl-hooks-api/utils"
import {useCollector} from "cyl-hooks-api/useCollector"
import {makeWebsocketUrlFromRelativeUrl,useWebsocket} from "cyl-hooks-api/useWebsocket"
import {getTests} from "./test.ts"
import {getAppByTestId} from "./app.ts"
export default function Component(){
const api = useApi()
useWebsocket("id",makeWebsocketUrlFromRelativeUrl("/ws"),(id,ws,eventName,eventData,data)=>{
logger.info(data)
},[])
const [testId,setTestId] = useState(0)
const [tests,loading,error] = useApiState(api,getTests)
const testIds = useCollector(tests,t=>t?.id)
return <div>
<div>状态:{loading ? "请求中" : "请求完成"}</div>
<div>错误:{error ? error?.message : "没有错误"}</div>
<div>结果:{JSON.stringify(testIds)}</div>
<button onclick={onChangeTest}>更新</button>
</div>
}
工具方法
FAQs
这是一套基于react上下文的api管理方法,内置许多功能性的hooks
The npm package cyl-hooks-api receives a total of 0 weekly downloads. As such, cyl-hooks-api popularity was classified as not popular.
We found that cyl-hooks-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.