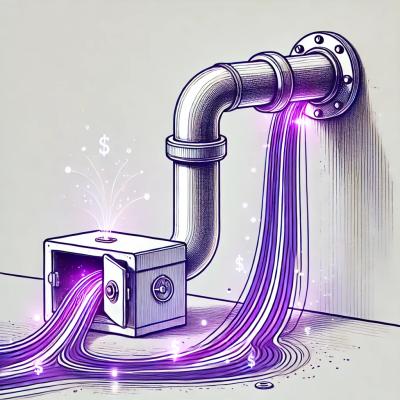
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A TypeScript/JavaScript library to query IP addresses using the ipquery.io API.
A TypeScript/JavaScript library to query IP addresses using the ipquery.io API. This package provides detailed information about IP addresses, including ISP details, geolocation, and risk assessment.
axios
for HTTP requests.Install the package using npm:
npm install enrichip
Or using yarn:
yarn add enrichip
You can import the package using ES Modules:
import { queryIP, queryOwnIP, queryBulk } from 'enrichip';
Or using CommonJS:
const { queryIP, queryOwnIP, queryBulk } = require('enrichip');
Retrieve detailed information about a specific IP address:
import { queryIP } from 'enrichip';
async function getIPInfo() {
try {
const ipInfo = await queryIP('8.8.8.8');
console.log(ipInfo);
} catch (error) {
console.error('Error fetching IP info:', error);
}
}
getIPInfo();
{
"ip": "8.8.8.8",
"isp": {
"asn": "AS15169",
"org": "Google LLC",
"isp": "Google LLC"
},
"location": {
"country": "United States",
"country_code": "US",
"city": "Mountain View",
"state": "California",
"zipcode": "94035",
"latitude": 37.386,
"longitude": -122.0838,
"timezone": "America/Los_Angeles",
"localtime": "2024-11-09T12:45:32"
},
"risk": {
"is_mobile": false,
"is_vpn": false,
"is_tor": false,
"is_proxy": false,
"is_datacenter": true,
"risk_score": 0
}
}
Retrieve the public IP address of the machine running your code:
import { queryOwnIP } from 'enrichip';
async function getOwnIP() {
try {
const ip = await queryOwnIP();
console.log('Your IP:', ip);
} catch (error) {
console.error('Error fetching own IP:', error);
}
}
getOwnIP();
Your IP: 203.0.113.45
Fetch details for multiple IP addresses in one go:
import { queryBulk } from 'enrichip';
async function getBulkIPInfo() {
try {
const ips = ['8.8.8.8', '1.1.1.1'];
const results = await queryBulk(ips);
console.log(results);
} catch (error) {
console.error('Error fetching bulk IP info:', error);
}
}
getBulkIPInfo();
[
{
"ip": "8.8.8.8",
"isp": { "asn": "AS15169", "org": "Google LLC", "isp": "Google LLC" },
"location": { "country": "United States", "city": "Mountain View" }
},
{
"ip": "1.1.1.1",
"isp": { "asn": "AS13335", "org": "Cloudflare, Inc.", "isp": "Cloudflare" },
"location": { "country": "Australia", "city": "Sydney" }
}
]
queryIP(ip: string): Promise<IPInfo>
Fetches detailed information about a specific IP address.
ip
: The IP address to query.IPInfo
object.queryOwnIP(): Promise<string>
Fetches the public IP address of the current machine.
queryBulk(ips: string[]): Promise<IPInfo[]>
Fetches information for multiple IP addresses.
ips
: An array of IP addresses to query.IPInfo
objects.IPInfo
interface ISPInfo {
asn?: string;
org?: string;
isp?: string;
}
interface LocationInfo {
country?: string;
country_code?: string;
city?: string;
state?: string;
zipcode?: string;
latitude?: number;
longitude?: number;
timezone?: string;
localtime?: string;
}
interface RiskInfo {
is_mobile?: boolean;
is_vpn?: boolean;
is_tor?: boolean;
is_proxy?: boolean;
is_datacenter?: boolean;
risk_score?: number;
}
interface IPInfo {
ip: string;
isp?: ISPInfo;
location?: LocationInfo;
risk?: RiskInfo;
}
To run the tests, use:
npm test
Ensure that ts-node
and jest
are properly configured before running the tests.
This project is licensed under the MIT License. See the LICENSE file for details.
FAQs
A TypeScript/JavaScript library to query IP addresses using the ipquery.io API.
We found that enrichip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.