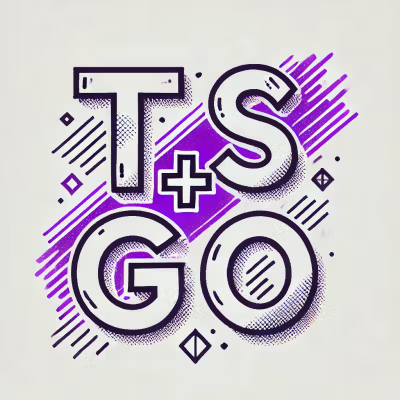
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
A Node.js implementation of EDHOC (Ephemeral Diffie-Hellman Over COSE) protocol for lightweight authenticated key exchange in IoT and other constrained environments.
A TypeScript Node.js library implemented as a native addon, built on top of the C library libedhoc
. It provides an efficient and lightweight way to use the Ephemeral Diffie-Hellman Over COSE (EDHOC) protocol, as specified in RFC 9528.
EDHOC is designed for lightweight communication and is particularly suitable for protocols like CoAP and OSCORE, but can work independently of the application and transport layers, ensuring minimal overhead while maintaining strong security guarantees. The library provides a default software implementation for X.509 credentials, with support for additional formats such as C509, CWT, and CCS coming soon. It also includes a software-based cryptographic implementation utilizing @noble/curves
. Additionally, it exposes credential and cryptographic API interfaces to allow for custom implementations, such as PKCS#11-based solutions.
libedhoc
, a proven C implementationInstall the package via npm:
npm install edhoc
The simplest EDHOC handshake using pre-shared keys (Method 0):
import { EDHOC, EdhocMethod, EdhocSuite } from 'node-edhoc';
// ...
const initiator = new EDHOC(10, [ EdhocMethod.Method0 ], [ EdhocSuite.Suite0 ], credentialsManager, cryptoManager);
const responder = new EDHOC(20, [ EdhocMethod.Method0 ], [ EdhocSuite.Suite0 ], credentialsManager, cryptoManager);
// Message 1: Initiator → Responder
const message1 = await initiator.composeMessage1();
await responder.processMessage1(message1);
// Message 2: Responder → Initiator
const message2 = await responder.composeMessage2();
await initiator.processMessage2(message2);
// Message 3: Initiator → Responder
const message3 = await initiator.composeMessage3();
await responder.processMessage3(message3);
// ...
You can include additional authorization data in EDHOC messages:
// initiator.js
const ead_1 = [{
label: 1000,
value: Buffer.from('External Data')
}];
const message1 = await initiator.composeMessage1(ead_1);
// responder.js
const receivedEAD = await responder.processMessage1(message1);
Using X.509 certificates for authentication:
import {
EDHOC,
X509CertificateCredentialManager,
DefaultEdhocCryptoManager
} from 'node-edhoc';
// Setup credential managers
const initiatorCreds = new X509CertificateCredentialManager(
[initiatorCert],
initiatorKeyID
);
initiatorCreds.addTrustedCA(trustedCA);
// Setup crypto managers
const initiatorCrypto = new DefaultEdhocCryptoManager();
// Initialize EDHOC with certificate-based auth
const initiator = new EDHOC(
10,
[ EdhocMethod.Method0 ],
[ EdhocSuite.Suite2 ],
initiatorCreds,
initiatorCrypto
);
After a successful handshake, you can export the OSCORE security context:
const initiatorContext = await initiator.exportOSCORE();
const responderContext = await responder.exportOSCORE();
console.log('Master Secret:', initiatorContext.masterSecret);
console.log('Master Salt:', initiatorContext.masterSalt);
console.log('Sender ID:', initiatorContext.senderId);
console.log('Recipient ID:', initiatorContext.recipientId);
Perform a key update for an existing OSCORE context:
const keyUpdateContext = Buffer.from('new-entropy-data');
// Update keys for both parties
await initiator.keyUpdate(keyUpdateContext);
await responder.keyUpdate(keyUpdateContext);
// Export new OSCORE context
const newContext = await initiator.exportOSCORE();
Export application-specific keys:
// Export a 32-byte key with label 40001
const key = await initiator.exportKey(40001, 32);
For more detailed examples and API documentation, please refer to our API Documentation.
For detailed documentation, refer to:
Contributions are welcome! To contribute:
Please ensure your code follows the existing style and structure of the project.
This project is licensed under the MIT License.
This implementation is based on the EDHOC specification as defined in RFC 9528. Special thanks to the developers of libedhoc
for their foundational work on EDHOC in C.
FAQs
A Node.js implementation of EDHOC (Ephemeral Diffie-Hellman Over COSE) protocol for lightweight authenticated key exchange in IoT and other constrained environments.
We found that edhoc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.