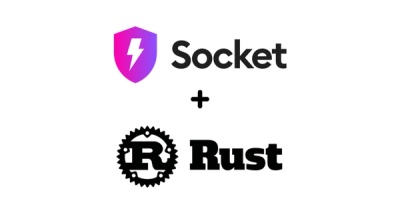
Product
Introducing Rust Support in Socket
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
The duplexer3 npm package is used to create a duplex stream that combines a writable and readable stream into a single stream. This is useful when you need to expose a single stream interface while internally piping data between two separate streams, such as a process's stdin and stdout.
Combining writable and readable streams
This code sample demonstrates how to create a duplex stream by combining a writable and a readable stream using duplexer3. The resulting duplexStream can be used to read from and write to, acting as a bridge between the two streams.
const { Duplex } = require('stream');
const duplexer3 = require('duplexer3');
const writable = new Duplex({
write(chunk, encoding, callback) {
// Write logic here
callback();
}
});
const readable = new Duplex({
read(size) {
// Read logic here
}
});
const duplexStream = duplexer3(writable, readable);
// Now duplexStream can be used as both a writable and readable stream.
Duplexify is similar to duplexer3 in that it also combines a writable and readable stream into a single duplex stream. However, duplexify allows for the streams to be set asynchronously and provides more options for error handling and stream destruction.
Pumpify combines an array of streams into a single duplex stream, similar to duplexer3's functionality of combining two streams. It uses pump for stream error handling, which automatically cleans up the streams if one of them closes or errors.
Stream-combiner2 is another package that combines multiple streams into one. It differs from duplexer3 by allowing more than two streams to be combined and by being a part of a larger stream transformation framework.
Modern version of
duplexer2
npm install duplexer3
import stream from 'node:stream';
import duplexer from 'duplexer3';
const writable = new stream.Writable({objectMode: true});
const readable = new stream.Readable({objectMode: true});
writable._write = function (input, encoding, done) {
if (readable.push(input)) {
done();
} else {
readable.once('drain', done);
}
};
readable._read = function () {
// Noop
};
// Simulate the readable thing closing after a bit
writable.once('finish', () => {
setTimeout(() => {
readable.push(null);
}, 500);
});
const duplex = duplexer3(writable, readable);
duplex.on('data', data => {
console.log('got data', JSON.stringify(data));
});
duplex.on('finish', () => {
console.log('got finish event');
});
duplex.on('end', () => {
console.log('got end event');
});
duplex.write('oh, hi there', () => {
console.log('finished writing');
});
duplex.end(() => {
console.log('finished ending');
});
got data 'oh, hi there'
finished writing
got finish event
finished ending
got end event
Type: object
Type: boolean
Default: true
Whether to bubble errors from the underlying readable/writable streams.
FAQs
Modern version of `duplexer2`
The npm package duplexer3 receives a total of 5,881,138 weekly downloads. As such, duplexer3 popularity was classified as popular.
We found that duplexer3 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.