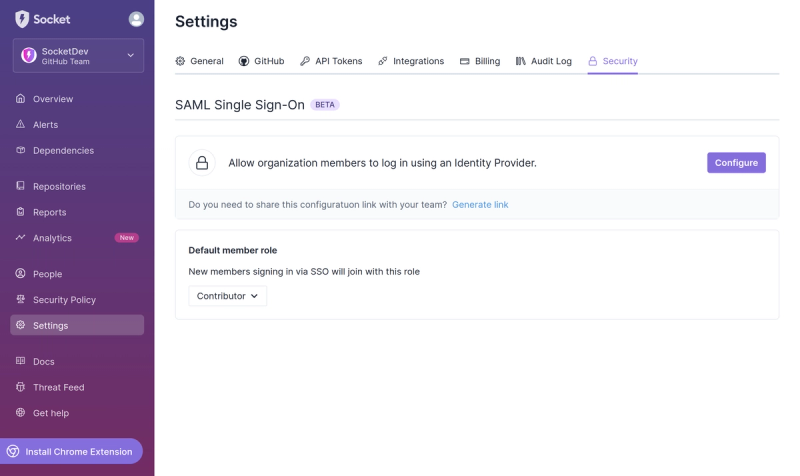
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
energize.js
Advanced tools
Readme
An assets loader that loads everything. Say you have some third party libraries with their own loader modules, you can pipe the loading progress into energize's loading pipeline. It also does basic loading for image
, json
, jsonp
, text
, video
and audio
.
Add several assets to the loader and get the percent of the batch loading.
// assuming you are using CommonJS
var energize = require('energize');
// load the asset with a certain type
energize.add('1.jpg', {type: 'image'});
// or let it guess the type by the url extension
energize.add('2.jpg');
// you can also define the weight of the asset which is 1 by default
energize.add('3.jpg', {weight: 2});
energize.start(function(percent) {
// assuming the files are loaded in the same order as above
// it will be logged as 0.25, 0.5, 1.0
console.log(percent);
if (percent === 1) {
init();
// the listender was removed at this point and it
// will not have any stacked async issues so you can
// load something else again.
energize.add(...);
energize.start(...);
}
});
You can add an onLoad callback to an individual asset.
energize.add('data.json', {
onLoad: function(data) {
console.log(data);
}
});
energize.add('img.jpg');
energize.start(...);
For all features that work with batch loading, it works with individual asset loading as well. Basically all you need is to change the add()
into load()
energize.load('data.json', {
onLoad: function(data) {
console.log(data);
}
});
Sometimes when you add the loading query to the batch loader, you want to have access to the asset instance immediately. This feature only works with asset type: image
, video
and audio
var img = energize.add('img.jpg').content;
energize.start(...);
energize.load('mesh.json', {
type: 'any',
loadFunc: function(url, cb) {
var loader = new THREE.JSONLoader();
loader.load(url, function(geometry, material) {
var mesh = new THREE.Mesh(geometry, material);
// tell energize the item is loaded and store
// the mesh instead as content
cb(mesh);
});
}
});
Normally after an item is loaded, the content will be stored and if you fetch the same url, it will not download the content again. But you can set noCache
to true in the item config and bypass this feature. It will remove the reference after the file is loaded.
energize.load('img.jpg', {
noCache: true
});
You can also add a listener to the individual asset. This feature only works with asset types json,
textand
any`.
energize.add('data.json', {
type: 'json',
weight: 5,
hasLoading: true,
onLoading: function(percent) {
console.log(percent);
}
});
This following example is to show you when you are using any
asset type, you can do whatever you want.
energize.add('a_fake_loader', {
type: 'any',
weight: 50,
hasLoading: true,
onLoading: function(percent) {
console.log('loading: ' + ~~(percent * 100) + '%');
},
onLoad: function(content) {
// some content here
console.log('loaded: ' + content);
},
loadFunc: function(url, cb, loadingSignal) {
var count = 0;
var interval = setInterval(function() {
count++;
loadingSignal.dispatch(count / 10);
if (count == 10) {
clearInterval(interval);
cb('some content here');
}
}, 100);
}
});
energize.addChunk(['1.jpg', '2.jpg', '3.jpg'], 'image');
// let energize guess the types
energize.addChunk(['1.jpg', '2.jpg', '3.jpg']);
It adds all images through the image tag and background images.
energize.addChunk(document.body.querySelectorAll('*'));
For some reason if you want to have 2 loaders, you can create a new one like this:
var energize = require('energize');
var batchLoader = energize.create();
batcherLoader.add(...);
var energize = require('energize');
// set everything to be cross-origin within a domain
energize.setCrossOrigin('http:///mydomain/', 'anonymous')
// set cross-origin for individual load item
energize.add('http://anotherdomain/image.jpg,', {
crossOrigin: 'anonymous'
})
Download the standalone version HERE
npm install energize.js
FAQs
An asset loader that loads everything
The npm package energize.js receives a total of 2 weekly downloads. As such, energize.js popularity was classified as not popular.
We found that energize.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.