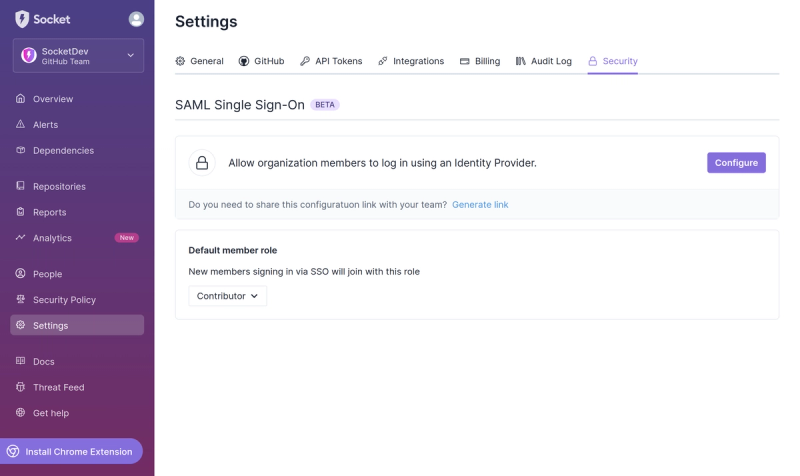
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
eris-contracts-promise
Advanced tools
Readme
This library is built to be able to work with Eris Contracts using JS and Promises
You will need ErisDB installed and running Also this library is using ES6 so you will have to use node.js > 4.0.0
$ npm i --save eris-contracts-promise
First of all to be able to use this module you have to have your account:
On most of Linux based OS you will find them into ~/.eris/chains/<your-chain-name>/priv_validator.json
Finding out ErisDB IP
$ eris chains inspect <name of ErisDB server> NetworkSettings.IPAddress
After that you will be able to create new instance of library:
const ContractContent = `
contract GetSetContract {
uint public something;
function setSomething(uint newSome) {
something = newSome;
}
}
`
const ErisContracts = require('eris-contracts-promise')
// Your ErisRPC url
const rpcUrl = 'http://localhost:1337/rpc'
// Account details
const account = {
address: 'someaddress',
privKey: 'accountPrivateKey',
pubKey: 'accountPublicKey'
}
const ContractManager = ErisContracts.newContractManager(rpcUrl, account)
/**
* You have to compile your contract before using it.
*/
const compiled = require('solc').compile(ContractContent, 1).contracts['ContractName']
const abi = JSON.parse(compiled.interface)
// Create contract instance
const SomeContract = ContractManager.newContract(abi, compiled.bytecode.toUpperCase())
// Now we could work with newly created contract
SampleContract.at('address')
.then((contract) => {
// And call it's functions
return contract
.setSomething(1)
.then(() => {
//new value set
})
})
For more information please take a look into test
folder. You will find more examples in there.
FAQs
This library is built to be able to work with Eris Contracts using JS and Promises
The npm package eris-contracts-promise receives a total of 7 weekly downloads. As such, eris-contracts-promise popularity was classified as not popular.
We found that eris-contracts-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.