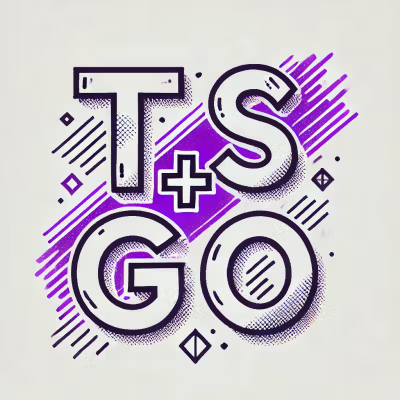
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
default-composer
Advanced tools
A JavaScript library that allows you to set default values for nested objects
A tiny (~500B) JavaScript library that allows you to set default values for nested objects
"default-composer" is a JavaScript library that allows you to set default values for nested objects. The library replaces empty strings/arrays/objects, null, or undefined values in an existing object with the defined default values, which helps simplify programming logic and reduce the amount of code needed to set default values.
Content:
You can install "default-composer" using npm:
npm install default-composer
or with yarn:
yarn add default-composer
To use "default-composer", simply import the library and call the defaultComposer()
function with the default values object and the original object that you want to set default values for. For example:
import { defaultComposer } from "default-composer";
const defaults = {
name: "Aral 😊",
surname: "",
isDeveloper: true,
isDesigner: false,
age: 33,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
},
emails: ["contact@aralroca.com"],
hobbies: ["programming"],
};
const originalObject = {
name: "Aral",
emails: [],
phone: "555555555",
age: null,
address: {
zip: "54321",
},
hobbies: ["parkour", "computer science", "books", "nature"],
};
const result = defaultComposer(defaults, originalObject);
console.log(result);
This will output:
{
name: 'Aral',
surname: '',
isDeveloper: true,
isDesigner: false,
emails: ['contact@aralroca.com'],
phone: '555555555',
age: 33,
address: {
street: '123 Main St',
city: 'Anytown',
state: 'CA',
zip: '54321'
},
hobbies: ['parkour', 'computer science', 'books', 'nature'],
}
defaultComposer
defaultComposer(defaultsPriorityN, [..., defaultsPriority2, defaultsPriority1, objectWithData])
This function takes one or more objects as arguments and returns a new object with default values applied. The first argument should be an object containing the default values to apply. Subsequent arguments should be the objects to apply the default values to.
If a property in a given object is either empty, null, or undefined, and the corresponding property in the defaults object is not empty, null, or undefined, the default value will be used.
Example:
import { defaultComposer } from "default-composer";
const defaultsPriority1 = {
name: "Aral 😊",
hobbies: ["reading"],
};
const defaultsPriority2 = {
name: "Aral 🤔",
age: 33,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
zip: "12345",
},
hobbies: ["reading", "hiking"],
};
const object = {
address: {
street: "",
city: "Anothercity",
state: "NY",
zip: "",
},
hobbies: ["running"],
};
const result = defaultComposer(defaultsPriority2, defaultsPriority1, object);
console.log(result);
This will output:
{
name: 'Aral 😊',
age: 33,
address: {
street: '123 Main St',
city: 'Anothercity',
state: 'NY',
zip: '12345'
},
hobbies: ['running']
}
setConfig
setConfig
is a function that allows you to set configuration options for defaultComposer
.
This is the available configuration:
isDefaultableValue
, is a function that determines whether a value should be considered defaultable or not.mergeArrays
, is a boolean to define if you want to merge arrays (true
) or not (false
), when is set to false
is just replacing to the default value when the original array is empty. By default is false
.isDefaultableValue
You can use setConfig
to provide your own implementation of isDefaultableValue
if you need to customize this behavior.
type IsDefaultableValueParams = ({
key,
value,
defaultableValue,
}: {
key: string;
value: unknown;
defaultableValue: boolean; // In case you want to re-use the default behavior
}) => boolean;
The defaultableValue
boolean is the result of the default behavior of isDefaultableValue
. By default, is detected as defaultableValue
when is null
, undefined
, an empty string
, an empty array
, or an empty object
.
Here is an example of how you can use setConfig
:
import { defaultComposer, setConfig } from "default-composer";
const isNullOrWhitespace = ({ key, value }) => {
return value === null || (typeof value === "string" && value.trim() === "");
};
setConfig({ isDefaultableValue: isNullOrWhitespace });
const defaults = { example: "replaced", anotherExample: "also replaced" };
const originalObject = { example: " ", anotherExample: null };
const result = defaultComposer<any>(defaults, originalObject);
console.log(result); // { example: 'replaced', anotherExample: 'also replaced' }
Here is another example of how you can use setConfig
reusing the defaultableValue
:
import { defaultComposer, setConfig } from "default-composer";
setConfig({
isDefaultableValue({ key, value, defaultableValue }) {
return (
defaultableValue || (typeof value === "string" && value.trim() === "")
);
},
});
const defaults = { example: "replaced", anotherExample: "also replaced" };
const originalObject = { example: " ", anotherExample: null };
const result = defaultComposer<any>(defaults, originalObject);
console.log(result); // { example: 'replaced', anotherExample: 'also replaced' }
mergeArrays
Example to merge arrays:
const defaults = {
hobbies: ["reading"],
};
const object = {
hobbies: ["running"],
};
setConfig({ mergeArrays: true});
defaultComposer<any>(defaults, object)) // { hobbies: ["reading", "running"]}
In order to use in TypeScript you can pass a generic with the expected output, and all the expected input by default should be partials of this generic.
Example:
type Addres = {
street: string;
city: string;
state: string;
zip: string;
};
type User = {
name: string;
age: number;
address: Address;
hobbies: string[];
};
const defaults = {
name: "Aral 😊",
hobbies: ["reading"],
};
const object = {
age: 33,
address: {
street: "",
city: "Anothercity",
state: "NY",
zip: "",
},
hobbies: [],
};
defaultComposer<User>(defaults, object);
Contributions to "default-composer" are welcome! If you find a bug or want to suggest a new feature, please open an issue on the GitHub repository. If you want to contribute code, please fork the repository and submit a pull request with your changes.
"default-composer" is licensed under the MIT license. See LICENSE for more information.
"default-composer" was created by Aral Roca.
Thanks goes to these wonderful people (emoji key):
Aral Roca Gomez 💻 🚧 | Robin Pokorny 💻 | Muslim Idris 💻 | namhtpyn 🚇 | Josu Martinez 🐛 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
A JavaScript library that allows you to set default values for nested objects
The npm package default-composer receives a total of 2,721 weekly downloads. As such, default-composer popularity was classified as popular.
We found that default-composer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.