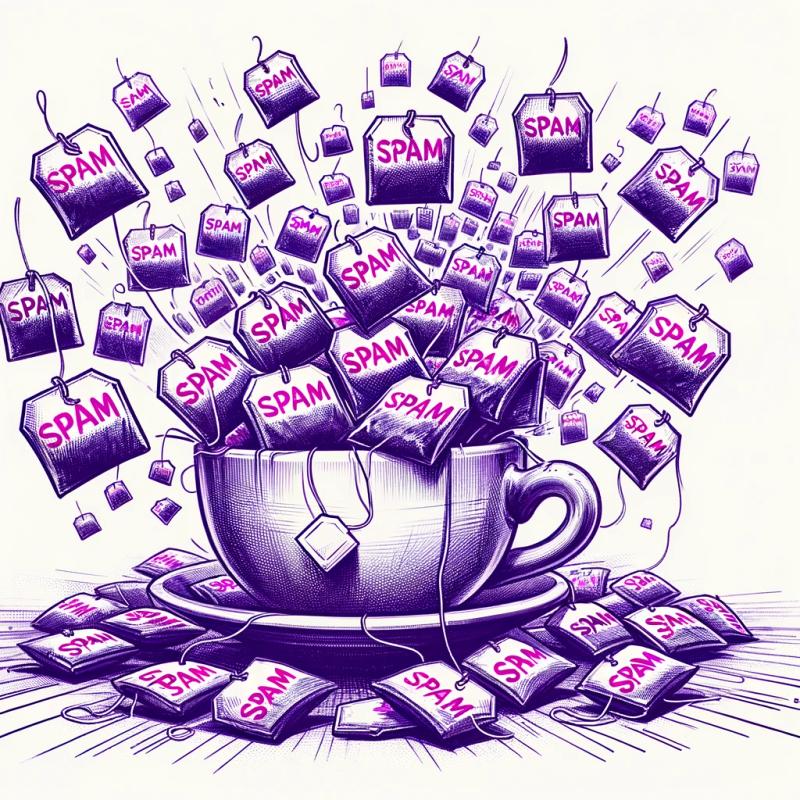
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
electron-event-dispatcher
Advanced tools
Readme
Helps you to organize communication between BrowserWindow
s, main/renderer IPCs,
Sockets or any other EventEmitter
s in your Electron applications.
NPM:
$ npm install electron-event-dispatcher
Yarn:
$ yarn add electron-event-dispatcher
lib/device-events-dispatcher.js
const EventDispatcher = require('electron-event-dispatcher');
class DeviceEventsDispatcher extends EventDispatcher {
constructor(driver, ipcMain) {
super();
// driver event handlers
this.connect(driver, {
connected: () => {
// send `device-connected` event to all bound windows
this.broadcast('device-connected');
},
disconnected: () => {
// send `device-disconnected` event to all bound windows
this.broadcast('device-disconnected');
},
data: (data) => {
// send device data to all bound windows
this.broadcast('device-data-received', data);
}
});
// windows' (renderer) event handlers
this.connect(ipcMain, {
'get-device-state': (event) => {
// respond to the requesting browser window
event.sender.send('device-state', {
connected: driver.connected || false
});
}
});
}
/**
* Override start/stop if you want to add some pre/post hooks.
* @return {Promise}
*/
start() {
// add some pre-start logic...
return super.start()
.then(() => {
// add some post-start logic...
});
}
/**
* Override start/stop if you want to add some pre/post hooks.
* @return {Promise}
*/
stop() {
// add some pre-stop logic...
return super.stop()
.then(() => {
// add some post-stop logic...
});
}
}
module.exports = DeviceEventsDispatcher;
app/main.js
const {app, BrowserWindow, ipcMain} = require('electron');
const DeviceEventsDispatcher = require('../lib/device-events-dispatcher');
const DeviceDriver = require('../lib/device-driver'); // an example event emitter
let mainWindow;
let settingsWindow;
let deviceEventsDispatcher;
function createMainWindow() {
mainWindow = new BrowserWindow({ ... });
mainWindow.loadUrl('../main.html');
deviceEventsDispatcher.attach(mainWindow);
mainWindow.on('closed', () => {
mainWindow = null;
});
}
function createSettingsWindow() {
settingsWindow = new BrowserWindow({ ... });
settingsWindow.loadUrl('../settings.html');
deviceEventsDispatcher.attach(settingsWindow);
settingsWindow.on('closed', () => {
settingsWindow = null;
});
}
app.on('ready', () => {
const driver = new DeviceDriver();
deviceEventsDispatcher = new DeviceEventsDispatcher(driver, ipcMain);
deviceEventsDispatcher.start()
.then(() => createMainWindow());
});
app.on('window-all-closed', () => {
deviceEventsDispatcher.stop()
.then(() => {
if (process.platform !== 'darwin') {
app.quit();
}
});
});
app.on('activate', () => {
deviceEventsDispatcher.start()
.then(() => createMainWindow());
});
An instance of EventDispatcher
is a unit that represents an event hub between
electron's BrowserWindow
s and any EventEmitter
s (electron's ipc, tcp/web sockets, device drivers, etc.).
#attach(window)
Attach a BrowserWindow
instance to the event dispatcher.
Attached windows will receive all #broadcast()
ing events.
Param | Type | Description |
---|---|---|
window | BrowserWindow | Electron's BrowserWindow instance. |
#detach(window)
Detach a given window
from the event dispatcher.
Technically, removes a given window
reference from
the attached windows collection to give'em be garbage collected.
Note that windows are detached automatically on
closed
.
Param | Type | Description |
---|---|---|
window | BrowserWindow | Electron's BrowserWindow instance. |
#broadcast(event, [...args])
Broadcast an event to all attached windows.
Param | Type | Description |
---|---|---|
event | String | The name of the event to broadcast. |
[...args] | EventEmitter | Any number of event-related arguments. |
#connect(emitter, handlers)
Connect event emitter handlers in the context of this dispatcher.
Param | Type | Description |
---|---|---|
emitter | EventEmitter | An event emitter instance. |
handlers | Object<String, Function> | An event => function object map, where event is a name of event to handle and function is a handler of this event. |
#disconnect(emitter)
Disconnect event emitter handlers from this dispatcher.
Param | Type | Description |
---|---|---|
emitter | EventEmitter | An event emitter instance. |
#start()
⇒ Promise
Start the event dispatcher. Binds all #connect()
ed event emitters' handlers.
#stop()
⇒ Promise
Stop the event dispatcher. Unbinds all #connect()
ed event emitters' handlers.
#restart()
⇒ Promise
Restart the event dispatcher.
#isRunning
⇒ boolean
Returns true
if the event dispatcher is running.
Feel free to dive in! Open an issue or submit PRs.
In your terminal run:
$ npm test
or
$ yarn test
Licensed under MIT © 2017 Holy Krab Labs
FAQs
Event Dispatcher Concept for Electron Apps
The npm package electron-event-dispatcher receives a total of 3 weekly downloads. As such, electron-event-dispatcher popularity was classified as not popular.
We found that electron-event-dispatcher demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.