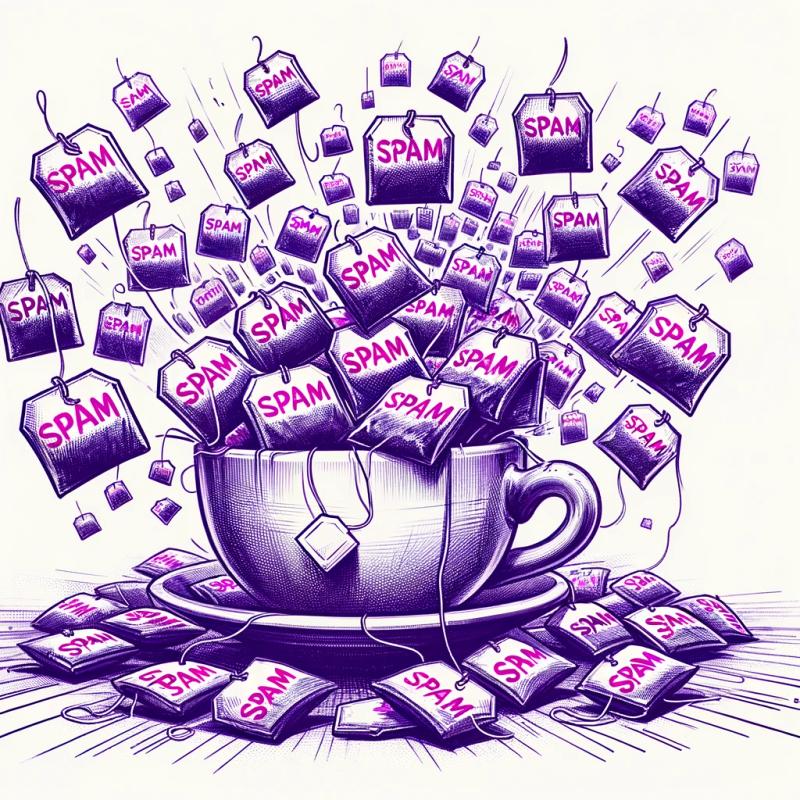
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
datastructuresbymick
Advanced tools
Readme
A simple yet powerful PDF generation tool written in TypeScript.
npm install pdf-generator
const PDFGenerator = require("pdf-generator");
// Create a new PDFGenerator instance
const pdfGenerator = new PDFGenerator();
// Add content to the PDF
pdfGenerator
.addText("Hello, this is a PDF generated using PDFGenerator!", {
fontSize: 20,
align: "center",
})
.addPage()
.addText("This is page 2 of the PDF.", {
y: 100,
align: "center",
});
// Save the PDF
pdfGenerator.save();
PDFGenerator(options?: PDFGeneratorOptions)
Creates a new instance of PDFGenerator with optional options.
options.filename
: Specify the filename for the generated PDF. Default is 'output.pdf'
.addText(text: string, options?: TextOptions): PDFGenerator
Adds text to the PDF document.
text
: The text content to add.options
: Optional parameters for text formatting, such as fontSize, font, alignment, etc.addPage(): PDFGenerator
Adds a new page to the PDF document.
save(): void
Saves the PDF document to the specified filename.
Check the example
directory for an example usage of the PDFGenerator.
This project is licensed under the MIT License - see the LICENSE file for details.
DataStructures is a lightweight library for common data structures in JavaScript.
You can install DataStructures via npm:
npm install data-structures
const { Stack, Queue } = require('data-structures');
// Create a new stack
const stack = new Stack();
// Push elements onto the stack
stack.push(1);
stack.push(2);
stack.push(3);
// Pop elements from the stack
console.log(stack.pop()); // Output: 3
// Create a new queue
const queue = new Queue();
// Enqueue elements into the queue
queue.enqueue('a');
queue.enqueue('b');
queue.enqueue('c');
// Dequeue elements from the queue
console.log(queue.dequeue()); // Output: 'a'
Stack
A stack is a collection of elements that follows the Last In, First Out (LIFO) principle.
push(element)
: Add an element to the top of the stack.pop()
: Remove and return the top element from the stack.peek()
: Return the top element of the stack without removing it.isEmpty()
: Check if the stack is empty.size()
: Return the number of elements in the stack.clear()
: Remove all elements from the stack.Queue
A queue is a collection of elements that follows the First In, First Out (FIFO) principle.
enqueue(element)
: Add an element to the back of the queue.dequeue()
: Remove and return the front element from the queue.front()
: Return the front element of the queue without removing it.isEmpty()
: Check if the queue is empty.size()
: Return the number of elements in the queue.clear()
: Remove all elements from the queue.This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Implement common data structures like stacks, queues, linked lists, trees, graphs, etc. This package could be helpful for developers who need to work with complex data structures in their applications
The npm package datastructuresbymick receives a total of 1 weekly downloads. As such, datastructuresbymick popularity was classified as not popular.
We found that datastructuresbymick demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.