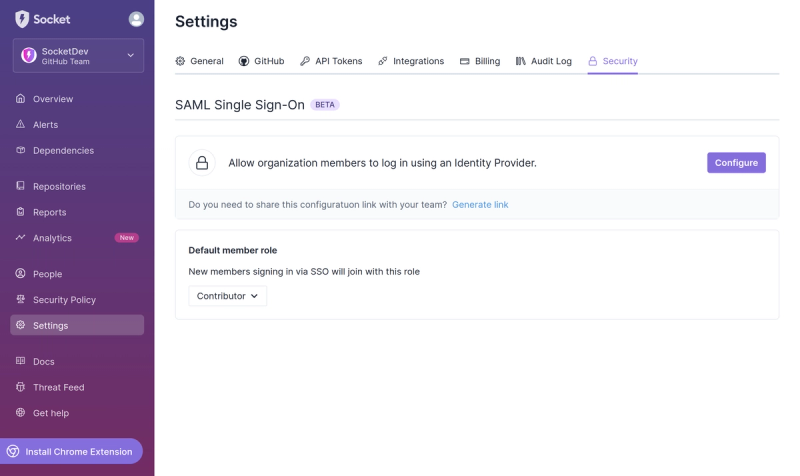
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
device-decoder
Advanced tools
Customizable multithreaded BLE and USB (or other) device connecting, sensor decoding, and (threaded) output rendering
Readme
device-decoder
is a JavaScript library for uniformly and efficiently working with streaming devices on web pages and native apps. It is multithreaded by default with dedicated codec threads, serial threads, and can be used with custom codecs you write following our conventions.
This supports Web Bluetooth API + Native Mobile (via @capacitor-community/bluetooth-le
) and Web Serial API using convenient wrappers.
Note: Android has severe limits with Web Bluetooth (e.g. 20 byte MTU limit, 512 byte limit elsewhere) so the native library is necessary for mobile builds.
device-decoder
is the main package for decoding and rendering data from streaming devices (source)ble-wrapper
wraps @capacitor-community/bluetooth-le with easier handles (source)webserial-wrapper
wraps the Web Serial API with easy handles and buffering + transform stream support (source)device-decoder.third_party
contains wrappers for third party libraries. Includes Muse, Ganglion, and Webgazer (eye tracking) (source). Import Devices
from this to get a complete device list including these extra, much fatter drivers.You can use the device-decoder
library in your project in a few different ways.
If you're using NPM to manage your project, you can install the library with the following command:
npm install device-decoder
Otherwise, it may be more convenient to use a CDN link to import the library directly into your project:
<script src="https://cdn.jsdelivr.net/npm/device-decoder@latest/dist/device.frontend.esm.js"></script>
Regardless of your import source, you may find it useful to use ES Modules to explicitly include variables from the library in your code:
import { Devices, initDevice } from 'device-decoder'
import { Devices, initDevice } from 'https://cdn.jsdelivr.net/npm/device-decoder@latest'
Provide a configuration object from Devices
as the first argument in initDevice
to initialize a multithreaded decoding and rendering pipeline.
let info = initDevice(
Devices['BLE']['hegduino'],
// Optional settings
{
//devices: Devices // A custom device list
//workerUrl: './stream.worker.js' // Specify a custom worker (using the template at the bottom of this documentation file)
//reconnect?:true //for USB connections, if usbVendorId and usbProductId are provided and a previous connection was permitted. For BLE provide the deviceId from info.device.deviceId saved from a previous connection. For USB ports use info.device.port.getInfo();
ondecoded: (data) => console.log(data), //results supplied through codec
ondata?:(data) => console.log(data), //results direct from device, e.g. bypass codec threads (or use both)
onconnect: (deviceInfo) => console.log(deviceInfo),
ondisconnect: (deviceInfo) => console.log(deviceInfo),
filterSettings: {red:{ useLowPass:true, lowpassHz:50 }, infrared:{ useLowpass:true, lowpassHz:50 }} //IIR Filter settings per output key based on the codec results. See FilterSettings type. sample rate is preprogrammed or can be set in each channel setting
}
)
The default Devices
object is organized as follows:
nrf5x
: Connect to our [nRF52 microcontroller prototypes] over BLE.hegduino
: Connect to the HEGduino over BLE.cognixionONE
: Connect to the Cognixion ONE over BLE.statechanger
: Connect to the Statechanger HEG over BLE.blueberry
: Connect to the Blueberry over USB.blueberry2
: Connect to the Blueberry (v2) over USB.heart_rate
: Connect to the generic bluetooth heart rate characteristic (compatible with many devices)freeEEG16
: Connect to Dmitry Sukhoruchkin's FreeEEG16 module (ESP32 based) over BLEnrf5x
: Connect to our [nRF52 microcontroller prototypes] over USB.freeEEG32
: Connect to the FreeEEG32 over USB.freeEEG32_optical
: Connect to the FreeEEG32 over optical USB.freeEEG128
: Connect to the FreeEEG128 over USB.hegduino
: Connect to the HEGduino over USB.cyton
: Connect to the OpenBCI Cyton over USB.cyton_daisy
: Connect to the OpenBCI Cyton + Daisy over USB.peanut
: Connect to the Peanut HEG over USB.statechanger
: Connect to the Statechanger HEG over USB.cognixionONE
: Connect to the Cognixion ONE over USB.freeEEG16
: Connect to Dmitry Sukhoruchkin's FreeEEG16 module (ESP32 based) over USBsimulator
: generates sine waves. You can easily modify this protocol.webgazer
: (via device-decoder.third_party
), webcam-based eye tracking via Webgazer.js!device-decoder.third_party
)muse
: Integrates with the muse-js library.ganglion
: Modifies the ganglion-ble library.Other ideas would be creating media stream drivers to run processes on threads e.g. for audio and video.
These drivers are formatted with simple objects to generalize easily and get the multithreading benefits of device-decoder
.
You can create these simple objects to pipe anything through our threading system!
For BLE devices with multiple notification or read characteristics, you can supply an object to the ondecoded
property in initDevice
.
This allows you to specify callback functions for each characteristic.
You will need to know which characteristics to specify from the device profile.
const device = Devices['BLE']['nrf5x']
const services = device.services
let service = Object.keys(services)[0];
let characteristics = Object.keys(services[service]);
let info = initDevice(
device,
{
ondecoded: {
[characteristics[0]]: (data) => console.log(data),
[characteristics[1]]: (data) => console.log(data)
},
}
)
You may also specify roots
in the config object which will subscribe to all outputs from the stream worker thread to be used with graphscript
formatting. In the eegnfb
example in the GS repo we demonstrated piping multiple workers this way e.g. to run algorithms or build CSV files in IndexedDB.
See docs
This repository is maintained by Joshua Brewster and Garrett Flynn, who use contract work and community contributions through Open Collective to support themselves.
Support us with a monthly donation and help us continue our activities!
Become a sponsor and get your logo here with a link to your site!
[nRF52 microcontroller prototypes]: (https://github.com/brainsatplay/nRF52-Biosensing-Boards
FAQs
Customizable multithreaded BLE and USB (or other) device connecting, sensor decoding, and (threaded) output rendering
The npm package device-decoder receives a total of 1,115 weekly downloads. As such, device-decoder popularity was classified as popular.
We found that device-decoder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.