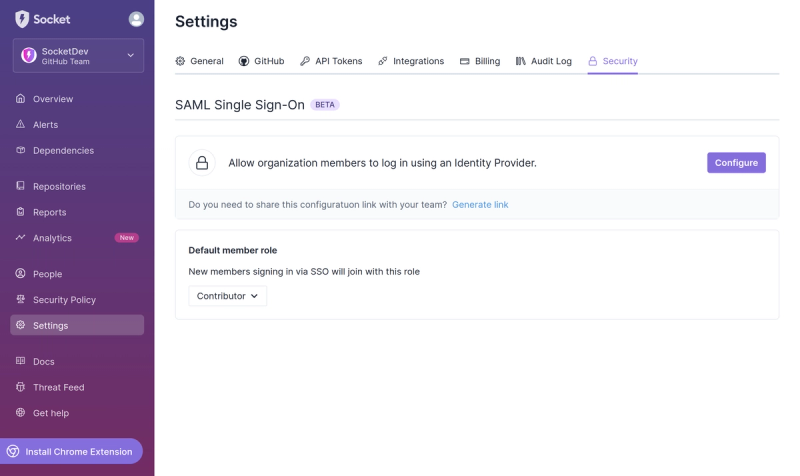
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
dam-wizmkj
Advanced tools
Changelog
Readme
Formik wizard form is a library which lets you build multistep form wizards in React with ease. It has been written over the famous form library formik and provides the enhanced versions of formik's <Formik />
and useFormik
hook. Formik wizard form requires few additional props along with <Formik />
original props as well as adds some properties to the render props object.
Apart from that, the library is style or css framework agnostic and leaves the rendering part completely up to you. It just provides render props and you build your UI out of them.
For more information on usage, please refer to the How To Use and Examples section.
Install the package from npm or yarn registry.
yarn add formik-wizard-form
npm install formik-wizard-form --save
Formik wizard form exports two components <FormWizard />
and useFormikWizard
similar to Formik's <Formik />
and useFormik
hook and their original props have been left untouched. Rather they require you to provide few additional props to create a form wizard.
import { FormikWizard } from 'formik-wizard-form';
<FormikWizard />
is a replacement of original <Formik />
component and accepts all the formik props along with the following listed:
steps: Step[]
steps is an array of form step objects where each object contains the information about a particular step component. Each step object must satisfy the below typescript type.
type Step = {
/** Validation schema object for the current step */
validationSchema?: any | (() => any);
/** Handler to be called before moving to previous step */
beforePrev?: (
values: FormikValues,
formikBag: FormikProps<FormikValues>,
currentStepIndex: number
) => Promise<any>;
/** Handler to be called before moving to next step */
beforeNext?: (
values: FormikValues,
formikBag: FormikProps<FormikValues>,
currentStepIndex: number
) => Promise<any>;
/** React functional or class component */
component: React.ComponentType<FormikProps<FormikValues>>;
};
Promise
. Rejecting this promise won't let you go to previous step.activeStepIndex: number
activeStepIndex is the index of step which you want to make active by default on form render. Starting from zero.
validateOnNext: boolean
validateOnNext is a boolean flag which controls whether to by pass the form validations or prevent moving backward/forward in case of invalid form.
children: ((props: RenderProps) => React.ReactNode) | React.ReactElement<RenderProps>
children prop type is the same as formik but to render stepper forms, it must be a function which will get RenderProps
as its arguments.
If you provide children
as a function, it will be provided the following arguments in return along with the standard/original formik render props.
interface RenderProps extends FormikProps<FormikValues> {
/** Handler to be called on previous button click */
handlePrev: () => void;
/** Handler to be called on next button click */
handleNext: () => void;
/** Current step index in number */
currentStepIndex?: number;
/** Flag to indicate previous button should be disabled */
isPrevDisabled: boolean;
/** Flag to indicate next button should be disabled */
isNextDisabled: boolean;
/** Flag to indicate current step is first step */
isFirstStep: boolean;
/** Flag to indicate current step is last step */
isLastStep: boolean;
/** Current step component renderer */
renderComponent: () => React.ReactNode;
}
<FormikWizard
initialValues={{ firstName: '', email: '', designation: '' }}
onSubmit={values => console.log(values)}
validateOnNext
activeStepIndex={0}
steps={[
{
component: PersonalDetails,
validationSchema: Yup.object().shape({
firstName: Yup.string().required('First name is required'),
}),
},
{
component: ContactDetails,
validationSchema: Yup.object().shape({
email: Yup.string().required('Email is required'),
}),
},
{
component: JobDetails,
validationSchema: Yup.object().shape({
designation: Yup.string().required('Designation is required'),
}),
},
]}
>
{({
renderComponent,
handlePrev,
handleNext,
isNextDisabled,
isPrevDisabled,
isLastStep,
}) => (
<>
{renderComponent()}
<button type="button" onClick={handlePrev} disabled={isPrevDisabled}>
Previous
</button>
<button type="button" onClick={handleNext} disabled={isNextDisabled}>
{isLastStep ? 'Finish' : 'Next'}
</button>
</>
)}
</FormikWizard>
import { useFormikWizard } from 'formik-wizard-form';
useFormikWizard
is a replacement of original useFormik
hook and accepts all the formik props along with WizardProps
interface:
interface WizardProps extends FormikConfig<FormikValues> {
/** Default active step index for the wizard */
activeStepIndex: number;
/** Wizard steps array given below */
steps: Step[];
/** Must be a function or react element */
children?:
| ((props: RenderProps) => React.ReactNode)
| React.ReactElement<RenderProps>;
/** Should validate the form before moving to next step */
validateOnNext?: boolean;
}
type Step = {
/** Validation schema object for the current step */
validationSchema?: any | (() => any);
/** Handler to be called before moving to previous step */
beforePrev?: (
values: FormikValues,
formikBag: FormikProps<FormikValues>,
currentStepIndex: number
) => Promise<any>;
/** Handler to be called before moving to next step */
beforeNext?: (
values: FormikValues,
formikBag: FormikProps<FormikValues>,
currentStepIndex: number
) => Promise<any>;
/** React functional or class component */
component: React.ComponentType<FormikProps<FormikValues>>;
};
steps: Step[]
steps is an array of form step objects where each object contains the information about a particular step component. Each step object must satisfy the below typescript type.
activeStepIndex: number
activeStepIndex is the index of step which you want to make active by default on form render. Starting from zero.
validateOnNext: boolean
validateOnNext is a boolean flag which controls whether to by pass the form validations or prevent moving backward/forward in case of invalid form.
useFormikWizard()
returns an object of render props and method containing the below interface:
interface RenderProps extends FormikProps<FormikValues> {
/** Handler to be called on previous button click */
handlePrev: () => void;
/** Handler to be called on next button click */
handleNext: () => void;
/** Current step index in number */
currentStepIndex?: number;
/** Flag to indicate previous button should be disabled */
isPrevDisabled: boolean;
/** Flag to indicate next button should be disabled */
isNextDisabled: boolean;
/** Flag to indicate current step is first step */
isFirstStep: boolean;
/** Flag to indicate current step is last step */
isLastStep: boolean;
/** Current step component renderer */
renderComponent: () => React.ReactNode;
}
const MultiStepForm = () => {
const {
renderComponent,
handlePrev,
handleNext,
isNextDisabled,
isPrevDisabled,
isLastStep,
} = useFormikWizard({
initialValues: { firstName: '', lastName: '' },
onSubmit: values => console.log(values),
validateOnNext: true,
activeStepIndex: 0,
steps: [
{
component: PersonalDetails,
validationSchema: Yup.object().shape({
firstName: Yup.string().required('First name is required'),
}),
},
{
component: ContactDetails,
validationSchema: Yup.object().shape({
email: Yup.string().required('Email is required'),
}),
},
{
component: JobDetails,
validationSchema: Yup.object().shape({
designation: Yup.string().required('Designation is required'),
}),
},
],
});
return (
<div>
{renderComponent()}
<button type="button" onClick={handlePrev} disabled={isPrevDisabled}>
Previous
</button>
<button type="button" onClick={handleNext} disabled={isNextDisabled}>
{isLastStep ? 'Finish' : 'Next'}
</button>
</div>
);
};
FAQs
Create stepper forms over formik with ease
The npm package dam-wizmkj receives a total of 0 weekly downloads. As such, dam-wizmkj popularity was classified as not popular.
We found that dam-wizmkj demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.