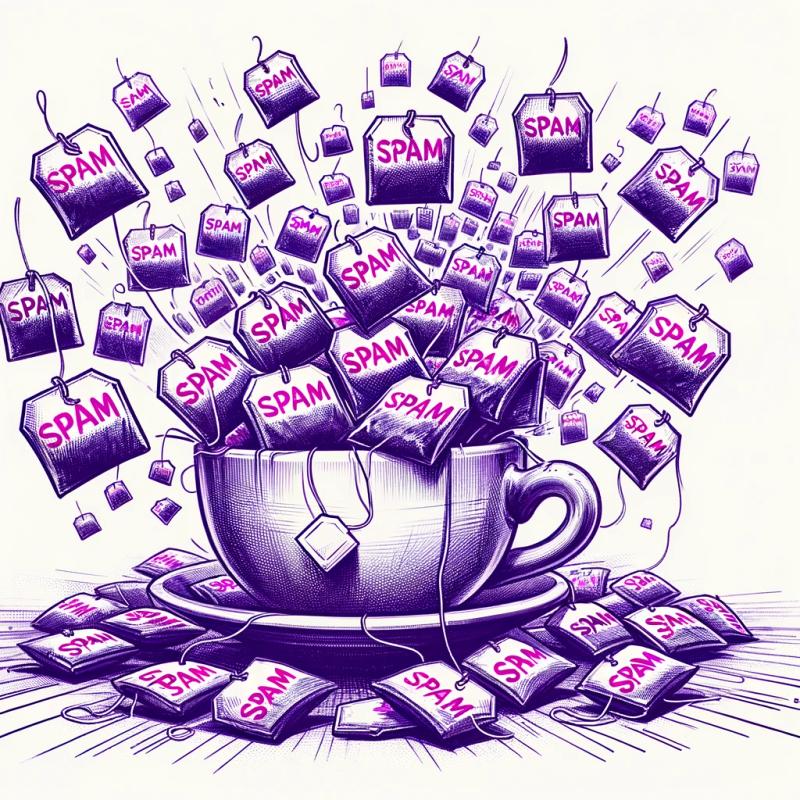
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
draggable-flatlist
Advanced tools
Readme
A drag-and-drop-enabled FlatList component for React Native.
Fully native interactions powered by Reanimated and React Native Gesture Handler.
To use swipeable list items in a DraggableFlatList see React Native Swipeable Item.
MainActivity.java
. Be sure to follow all Android instructions!npm
or yarn
with npm
:
npm install --save react-native-draggable-flatlist
with yarn
:
yarn add react-native-draggable-flatlist
import DraggableFlatList from 'react-native-draggable-flatlist'
All props are spread onto underlying FlatList
Name | Type | Description |
---|---|---|
data | T[] | Items to be rendered. |
horizontal | boolean | Orientation of list. |
renderItem | (params: { item: T, index: number, drag: () => void, isActive: boolean}) => JSX.Element | Call drag when the row should become active (i.e. in an onLongPress or onPressIn ). |
keyExtractor | (item: T, index: number) => string | Unique key for each item |
onDragBegin | (index: number) => void | Called when row becomes active. |
onRelease | (index: number) => void | Called when active row touch ends. |
onDragEnd | (params: { data: T[], from: number, to: number }) => void | Called after animation has completed. Returns updated ordering of data |
autoscrollThreshold | number | Distance from edge of container where list begins to autoscroll when dragging. |
autoscrollSpeed | number | Determines how fast the list autoscrolls. |
onRef | (ref: React.RefObject<DraggableFlatList<T>>) => void | Returns underlying Animated FlatList ref. |
animationConfig | Partial<Animated.SpringConfig> | Configure list animations. See reanimated spring config |
activationDistance | number | Distance a finger must travel before the gesture handler activates. Useful when using a draggable list within a TabNavigator so that the list does not capture navigator gestures. |
layoutInvalidationKey | string | Changing this value forces a remeasure of all item layouts. Useful if item size/ordering updates after initial mount. |
onScrollOffsetChange | (offset: number) => void | Called with scroll offset. Stand-in for onScroll . |
refreshControl | React Component | For iOS you can use standart RefreshControl component. For android you should use custom implementataion. You can left this field undefined, default implementation will be used. Se example for more details. |
refreshing | boolean | Android only! Set this true while waiting for new data from a refresh. |
onRefresh | () => void | Android only! Function that will be called when user pulls down for refresh. |
refreshControlOffset | number | Android only! Padding from top for flatlist container for pull to refresh implementation. |
Name | Type | Description |
:------------------------- | :---------------------------------------------------------------------------------------- | :--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
data | T[] | Items to be rendered. |
horizontal | boolean | Orientation of list. |
renderItem | (params: { item: T, index: number, drag: () => void, isActive: boolean}) => JSX.Element | Call drag when the row should become active (i.e. in an onLongPress or onPressIn ). |
renderPlaceholder | (params: { item: T, index: number }) => React.ReactNode | Component to be rendered underneath the hovering component |
keyExtractor | (item: T, index: number) => string | Unique key for each item |
onDragBegin | (index: number) => void | Called when row becomes active. |
onRelease | (index: number) => void | Called when active row touch ends. |
onDragEnd | (params: { data: T[], from: number, to: number }) => void | Called after animation has completed. Returns updated ordering of data |
autoscrollThreshold | number | Distance from edge of container where list begins to autoscroll when dragging. |
autoscrollSpeed | number | Determines how fast the list autoscrolls. |
onRef | (ref: React.RefObject<DraggableFlatList<T>>) => void | Returns underlying Animated FlatList ref. |
animationConfig | Partial<Animated.SpringConfig> | Configure list animations. See reanimated spring config |
activationDistance | number | Distance a finger must travel before the gesture handler activates. Useful when using a draggable list within a TabNavigator so that the list does not capture navigator gestures. |
layoutInvalidationKey | string | Changing this value forces a remeasure of all item layouts. Useful if item size/ordering updates after initial mount. |
onScrollOffsetChange | (offset: number) => void | Called with scroll offset. Stand-in for onScroll . |
onPlaceholderIndexChange | (index: number) => void | Called when the index of the placeholder changes |
dragItemOverflow | boolean | If true, dragged item follows finger beyond list boundary. |
import React, { Component } from "react";
import { View, TouchableOpacity, Text } from "react-native";
import DraggableFlatList from "react-native-draggable-flatlist";
const exampleData = [...Array(20)].map((d, index) => ({
key: `item-${index}`, // For example only -- don't use index as your key!
label: index,
backgroundColor: `rgb(${Math.floor(Math.random() * 255)}, ${
index * 5
}, ${132})`,
}));
class Example extends Component {
state = {
data: exampleData,
};
renderItem = ({ item, index, drag, isActive }) => {
return (
<TouchableOpacity
style={{
height: 100,
backgroundColor: isActive ? "blue" : item.backgroundColor,
alignItems: "center",
justifyContent: "center",
}}
onLongPress={drag}
>
<Text
style={{
fontWeight: "bold",
color: "white",
fontSize: 32,
}}
>
{item.label}
</Text>
</TouchableOpacity>
);
};
render() {
return (
<View style={{ flex: 1 }}>
<DraggableFlatList
data={this.state.data}
renderItem={this.renderItem}
keyExtractor={(item, index) => `draggable-item-${item.key}`}
onDragEnd={({ data }) => this.setState({ data })}
/>
</View>
);
}
}
export default Example;
import React, { Component } from "react";
import {
View,
TouchableOpacity,
Text,
RefreshControl,
ActivityIndicator,
Platform,
} from "react-native";
import DraggableFlatList from "react-native-draggable-flatlist";
const exampleData = [...Array(20)].map((d, index) => ({
key: `item-${index}`, // For example only -- don't use index as your key!
label: index,
backgroundColor: `rgb(${Math.floor(Math.random() * 255)}, ${
index * 5
}, ${132})`,
}));
class Example extends Component {
state = {
data: exampleData,
refreshing: false,
};
renderItem = ({ item, index, drag, isActive }) => {
return (
<TouchableOpacity
style={{
height: 100,
backgroundColor: isActive ? "blue" : item.backgroundColor,
alignItems: "center",
justifyContent: "center",
}}
onLongPress={drag}
>
<Text
style={{
fontWeight: "bold",
color: "white",
fontSize: 32,
}}
>
{item.label}
</Text>
</TouchableOpacity>
);
};
onRefresh: () => {
//update something
};
render() {
const iOSrefreshControl = (
<RefreshControl
onRefresh={this.onRefresh}
refreshing={this.state.refreshing}
/>
);
const androidRefreshControl = (
<View
style={{
height: 50,
zIndex: -1,
position: "absolute",
left: 0,
right: 0,
top: 0,
}}
>
<View
style={{
flex: 1,
justifyContent: "center",
alignItems: "center",
}}
>
<ActivityIndicator size="large" />
</View>
</View>
);
return (
<View style={{ flex: 1 }}>
<DraggableFlatList
data={this.state.data}
renderItem={this.renderItem}
keyExtractor={(item, index) => `draggable-item-${item.key}`}
onDragEnd={({ data }) => this.setState({ data })}
refreshControl={
Platform.OS === "ios" ? iOSrefreshControl : androidRefreshControl
}
onRefresh={this.onRefresh}
refreshing={this.state.refreshing}
refreshControlOffset={50}
/>
</View>
);
}
}
export default Example;
FAQs
A drag-and-drop-enabled FlatList component for React Native
The npm package draggable-flatlist receives a total of 125 weekly downloads. As such, draggable-flatlist popularity was classified as not popular.
We found that draggable-flatlist demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.