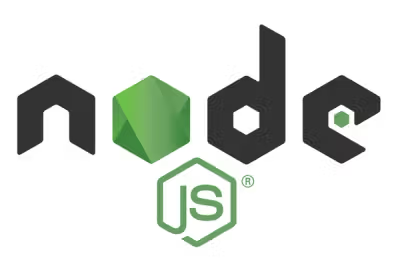
Security News
Node.js TSC Declines to Endorse Feature Bounty Program
The Node.js TSC opted not to endorse a feature bounty program, citing concerns about incentives, governance, and project neutrality.
A comprehensive data structures and algorithms library for JavaScript, featuring optimized implementations of common DS and algorithms.
A Comprehensive Data Structures and Algorithms Library for JavaScript
DSA-Algorithms-JS is a JavaScript library that provides efficient implementations of sorting algorithms, searching algorithms, and graph data structures. Whether you're a beginner learning DSA or an experienced developer working on competitive programming, this package will help you write optimized and well-structured code. It is designed for:
To use this library, first install it using npm:
npm install dsa-master
Then, require the necessary modules in your JavaScript file:
const dsa = require('dsa-master');
const { SearchingAlgorithm, SortingAlgorithm, DataStructure } = dsa;
Sorting Algorithms
This table provides the time and space complexities of common sorting algorithms.
Algorithm | Best Case | Worst Case | Average Case | Space Complexity |
---|---|---|---|---|
Bubble Sort | O(n) | O(n²) | O(n²) | O(1) |
Selection Sort | O(n²) | O(n²) | O(n²) | O(1) |
Insertion Sort | O(n) | O(n²) | O(n²) | O(1) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Quick Sort | O(n log n) | O(n²) | O(n log n) | O(log n) |
const arr1 = [6, 7, -1, 0, 100, 7];
SortingAlgorithm.BubbleSort(arr1);
console.log(arr1); // Output: [-1, 0, 6, 7, 7, 100]
Searching Algorithms
Algorithm | Best Case | Worst Case | Average Case |
---|---|---|---|
Linear Search | O(1) | O(n) | O(n) |
Binary Search | O(1) | O(log n) | O(log n) |
const arr2 = [-1, 0, 6, 7, 9, 100];
const index = SearchingAlgorithm.BinarySearch(arr2, 7);
console.log(index); // Output: 3
Dynamic Array
const DynamicArray = DataStructure.Array;
const array = new DynamicArray();
array.push(10);
array.push(20);
array.display(); // [10, 20]
array.pop();
array.display(); // [10]
Linked List
const LinkedList = DataStructure.LinkedList;
const list = new LinkedList();
list.append(5);
list.append(10);
list.display(); // 5 -> 10 -> null
list.delete(5);
list.display(); // 10 -> null
Stack
const Stack = DataStructure.Stack;
const stack = new Stack();
stack.push(5);
stack.push(10);
stack.display(); // [5, 10]
stack.pop();
stack.display(); // [5]
Queue
const Queue = DataStructure.Queue;
const queue = new Queue();
queue.enqueue(5);
queue.enqueue(10);
queue.display(); // [5, 10]
queue.dequeue();
queue.display(); // [10]
Heap
const Heap = DataStructure.PriorityQueue;
const minHeap = new Heap((a, b) => a < b); // Min Heap
minHeap.insert(10);
minHeap.insert(5);
minHeap.display(); // [5, 10]
minHeap.remove();
minHeap.display(); // [10]
Graph (Adjacency List Representation)
const Graph = dsa.Graph;
const graph = new Graph();
graph.addVertex('A');
graph.addVertex('B');
graph.addEdge('A', 'B');
graph.display();
// A : [ 'B' ]
// B : [ 'A' ]
Feel free to reach out:
FAQs
A comprehensive data structures and algorithms library for JavaScript, featuring optimized implementations of common DS and algorithms.
The npm package dsa-master receives a total of 0 weekly downloads. As such, dsa-master popularity was classified as not popular.
We found that dsa-master demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The Node.js TSC opted not to endorse a feature bounty program, citing concerns about incentives, governance, and project neutrality.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.