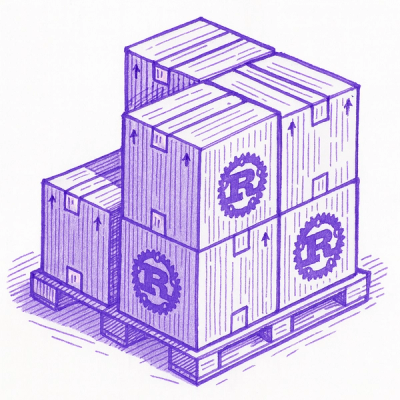
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
detect-port
Advanced tools
The detect-port npm package is a utility for detecting available network ports on a machine. It is useful for scenarios where you need to find an open port to start a server or service.
Detecting an available port
This feature allows you to check if a specific port is available. If the port is occupied, it suggests an alternative available port.
const detect = require('detect-port');
const port = 3000;
detect(port, (err, _port) => {
if (err) {
console.log(err);
}
if (port === _port) {
console.log(`port ${port} was not occupied`);
} else {
console.log(`port ${port} was occupied, try port ${_port}`);
}
});
Promise-based port detection
This feature provides a promise-based approach to detect an available port, making it easier to integrate with modern JavaScript code that uses async/await.
const detect = require('detect-port');
const port = 3000;
detect(port).then(_port => {
if (port === _port) {
console.log(`port ${port} was not occupied`);
} else {
console.log(`port ${port} was occupied, try port ${_port}`);
}
}).catch(err => {
console.log(err);
});
The portfinder package is another utility for finding open ports. It offers similar functionality to detect-port but includes additional features like specifying a range of ports to search within. It is also promise-based and has a more extensive API.
The get-port package is a lightweight utility for finding an available port. It is similar to detect-port but focuses on simplicity and ease of use. It is promise-based and allows specifying a preferred port or a range of ports.
Node.js implementation of port detector
npm i detect-port
const detect = require('detect-port');
/**
* use as a promise
*/
detect(port)
.then(_port => {
if (port == _port) {
console.log(`port: ${port} was not occupied`);
} else {
console.log(`port: ${port} was occupied, try port: ${_port}`);
}
})
.catch(err => {
console.log(err);
});
npm i detect-port -g
# get an available port randomly
$ detect
# detect pointed port
$ detect 80
# output verbose log
$ detect --verbose
# more help
$ detect --help
Most likely network error, check that your /etc/hosts
and make sure the content below:
127.0.0.1 localhost
255.255.255.255 broadcasthost
::1 localhost
Made with contributors-img.
2.0.0 (2024-12-08)
merge from https://github.com/node-modules/detect-port/pull/51
<!-- This is an auto-generated comment: release notes by coderabbit.ai -->New Features
Introduced a new waitPort
function to asynchronously wait for a
specified port to become available.
Bug Fixes
detect-port
package due to issues raised.Documentation
README.md
for improved clarity and updated badge links.CONTRIBUTING.md
to reflect changes in testing commands.Chores
tsconfig.json
).Updated package.json
to reflect changes in dependencies and project
structure.
Tests
Added comprehensive tests for the new waitPort
and updated tests for
the CLI and detectPort
function.
FAQs
Node.js implementation of port detector
The npm package detect-port receives a total of 4,433,502 weekly downloads. As such, detect-port popularity was classified as popular.
We found that detect-port demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.