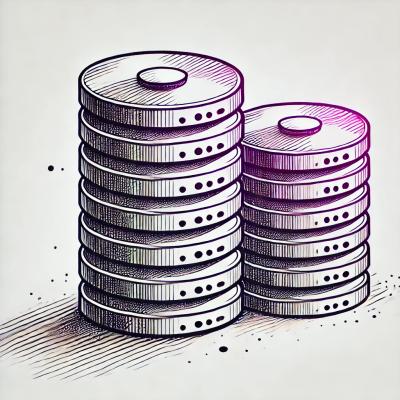
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
decimal-eval
Advanced tools
A tiny, safe, fast JavaScript library for decimal arithmetic expressions.
A tiny, safe, fast JavaScript library for decimal arithmetic expressions.
English | 简体中文
bignumber.js
)# use npm
npm i -S decimal-eval
# or use yarn
yarn add decimal-eval
Supports the four arithmetic operations (+
, -
, *
, /
),
and automatically deal with JavaScript decimal precision by bignumber.js, and supports big number.
import {evaluate} from 'decimal-eval';
evaluate('0.1 + 0.2') // '0.3'
evaluate('100 * (0.08 - 0.01)'); // '7'
evaluate('9007199254740992 + 1'); // '9007199254740993'
evaluate('1 + abc', { abc: 2 }); // '3'
In addition to the above operators, it also supports custom operator expansion, and supports unary operators and binary operators. The operator precedence according to: MDN operator precedence.
import {evaluate, Parser} from 'decimal-eval';
// create binary operator `add`, the precedence is 13
const addOp = Parser.createBinaryOperator('add', 13, (left, right) => {
return String(Number(left) + Number(left));
});
// create unary operator `sin`, the precedence is 16
const sinOp = Parser.createUnaryOperator('sin', 16, (value) => {
return String(Math.sin(value));
});
// install custom operators
Parser.useOperator(addOp);
Parser.useOperator(sinOp);
// same as: `1 + Math.sin(-2)`
evaluate('1 add sin -2') // '0.09070257317431829'
Parse the arithmetic expression and then calculate the result. The name of scope has the following restrictions:
a-z
or A-Z
a-z
、A-Z
、0-9
and _
import {evaluate} from 'decimal-eval';
evaluate('1 + 2'); // '3'
evaluate('1 + abc', { abc: 2 }); // '3'
Parse arithmetic expressions.
import {Parser} from 'decimal-eval';
const node = new Parser('1 + 2').parse();
Compile and cache the arithmetic expression.
import {Parser} from 'decimal-eval';
const evaluate = new Parser('1 + abc').compile();
evaluate({ abc: 2 }); // '3'
evaluate({ abc: 9 }); // '10'
evaluate({ def: 1 }); // throw error, the scope name `abc` is not initialized
Create a binary operator.
Create a unary operator.
Install an operator which created by the Parser.createBinaryOperator()
or Parser.createUnaryOperator()
method.
Set custom calculation adapter methods for four arithmetic (+
, -
, *
, /
).
bignumber.js is used by default.
Parser.useAdapter({
'+': (left, right) => String(Number(left) + Number(right)),
'-': (left, right) => String(Number(left) - Number(right)),
'*': (left, right) => String(Number(left) * Number(right)),
'/': (left, right) => String(Number(left) / Number(right))
})
When using a custom method to deal with the decimal precision problem, you can use a pure package, which can reduce the size by about 60%.
It doesn't include bignumber.js
.
import {evaluate, Parser} from 'decimal-eval/dist/pure';
// set custom calculation adapter
// Parser.useAdapter(adapter);
// Does not deal with precision by default
evaluate('0.1 + 0.2'); // '0.30000000000000004'
// not supports big number by default
evaluate('9007199254740992 + 1'); // '9007199254740992'
bignumber.js
Useful for deal JavaScript decimal precision problem without having to install bignumber.js again.
import {BigNumber} from 'decimal-eval';
const val = new BigNumber(0.1).plus(0.2);
console.log(String(val)); // '0.3'
The operator precedence according to: MDN operator precedence.
operator | precedence |
---|---|
... + ... | 13 |
... - ... | 13 |
... * ... | 14 |
... / ... | 14 |
+ ... | 16 |
- ... | 16 |
FAQs
A tiny, safe, fast JavaScript library for decimal arithmetic expressions.
The npm package decimal-eval receives a total of 1,300 weekly downloads. As such, decimal-eval popularity was classified as popular.
We found that decimal-eval demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.