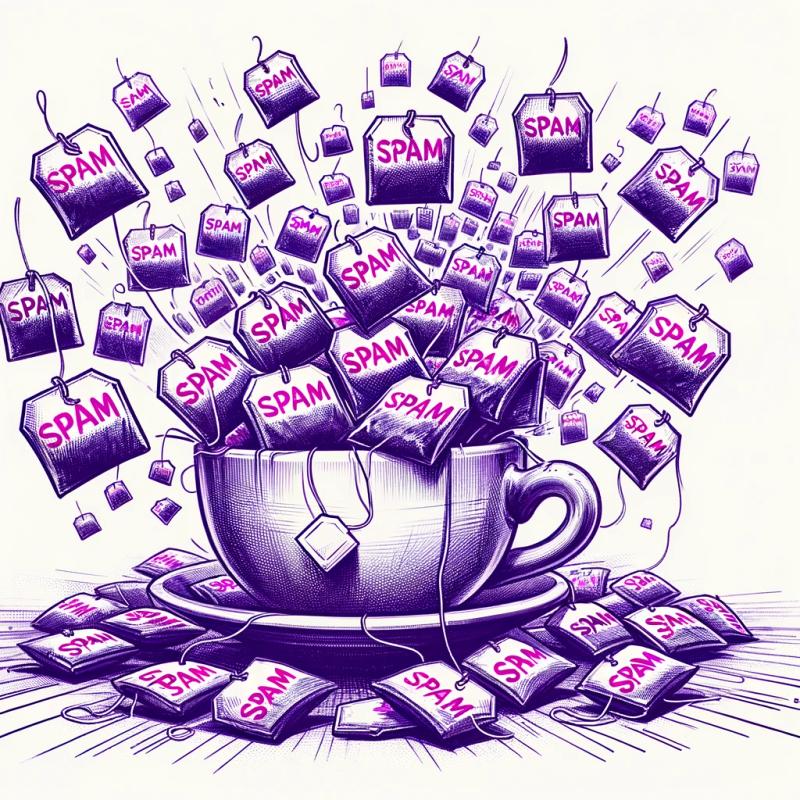
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
error-generator
Advanced tools
Readme
Error Generator is an error generation node module for easily creating and managing errors you plan on using in your application. One common use case for Error Generator is creating errors which are sent in an Express JS response. Error Generator is lightweight, fast, and has no dependencies.
Error Generator provides the following functionality:
npm install --save error-generator
// Registering a new error
const errorGenerator = require('error-generator');
errorGenerator.registerError('not_found', {
status_code: 404,
message: 'The requested resource was not found on the server.',
use_source: true
});
// Sending an error in an Express route
const express = require('express');
let app = express();
app.use(errorGenerator.errorGenerator);
app.get('/', (req, res) => {
res.sendError('not_found', {
source: {
parameter: 'email_address',
value: null
}
});
});
// Returns
{
"name": "not_found",
"status_code": 404,
"message": "The requested resource was not found on the server.",
"source": [{
parameter: 'email_address',
value: null
}]
}
Registers a new error with the given name and options.
name - Name of the error to register
options - Options that can be used for the error
new Date()
internally)Returns a registered error with its options if the error exists. Returns undefined
otherwise.
Attaches a callback to an error, which will be called with the return value of the createError
call. Can be called multiple times to add more callbacks to the error.
createError
Detaches a callback from an error so that it will no longer call on invocation of createError
. If all callbacks are detached from an error, the callback property will be removed.
Note: If you plan on removing a callback from an error make sure to keep a reference to it. We remove callbacks based on an equal reference using ==
.
Clears an error out of the error list. Returns the error that was removed.
Clears all of the errors out of the error list.
Creates the error object that will be sent in the response of the Express response. In the case that you don't use Express, you can always use this method to create the error to send yourself.
name - Name of the error to create
[options] - Options that can be used for the error
Note: If you plan on attaching a source of undefined, it is recommended to use arrays to avoid any complication. One common use-case for this is when a user calls an API point with missing data.
Creates multiple errors using createError
repeatedly internally. Useful when, for example, a user forgets to submit both their email address and password and you want to display an error for each.
errors - Array of valid error objects that will each pass through createError
on their own
options - Options that can be attached to the errors
Attaches the function sendError
to an Express response. Should be used when first setting up the Express application. See the example above for usage.
Follows the same API as createError
, see above. Sends the response with the status code attached to the error along with the contents of the error.
Also follows the API of bulkCreateErrors
, which means that you can either send one or multiple errors. The options in this case follow the options of bulkCreateErrors
, and the status code used for the overall error will be used at the status code of the response.
This project was built using Babel Stage 2. I am open to anyone who wishes to fork the project and create new test cases, add useful functionality, or anything else. I use this project in my own work so I will be adding to this library as functionality arises that I need.
All I ask is that if you add functionality please provide the necessary test cases and try to get as high code coverage as possible.
I am currently interested in adding more Express middleware for doing validation of request data and sending Error Generator errors in the case of a failure. My ideal setup would look something like:
// Error middleware
const router = require('express').router;
const errorGenerator = require('error-generator');
router.get(
'/',
errorGenerator.verify({
params: [{
name: 'user_id',
number: true,
error: 'invalid_parameter'
}],
body: [{
name: 'first_name',
text: true,
error: 'invalid_parameter'
}]
}),
function updateUser(req, res) { ... }
)
FAQs
Easily create and manage errors for any API.
The npm package error-generator receives a total of 12 weekly downloads. As such, error-generator popularity was classified as not popular.
We found that error-generator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.