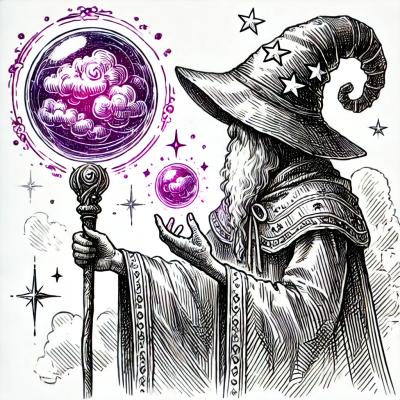
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
darvin-testing-framework
Advanced tools
A framework for testing Darvin.ai chatbots on nodejs with jasmine.
Install
npm install darvin-testing-framework
Require darvin-testing-framework
in a javascript file which will be executed by jasmine.
Click here for more info about jasmine test execution.
const DarvinTestingFramework = require('darvin-testing-framework');
const darvin = new DarvinTestingFramework();
Instruct the Darvin Testing Framework to define BDD specifications for your bot based on a declarative specification that you provide.
const accountantBotSpec = require('./accountant-bot.spec.json');
const appointmentsBotSpec = require('./appointments-bot.spec.json');
darvin.describe(accountantBotSpec);
darvin.describe(appointmentsBotSpec);
The JSON specification must have the following properties:
name
- This is the name of your chatbot. It will be used as a name for its own test suitebotId
- The Id of the chatbot that is being testedchannel
- An object that contains communication details of the target chatbot
id
- Id of the system communication channel of the target chatbottoken
- Verification token of the system communication channel of the target chatbotscenarios
- an array of scenarios describing the chatbot's behavior
it
- follows the idea of behavior-driven development and serves as the first word in the test name, which should be a complete sentencesteps
- sequence (array) of steps defining the scenario
user
- what the user says as a single message
text
- text of the messagebot
- what is the expected answer from the bot as an array of optional behavior. Each element is an array of messages. The step is considered valid if any sequence of messages matches the actual chatbot response
text
- text of a single messagequickReplies
- array of quick reply optionsThe following example spec verifies that the Sample bot introduces itself and enters into a conversation
.
{
"name": "Sample bot",
"botId": "[your bot id]",
"channel": {
"id": "[id of the system channel]",
"token": "[verification token]"
},
"scenarios": [
{
"it": "introduces itself and enters into a conversation",
"steps": [
{
"user": { "text": "hi" },
"bot": [
[
{
"text": "This is a getting started conversation for your chatbot.",
"quickReplies": ["Conversation 1", "Conversation 2"]
}
]
]
},
{
"user": { "text": "Conversation 1" },
"bot": [
[
{
"text": "This is conversation 1"
}
]
]
}
]
}
]
}
Our QuickStart sample has specifications with it. Feel free to explore it in order to get more familiar with the format. Click here to get it. It only has scenarios
since it is a general specification and it is not targeted at a specific chatbot isntance.
FAQs
A framework for testing Progress NativeChat bots.
The npm package darvin-testing-framework receives a total of 392 weekly downloads. As such, darvin-testing-framework popularity was classified as not popular.
We found that darvin-testing-framework demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.