decentraland-server
Advanced tools
Comparing version 2.0.1 to 2.1.0
@@ -0,5 +1,6 @@ | ||
import * as program from 'commander'; | ||
export declare function runProgram(clients: { | ||
addCommands: (program: any) => void; | ||
addCommands: (program: program.CommanderStatic) => void; | ||
}[]): void; | ||
export declare function confirm(text?: string, defaultAnswer?: boolean): Promise<boolean>; | ||
export declare function prompt(questions: any[]): Promise<any>; |
@@ -43,5 +43,2 @@ "use strict"; | ||
var client = clients_1[_i]; | ||
if (typeof client.addCommands !== 'function') { | ||
throw new Error('Each client supplied to `runProgram` must implement the `addCommands` function'); | ||
} | ||
client.addCommands(program); | ||
@@ -48,0 +45,0 @@ } |
@@ -5,5 +5,5 @@ import * as pg from 'pg'; | ||
client: pg.Client; | ||
connect(connectionString?: string): Promise<void>; | ||
connect(connectionString?: string): Promise<pg.Client>; | ||
query(queryString: string | QueryArgument, values?: any[]): Promise<any[]>; | ||
count(tableName: string, conditions: QueryPart, extra: string): Promise<{ | ||
count(tableName: string, conditions: QueryPart, extra?: string): Promise<{ | ||
count: string; | ||
@@ -13,3 +13,2 @@ }[]>; | ||
selectOne(tableName: string, conditions?: QueryPart, orderBy?: QueryPart): Promise<any>; | ||
private _query(method, tableName, conditions?, orderBy?, extra?); | ||
insert(tableName: string, changes: QueryPart, primaryKey?: string, onConflict?: OnConflict): Promise<any>; | ||
@@ -25,3 +24,3 @@ update(tableName: string, changes: QueryPart, conditions: QueryPart): Promise<any>; | ||
}): Promise<pg.QueryResult>; | ||
createSequence(name: string): Promise<pg.QueryResult>; | ||
createSequence(name: string): Promise<pg.QueryResult> | undefined; | ||
alterSequenceOwnership(name: string, owner: string, columnName?: string): Promise<pg.QueryResult>; | ||
@@ -35,3 +34,4 @@ truncate(tableName: string): Promise<pg.QueryResult>; | ||
close(): Promise<void>; | ||
private _query(method, tableName, conditions?, orderBy?, extra?); | ||
} | ||
export declare const postgres: Postgres; |
@@ -43,4 +43,14 @@ "use strict"; | ||
Postgres.prototype.connect = function (connectionString) { | ||
this.client = new pg.Client(connectionString); | ||
return this.client.connect(); | ||
return __awaiter(this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: | ||
this.client = new pg.Client(connectionString); | ||
return [4, this.client.connect()]; | ||
case 1: | ||
_a.sent(); | ||
return [2, this.client]; | ||
} | ||
}); | ||
}); | ||
}; | ||
@@ -61,3 +71,4 @@ Postgres.prototype.query = function (queryString, values) { | ||
Postgres.prototype.count = function (tableName, conditions, extra) { | ||
return this._query('SELECT COUNT(*) as count', tableName, conditions, null, extra); | ||
if (extra === void 0) { extra = ''; } | ||
return this._query('SELECT COUNT(*) as count', tableName, conditions, undefined, extra); | ||
}; | ||
@@ -80,27 +91,2 @@ Postgres.prototype.select = function (tableName, conditions, orderBy, extra) { | ||
}; | ||
Postgres.prototype._query = function (method, tableName, conditions, orderBy, extra) { | ||
if (extra === void 0) { extra = ''; } | ||
return __awaiter(this, void 0, void 0, function () { | ||
var values, where, order, result; | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: | ||
values = []; | ||
where = ''; | ||
order = ''; | ||
if (conditions) { | ||
values = Object.values(conditions); | ||
where = "WHERE " + this.toAssignmentFields(conditions).join(' AND '); | ||
} | ||
if (orderBy) { | ||
order = "ORDER BY " + this.getOrderValues(orderBy); | ||
} | ||
return [4, this.client.query(method + " FROM " + tableName + " " + where + " " + order + " " + extra, values)]; | ||
case 1: | ||
result = _a.sent(); | ||
return [2, result.rows]; | ||
} | ||
}); | ||
}); | ||
}; | ||
Postgres.prototype.insert = function (tableName, changes, primaryKey, onConflict) { | ||
@@ -112,12 +98,8 @@ if (primaryKey === void 0) { primaryKey = 'id'; } | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: | ||
if (!changes) { | ||
throw new Error("Tried to perform an insert on " + tableName + " without any values. Supply a changes object"); | ||
} | ||
values = Object.values(changes); | ||
conflictValues = Object.values(onConflict.changes || {}); | ||
return [4, this.client.query("INSERT INTO " + tableName + "(\n " + this.toColumnFields(changes) + "\n ) VALUES(\n " + this.toValuePlaceholders(changes) + "\n )\n " + this.toOnConflictUpsert(onConflict, values.length) + "\n RETURNING " + primaryKey, values.concat(conflictValues))]; | ||
case 1: return [2, _a.sent()]; | ||
if (!changes) { | ||
throw new Error("Tried to perform an insert on " + tableName + " without any values. Supply a changes object"); | ||
} | ||
values = Object.values(changes); | ||
conflictValues = Object.values(onConflict.changes || {}); | ||
return [2, this.client.query("INSERT INTO " + tableName + "(\n " + this.toColumnFields(changes) + "\n ) VALUES(\n " + this.toValuePlaceholders(changes) + "\n )\n " + this.toOnConflictUpsert(onConflict, values.length) + "\n RETURNING " + primaryKey, values.concat(conflictValues))]; | ||
}); | ||
@@ -130,17 +112,13 @@ }); | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: | ||
if (!changes) { | ||
throw new Error("Tried to update " + tableName + " without any values. Supply a changes object"); | ||
} | ||
if (!conditions) { | ||
throw new Error("Tried to update " + tableName + " without a WHERE clause. Supply a conditions object"); | ||
} | ||
changeValues = Object.values(changes); | ||
conditionValues = Object.values(conditions); | ||
whereClauses = this.toAssignmentFields(conditions, changeValues.length); | ||
values = changeValues.concat(conditionValues); | ||
return [4, this.client.query("UPDATE " + tableName + "\n SET " + this.toAssignmentFields(changes) + "\n WHERE " + whereClauses.join(' AND '), values)]; | ||
case 1: return [2, _a.sent()]; | ||
if (!changes) { | ||
throw new Error("Tried to update " + tableName + " without any values. Supply a changes object"); | ||
} | ||
if (!conditions) { | ||
throw new Error("Tried to update " + tableName + " without a WHERE clause. Supply a conditions object"); | ||
} | ||
changeValues = Object.values(changes); | ||
conditionValues = Object.values(conditions); | ||
whereClauses = this.toAssignmentFields(conditions, changeValues.length); | ||
values = changeValues.concat(conditionValues); | ||
return [2, this.client.query("UPDATE " + tableName + "\n SET " + this.toAssignmentFields(changes) + "\n WHERE " + whereClauses.join(' AND '), values)]; | ||
}); | ||
@@ -204,3 +182,3 @@ }); | ||
if (indexStart === void 0) { indexStart = 0; } | ||
return target.length > 0 | ||
return target.length > 0 && changes | ||
? "ON CONFLICT (" + target + ") DO\n UPDATE SET " + this.toAssignmentFields(changes, indexStart) | ||
@@ -229,5 +207,27 @@ : 'ON CONFLICT DO NOTHING'; | ||
return __generator(this, function (_a) { | ||
return [2, this.client.end()]; | ||
}); | ||
}); | ||
}; | ||
Postgres.prototype._query = function (method, tableName, conditions, orderBy, extra) { | ||
if (extra === void 0) { extra = ''; } | ||
return __awaiter(this, void 0, void 0, function () { | ||
var values, where, order, result; | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4, this.client.end()]; | ||
case 1: return [2, _a.sent()]; | ||
case 0: | ||
values = []; | ||
where = ''; | ||
order = ''; | ||
if (conditions) { | ||
values = Object.values(conditions); | ||
where = "WHERE " + this.toAssignmentFields(conditions).join(' AND '); | ||
} | ||
if (orderBy) { | ||
order = "ORDER BY " + this.getOrderValues(orderBy); | ||
} | ||
return [4, this.client.query(method + " FROM " + tableName + " " + where + " " + order + " " + extra, values)]; | ||
case 1: | ||
result = _a.sent(); | ||
return [2, result.rows]; | ||
} | ||
@@ -234,0 +234,0 @@ }); |
@@ -9,2 +9,6 @@ import { clients } from './db'; | ||
static db: Postgres; | ||
attributes: T; | ||
tableName: string; | ||
primaryKey: keyof T; | ||
constructor(attributes?: T); | ||
static setDb(clientName?: keyof typeof clients): void; | ||
@@ -18,9 +22,5 @@ static find<U extends QueryPart = any>(conditions?: Partial<U>, orderBy?: Partial<U>, extra?: string): Promise<U[]>; | ||
static upsert<U extends QueryPart = any>(row: U, onConflict?: OnConflict<U>): Promise<U>; | ||
protected static insert<U extends QueryPart = any>(row: U, onConflict?: OnConflict): Promise<U>; | ||
static update<U extends QueryPart = any, P extends QueryPart = any>(changes: Partial<U>, conditions: Partial<P>): Promise<any>; | ||
static delete<U extends QueryPart = any>(conditions: Partial<U>): Promise<any>; | ||
attributes: T; | ||
tableName: string; | ||
primaryKey: keyof T; | ||
constructor(attributes?: T); | ||
protected static insert<U extends QueryPart = any>(row: U, onConflict?: OnConflict): Promise<U>; | ||
getConstructor(): typeof Model; | ||
@@ -36,6 +36,6 @@ getDefaultQuery(): Partial<T>; | ||
getAll(): T; | ||
getIn(keyPath: string[]): T; | ||
getIn(keyPath: string[]): T | null; | ||
set<K extends keyof T>(key: K, value: T[K]): Model<T>; | ||
setIn(keyPath: string[], value: any): this; | ||
setIn(keyPath: string[], value: any): this | null; | ||
assign(template: Partial<T>): Model<T>; | ||
} |
@@ -43,10 +43,9 @@ "use strict"; | ||
this.tableName = Constructor.tableName; | ||
this.attributes = attributes; | ||
this.primaryKey = Constructor.primaryKey; | ||
if (attributes) { | ||
this.attributes = attributes; | ||
} | ||
} | ||
Model.setDb = function (clientName) { | ||
if (clientName === void 0) { clientName = 'postgres'; } | ||
if (typeof clientName === 'string' && !db_1.clients[clientName]) { | ||
throw new Error("Undefined db client " + db_1.clients); | ||
} | ||
this.db = db_1.clients[clientName]; | ||
@@ -80,6 +79,3 @@ }; | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4, this.db.query(queryString, values)]; | ||
case 1: return [2, _a.sent()]; | ||
} | ||
return [2, this.db.query(queryString, values)]; | ||
}); | ||
@@ -103,5 +99,14 @@ }); | ||
}; | ||
Model.update = function (changes, conditions) { | ||
if (this.withTimestamps) { | ||
changes.updated_at = changes.updated_at || new Date(); | ||
} | ||
return this.db.update(this.tableName, changes, conditions); | ||
}; | ||
Model.delete = function (conditions) { | ||
return this.db.delete(this.tableName, conditions); | ||
}; | ||
Model.insert = function (row, onConflict) { | ||
return __awaiter(this, void 0, void 0, function () { | ||
var createdAt, updatedAt, insertion, newRow; | ||
var createdAt, updatedAt, changes, insertion, newRow; | ||
return __generator(this, function (_a) { | ||
@@ -119,4 +124,5 @@ switch (_a.label) { | ||
if (onConflict) { | ||
onConflict.changes.updated_at = | ||
onConflict.changes.updated_at || updatedAt; | ||
changes = onConflict.changes || {}; | ||
changes.updated_at = changes.updated_at || updatedAt; | ||
onConflict.changes = changes; | ||
} | ||
@@ -136,11 +142,2 @@ } | ||
}; | ||
Model.update = function (changes, conditions) { | ||
if (this.withTimestamps) { | ||
changes.updated_at = changes.updated_at || new Date(); | ||
} | ||
return this.db.update(this.tableName, changes, conditions); | ||
}; | ||
Model.delete = function (conditions) { | ||
return this.db.delete(this.tableName, conditions); | ||
}; | ||
Model.prototype.getConstructor = function () { | ||
@@ -252,3 +249,3 @@ return this.constructor; | ||
}; | ||
Model.tableName = null; | ||
Model.tableName = ''; | ||
Model.primaryKey = 'id'; | ||
@@ -255,0 +252,0 @@ Model.withTimestamps = true; |
/// <reference types="express" /> | ||
import { Request, Response, RequestHandler } from 'express'; | ||
export declare function useRollbar(accessToken: string): void; | ||
export declare function handleRequest(callback: (req: Request, res: Response) => void): RequestHandler; | ||
export declare function handleRequest(callback: (req: Request, res: Response) => Promise<any>): RequestHandler; | ||
export declare function extractFromReq(req: Request, param: string): string; |
@@ -39,3 +39,3 @@ "use strict"; | ||
var Rollbar = require("rollbar"); | ||
var rollbar = null; | ||
var rollbar; | ||
function useRollbar(accessToken) { | ||
@@ -73,3 +73,3 @@ if (!accessToken) | ||
function extractFromReq(req, param) { | ||
var value = null; | ||
var value = ''; | ||
if (req.query[param]) { | ||
@@ -76,0 +76,0 @@ value = req.query[param]; |
{ | ||
"name": "decentraland-server", | ||
"version": "2.0.1", | ||
"version": "2.1.0", | ||
"description": "Set of common functionality accross Decentraland projects servers.", | ||
@@ -9,4 +9,4 @@ "main": "./dist/index.js", | ||
"docs": "npx jsdoc -c conf.json -r src/**/*", | ||
"lint": "tslint -p src/tsconfig.json --fix", | ||
"lint:fix": "tslint -p src/tsconfig.json", | ||
"lint": "tslint -p src/tsconfig.json", | ||
"lint:fix": "tslint -p src/tsconfig.json --fix", | ||
"init": "mkdir -p dist", | ||
@@ -64,2 +64,3 @@ "clean": "(rm -rf dist || true)", | ||
"tslint-language-service": "^0.9.8", | ||
"tslint-plugin-prettier": "^1.3.0", | ||
"typescript": "^2.7.2", | ||
@@ -66,0 +67,0 @@ "validate-commit-msg": "^2.14.0" |
@@ -56,1 +56,3 @@ 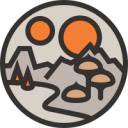 | ||
[`node-hid`](https://github.com/node-hid/node-hid) is a dependency of [`ledgerco`](https://github.com/LedgerHQ/ledgerjs), which in turn is a dependency of [`ledger-wallet-provider`](https://github.com/Neufund/ledger-wallet-provider), used by this lib. | ||
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
58
62252
19
894