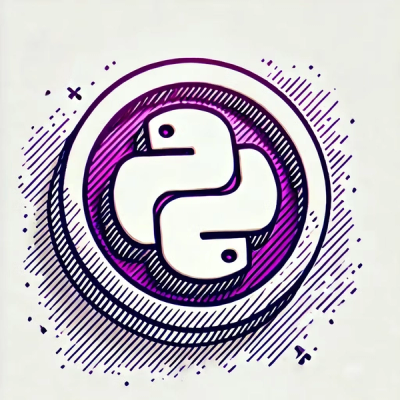
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
d3-regression
Advanced tools
Calculate statistical regressions from two-dimensional data.
If you use NPM, npm install d3-regression
. Otherwise, download the latest release. AMD, CommonJS, and vanilla environments are supported. In vanilla, a d3 global is exported:
<script src="https://unpkg.com/d3-regression@1.3.10/dist/d3-regression.min.js"></script>
<script>
const regression = d3.regressionLinear()
.x(d => d.x)
.y(d => d.y)
.domain([0, 100]);
</script>
# d3.regressionLinear() · Source, Example
Creates a new linear regression generator with default x- and y- accessors and a null domain.
Computes the linear regression, which takes the form y = ax + b, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a line represented as an array of two points, where each point is an array of two numbers representing the point's coordinates.
Also returns properties a and b, representing the equation's coefficients, and rSquared, representing the coefficient of determination. Lastly, returns a predict property, which is a function that outputs a y-coordinate given an input x-coordinate.
If x is specified, sets the x-coordinate accessor, which is passed passed the current datum (d), the current index (i), and the entire data array (data). If x is not specified, returns the current x-coordinate accessor, which defaults to:
function x(d, i, data) {
return d[0];
}
If y is specified, sets the y-coordinate accessor, which is passed passed the current datum (d), the current index (i), and the entire data array (data). If y is not specified, returns the current y-coordinate accessor, which defaults to:
function y(d, i, data) {
return d[1];
}
# linear.domain([domain]) · Source
If domain is specified, sets the minimum and maximum x-coordinates of the returned line to the specified array of numbers. The array must contain two elements. If the elements in the given array are not numbers, they will be coerced to numbers. If domain is not specified, returns a copy of the regression generator’s current domain.
If data is passed to the regression generator before a domain has been specified, the domain will be set to the minimum and maximum x-coordinate values of the data.
# d3.regressionExp() · Source, Example
Creates a new exponential regression generator with default x- and y- accessors and a null domain.
Computes the exponential regression, which takes the form y = aebx, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a smooth line represented as an array of points, where each point is an array of two numbers representing the point's coordinates.
Also returns properties a and b, representing the equation's coefficients, and rSquared, representing the coefficient of determination. Lastly, returns a predict property, which is a function that outputs a y-coordinate given an input x-coordinate.
See linear.x().
See linear.y().
# exp.domain([domain]) · Source
See linear.domain().
# d3.regressionLog() · Source, Example
Creates a new logarithmic regression generator with default x- and y- accessors and a null domain.
Computes the logarithmic regression, which takes the form y = a · ln(x) + b, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a smooth line represented as an array of points, where each point is an array of two numbers representing the point's coordinates.
Also returns properties a and b, representing the equation's coefficients, and rSquared, representing the coefficient of determination. Lastly, returns a predict property, which is a function that outputs a y-coordinate given an input x-coordinate.
See linear.x().
See linear.y().
# log.domain([domain]) · Source
See linear.domain().
If base is specified, sets the base of the logarithmic regression. If base is not specified, returns the current base, which defaults to Euler's number.
# d3.regressionQuad() · Source, Example
Creates a new quadratic regression generator with default x- and y- accessors and a null domain.
Computes the quadratic regression, which takes the form y = ax2 + bx + c, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a smooth line represented as an array of points, where each point is an array of two numbers representing the point's coordinates.
Also returns properties a, b, and c, representing the equation's coefficients, and rSquared, representing the coefficient of determination. Lastly, returns a predict property, which is a function that outputs a y-coordinate given an input x-coordinate.
See linear.x().
See linear.y().
# quad.domain([domain]) · Source
See linear.domain().
# d3.regressionPoly() · Source, Example
Creates a new polynomial regression generator with default x- and y- accessors, a null domain, and an order of 3. This implementation was adapted from regression-js.
Computes the polynomial regression, which takes the form y = anxn + ... + a1x + a0, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a smooth line represented as an array of points, where each point is an array of two numbers representing the point's coordinates.
Also returns three properties: coefficients, an array representing the equation's coefficients with the intercept as the first item and nth degree coefficient as the last item; rSquared, representing the coefficient of determination; and predict, a function that outputs a y-coordinate given an input x-coordinate.
See linear.x().
See linear.y().
# poly.domain([domain]) · Source
See linear.domain().
# poly.order([order]) · Source
If order is specified, sets the regression's order to the specified number. For example, if order is set to 4, the regression generator will perform a fourth-degree polynomial regression. Likewise, if order is set to 2, the regression generator will perform a quadratic regression. Be careful about attempting to fit your data with higher order polynomials; though the regression line will fit your data with a high determination coefficient, it may have little predictive power for data outside of your domain.
If order is not specified, returns the regression generator's current order, which defaults to 3.
# d3.regressionPow() · Source, Example
Creates a new power law regression generator with default x- and y- accessors and a null domain.
Computes the power law regression, which takes the form y = axb, for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a smooth line represented as an array of points, where each point is an array of two numbers representing the point's coordinates.
Also returns properties a and b, representing the equation's coefficients, and rSquared, representing the coefficient of determination. Lastly, returns a predict property, which is a function that outputs a y-coordinate given an input x-coordinate.
See linear.x().
See linear.y().
# pow.domain([domain]) · Source
See linear.domain().
# d3.regressionLoess() · Source, Example
Creates a new LOESS regression generator with default x- and y- accessors and a bandwidth of .3. This implementation was adapted from science.js.
Computes the LOESS regression for the specified data points, ignoring points with invalid values (null, undefined, NaN, Infinity).
Returns a line represented as an array of n points, where each point is an array of two numbers representing the point's coordinates.
See linear.x().
See linear.y().
# loess.bandwidth([bandwidth]) · Source
If bandwidth is specified, sets the LOESS regression's bandwidth, or smoothing parameter, to the specific number between 0 and 1. The bandwidth represents the share of the total data points that are used to calculate each local fit. Higher bandwidths produce smoother lines, and vice versa. If bandwidth is not specified, returns a copy of the regression generator’s current bandwidth, which defaults to .3.
FAQs
Calculate statistical regressions for two-dimensional data
The npm package d3-regression receives a total of 101,493 weekly downloads. As such, d3-regression popularity was classified as popular.
We found that d3-regression demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.