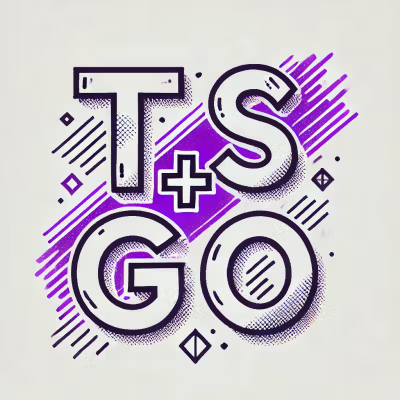
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
dsd-client-conn-lib
Advanced tools
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at [app.dase.io](https:*app.dase.io)
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at app.dase.io
var CONN = require("dsd-client-conn-lib");
var dsdConn = CONN.connClient2;
var dsdCst = CONN.Consts;
//default host for all is "partner.dase.io"
//but you may specify different hosts and ports like that:
//dsdConn.setCoreServer({ host: "partner.dase.io", port: 5090 });
//dsdConn.setDaseServer({ host: "partner.dase.io", port: 5100 });
//dsdConn.setPdsServer({ host: "partner.dase.io", port: 5200 });
//dsdConn.setKeychainServer({ host: "partner.dase.io", port: 5110 });
function log(title, msg) {
console.log(title, msg ? msg : "");
}
log("sendSilver should success");
dsdConn.getCoreClient().sendSilver({
senderId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
senderPassword: "12345678",
silverAmount: 100,
toUserId: "0xb35ef3e31f2944d252eda42801caf5d890d1d7f9"
}).then(
ok => { log("sendSilver ok: ", ok); },
err => { log("sendSilver err: ", err); }
);
log("sendSilver2 should error");
dsdConn.getDaseClient().sendSilver({
senderId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
senderPassword: "12345678",
toUserId: "0xb35ef3e31f2944d252eda42801caf5d890d1d7f9"
}).then(
ok => { log("sendSilver2 ok: ", ok); },
err => {
log("sendSilver2 err: ", err);
//specify error handlers if necessary
if(err.code === dsdCst.errorCodes.WrongParametersCount.id) {
log("I have wrong parameters count..."+dsdCst.errorCodes.WrongParametersCount.name);
//do something
}
else if(err.code === dsdCst.errorCodes.ServerSideError.id) {
log("I have server error..."+ dsdCst.errorCodes.ServerSideError.name);
//do something
}
}
);
console.log("addDab should success");
dsdConn.getPdsClient().addDab({
ownerId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
assetUrl: "http:*localhost",
ownerPassword: "12345678",
publicDescription: "new client lib asset"
}).then(
ok => { log("addDab ok: ", ok); },
err => { log("addDab err: ", err); }
);
(Back)
Keys
Description: Method newUser
Integer
Description: Method getLastBlock
Array_of_Integer
Description: Method getTimestamps
Array_of_Object
Description: Method getBlocks
Integer
Description: Method sendLeos
Integer
Description: Method transfer
Integer
Description: Method silverCostInGold
Integer
Description: Method goldCostInSilver
Integer
Description: Method completeAppCashProductPurchase
Object
Description: Method getAppCashProducts
Integer
Description: Method sendWeis_Ethereum
Object
Description: Method getSystemAccounts
Object
Description: Method getBalances
Integer
Description: Method v_getEligibilityValue
Boolean
Description: Method v_wantVote
Boolean
Description: Method v_supportVoter
Array_of_Address
Description: Method v_getAccountsByCoinbases
Integer
Description: Method v_getVoteCostInSilverLeos
Array_of_Address
Description: Method v_getContractVoters
Array_of_Address
Description: Method v_getQuorumVoters
Array_of_Address
Description: Method v_getCandidatesPool
Boolean
Description: Method v_isInCandidatesPool
Boolean
Description: Method v_hasPledge
Boolean
Description: Method v_isLicensedForVoting
Address
Description: Method getMyCoinbase
Boolean
Description: Method v_withdrawPledge
Boolean
Description: Method v_offerBuyVoting
Boolean
Description: Method offerCurrencyExchangeGoldForWeis
Boolean
Description: Method offerCurrencyExchangeWeisForGold
Boolean
Description: Method cancelCurrencyExchangeOffer
Array_of_Object
Description: Method getCurrencyExchangeOffers
Boolean
Description: Method acceptCurrencyExchangeGoldForWeisOffer
Boolean
Description: Method acceptCurrencyExchangeWeisForGoldOffer
Boolean
Description: Method rejectCurrencyExchangeOffer
Array_of_Integer
Description: Method getCurrencyExchangeOffersIdsToMe
Integer
Description: Method getCurrencyExchangeOffersCountToMe
Array_of_Event
Description: Method getCurrencyExchangeTradeHistory
Boolean
Description: Method offerTokenExchangeGoldForPnft
Boolean
Description: Method offerTokenExchangePnftForGold
Boolean
Description: Method cancelTokenExchangeOffer
Array_of_Object
Description: Method getTokenExchangeOffers
Boolean
Description: Method acceptTokenExchangeGoldForPnftOffer
Boolean
Description: Method acceptTokenExchangePnftForGoldOffer
Boolean
Description: Method rejectTokenExchangeOffer
Array_of_Integer
Description: Method getTokenExchangeOffersIdsToMe
Integer
Description: Method getTokenExchangeOffersCountToMe
Array_of_Event
Description: Method getTokenExchangeTradeHistory
Boolean
Description: Method payPnftRoyalties
Integer
Description: Method getTotalRoyaltyPctgForPnft
Integer
Description: Method getTotalSatEthereumRoyaltyPercent
Array_of_Integer
Description: Method getTotalSatRoyaltyPacks
Integer
Description: Method importFromNft
Boolean
Description: Method exportToNft
Boolean
Description: Method mapUser
Object
Description: Method getMappedUser
Object
Description: Method getPnft
Boolean
Description: Method erc721_safeTransferFrom
Boolean
Description: Method erc721_transferFrom
Boolean
Description: Method erc721_approve
Boolean
Description: Method erc721_setApprovalForAll
Integer
Description: Method erc721_balanceOf
Address
Description: Method erc721_ownerOf
Boolean
Description: Method erc721_isOwnerOf
Address
Description: Method erc721_getApproved
Boolean
Description: Method erc721_isApprovedForAll
Integer
Description: Method erc721_tokenOfOwnerByIndex
Keys
Description: Method newUser
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
credentials | String | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
newUser(1,'12345678');
Response json-data:
{"result":{"userId":"0xa6338a68b941684b5adb7524a06ccc396ebad5a6","pubKey":"0x0535aaa59911be010f44950df20f9b680b90f77f8d6b27fef5615b208dbdc20ca2ab237d56bd5d4aee340192e0f385e8a53d49d82e02b0d5e9dcec5abb845d31","prvKey":"0x43725c1cf6ae8ad9b88d0f49e24e2181c6ac5b9e01d4c00f578ae0909fdc07ea"}}
Example
2nd:
Call (in pseudo-code):
newUser(1,'12345678_Zetonium');
Response json-data:
{"result":{"userId":"0x5387953b5ae9761ba82381cd4bf90c0c2d610836","pubKey":"0x5749e2716c8e1f27f7f566ffef4c3862cc26fd4cfacb136c37a2f7f660b771ec9d7edb4cb0da980ceb9b8aef7bff16e6749be5627da182b79cc953831f7129f2","prvKey":"0xf3bfb49352772533c94e3363a64517e676e8cc802577045061002515114c143c"}}
Example
3th:
Call (in pseudo-code):
newUser(2,'12345678_Ethereum');
Response json-data:
{"result":{"userId":"0xec437de270daa9c9afbc431e8b280da08433c65e","pubKey":"0x8a84cff8fde8a9c2b3e166a846f1002903807238a0dfe78fd2b1837dc3e335b9ba296676e18c2f441a4e011f79d42444bb197c8e4517e5b4b24361e1f828027b","prvKey":"0xd53db5f115befcb00b7d3ea019daf731e2bfa72cfb0918de106664d79ce330ec"}}
Integer
Description: Method getLastBlock
Kind: global function
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
Array_of_Integer
Description: Method getTimestamps
Kind: global function
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
blocksNumbers | Array_of_Integer |
Array_of_Object
Description: Method getBlocks
Kind: global function
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
blocksNumbers | Array_of_Integer |
Integer
Description: Method sendLeos
Kind: global function
Returns: Integer
- newSrcBalance
Param | Type | Description |
---|---|---|
currencyTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
senderId | Address | |
senderCredentials | String | |
amount | Integer | |
toUserId | Address |
Example
1st:
Call (in pseudo-code):
sendLeos(1,'0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','12345678',500000000,'0xa6338a68b941684b5adb7524a06ccc396ebad5a6');
Response json-data:
{"result":"855736620000"}
Example
2nd:
Call (in pseudo-code):
sendLeos(1,'0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','12345678',500000000,'0x9189313f9823231685571d81bc084f35617a9f4c');
Response json-data:
{"result":"855236620000"}
Example
3th:
Call (in pseudo-code):
sendLeos(1,'0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','12345678',500000000,'0x14302f1785fd47583c929cf3684953116cbeaa95');
Response json-data:
{"result":"854736620000"}
Integer
Description: Method transfer
Kind: global function
Returns: Integer
- newSrcBalance
Param | Type | Description |
---|---|---|
srcCurrencyTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
senderId | Address | |
senderCredentials | String | |
srcLeosAmount | Integer |
Integer
Description: Method silverCostInGold
Kind: global function
Param | Type |
---|---|
userId | Address |
silverLeosAmount | Integer |
Integer
Description: Method goldCostInSilver
Kind: global function
Param | Type |
---|---|
userId | Address |
goldLeosAmount | Integer |
Example
1st:
Call (in pseudo-code):
goldCostInSilver('0xe7e0f3cddec426b44589cd1877f0aa392b207c13',1000000);
Response json-data:
{"result":"1000000"}
Integer
Description: Method completeAppCashProductPurchase
Kind: global function
Returns: Integer
- statusCode
Param | Type |
---|---|
userId | Address |
paymentInfo | Object |
engineName | String |
Object
Description: Method getAppCashProducts
Kind: global function
Returns: Object
- products
Param | Type |
---|---|
appId | String |
engineName | String |
Integer
Description: Method sendWeis_Ethereum
Kind: global function
Returns: Integer
- newSrcBalance
Param | Type | Description |
---|---|---|
sender | Address | |
senderCredentials | String | |
receiver | Address | |
weisAmount | Integer | |
databytes | String | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Example
1st:
Call (in pseudo-code):
sendWeis_Ethereum('0x0c46effd09207e466fdf188ab12c352fbe3377ec','12345678','0xe7e0f3cddec426b44589cd1877f0aa392b207c13',100000,null);
Response json-data:
{"result":"9998999999999999400000"}
Object
Description: Method getSystemAccounts
Object
Description: Method getBalances
Kind: global function
Param | Type | Description |
---|---|---|
zetUserId | Address | (optional. default: null) |
ethUserId | Address | (optional. default: null) |
pgnUserId | Address | (optional. default: null) |
flwUserId | Address | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
getBalances('0xa6338a68b941684b5adb7524a06ccc396ebad5a6',null);
Response json-data:
{"result":{"silver_leos":"489000000"}}
Example
2nd:
Call (in pseudo-code):
getBalances('0x9189313f9823231685571d81bc084f35617a9f4c',null);
Response json-data:
{"result":{"silver_leos":"489000000"}}
Example
3th:
Call (in pseudo-code):
getBalances('0x14302f1785fd47583c929cf3684953116cbeaa95',null);
Response json-data:
{"result":{"silver_leos":"489000000"}}
Integer
Description: Method v_getEligibilityValue
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method v_wantVote
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
iWish | Boolean |
Boolean
Description: Method v_supportVoter
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
voterToSupport | Address |
Example
1st:
Call (in pseudo-code):
v_supportVoter('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','12345678','0x082b4e4e0f686a9a249799acea9f05dc2fe83a4a');
Response json-data:
{"result":true}
Array_of_Address
Description: Method v_getAccountsByCoinbases
Kind: global function
Param | Type |
---|---|
coinbases | Array_of_Address |
Integer
Description: Method v_getVoteCostInSilverLeos
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Address
Description: Method v_getContractVoters
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Address
Description: Method v_getQuorumVoters
Array_of_Address
Description: Method v_getCandidatesPool
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method v_isInCandidatesPool
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method v_hasPledge
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method v_isLicensedForVoting
Kind: global function
Param | Type |
---|---|
userId | Address |
Address
Description: Method getMyCoinbase
Boolean
Description: Method v_withdrawPledge
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
Boolean
Description: Method v_offerBuyVoting
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
silverLeosAmount | Integer |
Boolean
Description: Method offerCurrencyExchangeGoldForWeis
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
goldLeosAmount | Integer |
weisAmount | Integer |
Boolean
Description: Method offerCurrencyExchangeWeisForGold
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
goldLeosAmount | Integer |
weisAmount | Integer |
Boolean
Description: Method cancelCurrencyExchangeOffer
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
offerId | Integer |
Array_of_Object
Description: Method getCurrencyExchangeOffers
Kind: global function
Param | Type |
---|---|
userId | Address |
offersIds | Array_of_Integer |
Boolean
Description: Method acceptCurrencyExchangeGoldForWeisOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
goldLeosAmount | Integer |
Boolean
Description: Method acceptCurrencyExchangeWeisForGoldOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
weisAmount | Integer |
Boolean
Description: Method rejectCurrencyExchangeOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
Array_of_Integer
Description: Method getCurrencyExchangeOffersIdsToMe
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method getCurrencyExchangeOffersCountToMe
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Event
Description: Method getCurrencyExchangeTradeHistory
Kind: global function
Returns: Array_of_Event
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userId | Address | |
startBlock | Integer |
Boolean
Description: Method offerTokenExchangeGoldForPnft
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
goldLeosAmount | Integer |
pnftId | Integer |
Boolean
Description: Method offerTokenExchangePnftForGold
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
goldLeosAmount | Integer |
pnftId | Integer |
Boolean
Description: Method cancelTokenExchangeOffer
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
offerId | Integer |
Array_of_Object
Description: Method getTokenExchangeOffers
Kind: global function
Param | Type |
---|---|
userId | Address |
offersIds | Array_of_Integer |
Boolean
Description: Method acceptTokenExchangeGoldForPnftOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
goldLeosAmount | Integer |
Boolean
Description: Method acceptTokenExchangePnftForGoldOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
pnftId | Integer |
Boolean
Description: Method rejectTokenExchangeOffer
Kind: global function
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
offerId | Integer |
Array_of_Integer
Description: Method getTokenExchangeOffersIdsToMe
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method getTokenExchangeOffersCountToMe
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Event
Description: Method getTokenExchangeTradeHistory
Kind: global function
Returns: Array_of_Event
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userId | Address | |
startBlock | Integer |
Boolean
Description: Method payPnftRoyalties
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
pnftId | Integer | |
weisToPay | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Integer
Description: Method getTotalRoyaltyPctgForPnft
Kind: global function
Returns: Integer
- pctg
Param | Type | Description |
---|---|---|
pnftId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
ownerId | Address | (optional. default: null) |
Integer
Description: Method getTotalSatEthereumRoyaltyPercent
Kind: global function
Returns: Integer
- pctg
Param | Type |
---|---|
satId | Integer |
Array_of_Integer
Description: Method getTotalSatRoyaltyPacks
Kind: global function
Returns: Array_of_Integer
- packs
Param | Type |
---|---|
satId | Integer |
Integer
Description: Method importFromNft
Kind: global function
Returns: Integer
- pnftId
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
erc721Addr | String | |
tokenId | Integer | |
pnftId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method exportToNft
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
pnftId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method mapUser
Kind: global function
Param | Type | Description |
---|---|---|
mappedUserId | String | |
mappedUserCredentials | String | |
zetoniumUserId | Address | |
zetoniumUserCredentials | String | |
zetoniumUserPubKey | String | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
isUnmap | Boolean | (optional. default: false) |
Object
Description: Method getMappedUser
Kind: global function
Param | Type | Description |
---|---|---|
mappedUserId | String | (optional. default: null) |
zetoniumUserId | Address | (optional. default: null) |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Object
Description: Method getPnft
Kind: global function
Param | Type | Description |
---|---|---|
pnftId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_safeTransferFrom
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
erc721Addr | String | (optional. default: null) |
from | Address | |
to | Address | |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_transferFrom
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
erc721Addr | String | (optional. default: null) |
from | Address | |
to | Address | |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_approve
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
erc721Addr | String | (optional. default: null) |
approved | Address | |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_setApprovalForAll
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
erc721Addr | String | (optional. default: null) |
operator | Address | |
approved | Boolean | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Integer
Description: Method erc721_balanceOf
Kind: global function
Returns: Integer
- numberOfNfts
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
owner | Address | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Address
Description: Method erc721_ownerOf
Kind: global function
Returns: Address
- owner
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_isOwnerOf
Kind: global function
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
owner | Address | |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Address
Description: Method erc721_getApproved
Kind: global function
Returns: Address
- operator
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
tokenId | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Boolean
Description: Method erc721_isApprovedForAll
Kind: global function
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
owner | Address | |
operator | Address | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Integer
Description: Method erc721_tokenOfOwnerByIndex
Kind: global function
Returns: Integer
- tokenId
Param | Type | Description |
---|---|---|
erc721Addr | String | (optional. default: null) |
owner | Address | |
index | Integer | |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
(Back)
Integer
Description: Method getCountOfSatsForTag
Array_of_Integer
Description: Method getSatsForTag
Object
Description: Method getSatLimitsForDab
Object
Description: Method setSatLimitsForDab
Integer
Description: Method createSat
Boolean
Description: Method linkSat
Integer
Description: Method getCountOfSatsForDab
Integer
Description: Method getCountOfDabsForSat
Array_of_Integer
Description: Method getSatsForDab
Array_of_Integer
Description: Method getDabsForSat
Integer
Description: Method getTotalDealRoyaltyForDab
Integer
Description: Method addAsset
Array_of_Integer
Description: Method addAssetsList
Integer
Description: Method addTag
Array_of_Dab
Description: Method getDirectLabelsForDab
Array_of_Asset
Description: Method getDabs
Array_of_Integer
Description: Method getDabPreviousVersionsIds
Key
Description: Method getTagEncryptedKey
Array_of_Dab
Description: Method exploreLabelsHierarchy
Integer
Description: Method getCountForLicenseAgreements
Integer
Description: Method getCountForLicenseOffers
Integer
Description: Method getCountForTradedDabs
Integer
Description: Method getCountForLabelsHierarchy
Integer
Description: Method getCountForDirectLabelsForDab
Array_of_License
Description: Method getLicenseAgreements
Array_of_License
Description: Method getLicenseOffers
Array_of_Object
Description: Method getTradedDabs
Array_of_Object
Description: Method getTradedDabsIds
Boolean
Description: Method actualiseDeals
Constant_of_Integer
Description: Method marketSellLicenseOffer
Boolean
Description: Method cancelMarketSellLicenseOffer
Boolean
Description: Method areVerifiedParticipants
Constant_of_Integer
Description: Method marketSellRoyaltyLicenseOffer
Constant_of_Integer
Description: Method marketSellSatLinkLicenseOffer
Boolean
Description: Method isRoyaltyRelationAllowed
Boolean
Description: Method appendUserToDealVerifierList
Boolean
Description: Method removeUserFromDealVerifierList
Boolean
Description: Method clearDealVerifierListByUsers
Boolean
Description: Method appendDabIdToDealVerifierList
Boolean
Description: Method removeDabIdFromDealVerifierList
Boolean
Description: Method clearDealVerifierListByDabId
Boolean
Description: Method appendLicenseTypeToDealVerifierList
Boolean
Description: Method removeLicenseTypeFromDealVerifierList
Boolean
Description: Method clearDealVerifierListByLicenseType
Array_of_Address
Description: Method getRestrictionsRange
Array_of_Address
Description: Method getRestrictionsRangeForDabId
Array_of_Address
Description: Method getRestrictionsRangeForLicenseType
Integer
Description: Method getRestrictionsCount
Integer
Description: Method getRestrictionsCountForDabId
Integer
Description: Method getRestrictionsCountForLicenseType
String
Description: Method calcHashToSignBuyOffer
Boolean
Description: Method askForZFeeFunding
Object
Description: Method getZFeeFunding
Object
Description: Method getFundingOffer
Object
Description: Method fundZFeeForContractMethod
Object
Description: Method unfundZFeeForContractMethod
Object
Description: Method funding_createKeyFunding
Boolean
Description: Method funding_deleteKeyFunding
Boolean
Description: Method funding_activateKeyFunding
Array_of_Object
Description: Method funding_getFunderTxHistory
Array_of_Object
Description: Method funding_getFundedTxHistory
Boolean
Description: Method fundSellerToAcceptBuyOffer
Boolean
Description: Method fundBuyerToAcceptSellOffer
Boolean
Description: Method unfundSellerToAcceptBuyOffer
Boolean
Description: Method unfundBuyerToAcceptSellOffer
Constant_of_Integer
Description: Method offerSellCommonLicense
Constant_of_Integer
Description: Method offerBuyCommonLicense
Boolean
Description: Method offerBuyAccessLicenseFromReseller
Constant_of_Integer
Description: Method offerSellExploreLicense
Constant_of_Integer
Description: Method offerSellLabelLicense
Constant_of_Integer
Description: Method offerBuyLabelLicense
Constant_of_Integer
Description: Method offerSellRoyaltyLicense
Constant_of_Integer
Description: Method offerBuyRoyaltyLicense
Constant_of_Integer
Description: Method offerSellSatLinkLicense
Constant_of_Integer
Description: Method offerBuySatLinkLicense
Boolean
Description: Method approveCommonLicenseDeal
Boolean
Description: Method approveLabelLicenseDeal
Boolean
Description: Method approveRoyaltyLicenseDeal
Boolean
Description: Method approveSatLinkLicenseDeal
Object
Description: Method getCustodiansForCommonOffer
Object
Description: Method getCustodiansForLabelOffer
Object
Description: Method getCustodiansForRoyaltyOffer
Object
Description: Method getCustodiansForSatLinkOffer
Integer
Description: Method smart_proposeRelation
Integer
Description: Method smart_destroyRelation
Array_of_Dab
Description: Method smart_getDirectParentsForDab
Integer
Description: Method smart_getCountOfDirectParentsForDab
Array_of_Dab
Description: Method smart_getDirectChildsForDab
Integer
Description: Method smart_getCountOfDirectChildsForDab
Array_of_Dab
Description: Method smart_getAllParentsForDab
Integer
Description: Method smart_getCountOfAllParentsForDab
Array_of_Dab
Description: Method smart_getAllChildsForDab
Integer
Description: Method smart_getCountOfAllChildsForDab
Boolean
Description: Method smart_isHierarchicalChildOf
Boolean
Description: Method smart_isDirectChildOf
Boolean
Description: Method smart_isAllowedRelation
Boolean
Description: Method addRelation
Boolean
Description: Method isHierarchicalChildOf
Boolean
Description: Method cancelCommonLicenseOffer
Boolean
Description: Method cancelLabelLicenseOffer
Boolean
Description: Method cancelRoyaltyLicenseOffer
Boolean
Description: Method cancelSatLinkLicenseOffer
Boolean
Description: Method revokeCommonLicenseDeal
Boolean
Description: Method revokeSatCreateLicenseDeal
Boolean
Description: Method revokeLabelLicenseDeal
Boolean
Description: Method revokeRoyaltyLicenseDeal
Boolean
Description: Method revokeSatLinkLicenseDeal
Integer
Description: Method getLicenseUsageCount
Boolean
Description: Method setPaymentSource
Object
Description: Method getPaymentSource
Boolean
Description: Method fundUser
Boolean
Description: Method unfundUser
Array_of_Object
Description: Method getFunders
Array_of_Address
Description: Method getUsersIFund
Array_of_Object
Description: Method getFunderFundingHistory
Array_of_Object
Description: Method getFunding
Array_of_Object
Description: Method getFunderInfo
Array_of_Object
Description: Method getFundedInfo
Array_of_Object
Description: Method getBalanceHistory
Object
Description: Method transferSync
Object
Description: Method transferIn
Object
Description: Method transferOut
Object
Description: Method getTransferStatus
Array_of_Integer
Description: Method getIncompleteRequestsIds
Object
Description: Method sat_getPaired
Object
Description: Method sat_transferSync
Object
Description: Method sat_transferIn
Object
Description: Method sat_transferOut
Object
Description: Method sat_getTransferStatus
Array_of_Integer
Description: Method sat_getIncompleteRequestsIds
Integer
Description: Method getCountOfSatsForTag
Kind: global function
Returns: Integer
- countOfSats
Param | Type | Description |
---|---|---|
tagId | Integer | |
doFiltration | Boolean | (optional. default: false) |
apiVersionCode | Integer | (optional. default: 0) |
Array_of_Integer
Description: Method getSatsForTag
Kind: global function
Returns: Array_of_Integer
- satIds
Param | Type | Description |
---|---|---|
tagId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
doFiltration | Boolean | (optional. default: false) |
apiVersionCode | Integer | (optional. default: 0) |
Object
Description: Method getSatLimitsForDab
Kind: global function
Param | Type | Description |
---|---|---|
dabId | Integer | |
apiVersionCode | Integer | (optional. default: 0) |
Object
Description: Method setSatLimitsForDab
Kind: global function
Returns: Object
- isOk
Param | Type | Description |
---|---|---|
dabOwnerId | Address | |
dabOwnerCredentials | String | |
dabId | Integer | |
globalCountLimit | Integer | |
apiVersionCode | Integer | (optional. default: 0) |
Integer
Description: Method createSat
Kind: global function
Returns: Integer
- satId
Param | Type | Description |
---|---|---|
satSellerId | Address | |
satSellerCredentials | String | |
dabId | Integer | |
description | String | (optional. default: "Regular SAT") |
dealIndex | Integer | (optional. default: -1) |
apiVersionCode | Integer | (optional. default: 0) |
Boolean
Description: Method linkSat
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
satSellerId | Address | |
satSellerCredentials | String | |
satId | Integer | |
dabIds | Array_of_Integer | |
apiVersionCode | Integer | (optional. default: 0) |
Integer
Description: Method getCountOfSatsForDab
Kind: global function
Returns: Integer
- countOfSats
Param | Type | Description |
---|---|---|
dabId | Integer | |
apiVersionCode | Integer | (optional. default: 0) |
Integer
Description: Method getCountOfDabsForSat
Kind: global function
Returns: Integer
- countOfDabs
Param | Type | Description |
---|---|---|
satId | Integer | |
apiVersionCode | Integer | (optional. default: 0) |
Array_of_Integer
Description: Method getSatsForDab
Kind: global function
Returns: Array_of_Integer
- satIds
Param | Type | Description |
---|---|---|
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
apiVersionCode | Integer | (optional. default: 0) |
Array_of_Integer
Description: Method getDabsForSat
Kind: global function
Returns: Array_of_Integer
- dabIds
Param | Type | Description |
---|---|---|
satId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
apiVersionCode | Integer | (optional. default: 0) |
Integer
Description: Method getTotalDealRoyaltyForDab
Kind: global function
Returns: Integer
- percents
Param | Type |
---|---|
dabId | Integer |
Integer
Description: Method addAsset
Kind: global function
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerCredentials | String | |
assetUrl | String | |
publicDescription | String | |
linkedDabId | Integer | (optional. default: 0) |
custodians | Array_of_Address | (optional. default: []) |
dataHash | String | (optional. default: 0) |
Array_of_Integer
Description: Method addAssetsList
Kind: global function
Param | Type |
---|---|
ownerId | Address |
ownerCredentials | String |
dataArr | Array_of_Object |
Example
1st:
Call (in pseudo-code):
addAssetsList('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678',[{'assetUrl':null,'publicDescription':'dab 01'},{'assetUrl':null,'publicDescription':'dab 02'},{'assetUrl':null,'publicDescription':'dab 03'}]);
Response json-data:
{"result":["639","640","641"]}
Integer
Description: Method addTag
Kind: global function
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerCredentials | String | |
publicDescription | String | |
custodians | Array_of_Address | (optional. default: []) |
title | String | |
encryptedKey | String | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
addTag('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','custody-label/tag/description',[],'custody-label/tag/title',null);
Response json-data:
{"result":"568"}
Example
2nd:
Call (in pseudo-code):
addTag('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','custody-label/tag/description',[],'custody-label/tag/title',null);
Response json-data:
{"result":"569"}
Example
3th:
Call (in pseudo-code):
addTag('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','TAG description',[],'TAG title','TAG encryption key');
Response json-data:
{"result":"576"}
Example
4th:
Call (in pseudo-code):
addTag('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','harmed_passwd_12345678','the tag should be created with correct password',[],'the tag should be created with correct password',null);
Response json-data:
{"result":"Invalid credentials (user's id/password) - account unlock was failed. For method addTag(0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2,harmed_passwd_12345678,the tag should be created with correct password,,the tag should be created with correct password,); "}
Array_of_Dab
Description: Method getDirectLabelsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Example
1st:
Call (in pseudo-code):
getDirectLabelsForDab('0xbf903df49703dd05709a9a3bb542d7229b3049c9','598',0,100);
Response json-data:
{"result":[{"dabId":"597","dabTypeCode":"2","description":"the buyer's tag to mark the seller's dab","ownerId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","custodians":[],"timestamp":"1579640973"}]}
Example
2nd:
Call (in pseudo-code):
getDirectLabelsForDab('0xbf903df49703dd05709a9a3bb542d7229b3049c9','600',0,100);
Response json-data:
{"result":[{"dabId":"599","dabTypeCode":"2","description":"the buyer's tag to mark the seller's dab","ownerId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","custodians":[],"timestamp":"1579640976"}]}
Example
3th:
Call (in pseudo-code):
getDirectLabelsForDab('0xbf903df49703dd05709a9a3bb542d7229b3049c9','602',0,100);
Response json-data:
{"result":[{"dabId":"601","dabTypeCode":"2","description":"the seller's tag to mark the buyer's dab","ownerId":"0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2","custodians":[],"timestamp":"1579640980"}]}
Array_of_Asset
Description: Method getDabs
Kind: global function
Returns: Array_of_Asset
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabIds | Array_of_Integer | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
Example
1st:
Call (in pseudo-code):
getDabs('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2',['575'],null);
Response json-data:
{"result":[{"dabId":"575","dabTypeCode":"1","description":"Data-asset for sell testing","ownerId":"0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2","custodians":[],"timestamp":"1579640642"}]}
Example
2nd:
Call (in pseudo-code):
getDabs('0xa6338a68b941684b5adb7524a06ccc396ebad5a6',['580'],null);
Response json-data:
{"result":[{"dabId":"580","dabTypeCode":"1","description":"Data-asset for sell testing","ownerId":"0xa6338a68b941684b5adb7524a06ccc396ebad5a6","custodians":[],"timestamp":"1579640735"}]}
Example
3th:
Call (in pseudo-code):
getDabs('0x9189313f9823231685571d81bc084f35617a9f4c',['581'],null);
Response json-data:
{"result":[{"dabId":"581","dabTypeCode":"1","description":"Data-asset for sell testing","ownerId":"0x9189313f9823231685571d81bc084f35617a9f4c","custodians":[],"timestamp":"1579640736"}]}
Array_of_Integer
Description: Method getDabPreviousVersionsIds
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
maxLength | Integer | (optional. default: 1) |
Key
Description: Method getTagEncryptedKey
Kind: global function
Returns: Key
- Look at Type description in Docs.
Param | Type |
---|---|
buyerId | Address |
sellerId | Address |
tagId | Integer |
Array_of_Dab
Description: Method exploreLabelsHierarchy
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
rootTagId | Integer | |
tagId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method getCountForLicenseAgreements
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
userId | Address | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
dabId | Integer | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
getCountForLicenseAgreements('0xacb3a424d5e926e7e798b13190a25e11ba41884c',2,1,2,null);
Response json-data:
{"result":"3"}
Integer
Description: Method getCountForLicenseOffers
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
userId | Address | |
offerDirectionTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
dabId | Integer | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
getCountForLicenseOffers('0xacb3a424d5e926e7e798b13190a25e11ba41884c',1,1,1,2,null);
Response json-data:
{"result":"0"}
Example
2nd:
Call (in pseudo-code):
getCountForLicenseOffers('0xacb3a424d5e926e7e798b13190a25e11ba41884c',1,1,1,1,null);
Response json-data:
{"result":"1"}
Integer
Description: Method getCountForTradedDabs
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
userId | Address | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
Example
1st:
Call (in pseudo-code):
getCountForTradedDabs('0xacb3a424d5e926e7e798b13190a25e11ba41884c',1,1,2);
Response json-data:
{"result":"1"}
Integer
Description: Method getCountForLabelsHierarchy
Kind: global function
Returns: Integer
- count
Param | Type |
---|---|
userId | Address |
userCredentials | String |
rootTagId | Integer |
tagId | Integer |
Example
1st:
Call (in pseudo-code):
getCountForLabelsHierarchy('0xacb3a424d5e926e7e798b13190a25e11ba41884c','12345678',1,1);
Response json-data:
{"result":"0"}
Integer
Description: Method getCountForDirectLabelsForDab
Kind: global function
Returns: Integer
- count
Param | Type |
---|---|
userId | Address |
dabId | Integer |
Example
1st:
Call (in pseudo-code):
getCountForDirectLabelsForDab('0xacb3a424d5e926e7e798b13190a25e11ba41884c','673');
Response json-data:
{"result":"0"}
Array_of_License
Description: Method getLicenseAgreements
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
dabId | Integer | (optional. default: null) |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Example
1st:
Call (in pseudo-code):
getLicenseAgreements('0xbf903df49703dd05709a9a3bb542d7229b3049c9',2,null,null,566,0,10);
Response json-data:
{"result":[{"dabId":"566","buyer":"0xBF903dF49703dD05709A9A3bB542D7229b3049c9","seller":"0xB9AC1D430841bC99B6C03E772E6F7d755eFb1dF2","timestamp":"1579640589","relatedDabId":"0","sellerReceivedValue":"14700000","buyerSpentValue":"25000000","dealRevokeValue":"15000000","sellOfferId":"143","buyOfferId":"148","stateBits":"1","totalUsageCount":"0","termSheetData":null,"termSheetTemplate":"licenseUnrestrictedTerms","licenseTypeCode":"2","dabTypeCode":"1"}]}
Example
2nd:
Call (in pseudo-code):
getLicenseAgreements('0xdfe1fcc2d0b7cc479b9b8a78fdbc58083779be40',2,null,610,583,0,10);
Response json-data:
{"result":[{"dabId":"583","buyer":"0xDFE1fCC2D0b7Cc479b9B8a78FdBC58083779bE40","seller":"0x9F97E747eACBDB78CFd422ED407C42fB9E78FFF3","timestamp":"1579640782","relatedDabId":"582","sellerReceivedValue":"98000000","buyerSpentValue":"110000000","dealRevokeValue":"100000000","sellOfferId":"77","buyOfferId":"55","stateBits":"1","totalUsageCount":"0","termSheetData":null,"termSheetTemplate":"licenseUnrestrictedTerms","licenseTypeCode":"610","dabTypeCode":"1"}]}
Example
3th:
Call (in pseudo-code):
getLicenseAgreements('0xef6bf44c7371a2dbcbc0a0c4a45ad7fc281b8195',2,null,2,587,0,10);
Response json-data:
{"result":[{"dabId":"587","buyer":"0xEf6bF44C7371A2DbcBc0A0C4A45ad7Fc281B8195","seller":"0x63Fc4DeC08384A2622a758fAF70Fb4CcbaC28790","timestamp":"1579640891","relatedDabId":"0","sellerReceivedValue":"14700000","buyerSpentValue":"25000000","dealRevokeValue":"15000000","sellOfferId":"153","buyOfferId":"158","stateBits":"1","totalUsageCount":"0","termSheetData":null,"termSheetTemplate":"licenseUnrestrictedTerms","licenseTypeCode":"2","dabTypeCode":"1"}]}
Array_of_License
Description: Method getLicenseOffers
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
offerDirectionTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
dabId | Integer | (optional. default: null) |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Example
1st:
Call (in pseudo-code):
getLicenseOffers('0x9f97e747eacbdb78cfd422ed407c42fb9e78fff3',2,1,null,null,null,0,1);
Response json-data:
{"result":[{"dabId":"583","buyer":"0xDFE1fCC2D0b7Cc479b9B8a78FdBC58083779bE40","termSheetData":null,"timestamp":"1579640779","relatedDabId":"582","seller":"0x9F97E747eACBDB78CFd422ED407C42fB9E78FFF3","termSheetTemplate":"licenseUnrestrictedTerms","sellerMinPrice":"100000000","licenseTypeCode":"610","dabTypeCode":"1"}]}
Example
2nd:
Call (in pseudo-code):
getLicenseOffers('0x0000000000000000000000000000000000000001',1,1,null,null,587,0,10);
Response json-data:
{"result":[{"dabId":"587","buyer":"0x0000000000000000000000000000000000000001","termSheetData":null,"timestamp":"1579640887","relatedDabId":"0","seller":"0x63Fc4DeC08384A2622a758fAF70Fb4CcbaC28790","termSheetTemplate":"licenseUnrestrictedTerms","sellerMinPrice":"15000000","licenseTypeCode":"2","dabTypeCode":"1"}]}
Example
3th:
Call (in pseudo-code):
getLicenseOffers('0x0000000000000000000000000000000000000001',1,1,null,null,588,0,10);
Response json-data:
{"result":[{"dabId":"588","buyer":"0x0000000000000000000000000000000000000001","termSheetData":null,"timestamp":"1579640892","relatedDabId":"0","seller":"0x02Cf3218906B3512cd9Eee3D43c15912a9f3558E","termSheetTemplate":"licenseUnrestrictedTerms","sellerMinPrice":"15000000","licenseTypeCode":"64","dabTypeCode":"1"}]}
Array_of_Object
Description: Method getTradedDabs
Kind: global function
Returns: Array_of_Object
- dabsAndLicensesCodes
Param | Type | Description |
---|---|---|
userId | Address | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_Object
Description: Method getTradedDabsIds
Kind: global function
Returns: Array_of_Object
- dabsIdsAndLicensesCodes
Param | Type | Description |
---|---|---|
userId | Address | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Boolean
Description: Method actualiseDeals
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
tradeTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
Constant_of_Integer
Description: Method marketSellLicenseOffer
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
sellerDabId | Integer | |
buyerDabId | Integer | (optional. default: 0) |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
minLeosPrice | Integer | |
maxSells | Integer | (optional. default: -1) |
ttlInSec | Integer | (optional. default: -1) |
licenseTermSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
licenseTerms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
marketSellLicenseOffer('0x4e31c24881124358301e246ce5df77f42bcc0d99','123',587,0,2,15000000,-1,-1,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"401"}
Example
2nd:
Call (in pseudo-code):
marketSellLicenseOffer('0x63fc4dec08384a2622a758faf70fb4ccbac28790','12345678',587,0,2,15000000,-1,-1,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
3th:
Call (in pseudo-code):
marketSellLicenseOffer('0x63fc4dec08384a2622a758faf70fb4ccbac28790','12345678','587',0,2,15000000,1,-1,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Boolean
Description: Method cancelMarketSellLicenseOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userId | Address | |
userCredentials | String | |
sellerDabId | Integer | |
buyerDabId | Integer | (optional. default: 0) |
Example
1st:
Call (in pseudo-code):
cancelMarketSellLicenseOffer(610,'0x9f97e747eacbdb78cfd422ed407c42fb9e78fff3','12345678',583,0);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
cancelMarketSellLicenseOffer(2,'0x63fc4dec08384a2622a758faf70fb4ccbac28790','12345678',587,0);
Response json-data:
{"result":true}
Boolean
Description: Method areVerifiedParticipants
Kind: global function
Returns: Boolean
- verified
Param | Type | Description |
---|---|---|
sellerId | Address | |
buyerId | Address | |
dabId | Integer | (optional. default: 0) |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
Example
1st:
Call (in pseudo-code):
areVerifiedParticipants('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','0x34b50375e1b7a7588c93873852029563b9ed00f3',0,null);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
areVerifiedParticipants('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','0x5b995cc4482793ff875a62f97112287cd7b9cc72','586',null);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
areVerifiedParticipants('0xc24aca7b98a980d5662a365d1609be436b2ce988','0xd27fdf74f0d03c4a8558b6b6c29367cf0c677e79',0,512);
Response json-data:
{"result":true}
Constant_of_Integer
Description: Method marketSellRoyaltyLicenseOffer
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
sellerDabId | Integer | |
minLeosPrice | Integer | |
dealPctg | Integer | |
usagePctg | Integer | |
maxSells | Integer | (optional. default: -1) |
ttlInSec | Integer | (optional. default: -1) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
marketSellRoyaltyLicenseOffer('0x9f97e747eacbdb78cfd422ed407c42fb9e78fff3','12345678',583,20000000,30,20,-1,-1,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method marketSellSatLinkLicenseOffer
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
sellerDabId | Integer | |
linkLimit | Integer | |
minLeosPrice | Integer | |
dealPctg | Integer | |
usagePctg | Integer | |
buyerDabId | Integer | (optional. default: 0) |
maxSells | Integer | (optional. default: -1) |
ttlInSec | Integer | (optional. default: -1) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Description: Method isRoyaltyRelationAllowed
Kind: global function
Returns: Boolean
- isAllowed
Param | Type |
---|---|
paidDabId | Integer |
beneficiaryDabId | Integer |
Example
1st:
Call (in pseudo-code):
isRoyaltyRelationAllowed(577,580);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
isRoyaltyRelationAllowed(577,581);
Response json-data:
{"result":true}
Boolean
Description: Method appendUserToDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
userRestrictedId | Address |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
appendUserToDealVerifierList('0x5b995cc4482793ff875a62f97112287cd7b9cc72','12345678','0xd27fdf74f0d03c4a8558b6b6c29367cf0c677e79',true,true);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
appendUserToDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','0xd27fdf74f0d03c4a8558b6b6c29367cf0c677e79',true,false);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
appendUserToDealVerifierList('0x7f17359d286eb7f85a1af110f7aaf5cdd4a6d687','12345678','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',false,true);
Response json-data:
{"result":true}
Boolean
Description: Method removeUserFromDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
userRestrictedId | Address |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
removeUserFromDealVerifierList('0x7f17359d286eb7f85a1af110f7aaf5cdd4a6d687','12345678','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',false,true);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
removeUserFromDealVerifierList('0x7f17359d286eb7f85a1af110f7aaf5cdd4a6d687','12345678','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',true,true);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
removeUserFromDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','0xd27fdf74f0d03c4a8558b6b6c29367cf0c677e79',true,false);
Response json-data:
{"result":true}
Boolean
Description: Method clearDealVerifierListByUsers
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
isSeller | Boolean |
isRestricted | Boolean |
Boolean
Description: Method appendDabIdToDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
dabId | Integer |
userRestrictedId | Address |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
appendDabIdToDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',false,true);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
appendDabIdToDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',true,true);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
appendDabIdToDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',false,false);
Response json-data:
{"result":true}
Boolean
Description: Method removeDabIdFromDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
dabId | Integer |
userRestrictedId | Address |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
removeDabIdFromDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',false,true);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
removeDabIdFromDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',true,true);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
removeDabIdFromDealVerifierList('0x46abca4bb6111a77ae95789ff961b230ef23a1e1','12345678','584','0x46abca4bb6111a77ae95789ff961b230ef23a1e1',true,false);
Response json-data:
{"result":true}
Boolean
Description: Method clearDealVerifierListByDabId
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
restrictorId | Address |
restrictorCredentials | String |
dabId | Integer |
isSeller | Boolean |
isRestricted | Boolean |
Boolean
Description: Method appendLicenseTypeToDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
restrictorId | Address | |
restrictorCredentials | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userRestrictedId | Address | |
isSeller | Boolean | |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
appendLicenseTypeToDealVerifierList('0x5b995cc4482793ff875a62f97112287cd7b9cc72','12345678',600,'0x5b995cc4482793ff875a62f97112287cd7b9cc72',false,true);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
appendLicenseTypeToDealVerifierList('0x5b995cc4482793ff875a62f97112287cd7b9cc72','12345678',600,'0x5b995cc4482793ff875a62f97112287cd7b9cc72',true,true);
Response json-data:
{"result":true}
Boolean
Description: Method removeLicenseTypeFromDealVerifierList
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
restrictorId | Address | |
restrictorCredentials | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userRestrictedId | Address | |
isSeller | Boolean | |
isRestricted | Boolean |
Boolean
Description: Method clearDealVerifierListByLicenseType
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
restrictorId | Address | |
restrictorCredentials | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
isSeller | Boolean | |
isRestricted | Boolean |
Array_of_Address
Description: Method getRestrictionsRange
Kind: global function
Returns: Array_of_Address
- restricted
Param | Type | Description |
---|---|---|
restrictorId | Address | |
isSeller | Boolean | |
isRestricted | Boolean | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Example
1st:
Call (in pseudo-code):
getRestrictionsRange('0x7f17359d286eb7f85a1af110f7aaf5cdd4a6d687',false,true,0,10);
Response json-data:
{"result":["0x46AbcA4bb6111a77ae95789FF961B230EF23a1E1"]}
Array_of_Address
Description: Method getRestrictionsRangeForDabId
Kind: global function
Returns: Array_of_Address
- restricted
Param | Type | Description |
---|---|---|
restrictorId | Address | |
dabId | Integer | |
isSeller | Boolean | |
isRestricted | Boolean | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_Address
Description: Method getRestrictionsRangeForLicenseType
Kind: global function
Returns: Array_of_Address
- restricted
Param | Type | Description |
---|---|---|
restrictorId | Address | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
isSeller | Boolean | |
isRestricted | Boolean | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method getRestrictionsCount
Kind: global function
Returns: Integer
- count
Param | Type |
---|---|
restrictorId | Address |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
getRestrictionsCount('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c',false,false);
Response json-data:
{"result":"0"}
Example
2nd:
Call (in pseudo-code):
getRestrictionsCount('0x34b50375e1b7a7588c93873852029563b9ed00f3',false,false);
Response json-data:
{"result":"0"}
Example
3th:
Call (in pseudo-code):
getRestrictionsCount('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c',false,true);
Response json-data:
{"result":"0"}
Integer
Description: Method getRestrictionsCountForDabId
Kind: global function
Returns: Integer
- count
Param | Type |
---|---|
restrictorId | Address |
dabId | Integer |
isSeller | Boolean |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
getRestrictionsCountForDabId('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','586',false,false);
Response json-data:
{"result":"0"}
Example
2nd:
Call (in pseudo-code):
getRestrictionsCountForDabId('0x34b50375e1b7a7588c93873852029563b9ed00f3','586',false,false);
Response json-data:
{"result":"0"}
Example
3th:
Call (in pseudo-code):
getRestrictionsCountForDabId('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','586',false,true);
Response json-data:
{"result":"0"}
Integer
Description: Method getRestrictionsCountForLicenseType
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
restrictorId | Address | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
isSeller | Boolean | |
isRestricted | Boolean |
Example
1st:
Call (in pseudo-code):
getRestrictionsCountForLicenseType('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c',512,false,false);
Response json-data:
{"result":"0"}
Example
2nd:
Call (in pseudo-code):
getRestrictionsCountForLicenseType('0x34b50375e1b7a7588c93873852029563b9ed00f3',512,false,false);
Response json-data:
{"result":"0"}
Example
3th:
Call (in pseudo-code):
getRestrictionsCountForLicenseType('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c',512,false,true);
Response json-data:
{"result":"0"}
String
Description: Method calcHashToSignBuyOffer
Kind: global function
Returns: String
- hash
Param | Type | Description |
---|---|---|
buyerId | Address | |
terms | Object | (optional. default: null) |
Boolean
Description: Method askForZFeeFunding
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
fundingPubK | String |
fundingPrvK | String |
Object
Description: Method getZFeeFunding
Kind: global function
Returns: Object
- zfeeFunding
Param | Type | Description |
---|---|---|
fundingPubK | String | (optional. default: null) |
contractId | Constant_of_Integer | (optional. default: 0) Look at Constants description in Docs. |
contractMethodName | String | (optional. default: null) |
Object
Description: Method getFundingOffer
Kind: global function
Returns: Object
- offerFunding
Param | Type | Description |
---|---|---|
fundingPubK | String | (optional. default: null) |
contractId | Constant_of_Integer | (optional. default: 0) Look at Constants description in Docs. |
contractMethodName | String | (optional. default: null) |
Object
Description: Method fundZFeeForContractMethod
Kind: global function
Returns: Object
- zfeeFunding
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | |
contractId | Constant_of_Integer | Look at Constants description in Docs. |
contractMethodName | String |
Object
Description: Method unfundZFeeForContractMethod
Kind: global function
Returns: Object
- offerFunding
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | |
contractId | Constant_of_Integer | (optional. default: 0) Look at Constants description in Docs. |
contractMethodName | String | (optional. default: null) |
Object
Description: Method funding_createKeyFunding
Kind: global function
Returns: Object
- keyFunding
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Description: Method funding_deleteKeyFunding
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
funderId | Address |
funderCredentials | String |
fundingPubK | String |
Boolean
Description: Method funding_activateKeyFunding
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
fundedId | Address |
fundedCredentials | String |
fundingPubK | String |
fundingPrvK | String |
Array_of_Object
Description: Method funding_getFunderTxHistory
Kind: global function
Returns: Array_of_Object
- fundingUsages
Param | Type | Description |
---|---|---|
funderId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 31536000) |
Array_of_Object
Description: Method funding_getFundedTxHistory
Kind: global function
Returns: Array_of_Object
- fundingUsages
Param | Type | Description |
---|---|---|
fundedId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 31536000) |
Boolean
Description: Method fundSellerToAcceptBuyOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
forBuyOfferId | Integer |
Boolean
Description: Method fundBuyerToAcceptSellOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
forSellOfferId | Integer |
Boolean
Description: Method unfundSellerToAcceptBuyOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | (optional. default: null) |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
forBuyOfferId | Integer |
Boolean
Description: Method unfundBuyerToAcceptSellOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
fundingPubK | String | (optional. default: null) |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
forSellOfferId | Integer |
Constant_of_Integer
Description: Method offerSellCommonLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minLeosPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerSellCommonLicense(2,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','566',15000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerSellCommonLicense(2,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','575',55000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
3th:
Call (in pseudo-code):
offerSellCommonLicense(1,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','576',55000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method offerBuyCommonLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxLeosPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerBuyCommonLicense(2,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','566',15000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerBuyCommonLicense(1,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678',576,55000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
3th:
Call (in pseudo-code):
offerBuyCommonLicense(2,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',575,55000000,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Boolean
Description: Method offerBuyAccessLicenseFromReseller
Kind: global function
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxLeosPrice | Integer | |
resellerId | Address | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerBuyAccessLicenseFromReseller('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',595,5000000,'0xb6cc85fb525c1686831fc1029fb0d96e19c532c9','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerBuyAccessLicenseFromReseller('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',596,5000000,'0xb6cc85fb525c1686831fc1029fb0d96e19c532c9','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method offerSellExploreLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minLeosPrice | Integer | |
buyerPubKeyEncryptedTagKey | String | (optional. default: null) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Constant_of_Integer
Description: Method offerSellLabelLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
minLeosPrice | Integer | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerSellLabelLicense(8,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9',15000000,'568','567','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerSellLabelLicense(16,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9',15000000,'569','570','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"202"}
Example
3th:
Call (in pseudo-code):
offerSellLabelLicense(32,'0x14302f1785fd47583c929cf3684953116cbeaa95','12345678','0xc61481fff926a24145fd446863021b76c47bca12',55000000,577,0,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method offerBuyLabelLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
buyerCredentials | String | |
maxLeosPrice | Integer | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerBuyLabelLicense(8,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',15000000,'568','567','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerBuyLabelLicense(16,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',15000000,'569','570','licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
3th:
Call (in pseudo-code):
offerBuyLabelLicense(32,'0xc61481fff926a24145fd446863021b76c47bca12','12345678',55000000,577,0,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"202"}
Constant_of_Integer
Description: Method offerSellRoyaltyLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
minLeosPrice | Integer | |
sellerDabId | Integer | |
buyerDabId | Integer | |
dealPctg | Integer | |
usagePctg | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerSellRoyaltyLicense('0xa6338a68b941684b5adb7524a06ccc396ebad5a6','12345678','0x14302f1785fd47583c929cf3684953116cbeaa95',100000000,580,577,30,20,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
2nd:
Call (in pseudo-code):
offerSellRoyaltyLicense('0x9189313f9823231685571d81bc084f35617a9f4c','12345678','0xc61481fff926a24145fd446863021b76c47bca12',100000000,581,578,40,20,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Example
3th:
Call (in pseudo-code):
offerSellRoyaltyLicense('0x9189313f9823231685571d81bc084f35617a9f4c','12345678','0x14302f1785fd47583c929cf3684953116cbeaa95',100000000,581,577,100,10,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method offerBuyRoyaltyLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
maxLeosPrice | Integer | |
sellerDabId | Integer | |
buyerDabId | Integer | |
dealPctg | Integer | |
usagePctg | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
offerBuyRoyaltyLicense('0x14302f1785fd47583c929cf3684953116cbeaa95','12345678',100000000,580,577,30,20,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"202"}
Example
2nd:
Call (in pseudo-code):
offerBuyRoyaltyLicense('0xc61481fff926a24145fd446863021b76c47bca12','12345678',100000000,581,578,40,20,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"202"}
Example
3th:
Call (in pseudo-code):
offerBuyRoyaltyLicense('0x14302f1785fd47583c929cf3684953116cbeaa95','12345678',100000000,581,577,100,10,'licenseUnrestrictedTerms',null);
Response json-data:
{"result":"201"}
Constant_of_Integer
Description: Method offerSellSatLinkLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
minLeosPrice | Integer | |
sellerDabId | Integer | |
linkLimit | Integer | |
buyerDabId | Integer | (optional. default: 0) |
dealPctg | Integer | |
usagePctg | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Constant_of_Integer
Description: Method offerBuySatLinkLicense
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
maxLeosPrice | Integer | |
sellerDabId | Integer | |
linkLimit | Integer | |
buyerDabId | Integer | (optional. default: 0) |
dealPctg | Integer | |
usagePctg | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Description: Method approveCommonLicenseDeal
Kind: global function
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
custodianId | Address | |
custodianCredentials | String | |
buyerId | Address | |
dabId | Integer |
Example
1st:
Call (in pseudo-code):
approveCommonLicenseDeal(2,'0xebe59080e76f895899d4258970336ab56bd90b29','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','566');
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
approveCommonLicenseDeal(2,'0xecd36c088f63bd991373566079729c59f1d72060','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','566');
Response json-data:
{"result":true}
Boolean
Description: Method approveLabelLicenseDeal
Kind: global function
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
custodianId | Address | |
custodianCredentials | String | |
buyerId | Address | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
Example
1st:
Call (in pseudo-code):
approveLabelLicenseDeal(8,'0xecd36c088f63bd991373566079729c59f1d72060','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','568','567');
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
approveLabelLicenseDeal(8,'0xebe59080e76f895899d4258970336ab56bd90b29','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','568','567');
Response json-data:
{"result":true}
Boolean
Description: Method approveRoyaltyLicenseDeal
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
sellerDabId | Integer |
buyerDabId | Integer |
Example
1st:
Call (in pseudo-code):
approveRoyaltyLicenseDeal('0xebe59080e76f895899d4258970336ab56bd90b29','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','567','568');
Response json-data:
{"result":true}
Boolean
Description: Method approveSatLinkLicenseDeal
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
sellerDabId | Integer |
Object
Description: Method getCustodiansForCommonOffer
Kind: global function
Returns: Object
- custodiansArrays
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
dabId | Integer |
Example
1st:
Call (in pseudo-code):
getCustodiansForCommonOffer(2,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','566');
Response json-data:
{"result":{"licenseTypeCode":"2","tradeId":"153","got":[],"need":["0xEcD36C088f63BD991373566079729c59F1D72060","0xEbe59080e76f895899D4258970336aB56Bd90B29"]}}
Example
2nd:
Call (in pseudo-code):
getCustodiansForCommonOffer(2,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','567');
Response json-data:
{"result":{"licenseTypeCode":"2","tradeId":"0","got":[],"need":["0xEcD36C088f63BD991373566079729c59F1D72060","0xEbe59080e76f895899D4258970336aB56Bd90B29"]}}
Object
Description: Method getCustodiansForLabelOffer
Kind: global function
Returns: Object
- custodiansArrays
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
Example
1st:
Call (in pseudo-code):
getCustodiansForLabelOffer(8,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','568','567');
Response json-data:
{"result":{"licenseTypeCode":"8","tradeId":"0","got":[],"need":["0xEcD36C088f63BD991373566079729c59F1D72060","0xEbe59080e76f895899D4258970336aB56Bd90B29"]}}
Example
2nd:
Call (in pseudo-code):
getCustodiansForLabelOffer(16,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','569','570');
Response json-data:
{"result":{"licenseTypeCode":"16","tradeId":"24","got":[],"need":[]}}
Example
3th:
Call (in pseudo-code):
getCustodiansForLabelOffer(8,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','569','570');
Response json-data:
{"result":{"licenseTypeCode":"8","tradeId":"0","got":[],"need":["0xEcD36C088f63BD991373566079729c59F1D72060","0xEbe59080e76f895899D4258970336aB56Bd90B29"]}}
Object
Description: Method getCustodiansForRoyaltyOffer
Kind: global function
Returns: Object
- custodiansArrays
Param | Type |
---|---|
buyerId | Address |
sellerDabId | Integer |
buyerDabId | Integer |
Example
1st:
Call (in pseudo-code):
getCustodiansForRoyaltyOffer('0xbf903df49703dd05709a9a3bb542d7229b3049c9','567','568');
Response json-data:
{"result":{"licenseTypeCode":"610","tradeId":"0","got":[],"need":["0xEcD36C088f63BD991373566079729c59F1D72060","0xEbe59080e76f895899D4258970336aB56Bd90B29"]}}
Example
2nd:
Call (in pseudo-code):
getCustodiansForRoyaltyOffer('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','567','568');
Response json-data:
{"result":{"licenseTypeCode":"610","tradeId":"55","got":["0xEbe59080e76f895899D4258970336aB56Bd90B29"],"need":["0xEcD36C088f63BD991373566079729c59F1D72060"]}}
Example
3th:
Call (in pseudo-code):
getCustodiansForRoyaltyOffer('0xdfe1fcc2d0b7cc479b9b8a78fdbc58083779be40',583,582);
Response json-data:
{"result":{"licenseTypeCode":"610","tradeId":"0","got":[],"need":[]}}
Object
Description: Method getCustodiansForSatLinkOffer
Kind: global function
Returns: Object
- custodiansArrays
Param | Type |
---|---|
buyerId | Address |
sellerDabId | Integer |
Integer
Description: Method smart_proposeRelation
Kind: global function
Returns: Integer
- status
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
eitherDabOwnerId | Address | |
eitherDabOwnerCredentials | String | |
parentDabId | Integer | |
childDabId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Integer
Description: Method smart_destroyRelation
Kind: global function
Returns: Integer
- status
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
eitherDabOwnerId | Address | |
eitherDabOwnerCredentials | String | |
parentDabId | Integer | |
childDabId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Array_of_Dab
Description: Method smart_getDirectParentsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Integer
Description: Method smart_getCountOfDirectParentsForDab
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer |
Array_of_Dab
Description: Method smart_getDirectChildsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Integer
Description: Method smart_getCountOfDirectChildsForDab
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer |
Array_of_Dab
Description: Method smart_getAllParentsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Integer
Description: Method smart_getCountOfAllParentsForDab
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer |
Array_of_Dab
Description: Method smart_getAllChildsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Integer
Description: Method smart_getCountOfAllChildsForDab
Kind: global function
Returns: Integer
- count
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
dabId | Integer |
Boolean
Description: Method smart_isHierarchicalChildOf
Kind: global function
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
parentDabId | Integer | |
childDabId | Integer |
Boolean
Description: Method smart_isDirectChildOf
Kind: global function
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
parentDabId | Integer | |
childDabId | Integer |
Boolean
Description: Method smart_isAllowedRelation
Kind: global function
Param | Type | Description |
---|---|---|
relationTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
parentDabId | Integer | |
childDabId | Integer |
Boolean
Description: Method addRelation
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
fromDabId | Integer | |
toDabId | Integer | |
usageArgsTerms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
addRelation('0xc61481fff926a24145fd446863021b76c47bca12','12345678',1,'578','579',null);
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
addRelation('0xc61481fff926a24145fd446863021b76c47bca12','12345678',32,'577','578',null);
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
addRelation('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',8,'597','598',null);
Response json-data:
{"result":true}
Boolean
Description: Method isHierarchicalChildOf
Kind: global function
Param | Type |
---|---|
userId | Address |
parentDabId | Integer |
dabId | Integer |
Boolean
Description: Method cancelCommonLicenseOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userId | Address | |
userCredentials | String | |
buyerId | Address | |
dabId | Integer |
Example
1st:
Call (in pseudo-code):
cancelCommonLicenseOffer(1,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','576');
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
cancelCommonLicenseOffer(2,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','575');
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
cancelCommonLicenseOffer(1,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','621');
Response json-data:
{"result":true}
Boolean
Description: Method cancelLabelLicenseOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
userId | Address | |
userCredentials | String | |
buyerId | Address | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
Boolean
Description: Method cancelRoyaltyLicenseOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
sellerDabId | Integer |
buyerDabId | Integer |
Example
1st:
Call (in pseudo-code):
cancelRoyaltyLicenseOffer('0x9f97e747eacbdb78cfd422ed407c42fb9e78fff3','12345678','0xdfe1fcc2d0b7cc479b9b8a78fdbc58083779be40',583,582);
Response json-data:
{"result":true}
Boolean
Description: Method cancelSatLinkLicenseOffer
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
sellerDabId | Integer |
Boolean
Description: Method revokeCommonLicenseDeal
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer |
Example
1st:
Call (in pseudo-code):
revokeCommonLicenseDeal(2,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','575');
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
revokeCommonLicenseDeal(4,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','626');
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
revokeCommonLicenseDeal(2,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','627');
Response json-data:
{"result":true}
Boolean
Description: Method revokeSatCreateLicenseDeal
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
dealIndex | Integer |
Boolean
Description: Method revokeLabelLicenseDeal
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
tagId | Integer | (optional. default: 0) |
dabId | Integer | (optional. default: 0) |
Example
1st:
Call (in pseudo-code):
revokeLabelLicenseDeal(8,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','629','628');
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
revokeLabelLicenseDeal(8,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','631','630');
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
revokeLabelLicenseDeal(16,'0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9','633','632');
Response json-data:
{"result":true}
Boolean
Description: Method revokeRoyaltyLicenseDeal
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
sellerDabId | Integer |
buyerDabId | Integer |
Example
1st:
Call (in pseudo-code):
revokeRoyaltyLicenseDeal('0x9f97e747eacbdb78cfd422ed407c42fb9e78fff3','12345678','0xdfe1fcc2d0b7cc479b9b8a78fdbc58083779be40',583,582);
Response json-data:
{"result":true}
Boolean
Description: Method revokeSatLinkLicenseDeal
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
sellerDabId | Integer |
Integer
Description: Method getLicenseUsageCount
Kind: global function
Returns: Integer
- newCount
Param | Type | Description |
---|---|---|
userId | Address | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dabId | Integer | |
buyerId | Address |
Example
1st:
Call (in pseudo-code):
getLicenseUsageCount('0xbf903df49703dd05709a9a3bb542d7229b3049c9',2,'649','0xbf903df49703dd05709a9a3bb542d7229b3049c9');
Response json-data:
{"result":"1"}
Example
2nd:
Call (in pseudo-code):
getLicenseUsageCount('0xbf903df49703dd05709a9a3bb542d7229b3049c9',256,'660','0xbf903df49703dd05709a9a3bb542d7229b3049c9');
Response json-data:
{"result":"0"}
Example
3th:
Call (in pseudo-code):
getLicenseUsageCount('0xbf903df49703dd05709a9a3bb542d7229b3049c9',256,'658','0xbf903df49703dd05709a9a3bb542d7229b3049c9');
Response json-data:
{"result":"0"}
Boolean
Description: Method setPaymentSource
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
userId | Address | |
credentials | String | |
termSheetTemplate | Constant_of_String | (optional. default: "selfPaymentTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
setPaymentSource('0x75d64f67fc606a882d395bb37143258bca57f26d','12345678','externalPaymentTerms',null);
Response json-data:
{"result":true}
Object
Description: Method getPaymentSource
Kind: global function
Returns: Object
- source
Param | Type |
---|---|
userId | Address |
Example
1st:
Call (in pseudo-code):
getPaymentSource('0x75d64f67fc606a882d395bb37143258bca57f26d');
Response json-data:
{"result":{"termSheetTemplate":"selfPaymentTerms","terms":null}}
Boolean
Description: Method fundUser
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
funderId | Address | |
funderCredentials | String | |
userToFund | Address | |
termSheetTemplate | Constant_of_String | (optional. default: "fundingTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Example
1st:
Call (in pseudo-code):
fundUser('0x0709833ca65d49c0ece401b2759b58f2e6b8fe37','12345678','0x75d64f67fc606a882d395bb37143258bca57f26d','fundingTerms',{'totalLeos':12000000});
Response json-data:
{"result":true}
Example
2nd:
Call (in pseudo-code):
fundUser('0x0709833ca65d49c0ece401b2759b58f2e6b8fe37','12345678','0x75d64f67fc606a882d395bb37143258bca57f26d','fundingTerms',{'totalLeos':22000000,'leosPerTx':11000000,'ttl':60});
Response json-data:
{"result":true}
Example
3th:
Call (in pseudo-code):
fundUser('0x0b8bbf228470d8c5d9d73a9294cb93d71c976110','12345678','0x75d64f67fc606a882d395bb37143258bca57f26d','fundingTerms',{'totalLeos':1000000});
Response json-data:
{"result":true}
Boolean
Description: Method unfundUser
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
funderId | Address |
funderCredentials | String |
userToUnfund | Address |
Example
1st:
Call (in pseudo-code):
unfundUser('0x0709833ca65d49c0ece401b2759b58f2e6b8fe37','12345678','0x75d64f67fc606a882d395bb37143258bca57f26d');
Response json-data:
{"result":true}
Array_of_Object
Description: Method getFunders
Kind: global function
Returns: Array_of_Object
- funders
Param | Type |
---|---|
userId | Address |
Example
1st:
Call (in pseudo-code):
getFunders('0x75d64f67fc606a882d395bb37143258bca57f26d');
Response json-data:
{"result":[{"funderId":"0x0709833Ca65d49C0ece401b2759B58f2e6b8FE37","termSheetTemplate":"fundingTerms","terms":{"totalLeos":"12000001","leosPerTx":"0","lastBlockSec":"0"},"isActualTerms":true}]}
Array_of_Address
Description: Method getUsersIFund
Kind: global function
Returns: Array_of_Address
- usersIds
Param | Type | Description |
---|---|---|
funderId | Address | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 31536000) |
Array_of_Object
Description: Method getFunderFundingHistory
Kind: global function
Returns: Array_of_Object
- fundings
Param | Type | Description |
---|---|---|
funderId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
fromTimestampInSec | Integer | (optional. default: 0) |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Object
Description: Method getFunding
Kind: global function
Returns: Array_of_Object
- fundings
Param | Type | Description |
---|---|---|
funderId | Address | |
userId | Address | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Object
Description: Method getFunderInfo
Kind: global function
Returns: Array_of_Object
- funderInfo
Param | Type | Description |
---|---|---|
funderId | Address | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Object
Description: Method getFundedInfo
Kind: global function
Returns: Array_of_Object
- fundedInfo
Param | Type | Description |
---|---|---|
userId | Address | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Object
Description: Method getBalanceHistory
Kind: global function
Returns: Array_of_Object
- transfers
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
fromTimestampInSec | Integer | (optional. default: 0) |
timeAmountInSec | Integer | (optional. default: 2678400) |
isFlowIn | Boolean | (optional. default: false) |
Object
Description: Method transferSync
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
srcUserCredentials | String | |
dstUserId | Address | |
dstUserCredentials | String | |
srcLeosAmount | Integer |
Object
Description: Method transferIn
Kind: global function
Param | Type | Description |
---|---|---|
dstBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcReqId | Integer | |
dstUserCredentials | String |
Object
Description: Method transferOut
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
srcUserCredentials | String | |
dstUserId | Address | |
srcLeosAmount | Integer |
Example
1st:
Call (in pseudo-code):
transferOut(1,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','0xe7e0f3cddec426b44589cd1877f0aa392b207c13',10000000);
Response json-data:
{"result":"7612000000"}
Example
2nd:
Call (in pseudo-code):
transferOut(1,'0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','0xe7e0f3cddec426b44589cd1877f0aa392b207c13',20000000);
Response json-data:
{"result":"7522700000"}
Example
3th:
Call (in pseudo-code):
transferOut(2,'0xe7e0f3cddec426b44589cd1877f0aa392b207c13','12345678','0xbf903df49703dd05709a9a3bb542d7229b3049c9',5000000);
Response json-data:
{"result":"124989500"}
Object
Description: Method getTransferStatus
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcReqId | Integer | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Integer
Description: Method getIncompleteRequestsIds
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
fromTimeInSec | Integer | |
timeAmountInSec | Integer |
Object
Description: Method sat_getPaired
Kind: global function
Returns: Object
- pair
Param | Type | Description |
---|---|---|
satId | Integer | (optional. default: null) |
nftId | Integer | (optional. default: null) |
erc721Addr | String | (optional. default: null) |
bchainTypeCode | Constant_of_Integer | (optional. default: 2) Look at Constants description in Docs. |
Object
Description: Method sat_transferSync
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dstBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
srcUserCredentials | String | |
dstUserId | Address | |
dstUserCredentials | String | |
srcNftId | Integer |
Object
Description: Method sat_transferIn
Kind: global function
Param | Type | Description |
---|---|---|
dstBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcReqId | String | |
dstUserId | Address | |
dstUserCredentials | String |
Object
Description: Method sat_transferOut
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
srcUserCredentials | String | |
dstUserId | Address | |
srcNftId | Integer |
Object
Description: Method sat_getTransferStatus
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dstBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcReqId | String | |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 2678400) |
Array_of_Integer
Description: Method sat_getIncompleteRequestsIds
Kind: global function
Param | Type | Description |
---|---|---|
srcBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
dstBlockchainTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
srcUserId | Address | |
fromTimeInSec | Integer | |
timeAmountInSec | Integer |
(Back)
Array_of_Integer
Description: Method addAssetsList
Boolean
Description: Method basket_putDab
Boolean
Description: Method basket_extractDab
Integer
Description: Method basket_rootDab
Integer
Description: Method getTotalBasketSellRoyaltyForDab
Integer
Description: Method getTotalPnftTransferRoyaltyForDab
Dab
Description: Method basket_getDirectParentForDab
Array_of_Dab
Description: Method basket_getDirectChildsForDab
Integer
Description: Method basket_getCountOfDirectChildsForDab
Boolean
Description: Method basket_isDirectChildOf
Boolean
Description: Method basket_isHierarchicalChildOf
Boolean
Description: Method basket_isAllowedRelation
Integer
Description: Method useBulkLicense
Array_of_Integer
Description: Method useLicenses
Array_of_Integer
Description: Method useAISearchLicenses
Array_of_Integer
Description: Method useReadOnlyLicenses
String
Description: Method getPdsPublicKey
Object
Description: Method getSystemInfo
Ticket
Description: Method getUploadTicket
Array_of_Object
Description: Method getLicenseUsageHistory
Array_of_Object
Description: Method getDealsHistory
Ticket
Description: Method getAccessTicketSecured
Ticket
Description: Method getAccessTickets
Ticket
Description: Method getAISearchAccessTickets
Ticket
Description: Method getReadOnlyAccessTickets
Ticket
Description: Method getBulkAccessTicket
Ticket
Description: Method getSatAccessTicket
Ticket
Description: Method getCopyTicket
Dab
Description: Method getAccessDab
Dab
Description: Method getBulkAccessDab
String
Description: Method encrypt
Boolean
Description: Method setUsp
String
Description: Method getUsp
Boolean
Description: Method setAak
String
Description: Method getAak
Array_of_Integer
Description: Method getVaultsIds
Boolean
Description: Method isVaultedDab
Object
Description: Method getVaultById
Object
Description: Method getVaultManifestById
Array_of_Object
Description: Method getVaultManifests
Integer
Description: Method setVaultManifest
Array_of_Integer
Description: Method addAssetsList
Kind: global function
Param | Type |
---|---|
ownerId | Address |
ownerCredentials | String |
dataArr | Array_of_Object |
Example
1st:
Call (in pseudo-code):
addAssetsList('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','12345678',[{'assetUrl':null,'publicDescription':'dab 01'},{'assetUrl':null,'publicDescription':'dab 02'},{'assetUrl':null,'publicDescription':'dab 03'}]);
Response json-data:
{"result":["639","640","641"]}
Boolean
Description: Method basket_putDab
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
ownerId | Address |
ownerCredentials | String |
rootDabId | Integer |
itemDabId | Integer |
Boolean
Description: Method basket_extractDab
Kind: global function
Returns: Boolean
- isOk
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerCredentials | String | |
rootDabId | Integer | (optional. default: 0) |
itemDabId | Integer |
Integer
Description: Method basket_rootDab
Kind: global function
Returns: Integer
- rootDabId
Param | Type |
---|---|
dabId | Integer |
Integer
Description: Method getTotalBasketSellRoyaltyForDab
Kind: global function
Returns: Integer
- price
Param | Type |
---|---|
dabId | Integer |
Integer
Description: Method getTotalPnftTransferRoyaltyForDab
Kind: global function
Returns: Integer
- price
Param | Type |
---|---|
dabId | Integer |
Dab
Description: Method basket_getDirectParentForDab
Kind: global function
Returns: Dab
- Look at Type description in Docs.
Param | Type |
---|---|
dabId | Integer |
Array_of_Dab
Description: Method basket_getDirectChildsForDab
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Integer
Description: Method basket_getCountOfDirectChildsForDab
Kind: global function
Returns: Integer
- count
Param | Type |
---|---|
dabId | Integer |
Boolean
Description: Method basket_isDirectChildOf
Kind: global function
Param | Type |
---|---|
parentDabId | Integer |
childDabId | Integer |
Boolean
Description: Method basket_isHierarchicalChildOf
Kind: global function
Param | Type |
---|---|
parentDabId | Integer |
childDabId | Integer |
Boolean
Description: Method basket_isAllowedRelation
Kind: global function
Param | Type |
---|---|
parentDabId | Integer |
childDabId | Integer |
Integer
Description: Method useBulkLicense
Kind: global function
Returns: Integer
- newUsageCount
Param | Type |
---|---|
buyerId | Address |
buyerCredentials | String |
dabId | Integer |
tagId | Integer |
bulkSellerId | Address |
Example
1st:
Call (in pseudo-code):
useBulkLicense('0xa6338a68b941684b5adb7524a06ccc396ebad5a6','12345678','579',578,'0xc61481fff926a24145fd446863021b76c47bca12');
Response json-data:
{"result":"1"}
Example
2nd:
Call (in pseudo-code):
useBulkLicense('0x9189313f9823231685571d81bc084f35617a9f4c','12345678','578',577,'0x14302f1785fd47583c929cf3684953116cbeaa95');
Response json-data:
{"result":"1"}
Example
3th:
Call (in pseudo-code):
useBulkLicense('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678','659','660','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2');
Response json-data:
{"result":"1"}
Array_of_Integer
Description: Method useLicenses
Kind: global function
Returns: Array_of_Integer
- newUsageCounts
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabIds | Array_of_Integer | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
termsArray | Array_of_Object | (optional. default: []) |
Example
1st:
Call (in pseudo-code):
useLicenses('0xbf903df49703dd05709a9a3bb542d7229b3049c9','12345678',['650','651'],2,[]);
Response json-data:
{"result":["1","1"]}
Array_of_Integer
Description: Method useAISearchLicenses
Kind: global function
Returns: Array_of_Integer
- newUsageCounts
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabIds | Array_of_Integer | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
termsArray | Array_of_Object | (optional. default: []) |
Array_of_Integer
Description: Method useReadOnlyLicenses
Kind: global function
Returns: Array_of_Integer
- newUsageCounts
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabIds | Array_of_Integer | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
termsArray | Array_of_Object | (optional. default: []) |
String
Description: Method getPdsPublicKey
Kind: global function
Returns: String
- pubKey
Example
1st:
Call (in pseudo-code):
getPdsPublicKey();
Response json-data:
{"result":"0x533cd169d224435cf9ae352736257a033ce591f8629d294800c3cc7960ac010f784082f236db170372811231de9a8738abf9e94ecfb9ce099bb0c2bda7a4d273"}
Object
Description: Method getSystemInfo
Kind: global function
Returns: Object
- systemInfo
Example
1st:
Call (in pseudo-code):
getSystemInfo();
Response json-data:
{"result":{"pdsPublicKey":"0x533cd169d224435cf9ae352736257a033ce591f8629d294800c3cc7960ac010f784082f236db170372811231de9a8738abf9e94ecfb9ce099bb0c2bda7a4d273","systemAccounts":{"edzLabs":"0x003e8c791cb39b4cad756b9c25431d6eb3fed85c","treasury":"0x003e8c791cb39b4cad756b9c25431d6eb3fed85c","treasuryPublic":"0x0709833ca65d49c0ece401b2759b58f2e6b8fe37","reactionsTreasury":"0x003e8c791cb39b4cad756b9c25431d6eb3fed85c"},"feesFor1000leos":{"zFee":"1000000","sendMoneyFee":"30","transferOutFee":"30","tradeSellerGets":"980","tradeBuyerSpends":"10001000","depositFee":"10000000"}}}
Ticket
Description: Method getUploadTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
userPubKey | String | (optional. default: null) |
vaultId | Integer | (optional. default: 1) |
Example
1st:
Call (in pseudo-code):
getUploadTicket('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','0x04da11059055b2b4f12f6e89f330308ed1289b3c1f7987830ab8f9c013fc9831849f291b9f2dcf92b549bf8e7baa2f1c8a8b0a90210ae931398cbdd2ce90364d52','1');
Response json-data:
{"result":{"id":"tid-637166.232378","type":"create","creationTimeInMs":"1579641133138","timeToLiveInMs":"1200000","userId":"0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2","assetUrl":null,"vaultUrl":"https://partner.dase.io:5081","vaultAuth":"ed1aef1da30d1537a841eef97c3e928124cc5a6bd5e45bb138ec7ff42c713ab65f07a6a32d18ab62abbf92a0c4e47c6cc55e52b94377f46d172e1333c2078e9f880780294b47ac071772666037d04c5224006a494cee95ad386f363a0a097fc991c7adf4","nonce":"n-575368","checksum":null,"pubKey":"0x04da11059055b2b4f12f6e89f330308ed1289b3c1f7987830ab8f9c013fc9831849f291b9f2dcf92b549bf8e7baa2f1c8a8b0a90210ae931398cbdd2ce90364d52","signature":"3044022027adda872c1fef13d8ec5c28d77ca33100f8221a558f06b0ecc53e34e577eac5022063775fbc3d8565402c262dc3e298e14dc541b7d3bb2b3f9fdffd66138e537ca0"}}
Array_of_Object
Description: Method getLicenseUsageHistory
Kind: global function
Returns: Array_of_Object
- usages
Param | Type | Description |
---|---|---|
userId | Address | (optional. default: null) |
dabId | Integer | (optional. default: null) |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 31536000) |
Array_of_Object
Description: Method getDealsHistory
Kind: global function
Returns: Array_of_Object
- deals
Param | Type | Description |
---|---|---|
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
fromTimestampInSec | Integer | |
timeAmountInSec | Integer | (optional. default: 31536000) |
Ticket
Description: Method getAccessTicketSecured
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dealMessageId | Integer | |
buyerId | Address | |
sellerId | Address | |
argsBuyerSignature | String | |
publicKey | String | |
vaultAuth | Object | (optional. default: null) |
Ticket
Description: Method getAccessTickets
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
dabIds | Array_of_Integer |
buyerId | Address |
sellerIds | Array_of_Address |
argsBuyerSignature | String |
publicKey | String |
Example
1st:
Call (in pseudo-code):
getAccessTickets(['593'],'0xbf903df49703dd05709a9a3bb542d7229b3049c9',['0xbf903df49703dd05709a9a3bb542d7229b3049c9'],'30460221009d2be0357e6a0f1394ecd093c7dece1d129c50d62d45c2bab2a2facfe9ea6248022100ddc14f2151eb6a121ea91c36a812b064a52f63123962b9618e9601f17376ec14','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":[{"id":"tid-140775.248061","type":"read","creationTimeInMs":"1579640939399","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-345068","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"304502210085ecdad2a070a5ba8a197264bb34dcdd146d9448701697cecdf13c63ae9fbf9502201205cb07e0483beffc73f1881a344de23d16f4fb56212010882ba6d52645d436"}]}
Example
2nd:
Call (in pseudo-code):
getAccessTickets(['595'],'0xbf903df49703dd05709a9a3bb542d7229b3049c9',['0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2'],'304502205cf66be072c9ad217fae84b4044d1cab9d00c3c82a66702f215631e2c5f60fcf022100848ba6fb64eb8084af2bbc31d62781ba6ee4eb9c27c1372d50869e8dfbcc99c2','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":[{"id":"tid-707711.72273","type":"read","creationTimeInMs":"1579640967674","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-874048","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"3044022043eee7e0b07a934d335f009350c9ceddde52911923e92a9c1ea6354bbded86c8022064bbf7decc8dfee217ba7391b21e12ef6f0e93406a56007291b839ac91a56491"}]}
Example
3th:
Call (in pseudo-code):
getAccessTickets(['644'],'0xbf903df49703dd05709a9a3bb542d7229b3049c9',['0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2'],'3046022100fafc4690045eaff32487170d2d2eb17fd8ed0882b5d494f3a83c1dbd2cbfdd81022100f275efbcc2a28b208e2a44516f42c681fdc694315947c3eb20d47c5697a83584','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":[{"id":"tid-790281.203262","type":"read","creationTimeInMs":"1579641100862","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-822299","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"3045022100b4fdca6b8cd8f7488a032556bce09411764af657f79509c2b5fbf843d8a932ef022023e4ba3f56ddb5c47eb668f64beedb1d419d2a0a3f0b30b23217813805ce175c"}]}
Example
4th:
Call (in pseudo-code):
getAccessTickets(['648'],'0xbf903df49703dd05709a9a3bb542d7229b3049c9',['0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2'],'30460221009521d160b62c88039259956abb9f794c9e013404f4956ec840e1c0df6b4dc7a0022100ea1651607e5376481587a01a418176dcd36f33a4ef186c7f33b57297dab3daf0','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":"Invalid request (wrong method or parameters). For method getAccessTickets(648,0xbf903df49703dd05709a9a3bb542d7229b3049c9,0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2,30460221009521d160b62c88039259956abb9f794c9e013404f4956ec840e1c0df6b4dc7a0022100ea1651607e5376481587a01a418176dcd36f33a4ef186c7f33b57297dab3daf0,0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7); Error: Access denied..."}
Example
5th:
Call (in pseudo-code):
getAccessTickets(['649'],'0xbf903df49703dd05709a9a3bb542d7229b3049c9',['0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2'],'3046022100897a133ccce335d14eefa12a3fa4194bfa822d44d2b5c944cc7c07ce8485649702210084bd5613415bcce99f2cbb35122745a0d0e49f25ce462c624355a25898852241','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":"Invalid request (wrong method or parameters). For method getAccessTickets(649,0xbf903df49703dd05709a9a3bb542d7229b3049c9,0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2,3046022100897a133ccce335d14eefa12a3fa4194bfa822d44d2b5c944cc7c07ce8485649702210084bd5613415bcce99f2cbb35122745a0d0e49f25ce462c624355a25898852241,0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7); Error: Access denied..."}
Ticket
Description: Method getAISearchAccessTickets
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
dabIds | Array_of_Integer |
buyerId | Address |
sellerIds | Array_of_Address |
argsBuyerSignature | String |
publicKey | String |
Ticket
Description: Method getReadOnlyAccessTickets
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
dabIds | Array_of_Integer |
buyerId | Address |
sellerIds | Array_of_Address |
argsBuyerSignature | String |
publicKey | String |
Ticket
Description: Method getBulkAccessTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
dabId | Integer |
buyerId | Address |
bulkSellerId | Address |
tagId | Integer |
argsBuyerSignature | String |
publicKey | String |
Example
1st:
Call (in pseudo-code):
getBulkAccessTicket('656','0xbf903df49703dd05709a9a3bb542d7229b3049c9','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','654','304402206b05f13c4f0664d1a030f5923c3adad24fe102158afadbadedaa6b8daa65eb1802206df2515ab4466e39e24e6cf3d4201fd5d672751271613ff05a324727e8cd732d','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":{"id":"tid-903358.414322","type":"read","creationTimeInMs":"1579641695946","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-807414","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"30450220291d16dc45d005a98498a970f9ebff0d42c277ea2899ef67684e71b592609c9f0221009570ea0dfab2910a1ce7ebfb1c1b215e1c2cfc931274ba946c1e3a0bad7a04a4"}}
Example
2nd:
Call (in pseudo-code):
getBulkAccessTicket('655','0xbf903df49703dd05709a9a3bb542d7229b3049c9','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','654','304602210094577ab73c6c7623876f32276b7db8f8522c126b025d2aa427e612b1a26477e5022100d0fa5feb1625fca943770caab1de65e8e9dad674a7e71108666c9d3f527f85f1','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":{"id":"tid-960425.610091","type":"read","creationTimeInMs":"1579641710484","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-214791","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"304502210093e488d76642cd375ba3e2d3b3b713a67330ea04fa3749ee036bd25d742f1dc9022023c4e93ffcec85b95b66bddc89b3e92953047c93fee2484f1fbf9b5bba681e45"}}
Example
3th:
Call (in pseudo-code):
getBulkAccessTicket('657','0xbf903df49703dd05709a9a3bb542d7229b3049c9','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','654','30440220261a472d16900c2af03ad6c4ee1d942372f868edfae38f041912c2fd0af125d5022025f645e730968a2d3a28f22f3f6fc6510469d8d9d3e5c5f641eaff1d5b6c38af','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":"Invalid request (wrong method or parameters). For method getBulkAccessTicket(657,0xbf903df49703dd05709a9a3bb542d7229b3049c9,0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2,654,30440220261a472d16900c2af03ad6c4ee1d942372f868edfae38f041912c2fd0af125d5022025f645e730968a2d3a28f22f3f6fc6510469d8d9d3e5c5f641eaff1d5b6c38af,0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7); Error: Access denied. Error: Error: Bulk Access denied..."}
Example
4th:
Call (in pseudo-code):
getBulkAccessTicket('659','0xbf903df49703dd05709a9a3bb542d7229b3049c9','0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','660','3045022100bad63cf5f9e722f881e1db2b93db32cc4db4b6667ada59a815151240c043bb910220067816b1b783276d82f0b58a010b313b25777280ac2390f8f01a0d6dcf33b058','0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7');
Response json-data:
{"result":{"id":"tid-69835.67169","type":"read","creationTimeInMs":"1579641751356","timeToLiveInMs":"1200000","userId":"0xbf903df49703dd05709a9a3bb542d7229b3049c9","assetUrl":"proto://user@addr/path/to/asset","vaultUrl":null,"vaultAuth":null,"nonce":"n-681063","checksum":null,"pubKey":"0xfaa8fb6ab825488989fca408e69f855da2db64e9b1db00f1ad6754bbfb5f5bbafd3ece9683ae9e0a1f7234bd32cef2f6b33528275fdd4b96be709843910255b7","signature":"3044022006fdf71894f96d1d01eda2ecf974a19c71220412e9455728b707317ae11e10460220266db8b3c84d6faa07c25de55a79ea1913d8bd80f9a9c4ec2565f1e508fbd6cf"}}
Ticket
Description: Method getSatAccessTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dabId | Integer | |
buyerId | Address | |
satId | Integer | |
argsBuyerSignature | String | |
publicKey | String | |
apiVersionCode | Integer | (optional. default: 0) |
Ticket
Description: Method getCopyTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
dabId | Integer |
buyerId | Address |
argsBuyerSignature | String |
publicKey | String |
Dab
Description: Method getAccessDab
Kind: global function
Returns: Dab
- Look at Type description in Docs.
Param | Type |
---|---|
dabId | Integer |
buyerId | Address |
sellerId | Address |
argsBuyerSignature | String |
publicKey | String |
Dab
Description: Method getBulkAccessDab
Kind: global function
Returns: Dab
- Look at Type description in Docs.
Param | Type |
---|---|
dabId | Integer |
buyerId | Address |
bulkSellerId | Address |
tagId | Integer |
argsBuyerSignature | String |
publicKey | String |
String
Description: Method encrypt
Kind: global function
Returns: String
- hex
Param | Type |
---|---|
data | Object |
Example
1st:
Call (in pseudo-code):
encrypt({'encodedByVault':'ed1aef1da30d1537a841eef97c3e928124cc5a6bd5e45bb138ec7ff42c713ab65f07f9a42718af31a8e29ef6c1e02e32c70a07ec4577a431137915609604dbca8c03832e1617f8011427306663814e5725526b4e10ebc7fc383131380b0b2ecfc39eadf4','encodedByUserPubKey':'84a26976c410acd8b0fdfd9171f84d9e4ab3b8164688ae657068656d5075626c69634b6579c44104415184b984979831d069d988e11850faeec2d38e5c903a0ccb1beec8f979be300c17e187f9e85f206497865dcde7e444df915b552391e34b4f2c6e13aaf7c75daa63697068657274657874c470a54a90608d6e3ce9c1c5c4186d562d6b0acc85be0010b83297f165ccb67316164e1a618e8b34584e728940e1fa9508743f91af2a9067a72f64406353decf198d24ff4bd73e4748803348df88e2fb0d6dc25920b64467b06cab9f3ac80029334a80f6777cb08475b17f89f79e33ac61f1a36d6163c4206e1ac5ff6e1dbfeec57599a07f60df75f08a43b7addd977d132b8ed9e36b2a0e'});
Response json-data:
{"result":"v2.0|8dc8097d0b0ca20e22fbae13a2390447f8d6362d0dae74abe64230ff1da0a04ad54405e8eccc81fd770f07f2135cb160098533a5dcb8aeaf741510dc8eae3fc20b76299175f314889009ff5b191ea773281c127c05320d64357beacaa0817ce25b362a86d5bba330cfbbab0b6dd98bc474cdd3fc0cf59a358122844b463b09b55b9925b4ea788efd383fce2f74c5b40eef102012ca551b9bd8a732fc981888f2af5c433c21c886695bf99fc818605e0c31ed9f410bb8c1467d384e35d9a297241911bf18bdb87889176848fa09ce13b1b7cb7d4c0c545ebc38badc59850b503f842e26bd48cd2512ade646ba4959647b127910b0fa07e2f0804c76ca2a474fb8b26d6cdd90577a42c564d3c3f29ba0467e399e9fa81f0143209412d109b13d55345b79336ae5e54ae7b403a99241b28019bc5bf1877fead56053e9be513f9f47d8e0160ceeda28e0b0dec5238cf5412d7f495e6539cd917e26c1b93717b1be02f829688d07603a72181b15d332349bb527c616b260d777644610679063d1646ff1dc8b25cecc3ab284bd87964501eaf8d421b56d145d6fb2cb3ac6a89265289b3d2553de8fc1943dc44a5c1793c02f65b87cdcac3a4f117e963d63dbbcd6814eb54433eba846d7d47bda1196e3dfb587ece9fe09b94e93c45dea4fdcec62620e6edf27335a9b90ec54c1a4394db9d15418d422152cb91c6213c4803a1165648f41b471adf480a94678ee0813cf2e4d337722fcc8aa3da0a8635ebf504ba745dac53f22a09a1c53b64c9dee2932b85476a47bd2c803efd661b7160e52a1f55b537eef09188f3e3b7cdfb7212bca67ed25762dd6db2731263f6f36b37b6d75657321e1baea1c33fa6b52c04f0db9ef41abb796bab4cebbdab8abd125dafc05ccdd3a1f2988836a9a9f754131e797b1d8048c2edec5af23410cb2464cc95a13b536926c9ce58f61b915987425234d0bfca45565b805e0fdc94d4c0aab69d1a076934cc6ef4721d36e8599717f56f86d2a1c4ef7f7909c04106c7ad0cb4b1785e40e5ca5dd598284d6b10952c62564fb478b171c479b0c2ce2f99d84d28e3d1aa19e6f0e8d135946145d1d31065a6d14d8f9d65aaa1872da79515f03ae9ec41c8ebe81351e2cb32e1a117392913d63cf30318b7b1881c08b1be85f45f32e0f81191633ad8a098bf7bf38a45b78aafe459e5f112a43ee1359297e99803683bb20f2ada78464d0503c91533614e4c6cbece75df79555c96f8cab2a769ff34e5d1062924211d0badf6dc67be9b12c2fb193a744d593b28b26faa8041fbb091d47e787"}
Boolean
Description: Method setUsp
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userPublicKey | String |
vaultId | Integer |
usp | String |
argsSignature | String |
Example
1st:
Call (in pseudo-code):
setUsp('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','0x86e6f8578e725eb36e856580b1089255c79ecfe06bd79b6a08832be869871b90022c7c134e24c9ca0c36c3372be40fccc6a9875c650a6291b4e2a2eae881a5a1','1','v2.0|8dc8097d0b0ca20e22fbae13a2390447f8d6362d0dae74abe64230ff1da0a04ad54405e8eccc81fd770f07f2135cb160098533a5dcb8aeaf741510dc8eae3fc20b76299175f314889009ff5b191ea773281c127c05320d64357beacaa0817ce25b362a86d5bba330cfbbab0b6dd98bc474cdd3fc0cf59a358122844b463b09b55b9925b4ea788efd383fce2f74c5b40eef102012ca551b9bd8a732fc981888f2af5c433c21c886695bf99fc818605e0c31ed9f410bb8c1467d384e35d9a297241911bf18bdb87889176848fa09ce13b1b7cb7d4c0c545ebc38badc59850b503f842e26bd48cd2512ade646ba4959647b127910b0fa07e2f0804c76ca2a474fb8b26d6cdd90577a42c564d3c3f29ba0467e399e9fa81f0143209412d109b13d55345b79336ae5e54ae7b403a99241b28019bc5bf1877fead56053e9be513f9f47d8e0160ceeda28e0b0dec5238cf5412d7f495e6539cd917e26c1b93717b1be02f829688d07603a72181b15d332349bb527c616b260d777644610679063d1646ff1dc8b25cecc3ab284bd87964501eaf8d421b56d145d6fb2cb3ac6a89265289b3d2553de8fc1943dc44a5c1793c02f65b87cdcac3a4f117e963d63dbbcd6814eb54433eba846d7d47bda1196e3dfb587ece9fe09b94e93c45dea4fdcec62620e6edf27335a9b90ec54c1a4394db9d15418d422152cb91c6213c4803a1165648f41b471adf480a94678ee0813cf2e4d337722fcc8aa3da0a8635ebf504ba745dac53f22a09a1c53b64c9dee2932b85476a47bd2c803efd661b7160e52a1f55b537eef09188f3e3b7cdfb7212bca67ed25762dd6db2731263f6f36b37b6d75657321e1baea1c33fa6b52c04f0db9ef41abb796bab4cebbdab8abd125dafc05ccdd3a1f2988836a9a9f754131e797b1d8048c2edec5af23410cb2464cc95a13b536926c9ce58f61b915987425234d0bfca45565b805e0fdc94d4c0aab69d1a076934cc6ef4721d36e8599717f56f86d2a1c4ef7f7909c04106c7ad0cb4b1785e40e5ca5dd598284d6b10952c62564fb478b171c479b0c2ce2f99d84d28e3d1aa19e6f0e8d135946145d1d31065a6d14d8f9d65aaa1872da79515f03ae9ec41c8ebe81351e2cb32e1a117392913d63cf30318b7b1881c08b1be85f45f32e0f81191633ad8a098bf7bf38a45b78aafe459e5f112a43ee1359297e99803683bb20f2ada78464d0503c91533614e4c6cbece75df79555c96f8cab2a769ff34e5d1062924211d0badf6dc67be9b12c2fb193a744d593b28b26faa8041fbb091d47e787','30460221009de6ae7a4a6a53675b70f401975cc4dd8ee76e59b9a288c16ffa840697e9a0cf022100aedf865aaf30a671d01c88caee6305eda096925b93f217320b92de96be0206fc');
Response json-data:
{"result":true}
String
Description: Method getUsp
Kind: global function
Returns: String
- usp
Param | Type |
---|---|
userId | Address |
vaultId | Integer |
Example
1st:
Call (in pseudo-code):
getUsp('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','1');
Response json-data:
{"result":"v2.0|8dc8097d0b0ca20e22fbae13a2390447f8d6362d0dae74abe64230ff1da0a04ad54405e8eccc81fd770f07f2145ee5395d876df483ebf2fc771d40828af83e950f262d9321af12d8c05bf750191ea773281c127c05320d64357beacaa0817ce25b362a86d5bba330cfbbab0b6dd98bc424c8d7fb50f1c8388c208a444a3509e60fcc27b8b97183f1643c992572cdb40cbc132618ca5416ccd5f23cfa9c1bd9f3f40d423c7dc986375bf2cec3433c0e0938bb9d460dbc98147a634e3e8fa594201c16e71de6bc2b8c463d48fa099945b0e29d764a58555eb16bbd865fd80b076ed52c25ea1d9b2247ffe411bb1c59337c127910b0fa07e2f0804c76ca2a474fb8b26d6cdd90577a42c56486c6f7c2a141713b97c2ab4d0541219914d05db03c0266562b316fb2e01eb4b451f1c112bbd44db858a48377eede3552e8bb0f3b9a47d8e2450db88b21e7e3dcc47e8ba215297315543f33cfc0722494eb614cbcb905ae7c688f5f653b781c1e47816a309bb621944ae433867437174266c765876038fcdddc219b986aea8defd1944001baff8726b53842596fbe973bc7f8956875cc672f018e8f96916dc11c064395c02e6dea2e8da0384e417997683adbe6d4d44bb24460b9ac4dd0d47ade4cc2e688b7d4bfeeae5fb11cc79209b91cdbb06d610f64892c610e9c97ea0b96a4394db9d15418d422152cb91c3747c1d26b42676adb49b322aaf7d0af1729eb001a987a4c667374fa9fac3af3a1690abf0048a3158f956c7daf9f405bb04d9ab27934b30924f47dd1cd06bcd661b7160e52a1f55b537eef09188f3e3b7cdfb7212bca67ed25762dd6db2731263f6f36b37b6d75657321e1baea1c33fa6b52c04f0db9ef41abb796bab4cebbdab8abd125dafc05ccdd3a1f2988836a9a9f754131e797b1df0b8c29de96af234108b7454c935d47b561956a9ce08f65bc139d252521480ff9a1523fb803e0fdce4d495fab6dd4fc769549c5b54321d03282c3767f0df8692a1f4eabf2c4990417377ad6cb481280e1085ca3d85dde85d6b70957c62461f94788174042c80c2bb8fb9a83d28e384da1996f578d10591d115e1d6e5c583746d8f9d65aaa1872da79515f03ae9ec41a85bb87371d2cb32b4e1626c6946965cf3f6488791e8997d84db45547ae2e0a871c4463fc8f57d8f0bb68f55d79a9ad43ca0d4f7045b8155d737c9e8062d4e926a3a0f18930d2006c91016713b797cde6eb09a99755993a8cab7f7198a01a5d18349c4147d0bede3ac12defe47b28b1c7a6148395b68420feaa5219bb0e1d12e787"}
Boolean
Description: Method setAak
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userPublicKey | String |
dabId | Integer |
aak | String |
argsSignature | String |
String
Description: Method getAak
Kind: global function
Returns: String
- aak
Param | Type |
---|---|
dabId | Integer |
Array_of_Integer
Description: Method getVaultsIds
Kind: global function
Returns: Array_of_Integer
- vaultsIds
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Example
1st:
Call (in pseudo-code):
getVaultsIds('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c',0,100);
Response json-data:
{"result":["1","3","4","6","7","9","10","12","13","15","16","18","19"]}
Example
2nd:
Call (in pseudo-code):
getVaultsIds('0xbf903df49703dd05709a9a3bb542d7229b3049c9',13,10);
Response json-data:
{"result":["20"]}
Example
3th:
Call (in pseudo-code):
getVaultsIds('0xbf903df49703dd05709a9a3bb542d7229b3049c9',0,200);
Response json-data:
{"result":["1","3","4","6","7","9","10","12","13","15","16","18","19","21","22"]}
Boolean
Description: Method isVaultedDab
Kind: global function
Returns: Boolean
- isVaulted
Param | Type |
---|---|
dabId | Integer |
Object
Description: Method getVaultById
Kind: global function
Returns: Object
- vault
Param | Type |
---|---|
userId | Address |
vaultId | Integer |
Example
1st:
Call (in pseudo-code):
getVaultById('0xbf903df49703dd05709a9a3bb542d7229b3049c9',1);
Response json-data:
{"result":{"ownerId":"0x003e8C791cb39b4CAD756b9C25431D6eb3Fed85c","pubKey":"0x046525fb685e4c366cea131b4c90f5213e10bceead084a71f3e954e13bfe0b22b3d2b0a29b68d483cc68cd32b361e856dfdc1f2c1189689100f8c7960b336d3cef","name":"DSD Google vault","id":"1","type":"1","url":"https://partner.dase.io:5081","description":"DSD Google vault"}}
Example
2nd:
Call (in pseudo-code):
getVaultById('0xbf903df49703dd05709a9a3bb542d7229b3049c9','20');
Response json-data:
{"result":{"ownerId":"0xB9AC1D430841bC99B6C03E772E6F7d755eFb1dF2","pubKey":"0x046882df9a080352541e74e5a19c25f3ff4dbb72f3edf16d0c7af3f2094dea61ac7caa94c7dc08b212f7b3d8b0a9fc5855aad7cff188583d5129d383e0eb2fc77a","name":"Lively NewVaultTest_0-12-2","id":"20","type":"1","url":"http://104.197.24.49:8085/vex-vault/api/lively/access","description":null}}
Example
3th:
Call (in pseudo-code):
getVaultById('0x003e8c791cb39b4cad756b9c25431d6eb3fed85c','20');
Response json-data:
{"result":{"ownerId":"0xBF903dF49703dD05709A9A3bB542D7229b3049c9","pubKey":"0x7b1fb93f375d0c3fa9d72668fa29287c06a30a2f7515755c1f3c058b3f7ae9edff","name":"name of the vault","id":"20","type":"2","url":null,"description":null}}
Object
Description: Method getVaultManifestById
Kind: global function
Returns: Object
- manifest
Param | Type |
---|---|
vaultBindingId | Integer |
Array_of_Object
Description: Method getVaultManifests
Kind: global function
Returns: Array_of_Object
- manifests
Param | Type | Description |
---|---|---|
userId | Address | |
vaultId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method setVaultManifest
Kind: global function
Returns: Integer
- vaultBindingId
Param | Type | Description |
---|---|---|
vaultBindingId | Integer | (optional. default: 0) |
userId | Address | |
userCredentials | String | |
vaultId | Integer | |
vaultManifest | Object |
(Back)
Boolean
Description: Method newAccount
String
Description: Method getChallenge
BytesArray
Description: Method getEncryptedKeyData
Boolean
Description: Method setUsp
String
Description: Method getUsp
Boolean
Description: Method setAak
String
Description: Method getAak
Boolean
Description: Method newAccount
Kind: global function
Param | Type |
---|---|
account | String |
secret | String |
encryptedKeyData | BytesArray |
String
Description: Method getChallenge
Kind: global function
Param | Type |
---|---|
account | String |
BytesArray
Description: Method getEncryptedKeyData
Kind: global function
Param | Type |
---|---|
account | String |
responseOnChallenge | String |
Boolean
Description: Method setUsp
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userPublicKey | String |
vaultId | Integer |
usp | String |
argsSignature | String |
Example
1st:
Call (in pseudo-code):
setUsp('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','0x86e6f8578e725eb36e856580b1089255c79ecfe06bd79b6a08832be869871b90022c7c134e24c9ca0c36c3372be40fccc6a9875c650a6291b4e2a2eae881a5a1','1','v2.0|8dc8097d0b0ca20e22fbae13a2390447f8d6362d0dae74abe64230ff1da0a04ad54405e8eccc81fd770f07f2135cb160098533a5dcb8aeaf741510dc8eae3fc20b76299175f314889009ff5b191ea773281c127c05320d64357beacaa0817ce25b362a86d5bba330cfbbab0b6dd98bc474cdd3fc0cf59a358122844b463b09b55b9925b4ea788efd383fce2f74c5b40eef102012ca551b9bd8a732fc981888f2af5c433c21c886695bf99fc818605e0c31ed9f410bb8c1467d384e35d9a297241911bf18bdb87889176848fa09ce13b1b7cb7d4c0c545ebc38badc59850b503f842e26bd48cd2512ade646ba4959647b127910b0fa07e2f0804c76ca2a474fb8b26d6cdd90577a42c564d3c3f29ba0467e399e9fa81f0143209412d109b13d55345b79336ae5e54ae7b403a99241b28019bc5bf1877fead56053e9be513f9f47d8e0160ceeda28e0b0dec5238cf5412d7f495e6539cd917e26c1b93717b1be02f829688d07603a72181b15d332349bb527c616b260d777644610679063d1646ff1dc8b25cecc3ab284bd87964501eaf8d421b56d145d6fb2cb3ac6a89265289b3d2553de8fc1943dc44a5c1793c02f65b87cdcac3a4f117e963d63dbbcd6814eb54433eba846d7d47bda1196e3dfb587ece9fe09b94e93c45dea4fdcec62620e6edf27335a9b90ec54c1a4394db9d15418d422152cb91c6213c4803a1165648f41b471adf480a94678ee0813cf2e4d337722fcc8aa3da0a8635ebf504ba745dac53f22a09a1c53b64c9dee2932b85476a47bd2c803efd661b7160e52a1f55b537eef09188f3e3b7cdfb7212bca67ed25762dd6db2731263f6f36b37b6d75657321e1baea1c33fa6b52c04f0db9ef41abb796bab4cebbdab8abd125dafc05ccdd3a1f2988836a9a9f754131e797b1d8048c2edec5af23410cb2464cc95a13b536926c9ce58f61b915987425234d0bfca45565b805e0fdc94d4c0aab69d1a076934cc6ef4721d36e8599717f56f86d2a1c4ef7f7909c04106c7ad0cb4b1785e40e5ca5dd598284d6b10952c62564fb478b171c479b0c2ce2f99d84d28e3d1aa19e6f0e8d135946145d1d31065a6d14d8f9d65aaa1872da79515f03ae9ec41c8ebe81351e2cb32e1a117392913d63cf30318b7b1881c08b1be85f45f32e0f81191633ad8a098bf7bf38a45b78aafe459e5f112a43ee1359297e99803683bb20f2ada78464d0503c91533614e4c6cbece75df79555c96f8cab2a769ff34e5d1062924211d0badf6dc67be9b12c2fb193a744d593b28b26faa8041fbb091d47e787','30460221009de6ae7a4a6a53675b70f401975cc4dd8ee76e59b9a288c16ffa840697e9a0cf022100aedf865aaf30a671d01c88caee6305eda096925b93f217320b92de96be0206fc');
Response json-data:
{"result":true}
String
Description: Method getUsp
Kind: global function
Returns: String
- usp
Param | Type |
---|---|
userId | Address |
vaultId | Integer |
Example
1st:
Call (in pseudo-code):
getUsp('0xb9ac1d430841bc99b6c03e772e6f7d755efb1df2','1');
Response json-data:
{"result":"v2.0|8dc8097d0b0ca20e22fbae13a2390447f8d6362d0dae74abe64230ff1da0a04ad54405e8eccc81fd770f07f2145ee5395d876df483ebf2fc771d40828af83e950f262d9321af12d8c05bf750191ea773281c127c05320d64357beacaa0817ce25b362a86d5bba330cfbbab0b6dd98bc424c8d7fb50f1c8388c208a444a3509e60fcc27b8b97183f1643c992572cdb40cbc132618ca5416ccd5f23cfa9c1bd9f3f40d423c7dc986375bf2cec3433c0e0938bb9d460dbc98147a634e3e8fa594201c16e71de6bc2b8c463d48fa099945b0e29d764a58555eb16bbd865fd80b076ed52c25ea1d9b2247ffe411bb1c59337c127910b0fa07e2f0804c76ca2a474fb8b26d6cdd90577a42c56486c6f7c2a141713b97c2ab4d0541219914d05db03c0266562b316fb2e01eb4b451f1c112bbd44db858a48377eede3552e8bb0f3b9a47d8e2450db88b21e7e3dcc47e8ba215297315543f33cfc0722494eb614cbcb905ae7c688f5f653b781c1e47816a309bb621944ae433867437174266c765876038fcdddc219b986aea8defd1944001baff8726b53842596fbe973bc7f8956875cc672f018e8f96916dc11c064395c02e6dea2e8da0384e417997683adbe6d4d44bb24460b9ac4dd0d47ade4cc2e688b7d4bfeeae5fb11cc79209b91cdbb06d610f64892c610e9c97ea0b96a4394db9d15418d422152cb91c3747c1d26b42676adb49b322aaf7d0af1729eb001a987a4c667374fa9fac3af3a1690abf0048a3158f956c7daf9f405bb04d9ab27934b30924f47dd1cd06bcd661b7160e52a1f55b537eef09188f3e3b7cdfb7212bca67ed25762dd6db2731263f6f36b37b6d75657321e1baea1c33fa6b52c04f0db9ef41abb796bab4cebbdab8abd125dafc05ccdd3a1f2988836a9a9f754131e797b1df0b8c29de96af234108b7454c935d47b561956a9ce08f65bc139d252521480ff9a1523fb803e0fdce4d495fab6dd4fc769549c5b54321d03282c3767f0df8692a1f4eabf2c4990417377ad6cb481280e1085ca3d85dde85d6b70957c62461f94788174042c80c2bb8fb9a83d28e384da1996f578d10591d115e1d6e5c583746d8f9d65aaa1872da79515f03ae9ec41a85bb87371d2cb32b4e1626c6946965cf3f6488791e8997d84db45547ae2e0a871c4463fc8f57d8f0bb68f55d79a9ad43ca0d4f7045b8155d737c9e8062d4e926a3a0f18930d2006c91016713b797cde6eb09a99755993a8cab7f7198a01a5d18349c4147d0bede3ac12defe47b28b1c7a6148395b68420feaa5219bb0e1d12e787"}
Boolean
Description: Method setAak
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userPublicKey | String |
dabId | Integer |
aak | String |
argsSignature | String |
String
Description: Method getAak
Kind: global function
Returns: String
- aak
Param | Type |
---|---|
dabId | Integer |
(Back)
Keys
Keys
Keys
Keys
Keys
Integer
Integer
Boolean
Boolean
Boolean
Transaction
Transaction
Integer
Integer
Integer
Integer
Object
Array_of_Event
Array_of_Event
Keys
Deprecated
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
credentials | String | (optional. default: null) |
Keys
Deprecated
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
credentials | String | (optional. default: null) |
Keys
Deprecated
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
userId | Address | |
credentials | String |
Keys
Deprecated
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
credentials | String |
Keys
Deprecated
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
credentials | String |
Integer
Deprecated
Integer
Deprecated
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
bchainTypeCode | Constant_of_Integer | (optional. default: 1) Look at Constants description in Docs. |
userId | Address | |
credentials | String |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
credentials | String |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
credentials | String |
Transaction
Deprecated
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
senderId | Address |
senderCredentials | String |
silverAmount | Integer |
toUserId | Address |
Transaction
Deprecated
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
senderId | Address |
senderCredentials | String |
goldAmount | Integer |
toUserId | Address |
Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Object
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
offerId | Integer |
Array_of_Event
Deprecated
Kind: global function
Returns: Array_of_Event
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
startBlock | Integer |
Array_of_Event
Deprecated
Kind: global function
Returns: Array_of_Event
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
startBlock | Integer |
(Back)
Integer
Array_of_Integer
Array_of_Integer
Array_of_Integer
Array_of_Integer
Array_of_Integer
Array_of_Tag
Array_of_Dab
Array_of_Dab
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Boolean
Array_of_Dab
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Array_of_License
Boolean
Constant_of_Integer
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Boolean
Integer
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerCredentials | String | |
assetUrl | String | |
publicDescription | String | |
linkedDabId | Integer | (optional. default: 0) |
custodians | Array_of_Address | (optional. default: []) |
dataHash | String | (optional. default: 0) |
Array_of_Integer
Deprecated
Kind: global function
Param | Type |
---|---|
ownerId | Address |
ownerCredentials | String |
dataArr | Array_of_Object |
Array_of_Integer
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 100) |
Array_of_Integer
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Integer
Deprecated
Kind: global function
Param | Type |
---|---|
userId | Address |
Array_of_Tag
Deprecated
Kind: global function
Returns: Array_of_Tag
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
tagIds | Array_of_Integer |
Array_of_Dab
Deprecated
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
rootTagId | Integer | |
tagId | Integer | |
pos | Integer | (optional. default: 0) |
Array_of_Dab
Deprecated
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
Array_of_Dab
Deprecated
Kind: global function
Returns: Array_of_Dab
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Array_of_License
Deprecated
Kind: global function
Returns: Array_of_License
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | (optional. default: null) Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userCredentials | String | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
Constant_of_Integer
Deprecated
Kind: global function
Returns: Constant_of_Integer
- tradeStatusCode Look at Constants description in Docs.
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxLeosPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | (optional. default: 0) |
minPrice | Integer | |
tagId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | (optional. default: 0) |
maxPrice | Integer | |
tagId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
custodianId | Address | |
custodianCredentials | String | |
buyerId | Address | |
dabId | Integer | |
tagId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
buyerTagId | Integer | (optional. default: 0) |
minPrice | Integer | |
sellerDabId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
buyerId | Address | |
buyerCredentials | String | |
buyerTagId | Integer | (optional. default: 0) |
maxPrice | Integer | |
sellerDabId | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
custodianId | Address | |
custodianCredentials | String | |
buyerId | Address | |
buyerTagId | Integer | |
sellerDabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
buyerPubKeyEncryptedTagKey | String | (optional. default: null) |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
sellerId | Address | |
sellerCredentials | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
maxPrice | Integer | |
termSheetTemplate | Constant_of_String | (optional. default: "licenseUnrestrictedTerms") Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodianId | Address |
custodianCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
tagId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
tagId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
tagId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
tagId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
userId | Address |
userCredentials | String |
buyerId | Address |
dabId | Integer |
Boolean
Deprecated
Kind: global function
Returns: Boolean
- isOk
Param | Type |
---|---|
sellerId | Address |
sellerCredentials | String |
buyerId | Address |
dabId | Integer |
(Back)
Integer
Ticket
Integer
Deprecated
Kind: global function
Returns: Integer
- newUsageCount
Param | Type | Description |
---|---|---|
buyerId | Address | |
buyerCredentials | String | |
dabId | Integer | |
licenseTypeCode | Constant_of_Integer | Look at Constants description in Docs. |
terms | Object | (optional. default: null) |
Ticket
Deprecated
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dabId | Integer | |
buyerId | Address | |
sellerId | Address | |
argsBuyerSignature | String | |
publicKey | String | |
vaultAuth | Object | (optional. default: null) |
(Back)
FAQs
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at [app.dase.io](https:*app.dase.io)
The npm package dsd-client-conn-lib receives a total of 106 weekly downloads. As such, dsd-client-conn-lib popularity was classified as not popular.
We found that dsd-client-conn-lib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.